Understanding Topological Sorting in Graphs: A Comprehensive Guide
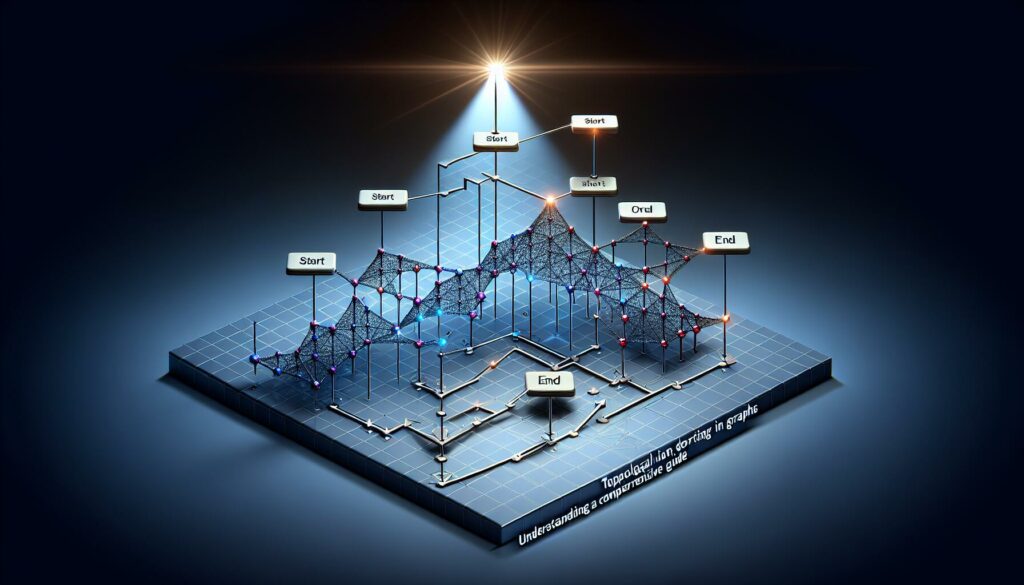
In the world of computer science and graph theory, topological sorting is a fundamental concept that plays a crucial role in solving various real-world problems. Whether you’re a beginner programmer or preparing for technical interviews at major tech companies, understanding topological sorting is essential. In this comprehensive guide, we’ll dive deep into the concept of topological sorting, its applications, and how to implement it efficiently.
What is Topological Sorting?
Topological sorting, also known as topological ordering, is an algorithm used to linearly order the vertices of a directed acyclic graph (DAG) in such a way that for every directed edge (u, v), vertex u comes before vertex v in the ordering. In simpler terms, it’s a way to arrange the nodes of a graph in a sequence where each node appears before all the nodes it points to.
To better understand this concept, let’s consider a real-world example:
Example: Course Prerequisites
Imagine you’re planning your college courses, and some courses have prerequisites. For instance:
- Data Structures (DS) must be taken before Algorithms (ALG)
- Calculus I (CAL1) must be taken before Calculus II (CAL2)
- Calculus II (CAL2) must be taken before Linear Algebra (LA)
- Programming Fundamentals (PF) must be taken before Data Structures (DS)
In this scenario, a topological sort would give you a valid sequence to take these courses without violating any prerequisites. One possible ordering could be:
PF → CAL1 → DS → CAL2 → ALG → LA
This ordering ensures that you take each course only after completing its prerequisites.
Properties of Topological Sorting
Before we dive into the implementation details, let’s discuss some important properties of topological sorting:
- Directed Acyclic Graph (DAG): Topological sorting is only possible for DAGs. If the graph contains a cycle, no valid topological ordering exists.
- Not Unique: A graph can have multiple valid topological orderings.
- Partial Ordering: Topological sort provides a partial ordering of the vertices, not necessarily a total ordering.
- Source and Sink: In a topological ordering, source nodes (nodes with no incoming edges) appear first, and sink nodes (nodes with no outgoing edges) appear last.
Applications of Topological Sorting
Topological sorting has numerous practical applications in computer science and real-world scenarios. Some common use cases include:
- Dependency Resolution: In build systems, package managers, and task schedulers to determine the order of compilation or execution.
- Course Scheduling: As in our earlier example, for creating valid academic schedules.
- Data Processing Pipelines: In data engineering, to determine the order of data transformation steps.
- Symbol Resolution in Programming Languages: To resolve dependencies between symbols in compilers.
- Task Scheduling in Project Management: To schedule tasks with dependencies in project planning tools.
Algorithms for Topological Sorting
There are two main algorithms for performing topological sorting:
- Kahn’s Algorithm (BFS-based)
- Depth-First Search (DFS) Algorithm
Let’s explore both of these algorithms in detail.
1. Kahn’s Algorithm
Kahn’s algorithm is an iterative approach that uses a queue to keep track of nodes with no incoming edges (in-degree of 0). Here’s how it works:
- Calculate the in-degree (number of incoming edges) for each vertex.
- Enqueue all vertices with in-degree 0 into a queue.
- While the queue is not empty:
- Dequeue a vertex and add it to the result list.
- Reduce the in-degree of all its neighbors by 1.
- If any neighbor’s in-degree becomes 0, enqueue it.
- If all vertices are in the result list, return the list. Otherwise, the graph has a cycle, and topological sorting is not possible.
Let’s implement Kahn’s algorithm in Python:
from collections import defaultdict, deque
def kahn_topological_sort(graph):
# Calculate in-degree for each vertex
in_degree = {v: 0 for v in graph}
for v in graph:
for neighbor in graph[v]:
in_degree[neighbor] += 1
# Initialize queue with vertices having in-degree 0
queue = deque([v for v in in_degree if in_degree[v] == 0])
result = []
while queue:
vertex = queue.popleft()
result.append(vertex)
for neighbor in graph[vertex]:
in_degree[neighbor] -= 1
if in_degree[neighbor] == 0:
queue.append(neighbor)
# Check if topological sort is possible
if len(result) != len(graph):
return None # Graph has a cycle
return result
# Example usage
graph = {
'PF': ['DS'],
'CAL1': ['CAL2'],
'DS': ['ALG'],
'CAL2': ['LA'],
'ALG': [],
'LA': []
}
sorted_order = kahn_topological_sort(graph)
print(sorted_order) # Output: ['PF', 'CAL1', 'DS', 'CAL2', 'ALG', 'LA']
Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Space Complexity: O(V), for storing the in-degree and queue.
2. Depth-First Search (DFS) Algorithm
The DFS-based approach for topological sorting uses recursion to explore the graph and build the sorted order. Here’s how it works:
- Perform a DFS traversal of the graph.
- As each vertex finishes its DFS (i.e., all its neighbors have been visited), push it onto a stack.
- After the DFS is complete, pop all vertices from the stack to get the topological order.
Let’s implement the DFS-based topological sort in Python:
from collections import defaultdict
def dfs_topological_sort(graph):
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
visited = set()
stack = []
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1] # Reverse the stack to get topological order
# Example usage
graph = {
'PF': ['DS'],
'CAL1': ['CAL2'],
'DS': ['ALG'],
'CAL2': ['LA'],
'ALG': [],
'LA': []
}
sorted_order = dfs_topological_sort(graph)
print(sorted_order) # Output: ['CAL1', 'PF', 'DS', 'CAL2', 'ALG', 'LA']
Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Space Complexity: O(V), for the recursion stack and visited set.
Detecting Cycles in a Graph
As mentioned earlier, topological sorting is only possible for directed acyclic graphs (DAGs). If the graph contains a cycle, no valid topological ordering exists. Both Kahn’s algorithm and the DFS-based approach can be modified to detect cycles in a graph.
Cycle Detection using Kahn’s Algorithm
In Kahn’s algorithm, if the final result doesn’t include all vertices of the graph, it indicates the presence of a cycle. We’ve already implemented this check in our earlier Kahn’s algorithm implementation.
Cycle Detection using DFS
For the DFS-based approach, we can detect cycles by keeping track of vertices in the current recursion stack. If we encounter a vertex that’s already in the recursion stack, we’ve found a cycle. Here’s an implementation of cycle detection using DFS:
def has_cycle(graph):
def dfs(v):
visited.add(v)
rec_stack.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(v)
return False
visited = set()
rec_stack = set()
for vertex in graph:
if vertex not in visited:
if dfs(vertex):
return True
return False
# Example usage
graph_with_cycle = {
'A': ['B'],
'B': ['C'],
'C': ['A']
}
graph_without_cycle = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': []
}
print(has_cycle(graph_with_cycle)) # Output: True
print(has_cycle(graph_without_cycle)) # Output: False
Advanced Topics and Variations
Now that we’ve covered the basics of topological sorting, let’s explore some advanced topics and variations:
1. All Possible Topological Orderings
In some cases, you might need to generate all possible topological orderings of a graph. This can be done using a backtracking approach. Here’s a Python implementation:
from collections import defaultdict
def all_topological_sorts(graph):
def backtrack(visited, result):
if len(result) == len(graph):
all_orders.append(result[:])
return
for vertex in graph:
if vertex not in visited and all(v in visited for v in reversed_graph[vertex]):
visited.add(vertex)
result.append(vertex)
backtrack(visited, result)
visited.remove(vertex)
result.pop()
reversed_graph = defaultdict(list)
for v in graph:
for neighbor in graph[v]:
reversed_graph[neighbor].append(v)
all_orders = []
backtrack(set(), [])
return all_orders
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': []
}
all_orders = all_topological_sorts(graph)
for order in all_orders:
print(order)
# Output:
# ['A', 'B', 'C', 'D']
# ['A', 'C', 'B', 'D']
2. Lexicographically Smallest Topological Ordering
In some problems, you might be asked to find the lexicographically smallest topological ordering. This can be achieved by modifying Kahn’s algorithm to use a min-heap instead of a queue. Here’s an implementation:
import heapq
from collections import defaultdict
def lexicographically_smallest_topological_sort(graph):
in_degree = {v: 0 for v in graph}
for v in graph:
for neighbor in graph[v]:
in_degree[neighbor] += 1
heap = [v for v in in_degree if in_degree[v] == 0]
heapq.heapify(heap)
result = []
while heap:
vertex = heapq.heappop(heap)
result.append(vertex)
for neighbor in graph[vertex]:
in_degree[neighbor] -= 1
if in_degree[neighbor] == 0:
heapq.heappush(heap, neighbor)
if len(result) != len(graph):
return None # Graph has a cycle
return result
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': []
}
smallest_order = lexicographically_smallest_topological_sort(graph)
print(smallest_order) # Output: ['A', 'B', 'C', 'D']
3. Topological Sorting with Weighted Edges
In some scenarios, the edges in the graph might have weights, and you need to find the longest path in the DAG. This problem is known as the “Critical Path Method” and is often used in project management. Here’s an implementation:
from collections import defaultdict
def longest_path(graph, weights):
def dfs(v):
if v in memo:
return memo[v]
max_length = 0
for neighbor in graph[v]:
length = dfs(neighbor) + weights[(v, neighbor)]
max_length = max(max_length, length)
memo[v] = max_length
return max_length
memo = {}
max_path_length = 0
for vertex in graph:
max_path_length = max(max_path_length, dfs(vertex))
return max_path_length
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': []
}
weights = {
('A', 'B'): 3,
('A', 'C'): 2,
('B', 'D'): 4,
('C', 'D'): 5
}
longest_path_length = longest_path(graph, weights)
print(f"Longest path length: {longest_path_length}") # Output: Longest path length: 7
Common Pitfalls and Best Practices
When working with topological sorting, keep these points in mind:
- Cycle Detection: Always check for cycles before attempting topological sorting.
- Graph Representation: Choose an appropriate graph representation (adjacency list or matrix) based on the problem requirements.
- Time Complexity: Both Kahn’s and DFS-based algorithms have O(V + E) time complexity, but Kahn’s algorithm might be faster in practice due to better cache locality.
- Space Complexity: Be mindful of space usage, especially for large graphs.
- Edge Cases: Handle empty graphs and single-node graphs correctly.
- Multiple Valid Orderings: Remember that there can be multiple valid topological orderings for a given graph.
Conclusion
Topological sorting is a powerful technique with numerous applications in computer science and real-world problem-solving. By understanding the concept, algorithms, and variations of topological sorting, you’ll be better equipped to tackle complex problems involving dependencies and ordering.
As you prepare for technical interviews or work on real-world projects, practice implementing topological sorting algorithms and solving related problems. This will help you develop a deeper understanding of graph algorithms and their applications.
Remember, mastering topological sorting is not just about memorizing algorithms; it’s about understanding the underlying principles and being able to apply them to solve diverse problems. Keep practicing, and you’ll be well-prepared to tackle graph-related challenges in your coding journey!