Algorithms for Natural Language Understanding: Unlocking the Power of Human-Computer Communication
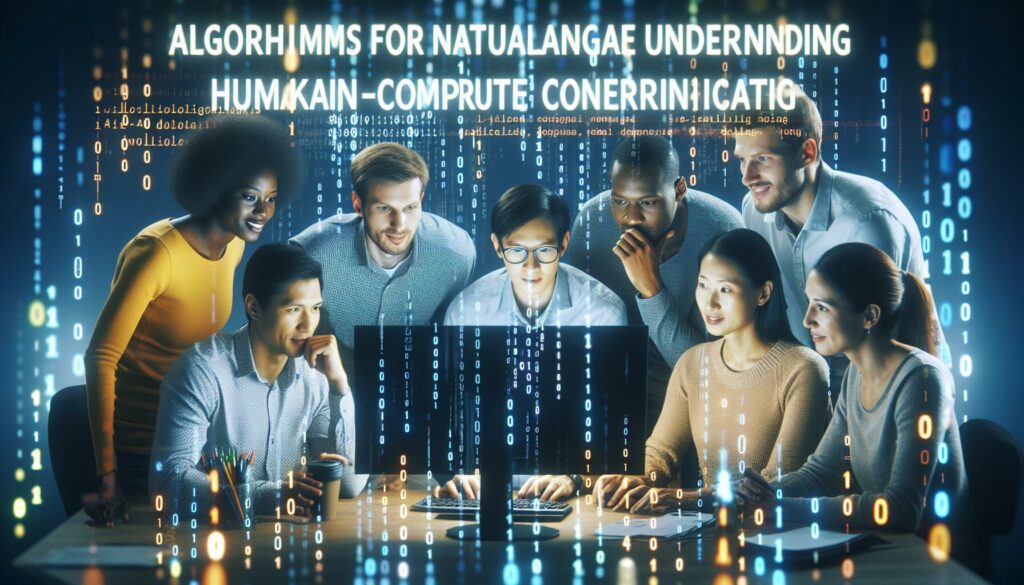
Natural Language Understanding (NLU) is a critical component of artificial intelligence that enables machines to comprehend and interpret human language. As technology continues to advance, the demand for sophisticated NLU algorithms has skyrocketed, driving innovation in fields such as virtual assistants, chatbots, and automated customer service. In this comprehensive guide, we’ll explore the fascinating world of algorithms for Natural Language Understanding, their applications, and how they’re shaping the future of human-computer interaction.
Table of Contents
- Introduction to Natural Language Understanding
- Key Concepts in NLU
- Text Preprocessing Techniques
- Tokenization Algorithms
- Part-of-Speech Tagging
- Named Entity Recognition
- Sentiment Analysis Algorithms
- Topic Modeling Techniques
- Word Embeddings and Distributed Representations
- Machine Learning Approaches for NLU
- Deep Learning in NLU
- Challenges and Future Directions
- Conclusion
1. Introduction to Natural Language Understanding
Natural Language Understanding is a subset of Natural Language Processing (NLP) that focuses on machine reading comprehension. While NLP deals with the interaction between computers and human language in general, NLU specifically aims to enable machines to understand and interpret the meaning behind human communication.
The goal of NLU is to bridge the gap between human communication and computer understanding. This involves tackling complex problems such as:
- Interpreting context and intent
- Handling ambiguity in language
- Understanding idiomatic expressions and figurative language
- Recognizing and interpreting emotions
- Extracting relevant information from text
As we delve deeper into the world of NLU algorithms, we’ll explore how these challenges are addressed through various techniques and approaches.
2. Key Concepts in NLU
Before we dive into specific algorithms, it’s essential to understand some fundamental concepts in Natural Language Understanding:
Syntax vs. Semantics
Syntax refers to the grammatical structure of language, while semantics deals with the meaning of words and sentences. NLU algorithms must handle both aspects to truly understand human language.
Pragmatics
Pragmatics involves understanding the context and intent behind language use. This is crucial for interpreting things like sarcasm, humor, and indirect requests.
Discourse Analysis
This involves understanding how sentences and ideas are connected within a larger context, such as a conversation or a document.
Ambiguity Resolution
Natural language is often ambiguous, with words and phrases having multiple possible meanings. NLU algorithms must be able to resolve these ambiguities based on context.
3. Text Preprocessing Techniques
Before applying more advanced NLU algorithms, it’s crucial to preprocess the text data. This step helps to clean and standardize the input, making it easier for subsequent algorithms to extract meaningful information. Common preprocessing techniques include:
Lowercasing
Converting all text to lowercase helps to standardize the input and reduce the vocabulary size.
def lowercase_text(text):
return text.lower()
Removing Punctuation
Eliminating punctuation can help reduce noise in the data.
import string
def remove_punctuation(text):
return text.translate(str.maketrans("", "", string.punctuation))
Removing Stop Words
Stop words are common words (e.g., “the”, “is”, “at”) that often don’t contribute much to the meaning of a text. Removing them can help focus on more important words.
from nltk.corpus import stopwords
def remove_stopwords(text):
stop_words = set(stopwords.words("english"))
return " ".join([word for word in text.split() if word.lower() not in stop_words])
Stemming and Lemmatization
These techniques reduce words to their root form, helping to normalize variations of the same word.
from nltk.stem import PorterStemmer, WordNetLemmatizer
def stem_text(text):
stemmer = PorterStemmer()
return " ".join([stemmer.stem(word) for word in text.split()])
def lemmatize_text(text):
lemmatizer = WordNetLemmatizer()
return " ".join([lemmatizer.lemmatize(word) for word in text.split()])
4. Tokenization Algorithms
Tokenization is the process of breaking down text into smaller units, typically words or subwords. This is a crucial step in many NLU tasks. Here are some common tokenization approaches:
Word Tokenization
This involves splitting text into individual words. While it may seem straightforward, challenges arise with contractions, hyphenated words, and multi-word expressions.
from nltk.tokenize import word_tokenize
def tokenize_words(text):
return word_tokenize(text)
Sentence Tokenization
Splitting text into sentences is important for tasks that operate at the sentence level, such as machine translation or summarization.
from nltk.tokenize import sent_tokenize
def tokenize_sentences(text):
return sent_tokenize(text)
Subword Tokenization
Techniques like Byte-Pair Encoding (BPE) or WordPiece tokenization break words into subword units, which can be particularly useful for handling out-of-vocabulary words and morphologically rich languages.
from tokenizers import ByteLevelBPETokenizer
def train_bpe_tokenizer(texts, vocab_size=30000):
tokenizer = ByteLevelBPETokenizer()
tokenizer.train_from_iterator(texts, vocab_size=vocab_size)
return tokenizer
def tokenize_subwords(text, tokenizer):
return tokenizer.encode(text).tokens
5. Part-of-Speech Tagging
Part-of-Speech (POS) tagging is the process of assigning grammatical categories (e.g., noun, verb, adjective) to each word in a text. This information is valuable for many NLU tasks, including named entity recognition and syntactic parsing.
Rule-Based POS Tagging
These algorithms use hand-crafted rules to assign POS tags based on word patterns and context.
Statistical POS Tagging
Statistical models, such as Hidden Markov Models (HMMs) or Maximum Entropy Markov Models (MEMMs), learn tag probabilities from annotated corpora.
Neural POS Tagging
Modern approaches use neural networks, particularly recurrent neural networks (RNNs) or transformers, to achieve state-of-the-art performance in POS tagging.
from nltk import pos_tag
def pos_tag_text(text):
tokens = word_tokenize(text)
return pos_tag(tokens)
6. Named Entity Recognition
Named Entity Recognition (NER) is the task of identifying and classifying named entities (e.g., person names, organizations, locations) in text. NER is crucial for many NLU applications, including information extraction and question answering.
Rule-Based NER
These systems use hand-crafted rules and gazetteers (predefined lists of entities) to identify named entities.
Machine Learning Approaches
Supervised learning techniques, such as Conditional Random Fields (CRFs) or Support Vector Machines (SVMs), can be trained on annotated data to recognize named entities.
Deep Learning for NER
Neural network architectures, particularly Bidirectional LSTMs with CRF layers or transformer-based models like BERT, have achieved state-of-the-art results in NER tasks.
from nltk import ne_chunk
def recognize_named_entities(text):
tokens = word_tokenize(text)
pos_tags = pos_tag(tokens)
return ne_chunk(pos_tags)
7. Sentiment Analysis Algorithms
Sentiment analysis involves determining the emotional tone or opinion expressed in a piece of text. This is valuable for understanding customer feedback, social media monitoring, and market research.
Lexicon-Based Approaches
These methods use predefined dictionaries of words associated with positive or negative sentiments to calculate an overall sentiment score.
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
return blob.sentiment.polarity
Machine Learning Classifiers
Supervised learning algorithms like Naive Bayes, Support Vector Machines, or Random Forests can be trained on labeled data to classify sentiment.
Deep Learning for Sentiment Analysis
Neural network models, such as Convolutional Neural Networks (CNNs) or Long Short-Term Memory (LSTM) networks, have shown excellent performance in capturing complex sentiment patterns.
8. Topic Modeling Techniques
Topic modeling is the process of discovering abstract topics that occur in a collection of documents. This is useful for content organization, recommendation systems, and trend analysis.
Latent Dirichlet Allocation (LDA)
LDA is a generative probabilistic model that represents documents as mixtures of topics, where each topic is a distribution over words.
from gensim import corpora
from gensim.models import LdaModel
def perform_lda(texts, num_topics=10):
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
lda_model = LdaModel(corpus=corpus, id2word=dictionary, num_topics=num_topics)
return lda_model
Non-Negative Matrix Factorization (NMF)
NMF is a linear algebra approach that factorizes the document-term matrix into two non-negative matrices, representing topics and their word distributions.
Neural Topic Models
Recent approaches use neural networks, such as variational autoencoders, to learn topic representations in an unsupervised manner.
9. Word Embeddings and Distributed Representations
Word embeddings are dense vector representations of words that capture semantic relationships. These representations are crucial for many modern NLU algorithms.
Word2Vec
Word2Vec uses shallow neural networks to learn word embeddings based on the context in which words appear.
from gensim.models import Word2Vec
def train_word2vec(sentences, vector_size=100, window=5, min_count=1):
model = Word2Vec(sentences, vector_size=vector_size, window=window, min_count=min_count)
return model
GloVe (Global Vectors)
GloVe learns word embeddings by factorizing the logarithm of the word co-occurrence matrix.
FastText
FastText extends Word2Vec by incorporating subword information, making it better suited for handling out-of-vocabulary words and morphologically rich languages.
10. Machine Learning Approaches for NLU
Machine learning algorithms play a crucial role in many NLU tasks. Here are some common approaches:
Naive Bayes
A probabilistic classifier based on Bayes’ theorem, often used for text classification tasks like spam detection or sentiment analysis.
from sklearn.naive_bayes import MultinomialNB
from sklearn.feature_extraction.text import CountVectorizer
def train_naive_bayes(texts, labels):
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(texts)
clf = MultinomialNB()
clf.fit(X, labels)
return vectorizer, clf
Support Vector Machines (SVM)
SVMs are powerful classifiers that find the optimal hyperplane to separate different classes in high-dimensional space.
Random Forests
An ensemble learning method that constructs multiple decision trees and combines their predictions, often used for various NLU classification tasks.
11. Deep Learning in NLU
Deep learning has revolutionized the field of NLU, enabling more sophisticated and accurate models. Some key deep learning architectures for NLU include:
Recurrent Neural Networks (RNNs)
RNNs, particularly Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) variants, are well-suited for sequential data like text.
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dense
def create_lstm_model(vocab_size, embedding_dim, max_length):
model = Sequential([
Embedding(vocab_size, embedding_dim, input_length=max_length),
LSTM(64),
Dense(1, activation="sigmoid")
])
model.compile(optimizer="adam", loss="binary_crossentropy", metrics=["accuracy"])
return model
Convolutional Neural Networks (CNNs)
While primarily used in computer vision, CNNs have shown excellent performance in text classification tasks.
Transformer Models
Transformer architectures, such as BERT, GPT, and their variants, have achieved state-of-the-art results in various NLU tasks through self-attention mechanisms and transfer learning.
from transformers import BertTokenizer, BertForSequenceClassification
def load_bert_model():
tokenizer = BertTokenizer.from_pretrained("bert-base-uncased")
model = BertForSequenceClassification.from_pretrained("bert-base-uncased")
return tokenizer, model
12. Challenges and Future Directions
Despite significant progress in NLU algorithms, several challenges remain:
Handling Ambiguity and Context
Natural language is inherently ambiguous, and understanding context remains a significant challenge for machines.
Multilingual and Cross-lingual NLU
Developing NLU systems that work across multiple languages or can transfer knowledge between languages is an active area of research.
Common Sense Reasoning
Incorporating common sense knowledge and reasoning abilities into NLU systems is crucial for achieving human-like understanding.
Ethical Considerations
As NLU systems become more powerful, addressing biases, privacy concerns, and ethical use of these technologies becomes increasingly important.
Efficient and Interpretable Models
Developing NLU models that are both computationally efficient and interpretable is essential for real-world applications and trust in AI systems.
13. Conclusion
Natural Language Understanding is a rapidly evolving field at the intersection of linguistics, computer science, and artificial intelligence. The algorithms and techniques discussed in this article form the foundation of modern NLU systems, enabling machines to comprehend and interact with human language in increasingly sophisticated ways.
As research in NLU continues to advance, we can expect even more powerful and nuanced language understanding capabilities. These advancements will drive innovations in areas such as virtual assistants, automated customer service, content analysis, and human-computer interaction.
For developers and AI enthusiasts, mastering these NLU algorithms and staying updated with the latest developments in the field is crucial. By leveraging these techniques, you can create intelligent applications that truly understand and respond to human language, opening up a world of possibilities in the realm of artificial intelligence and beyond.
As we continue to push the boundaries of what’s possible in Natural Language Understanding, the dream of seamless human-computer communication comes ever closer to reality. The future of NLU is bright, and the potential applications are limited only by our imagination and ingenuity.