Introduction to Randomized Algorithms: Unlocking Efficiency Through Probability
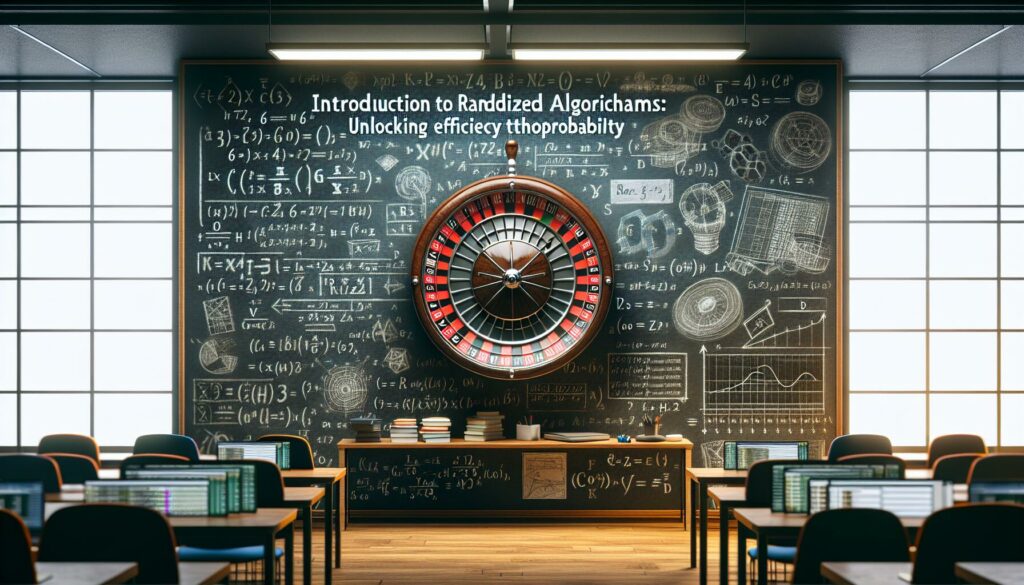
In the world of computer science and algorithm design, there’s a fascinating class of algorithms that harness the power of randomness to solve problems efficiently. These are known as randomized algorithms, and they’ve become an integral part of modern computing. In this comprehensive guide, we’ll dive deep into the concept of randomized algorithms, explore their advantages and applications, and see how they fit into the broader landscape of algorithmic problem-solving.
What Are Randomized Algorithms?
Randomized algorithms are algorithms that make random choices during their execution. Unlike deterministic algorithms, which always produce the same output for a given input, randomized algorithms may produce different outputs or take different paths of execution each time they’re run, even with the same input.
The key idea behind randomized algorithms is to use randomness as a tool to achieve better average-case performance, simplify complex algorithms, or solve problems that are difficult to approach deterministically.
Types of Randomized Algorithms
There are two main types of randomized algorithms:
- Las Vegas Algorithms: These algorithms always produce the correct result, but their running time is a random variable. The randomness is in the algorithm’s efficiency, not its correctness.
- Monte Carlo Algorithms: These algorithms may produce an incorrect result with a small probability. The running time is deterministic, but the correctness is probabilistic.
Advantages of Randomized Algorithms
Randomized algorithms offer several advantages over their deterministic counterparts:
- Simplicity: Many randomized algorithms are simpler to design and implement than their deterministic counterparts.
- Efficiency: In many cases, randomized algorithms can achieve better average-case performance than the best-known deterministic algorithms for the same problem.
- Versatility: Some problems that are difficult or impossible to solve deterministically can be approached effectively with randomized algorithms.
- Breaking worst-case scenarios: Randomization can help avoid consistently hitting worst-case scenarios that might plague deterministic algorithms.
Key Concepts in Randomized Algorithms
To understand randomized algorithms, it’s essential to grasp a few key concepts:
1. Probability and Expectation
Randomized algorithms rely heavily on probability theory. The concept of expected value is particularly important. For a randomized algorithm, we often analyze the expected running time or the expected quality of the solution.
2. Randomness as a Resource
In randomized algorithms, randomness is treated as a resource, much like time or space. The algorithm uses random bits to make decisions, and the analysis often considers how many random bits are needed.
3. Probabilistic Guarantees
Instead of worst-case guarantees, randomized algorithms often provide probabilistic guarantees. For example, “the algorithm runs in O(n log n) time with high probability” or “the algorithm finds the correct answer with probability at least 0.99”.
Common Techniques in Randomized Algorithms
Several techniques are commonly used in the design of randomized algorithms:
1. Random Sampling
This technique involves selecting a random subset of the input to work with, often leading to faster algorithms. It’s particularly useful when dealing with large datasets.
2. Randomized Rounding
This technique is used to convert fractional solutions of linear programming problems into integer solutions. It’s widely used in approximation algorithms.
3. Random Walks
Random walks are used in various algorithms, including those for graph problems and simulations. They involve making a sequence of random steps.
4. Probabilistic Counting
This technique is used to estimate the number of distinct elements in a large dataset using limited space.
Examples of Randomized Algorithms
Let’s explore some classic examples of randomized algorithms to see how these concepts are applied in practice.
1. Quicksort with Random Pivoting
Quicksort is a well-known sorting algorithm. In its randomized version, instead of always choosing the first or last element as the pivot, we choose a random element. This simple modification helps avoid worst-case scenarios and gives an expected running time of O(n log n).
Here’s a Python implementation of randomized Quicksort:
import random
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = random.choice(arr)
less = [x for x in arr if x < pivot]
equal = [x for x in arr if x == pivot]
greater = [x for x in arr if x > pivot]
return quicksort(less) + equal + quicksort(greater)
# Example usage
arr = [3, 6, 8, 10, 1, 2, 1]
sorted_arr = quicksort(arr)
print(sorted_arr) # Output: [1, 1, 2, 3, 6, 8, 10]
2. Randomized Primality Testing
Determining whether a large number is prime or composite can be computationally expensive. Randomized algorithms like the Miller-Rabin primality test can quickly determine with high probability whether a number is prime.
Here’s a simplified version of the Miller-Rabin test in Python:
import random
def miller_rabin(n, k=5):
if n < 2:
return False
for p in [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]:
if n % p == 0:
return n == p
s, d = 0, n - 1
while d % 2 == 0:
s, d = s + 1, d // 2
for i in range(k):
a = random.randrange(2, n - 1)
x = pow(a, d, n)
if x == 1 or x == n - 1:
continue
for r in range(s - 1):
x = pow(x, 2, n)
if x == n - 1:
break
else:
return False
return True
# Example usage
print(miller_rabin(997)) # Output: True (997 is prime)
print(miller_rabin(998)) # Output: False (998 is not prime)
3. Randomized Minimum Cut Algorithm
The randomized minimum cut algorithm, also known as Karger’s algorithm, is used to find the minimum cut of a graph. It repeatedly contracts random edges until only two vertices remain, and this process is repeated multiple times to increase the probability of finding the minimum cut.
Here’s a simplified implementation of Karger’s algorithm:
import random
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = []
def add_edge(self, u, v):
self.graph.append([u, v])
def find_parent(self, parent, i):
if parent[i] == i:
return i
return self.find_parent(parent, parent[i])
def union(self, parent, rank, x, y):
xroot = self.find_parent(parent, x)
yroot = self.find_parent(parent, y)
if rank[xroot] < rank[yroot]:
parent[xroot] = yroot
elif rank[xroot] > rank[yroot]:
parent[yroot] = xroot
else:
parent[yroot] = xroot
rank[xroot] += 1
def karger_min_cut(self):
parent = []
rank = []
for node in range(self.V):
parent.append(node)
rank.append(0)
vertices = self.V
while vertices > 2:
i = random.randint(0, len(self.graph) - 1)
subset1 = self.find_parent(parent, self.graph[i][0])
subset2 = self.find_parent(parent, self.graph[i][1])
if subset1 != subset2:
vertices -= 1
self.union(parent, rank, subset1, subset2)
cut_edges = 0
for i in range(len(self.graph)):
subset1 = self.find_parent(parent, self.graph[i][0])
subset2 = self.find_parent(parent, self.graph[i][1])
if subset1 != subset2:
cut_edges += 1
return cut_edges
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(0, 3)
g.add_edge(1, 2)
g.add_edge(2, 3)
print(f"Minimum cut: {g.karger_min_cut()}")
Applications of Randomized Algorithms
Randomized algorithms find applications in various areas of computer science and beyond:
1. Cryptography
Many cryptographic protocols rely on randomness for security. For example, generating random keys or nonces in encryption algorithms.
2. Machine Learning
Randomized algorithms are used in various machine learning techniques, such as random forests and stochastic gradient descent.
3. Big Data Analysis
When dealing with massive datasets, randomized algorithms can provide quick approximate answers. For instance, estimating the number of distinct elements in a stream of data.
4. Network Protocols
Randomization is used in network protocols to avoid collisions and improve efficiency. For example, the exponential backoff algorithm in Ethernet.
5. Computational Geometry
Many geometric algorithms use randomization to achieve better average-case performance. For instance, randomized incremental algorithms for constructing geometric structures.
Analyzing Randomized Algorithms
Analyzing randomized algorithms requires a different approach compared to deterministic algorithms. Here are some key aspects:
1. Expected Running Time
Instead of worst-case analysis, we often focus on the expected running time. This is the average time the algorithm takes over all possible random choices.
2. Probability of Correctness
For Monte Carlo algorithms, we analyze the probability that the algorithm gives the correct answer.
3. Tail Bounds
We often use probabilistic tail bounds (like Chernoff bounds or Markov’s inequality) to show that the algorithm’s performance is close to its expected performance with high probability.
4. Randomness Complexity
In addition to time and space complexity, we may analyze the number of random bits the algorithm needs.
Challenges and Considerations
While randomized algorithms offer many advantages, they also come with some challenges:
1. Reproducibility
The non-deterministic nature of randomized algorithms can make debugging and testing more challenging. It’s often necessary to use fixed seeds for random number generators to reproduce results.
2. Quality of Randomness
The performance of randomized algorithms can be affected by the quality of the random number generator used. In practice, we often use pseudo-random number generators, which may introduce subtle biases.
3. Theoretical vs. Practical Performance
While randomized algorithms may have better theoretical guarantees, their practical performance can sometimes be worse than deterministic alternatives due to factors like constant factors and cache effects.
Advanced Topics in Randomized Algorithms
As you delve deeper into the world of randomized algorithms, you’ll encounter more advanced concepts and techniques:
1. Derandomization
This is the process of converting a randomized algorithm into a deterministic one while preserving its performance guarantees. The method of conditional expectations is a common derandomization technique.
2. Lower Bounds for Randomized Algorithms
Proving lower bounds for randomized algorithms often involves information theory and communication complexity.
3. Online Algorithms with Randomization
Randomization can be particularly powerful in the online setting, where decisions must be made without knowledge of future inputs.
4. Quantum Algorithms
Quantum algorithms can be seen as a generalization of randomized algorithms, using quantum superposition instead of classical probability.
Conclusion
Randomized algorithms represent a powerful paradigm in algorithm design, offering a blend of simplicity, efficiency, and versatility. By harnessing the power of randomness, these algorithms can solve complex problems more efficiently than their deterministic counterparts in many cases.
As you continue your journey in computer science and algorithm design, keep randomized algorithms in your toolkit. They’re not just theoretical constructs but practical tools used in various real-world applications, from cryptography to big data analysis.
Remember, the key to mastering randomized algorithms is to think probabilistically. Instead of worst-case guarantees, focus on expected performance and high-probability bounds. With practice, you’ll develop an intuition for when and how to apply randomization to solve challenging computational problems.
As you explore more advanced topics in computer science, you’ll find that the concepts you’ve learned about randomized algorithms will serve you well, providing a foundation for understanding more complex ideas in areas like approximation algorithms, online algorithms, and even quantum computing.
Keep practicing, keep exploring, and don’t be afraid to embrace a little randomness in your algorithms. Happy coding!