Implementing the Hamiltonian Cycle Algorithm: A Comprehensive Guide
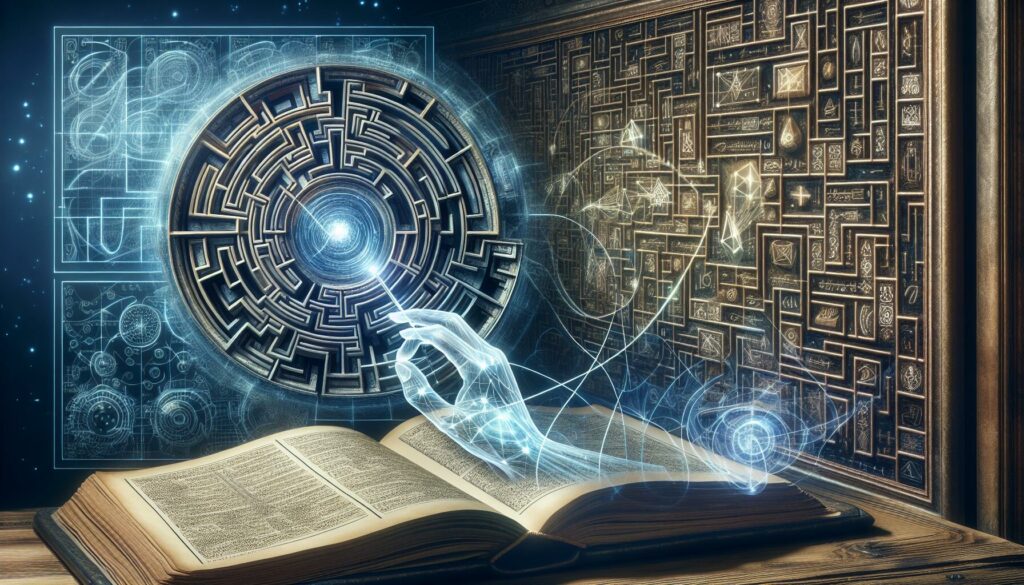
In the world of computer science and algorithmic problem-solving, the Hamiltonian Cycle algorithm stands out as a fascinating and challenging concept. This algorithm, named after the renowned mathematician William Rowan Hamilton, plays a crucial role in various applications, from network design to optimization problems. In this comprehensive guide, we’ll dive deep into the Hamiltonian Cycle algorithm, exploring its implementation, applications, and significance in the realm of coding education and programming skills development.
What is a Hamiltonian Cycle?
Before we delve into the implementation details, let’s first understand what a Hamiltonian Cycle is. In graph theory, a Hamiltonian Cycle is a cycle in an undirected or directed graph that visits each vertex exactly once and returns to the starting vertex. In other words, it’s a path that starts and ends at the same vertex, passing through every other vertex in the graph exactly once.
The concept might seem simple, but finding a Hamiltonian Cycle in a graph is actually a complex problem. In fact, determining whether a graph contains a Hamiltonian Cycle is NP-complete, which means there’s no known polynomial-time algorithm to solve it for all cases.
The Importance of the Hamiltonian Cycle Algorithm
Understanding and implementing the Hamiltonian Cycle algorithm is crucial for several reasons:
- Problem-Solving Skills: It helps develop strong algorithmic thinking and problem-solving abilities, which are essential for coding interviews and real-world programming challenges.
- Graph Theory Applications: Many real-world problems can be modeled as graph problems, and the Hamiltonian Cycle is a fundamental concept in graph theory.
- Optimization Problems: The algorithm is used in various optimization problems, including the famous Traveling Salesman Problem.
- Network Design: It has applications in network design and analysis, particularly in designing efficient routing protocols.
Implementing the Hamiltonian Cycle Algorithm
Now, let’s dive into the implementation of the Hamiltonian Cycle algorithm. We’ll use a backtracking approach, which is one of the most common methods to solve this problem. The algorithm will try different paths and backtrack when it reaches a dead end.
Here’s a step-by-step implementation in Python:
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[0 for column in range(vertices)]
for row in range(vertices)]
def is_safe(self, v, pos, path):
# Check if this vertex is an adjacent vertex of the previously added vertex
if self.graph[path[pos-1]][v] == 0:
return False
# Check if the vertex has already been included in the path
if v in path:
return False
return True
def hamiltonian_cycle_util(self, path, pos):
# Base case: If all vertices are included in the path
if pos == self.V:
# Check if there is an edge from the last vertex to the first vertex
if self.graph[path[pos-1]][path[0]] == 1:
return True
else:
return False
# Try different vertices as the next candidate in Hamiltonian Cycle
for v in range(1, self.V):
if self.is_safe(v, pos, path):
path[pos] = v
if self.hamiltonian_cycle_util(path, pos+1):
return True
# If adding vertex v doesn't lead to a solution, remove it
path[pos] = -1
return False
def hamiltonian_cycle(self):
path = [-1] * self.V
path[0] = 0 # Start from the first vertex
if not self.hamiltonian_cycle_util(path, 1):
print("Solution does not exist")
return False
self.print_solution(path)
return True
def print_solution(self, path):
print("Hamiltonian Cycle exists:")
for vertex in path:
print(vertex, end=" ")
print(path[0])
# Driver code
if __name__ == "__main__":
g = Graph(5)
g.graph = [
[0, 1, 0, 1, 0],
[1, 0, 1, 1, 1],
[0, 1, 0, 0, 1],
[1, 1, 0, 0, 1],
[0, 1, 1, 1, 0]
]
g.hamiltonian_cycle()
This implementation uses a Graph class to represent the graph and includes methods to check if a vertex can be added to the path (is_safe
), find the Hamiltonian Cycle (hamiltonian_cycle_util
), and print the solution.
Understanding the Algorithm
Let’s break down the key components of this implementation:
- Graph Representation: The graph is represented as an adjacency matrix, where
graph[i][j] = 1
if there’s an edge between vertices i and j, and 0 otherwise. - Backtracking: The
hamiltonian_cycle_util
method uses backtracking to explore different paths. It tries adding vertices to the path and backtracks if a solution is not found. - Safety Check: The
is_safe
method checks if it’s safe to add a vertex to the path at a given position. It ensures that the vertex is adjacent to the previous vertex and hasn’t been included in the path before. - Solution Verification: The algorithm checks if a complete cycle is formed by verifying if there’s an edge from the last vertex back to the starting vertex.
Time Complexity Analysis
The time complexity of this backtracking algorithm for finding a Hamiltonian Cycle is O(n!), where n is the number of vertices in the graph. This is because, in the worst case, we might need to explore all possible permutations of vertices.
While this exponential time complexity might seem daunting, it’s important to note that for many practical problems and specific graph structures, the algorithm can perform much better. Additionally, various heuristics and optimizations can be applied to improve performance for specific use cases.
Applications of the Hamiltonian Cycle Algorithm
Understanding and implementing the Hamiltonian Cycle algorithm opens up a world of applications and related problems. Here are some areas where this algorithm finds practical use:
- Traveling Salesman Problem (TSP): The Hamiltonian Cycle is closely related to the TSP, where the goal is to find the shortest possible route that visits each city exactly once and returns to the starting city.
- Network Design: In computer networks, finding Hamiltonian Cycles can help in designing efficient routing protocols and network topologies.
- DNA Fragment Assembly: In bioinformatics, Hamiltonian Cycles are used in algorithms for DNA fragment assembly.
- Game Design: Certain puzzle games, like the classic “Knight’s Tour” problem, are based on finding Hamiltonian paths or cycles.
- Operations Research: The algorithm is used in various optimization problems in operations research and logistics.
Variations and Related Concepts
As you delve deeper into the world of graph algorithms, you’ll encounter several concepts related to the Hamiltonian Cycle:
- Hamiltonian Path: Similar to a Hamiltonian Cycle, but doesn’t require returning to the starting vertex.
- Eulerian Cycle: A cycle that traverses every edge in a graph exactly once.
- Graph Coloring: The problem of assigning colors to vertices of a graph such that no two adjacent vertices have the same color.
- Vertex Cover: Finding a set of vertices that includes at least one endpoint of every edge of the graph.
Optimizations and Heuristics
While the basic backtracking algorithm we’ve implemented works well for small graphs, it can become impractical for larger instances. Here are some ways to optimize the algorithm:
- Branch and Bound: This technique can help prune the search space by eliminating paths that are guaranteed not to lead to a solution.
- Genetic Algorithms: For approximate solutions, genetic algorithms can be used to find near-optimal Hamiltonian Cycles in large graphs.
- Ant Colony Optimization: This nature-inspired algorithm can be effective for finding good solutions to the Hamiltonian Cycle problem in certain types of graphs.
- Dynamic Programming: For certain classes of graphs, dynamic programming approaches can be more efficient than backtracking.
Challenges and Learning Opportunities
Implementing and understanding the Hamiltonian Cycle algorithm presents several challenges and learning opportunities for programmers:
- Algorithmic Thinking: It requires deep understanding of graph theory and recursive algorithms.
- Optimization Skills: Improving the basic algorithm for better performance on large graphs is a great exercise in algorithm optimization.
- Problem Modeling: Learning to recognize real-world problems that can be modeled as Hamiltonian Cycle problems is a valuable skill.
- Complexity Analysis: Understanding why this problem is NP-complete and what that means in practice is crucial for computer science education.
Integrating Hamiltonian Cycle in Coding Education
For platforms like AlgoCademy that focus on coding education and preparing for technical interviews, the Hamiltonian Cycle algorithm serves as an excellent topic for several reasons:
- Graph Theory Fundamentals: It helps reinforce understanding of graph representations and traversals.
- Backtracking Paradigm: The algorithm is a classic example of the backtracking technique, which is crucial for many interview problems.
- Complexity Analysis: It provides a concrete example to discuss time complexity and the concept of NP-completeness.
- Real-world Applications: Discussing its applications helps students understand the relevance of theoretical concepts in practical scenarios.
- Problem-Solving Skills: Implementing this algorithm enhances problem-solving abilities and coding proficiency.
Conclusion
The Hamiltonian Cycle algorithm is a fascinating topic that sits at the intersection of graph theory, algorithm design, and computational complexity. While it may seem daunting at first, understanding and implementing this algorithm can significantly enhance a programmer’s problem-solving skills and algorithmic thinking.
As we’ve seen, the basic implementation using backtracking provides a solid foundation, but there’s always room for optimization and exploration of related concepts. Whether you’re preparing for technical interviews, working on graph-based problems, or simply expanding your algorithmic toolkit, the Hamiltonian Cycle algorithm is a valuable addition to your knowledge base.
Remember, the journey of learning algorithms is not just about memorizing implementations, but about understanding the underlying principles and developing the ability to apply these concepts to solve real-world problems. Keep practicing, exploring variations, and challenging yourself with increasingly complex graph problems. With persistence and curiosity, you’ll not only master the Hamiltonian Cycle algorithm but also develop a deeper appreciation for the beautiful world of algorithmic problem-solving.