Solving Problems with Constraint Satisfaction: A Comprehensive Guide
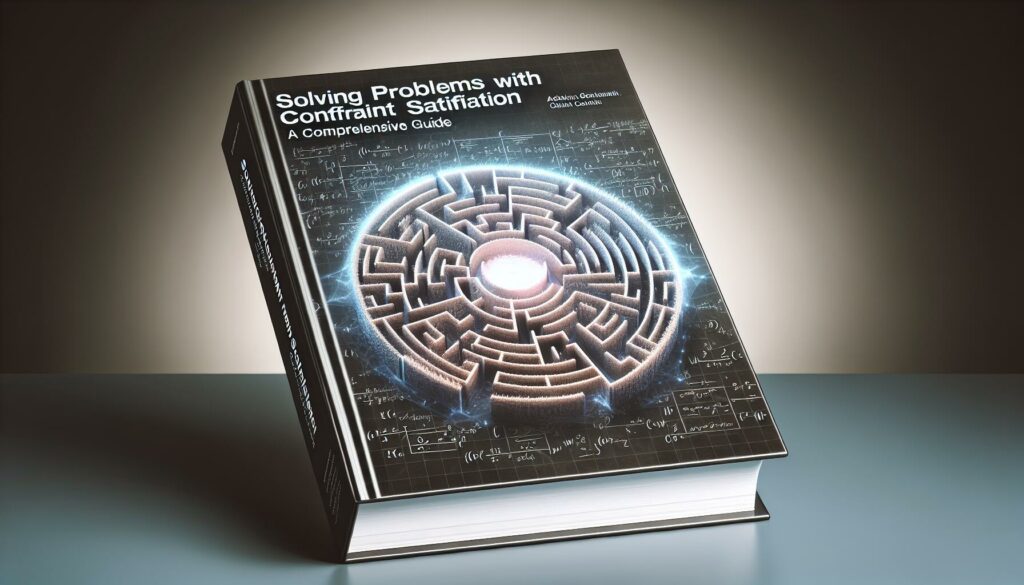
In the world of computer science and artificial intelligence, constraint satisfaction problems (CSPs) play a crucial role in solving complex real-world issues. From scheduling and resource allocation to configuration and design, CSPs offer a powerful framework for tackling a wide range of challenges. In this comprehensive guide, we’ll dive deep into the world of constraint satisfaction, exploring its principles, techniques, and applications.
What is Constraint Satisfaction?
Constraint satisfaction is a problem-solving paradigm where the goal is to find a solution that satisfies a set of constraints or conditions. These problems are typically represented as a set of variables, each with a domain of possible values, and a set of constraints that restrict the combinations of values that can be assigned to the variables.
Formally, a constraint satisfaction problem consists of:
- A set of variables X = {Xâ‚, Xâ‚‚, …, Xâ‚™}
- A domain D for each variable, specifying the possible values it can take
- A set of constraints C that specify allowable combinations of values for subsets of variables
The objective is to find an assignment of values to all variables that satisfies all the constraints.
Types of Constraints
Constraints in CSPs can be categorized into several types:
- Unary constraints: Involve a single variable (e.g., X > 0)
- Binary constraints: Involve two variables (e.g., X < Y)
- Global constraints: Involve three or more variables (e.g., all-different constraint)
- Hard constraints: Must be satisfied in any valid solution
- Soft constraints: Preferences that can be violated but at a cost
Solving Constraint Satisfaction Problems
There are several approaches to solving CSPs, ranging from simple backtracking algorithms to more sophisticated techniques. Let’s explore some of the most common methods:
1. Backtracking Search
Backtracking is a depth-first search algorithm that incrementally builds candidates to the solution and abandons a candidate as soon as it determines that it cannot lead to a valid solution. Here’s a basic implementation of backtracking for CSPs:
def backtrack(assignment, csp):
if len(assignment) == len(csp.variables):
return assignment
var = select_unassigned_variable(csp, assignment)
for value in order_domain_values(var, assignment, csp):
if is_consistent(var, value, assignment, csp):
assignment[var] = value
result = backtrack(assignment, csp)
if result is not None:
return result
del assignment[var]
return None
2. Forward Checking
Forward checking is an improvement over simple backtracking. It maintains arc consistency by checking the domains of future variables and pruning values that are no longer consistent with the current assignment.
def forward_checking(assignment, csp):
if len(assignment) == len(csp.variables):
return assignment
var = select_unassigned_variable(csp, assignment)
for value in order_domain_values(var, assignment, csp):
if is_consistent(var, value, assignment, csp):
assignment[var] = value
inferences = {}
for neighbor in csp.neighbors[var]:
if neighbor not in assignment:
for neighbor_value in csp.domains[neighbor]:
if not is_consistent(neighbor, neighbor_value, assignment, csp):
if neighbor not in inferences:
inferences[neighbor] = set()
inferences[neighbor].add(neighbor_value)
if all(len(csp.domains[v] - inferences.get(v, set())) > 0 for v in csp.variables if v not in assignment):
result = forward_checking(assignment, csp)
if result is not None:
return result
assignment.pop(var)
for v, pruned in inferences.items():
csp.domains[v] |= pruned
return None
3. Arc Consistency
Arc consistency is a technique used to reduce the domains of variables by enforcing local consistency. The most common algorithm for achieving arc consistency is AC-3:
def AC3(csp):
queue = [(Xi, Xj) for Xi in csp.variables for Xj in csp.neighbors[Xi]]
while queue:
(Xi, Xj) = queue.pop(0)
if revise(csp, Xi, Xj):
if len(csp.domains[Xi]) == 0:
return False
for Xk in csp.neighbors[Xi]:
if Xk != Xj:
queue.append((Xk, Xi))
return True
def revise(csp, Xi, Xj):
revised = False
for x in csp.domains[Xi][:]:
if not any(is_consistent(Xi, x, {Xj: y}, csp) for y in csp.domains[Xj]):
csp.domains[Xi].remove(x)
revised = True
return revised
4. Constraint Propagation
Constraint propagation is a process of inferring additional constraints from existing ones. It can significantly reduce the search space and improve efficiency. One common technique is node consistency:
def make_node_consistent(csp):
for var in csp.variables:
for value in csp.domains[var][:]:
if not is_consistent(var, value, {}, csp):
csp.domains[var].remove(value)
return csp
5. Local Search
For large-scale CSPs, complete search algorithms may be impractical. Local search algorithms, such as min-conflicts, can find solutions quickly but may not guarantee optimality:
def min_conflicts(csp, max_steps=1000):
current = {var: random.choice(csp.domains[var]) for var in csp.variables}
for i in range(max_steps):
conflicted = [var for var in csp.variables if not is_consistent(var, current[var], current, csp)]
if not conflicted:
return current
var = random.choice(conflicted)
current[var] = min(csp.domains[var], key=lambda val: conflicts(var, val, current, csp))
return None
def conflicts(var, val, assignment, csp):
return sum(not is_consistent(var, val, assignment, csp) for var in csp.variables if var != var)
Advanced Techniques in Constraint Satisfaction
As we delve deeper into the world of constraint satisfaction, several advanced techniques can significantly enhance the efficiency and effectiveness of solving complex CSPs:
1. Variable and Value Ordering Heuristics
The order in which variables are selected and values are tried can greatly impact the performance of CSP algorithms. Some common heuristics include:
- Minimum Remaining Values (MRV): Choose the variable with the fewest remaining values in its domain.
- Degree Heuristic: Select the variable involved in the largest number of constraints with other unassigned variables.
- Least Constraining Value: Choose the value that rules out the fewest choices for neighboring variables.
Here’s an example of implementing the MRV heuristic:
def select_unassigned_variable(csp, assignment):
unassigned = [var for var in csp.variables if var not in assignment]
return min(unassigned, key=lambda var: len(csp.domains[var]))
2. Constraint Learning
Constraint learning techniques dynamically add new constraints during the search process to avoid repeating the same mistakes. One popular method is conflict-directed backjumping:
def conflict_directed_backjumping(assignment, csp):
if len(assignment) == len(csp.variables):
return assignment
var = select_unassigned_variable(csp, assignment)
for value in order_domain_values(var, assignment, csp):
if is_consistent(var, value, assignment, csp):
assignment[var] = value
result, conflict_set = conflict_directed_backjumping(assignment, csp)
if result is not None:
return result, None
if var not in conflict_set:
return None, conflict_set
del assignment[var]
else:
conflict_set = get_conflicting_variables(var, value, assignment, csp)
return None, conflict_set
def get_conflicting_variables(var, value, assignment, csp):
return set(v for v in assignment if not is_consistent(var, value, {v: assignment[v]}, csp))
3. Symmetry Breaking
Many CSPs contain symmetries that can lead to redundant search. Symmetry breaking techniques aim to eliminate these redundancies and reduce the search space. One approach is to add symmetry-breaking constraints:
def add_symmetry_breaking_constraint(csp):
# Example: For a problem with n variables, add a constraint that the first variable
# must be less than or equal to the last variable
n = len(csp.variables)
if n > 1:
csp.add_constraint(lambda a, b: a <= b, (csp.variables[0], csp.variables[-1]))
return csp
4. Nogood Learning
Nogood learning is a technique that records combinations of variable assignments that lead to failures. These “nogoods” can be used to prune the search space in future iterations:
class NoGoodStore:
def __init__(self):
self.nogoods = set()
def add(self, assignment):
self.nogoods.add(frozenset(assignment.items()))
def contains(self, assignment):
return any(nogood.issubset(assignment.items()) for nogood in self.nogoods)
def backtrack_with_nogood_learning(assignment, csp, nogood_store):
if len(assignment) == len(csp.variables):
return assignment
var = select_unassigned_variable(csp, assignment)
for value in order_domain_values(var, assignment, csp):
new_assignment = assignment.copy()
new_assignment[var] = value
if not nogood_store.contains(new_assignment) and is_consistent(var, value, assignment, csp):
result = backtrack_with_nogood_learning(new_assignment, csp, nogood_store)
if result is not None:
return result
nogood_store.add(new_assignment)
return None
Real-World Applications of Constraint Satisfaction
Constraint satisfaction problems have a wide range of practical applications across various domains. Let’s explore some real-world scenarios where CSPs are effectively used:
1. Scheduling and Timetabling
CSPs are extensively used in creating schedules for schools, universities, sports leagues, and transportation systems. For example, creating a university course timetable involves assigning courses to time slots and rooms while satisfying constraints such as room capacity, professor availability, and avoiding conflicts.
class CourseSchedulingCSP:
def __init__(self, courses, time_slots, rooms):
self.variables = courses
self.domains = {course: [(t, r) for t in time_slots for r in rooms] for course in courses}
self.constraints = []
def add_room_capacity_constraint(self, course, capacity):
self.constraints.append(lambda c, v: v[1].capacity >= course.enrollment)
def add_professor_availability_constraint(self, course, available_times):
self.constraints.append(lambda c, v: v[0] in available_times)
def add_course_conflict_constraint(self, course1, course2):
self.constraints.append(lambda c1, v1, c2, v2: v1[0] != v2[0])
2. Resource Allocation
CSPs can optimize the allocation of limited resources in various scenarios, such as assigning tasks to machines in a factory or distributing network bandwidth.
class ResourceAllocationCSP:
def __init__(self, tasks, resources):
self.variables = tasks
self.domains = {task: resources for task in tasks}
self.constraints = []
def add_capacity_constraint(self, resource, max_tasks):
self.constraints.append(lambda assignment: sum(1 for t, r in assignment.items() if r == resource) <= max_tasks)
def add_compatibility_constraint(self, task, compatible_resources):
self.constraints.append(lambda t, r: r in compatible_resources)
3. Configuration and Design
CSPs are useful in configuring complex systems or designing products that must meet specific requirements. For instance, configuring a computer system with compatible components or designing an automobile that meets safety and performance standards.
class ComputerConfigurationCSP:
def __init__(self, components):
self.variables = components
self.domains = {
'CPU': ['Intel i5', 'Intel i7', 'AMD Ryzen 5', 'AMD Ryzen 7'],
'GPU': ['NVIDIA GTX 1660', 'NVIDIA RTX 3060', 'AMD RX 5700', 'AMD RX 6700'],
'RAM': ['8GB', '16GB', '32GB', '64GB'],
'Storage': ['256GB SSD', '512GB SSD', '1TB HDD', '2TB HDD']
}
self.constraints = []
def add_compatibility_constraint(self, component1, component2, compatibility_map):
self.constraints.append(lambda c1, v1, c2, v2: (v1, v2) in compatibility_map)
def add_budget_constraint(self, max_budget, price_map):
self.constraints.append(lambda assignment: sum(price_map[c][v] for c, v in assignment.items()) <= max_budget)
4. Map Coloring
The classic map coloring problem, where adjacent regions must be colored differently, is a perfect example of a CSP. This problem has applications in cartography, radio frequency assignment, and register allocation in compilers.
class MapColoringCSP:
def __init__(self, regions, colors):
self.variables = regions
self.domains = {region: colors for region in regions}
self.constraints = []
def add_adjacent_constraint(self, region1, region2):
self.constraints.append(lambda r1, c1, r2, c2: c1 != c2)
5. Puzzle Solving
Many popular puzzles, such as Sudoku, Kakuro, and Crosswords, can be formulated as CSPs. Solving these puzzles programmatically demonstrates the power of constraint satisfaction techniques.
class SudokuCSP:
def __init__(self, grid):
self.variables = [(i, j) for i in range(9) for j in range(9)]
self.domains = {
(i, j): list(range(1, 10)) if grid[i][j] == 0 else [grid[i][j]]
for i in range(9) for j in range(9)
}
self.constraints = []
def add_row_constraint(self):
for i in range(9):
self.constraints.append(lambda assignment: len(set(assignment[(i, j)] for j in range(9))) == 9)
def add_column_constraint(self):
for j in range(9):
self.constraints.append(lambda assignment: len(set(assignment[(i, j)] for i in range(9))) == 9)
def add_box_constraint(self):
for box_i in range(3):
for box_j in range(3):
cells = [(i + 3 * box_i, j + 3 * box_j) for i in range(3) for j in range(3)]
self.constraints.append(lambda assignment: len(set(assignment[cell] for cell in cells)) == 9)
Challenges and Future Directions in Constraint Satisfaction
While constraint satisfaction has proven to be a powerful paradigm for solving a wide range of problems, there are still several challenges and areas for future research:
1. Scalability
As problem sizes grow, traditional CSP techniques may struggle to find solutions in reasonable time. Researchers are exploring ways to improve scalability through:
- Parallel and distributed constraint solving algorithms
- Approximation techniques for large-scale CSPs
- Hybrid approaches combining CSP with other optimization methods
2. Handling Uncertainty
Many real-world problems involve uncertainty in constraints or variable domains. Developing robust CSP techniques that can handle probabilistic or fuzzy constraints is an active area of research.
3. Dynamic CSPs
In some applications, constraints or variable domains may change over time. Developing efficient algorithms for solving dynamic CSPs and adapting solutions in real-time is crucial for many practical applications.
4. Explanation and Interpretability
As CSP techniques are increasingly used in critical decision-making processes, there’s a growing need for methods that can explain the reasoning behind solutions and provide interpretable results.
5. Integration with Machine Learning
Combining constraint satisfaction with machine learning techniques offers exciting possibilities, such as:
- Learning constraint preferences from data
- Using neural networks to guide CSP search
- Developing hybrid models that leverage the strengths of both approaches
Conclusion
Constraint satisfaction problems provide a powerful framework for solving a wide range of complex real-world challenges. From simple backtracking algorithms to sophisticated techniques like constraint propagation and symmetry breaking, the field of CSP offers a rich set of tools for tackling diverse problems in scheduling, resource allocation, configuration, and beyond.
As we’ve explored in this comprehensive guide, the principles of constraint satisfaction can be applied to numerous domains, from academic puzzles to critical industrial applications. By understanding the fundamental concepts and advanced techniques in CSP, developers and researchers can leverage this powerful paradigm to create more efficient and effective solutions to complex problems.
The future of constraint satisfaction is bright, with ongoing research addressing challenges in scalability, uncertainty, and dynamic environments. As CSP techniques continue to evolve and integrate with other areas of artificial intelligence and machine learning, we can expect to see even more innovative applications and powerful problem-solving capabilities in the years to come.
Whether you’re a student learning about algorithmic problem-solving, a developer working on optimization challenges, or a researcher pushing the boundaries of artificial intelligence, mastering constraint satisfaction techniques will undoubtedly prove to be a valuable skill in your journey through the world of computer science and beyond.