Algorithmic Foundations of Data Privacy: Protecting Information in the Digital Age
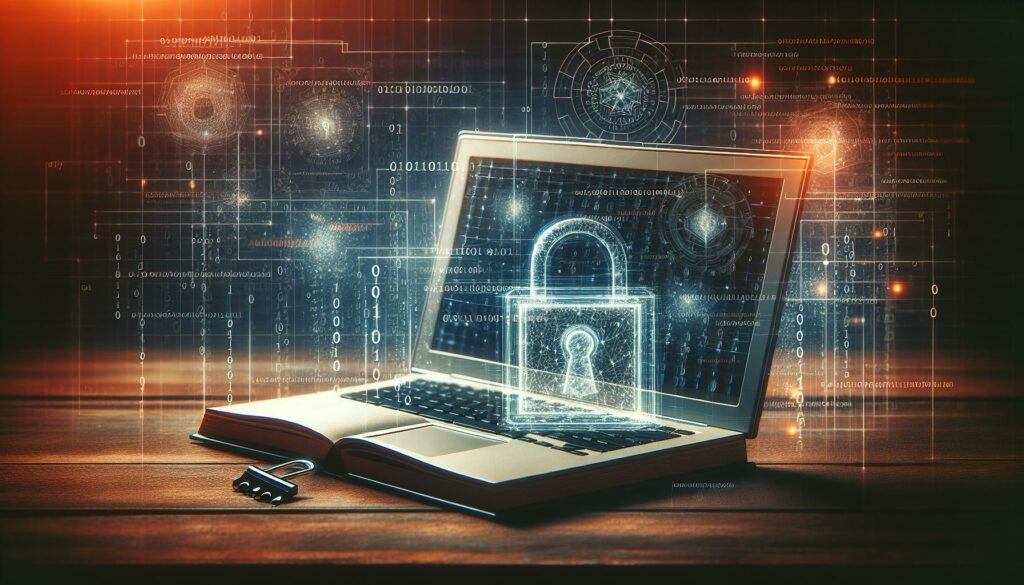
In today’s data-driven world, the importance of data privacy cannot be overstated. As we continue to generate and share vast amounts of personal information online, the need for robust privacy protection mechanisms has become paramount. This is where the algorithmic foundations of data privacy come into play, providing the theoretical and practical basis for safeguarding sensitive information. In this comprehensive guide, we’ll explore the key concepts, techniques, and challenges in the field of data privacy, with a focus on the algorithmic approaches that underpin modern privacy-preserving systems.
Understanding Data Privacy
Before diving into the algorithmic aspects, it’s crucial to understand what data privacy entails and why it matters. Data privacy refers to the proper handling, processing, and storage of personal information. It encompasses the rights of individuals to control their personal data and the obligations of organizations to protect that data from unauthorized access, use, or disclosure.
The importance of data privacy has grown exponentially in recent years due to:
- Increased digital footprints: As we spend more time online, we generate more data about our behaviors, preferences, and identities.
- Data breaches: High-profile incidents have highlighted the vulnerabilities in data storage and handling practices.
- Regulatory requirements: Laws like GDPR and CCPA have imposed strict data protection obligations on organizations.
- Ethical considerations: There’s growing awareness of the ethical implications of data collection and use.
Fundamental Concepts in Data Privacy
To understand the algorithmic foundations of data privacy, we need to familiarize ourselves with some key concepts:
1. Anonymization
Anonymization is the process of removing or modifying personally identifiable information (PII) from a dataset, making it impossible to identify specific individuals. This is often the first line of defense in protecting privacy.
2. Pseudonymization
Pseudonymization involves replacing identifying information with artificial identifiers or pseudonyms. Unlike anonymization, pseudonymized data can be traced back to its origins with the use of additional information.
3. Differential Privacy
Differential privacy is a mathematical definition of privacy that provides strong guarantees against the identification of individuals in a dataset. It involves adding carefully calibrated noise to query results to mask individual contributions.
4. k-Anonymity
k-Anonymity is a property of a dataset where each record is indistinguishable from at least k-1 other records with respect to certain identifying attributes.
5. Homomorphic Encryption
Homomorphic encryption is a form of encryption that allows computations to be performed on encrypted data without decrypting it first, preserving the privacy of the input data.
Algorithmic Approaches to Data Privacy
Now that we’ve covered the basic concepts, let’s explore some of the key algorithmic approaches used in data privacy:
1. Data Anonymization Algorithms
Data anonymization algorithms aim to transform data in ways that preserve privacy while maintaining utility for analysis. Some common techniques include:
- Suppression: Removing certain attributes or records entirely.
- Generalization: Replacing specific values with more general ones (e.g., exact age with age ranges).
- Perturbation: Adding noise to numerical values to obscure the original data.
Here’s a simple example of how data anonymization might work in practice:
# Original data
original_data = [
{"name": "Alice", "age": 32, "city": "New York"},
{"name": "Bob", "age": 28, "city": "Los Angeles"},
{"name": "Charlie", "age": 45, "city": "Chicago"}
]
# Anonymized data
anonymized_data = [
{"age_range": "30-40", "region": "East Coast"},
{"age_range": "20-30", "region": "West Coast"},
{"age_range": "40-50", "region": "Midwest"}
]
In this example, we’ve applied generalization to the age attribute and replaced specific cities with broader regions.
2. Differential Privacy Algorithms
Differential privacy algorithms add controlled noise to query results to protect individual privacy. The most common mechanism for achieving differential privacy is the Laplace Mechanism, which adds noise drawn from a Laplace distribution to the true query result.
Here’s a simplified implementation of the Laplace Mechanism in Python:
import numpy as np
def laplace_mechanism(true_value, sensitivity, epsilon):
scale = sensitivity / epsilon
noise = np.random.laplace(0, scale)
return true_value + noise
# Example usage
true_count = 100
sensitivity = 1 # Assuming each individual contributes at most 1 to the count
epsilon = 0.1 # Privacy parameter (smaller epsilon = more privacy)
private_count = laplace_mechanism(true_count, sensitivity, epsilon)
print(f"True count: {true_count}")
print(f"Private count: {private_count}")
This implementation adds Laplace noise to a count query, providing differential privacy guarantees.
3. k-Anonymity Algorithms
k-Anonymity algorithms transform data to ensure that each record is indistinguishable from at least k-1 other records. This is typically achieved through generalization and suppression techniques.
Here’s a conceptual example of how k-anonymity might be implemented:
def k_anonymize(data, k, quasi_identifiers):
# Group records by quasi-identifiers
groups = group_by_quasi_identifiers(data, quasi_identifiers)
anonymized_data = []
for group in groups:
if len(group) >= k:
# If group size is at least k, generalize quasi-identifiers
generalized_group = generalize_attributes(group, quasi_identifiers)
anonymized_data.extend(generalized_group)
else:
# If group size is less than k, suppress these records
continue
return anonymized_data
# Example usage
data = [
{"age": 32, "zipcode": "12345", "diagnosis": "Flu"},
{"age": 33, "zipcode": "12346", "diagnosis": "Cold"},
{"age": 35, "zipcode": "12345", "diagnosis": "Fever"},
# ... more records ...
]
k = 2
quasi_identifiers = ["age", "zipcode"]
anonymized_data = k_anonymize(data, k, quasi_identifiers)
This example demonstrates the basic concept of k-anonymity, where records are grouped and generalized to ensure each group has at least k members.
4. Homomorphic Encryption Algorithms
Homomorphic encryption allows computations on encrypted data without decryption. While fully homomorphic encryption (FHE) is still computationally expensive for many practical applications, partially homomorphic encryption schemes are more widely used.
Here’s a simple example of additive homomorphic encryption using the Paillier cryptosystem:
from phe import paillier
# Generate public and private keys
public_key, private_key = paillier.generate_paillier_keypair()
# Encrypt two numbers
x = 5
y = 3
encrypted_x = public_key.encrypt(x)
encrypted_y = public_key.encrypt(y)
# Perform addition on encrypted values
encrypted_sum = encrypted_x + encrypted_y
# Decrypt the result
decrypted_sum = private_key.decrypt(encrypted_sum)
print(f"x: {x}, y: {y}")
print(f"x + y: {decrypted_sum}")
This example demonstrates how homomorphic encryption allows addition to be performed on encrypted values, with the correct result obtained after decryption.
Challenges in Implementing Privacy-Preserving Algorithms
While these algorithmic approaches provide powerful tools for protecting data privacy, their implementation comes with several challenges:
1. Privacy-Utility Trade-off
One of the primary challenges in data privacy is balancing privacy protection with data utility. Stronger privacy guarantees often come at the cost of reduced data utility. Finding the right balance requires careful consideration of the specific use case and privacy requirements.
2. Computational Complexity
Many privacy-preserving algorithms, especially those involving cryptographic techniques like homomorphic encryption, can be computationally intensive. This can limit their applicability in real-time or large-scale data processing scenarios.
3. Dynamic Data Environments
Many privacy-preserving techniques are designed for static datasets. Adapting these approaches to dynamic data environments, where data is constantly being added or updated, presents additional challenges.
4. Composition of Privacy Guarantees
When multiple privacy-preserving operations are applied sequentially or in parallel, understanding and quantifying the overall privacy guarantee becomes complex. This is known as the composition problem in differential privacy.
5. Adversarial Attacks
As privacy-preserving techniques evolve, so do the methods for attacking them. Researchers continually discover new ways to potentially de-anonymize data or infer private information, necessitating ongoing refinement of privacy algorithms.
Emerging Trends and Future Directions
The field of data privacy is rapidly evolving, with several exciting trends and future directions:
1. Federated Learning
Federated learning allows machine learning models to be trained on distributed datasets without centralizing the data, preserving privacy and data locality. This approach is particularly promising for scenarios where data cannot be shared due to privacy or regulatory constraints.
2. Secure Multi-Party Computation (MPC)
MPC protocols allow multiple parties to jointly compute a function over their inputs while keeping those inputs private. These techniques are becoming increasingly practical for real-world applications in finance, healthcare, and other sensitive domains.
3. Privacy-Preserving Machine Learning
As machine learning becomes more pervasive, there’s growing interest in developing privacy-preserving machine learning techniques. This includes differentially private learning algorithms, encrypted inference, and privacy-preserving data synthesis.
4. Quantum-Resistant Privacy Algorithms
With the advent of quantum computing on the horizon, there’s a need to develop privacy-preserving algorithms that are resistant to quantum attacks. This is particularly important for long-term data protection.
5. Privacy-Enhancing Technologies (PETs)
PETs encompass a broad range of technologies designed to protect personal data and enable privacy-respecting data processing. The development and adoption of PETs are likely to accelerate as privacy concerns continue to grow.
Conclusion
The algorithmic foundations of data privacy provide a robust framework for protecting sensitive information in our increasingly data-driven world. From fundamental techniques like anonymization and differential privacy to advanced approaches like homomorphic encryption and federated learning, these algorithms form the backbone of modern privacy-preserving systems.
As we continue to generate and rely on vast amounts of data, the importance of these privacy-preserving techniques will only grow. Developers, data scientists, and organizations must stay informed about these approaches and incorporate them into their data handling practices. By doing so, we can harness the power of data while respecting individual privacy and complying with evolving regulatory requirements.
The field of data privacy is dynamic and challenging, requiring ongoing research and innovation to stay ahead of potential threats and address emerging use cases. As we look to the future, the continued development of privacy-preserving algorithms will play a crucial role in shaping a digital landscape that values and protects individual privacy.
By understanding and implementing these algorithmic foundations of data privacy, we can work towards a future where data-driven innovation and personal privacy coexist harmoniously, fostering trust and enabling the responsible use of data across all sectors of society.