Implementing Skip Lists for Fast Search: A Comprehensive Guide
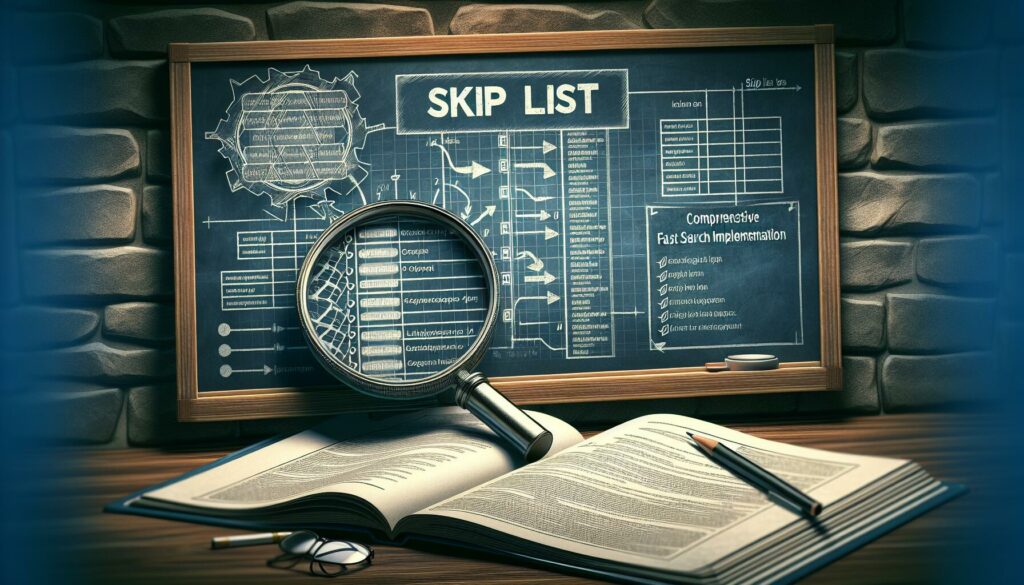
In the world of data structures and algorithms, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and improve search operations. One such data structure that offers an elegant solution for fast search operations is the skip list. In this comprehensive guide, we’ll dive deep into the concept of skip lists, understand their implementation, and explore how they can significantly enhance search performance in your applications.
Table of Contents
- What are Skip Lists?
- How Skip Lists Work
- Advantages of Skip Lists
- Implementing Skip Lists
- Time Complexity Analysis
- Use Cases for Skip Lists
- Comparison with Other Data Structures
- Optimization Techniques
- Conclusion
1. What are Skip Lists?
Skip lists are a probabilistic data structure that allows for fast search, insertion, and deletion operations. Invented by William Pugh in 1989, skip lists provide an alternative to balanced trees and offer a simpler implementation with comparable performance.
At its core, a skip list is a series of linked lists stacked on top of each other, with each level skipping over some elements of the level below it. This hierarchical structure allows for quick navigation through the data, significantly reducing the time required for search operations.
2. How Skip Lists Work
To understand how skip lists work, let’s break down their structure and search process:
Structure:
- The bottom level is a regular sorted linked list containing all elements.
- Each higher level acts as an “express lane” for the lists below, skipping over some elements.
- The number of levels and which elements to promote to higher levels are determined probabilistically.
Search Process:
- Start at the highest level of the skip list.
- Compare the current element with the search key.
- If the current element is smaller, move right.
- If the current element is larger or we’ve reached the end of the level, move down to the next level.
- Repeat steps 2-4 until the element is found or we’ve determined it doesn’t exist.
This process allows us to quickly eliminate large portions of the data set, resulting in faster search times compared to a standard linked list.
3. Advantages of Skip Lists
Skip lists offer several advantages that make them an attractive choice for certain applications:
- Efficient Search: O(log n) average time complexity for search operations.
- Simple Implementation: Easier to implement and maintain compared to balanced trees.
- Probabilistic Balance: No need for complex rebalancing operations.
- Memory Efficiency: Can be more memory-efficient than some tree structures.
- Parallelism: Allows for concurrent access and modifications with proper synchronization.
4. Implementing Skip Lists
Let’s implement a basic skip list in Python to illustrate its structure and operations:
import random
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.forward = []
class SkipList:
def __init__(self, max_level, p):
self.max_level = max_level
self.p = p
self.header = Node(None, None)
self.level = 0
def random_level(self):
lvl = 0
while random.random() < self.p and lvl < self.max_level:
lvl += 1
return lvl
def insert(self, key, value):
update = [None] * (self.max_level + 1)
current = self.header
for i in range(self.level, -1, -1):
while current.forward[i] and current.forward[i].key < key:
current = current.forward[i]
update[i] = current
current = current.forward[0]
if current is None or current.key != key:
lvl = self.random_level()
if lvl > self.level:
for i in range(self.level + 1, lvl + 1):
update[i] = self.header
self.level = lvl
new_node = Node(key, value)
for i in range(lvl + 1):
new_node.forward.append(update[i].forward[i])
update[i].forward[i] = new_node
def search(self, key):
current = self.header
for i in range(self.level, -1, -1):
while current.forward[i] and current.forward[i].key < key:
current = current.forward[i]
current = current.forward[0]
if current and current.key == key:
return current.value
return None
def delete(self, key):
update = [None] * (self.max_level + 1)
current = self.header
for i in range(self.level, -1, -1):
while current.forward[i] and current.forward[i].key < key:
current = current.forward[i]
update[i] = current
current = current.forward[0]
if current and current.key == key:
for i in range(self.level + 1):
if update[i].forward[i] != current:
break
update[i].forward[i] = current.forward[i]
while self.level > 0 and not self.header.forward[self.level]:
self.level -= 1
def display(self):
for level in range(self.level, -1, -1):
print(f"Level {level}: ", end="")
node = self.header.forward[level]
while node:
print(f"({node.key}: {node.value})", end=" -> ")
node = node.forward[level]
print("None")
This implementation includes the basic operations of insertion, search, and deletion, as well as a display method to visualize the skip list structure.
Usage Example:
skip_list = SkipList(max_level=4, p=0.5)
skip_list.insert(3, "Value 3")
skip_list.insert(6, "Value 6")
skip_list.insert(7, "Value 7")
skip_list.insert(9, "Value 9")
skip_list.insert(12, "Value 12")
skip_list.insert(19, "Value 19")
skip_list.insert(17, "Value 17")
skip_list.insert(26, "Value 26")
skip_list.insert(21, "Value 21")
skip_list.insert(25, "Value 25")
skip_list.display()
print(f"Search for key 19: {skip_list.search(19)}")
skip_list.delete(19)
print("After deleting 19:")
skip_list.display()
5. Time Complexity Analysis
The time complexity of skip list operations is as follows:
- Search: O(log n) average case, O(n) worst case
- Insert: O(log n) average case, O(n) worst case
- Delete: O(log n) average case, O(n) worst case
The logarithmic time complexity is achieved due to the skip list’s ability to skip over large portions of the data during search operations. However, in the worst case (when all elements are in a single level), the performance degrades to linear time.
6. Use Cases for Skip Lists
Skip lists are particularly useful in scenarios where fast search and efficient insertion/deletion are required. Some common use cases include:
- In-memory databases: For fast lookups and range queries
- File systems: To manage and search large directory structures
- Peer-to-peer systems: For efficient routing and data location
- Caching systems: To implement efficient LRU (Least Recently Used) caches
- Sorted data storage: When frequent insertions and deletions are needed
7. Comparison with Other Data Structures
Let’s compare skip lists with other common data structures used for similar purposes:
Skip Lists vs. Balanced Trees (e.g., AVL Trees, Red-Black Trees):
- Complexity: Both have O(log n) average time complexity for main operations.
- Implementation: Skip lists are generally simpler to implement and maintain.
- Balance: Skip lists use probabilistic balance, while trees require explicit rebalancing.
- Memory usage: Skip lists can use more memory due to multiple levels.
Skip Lists vs. Hash Tables:
- Ordered data: Skip lists maintain order, hash tables do not.
- Range queries: Efficient in skip lists, not supported in hash tables.
- Collision handling: Not an issue in skip lists, crucial in hash tables.
- Worst-case performance: Skip lists degrade more gracefully than hash tables.
Skip Lists vs. Linked Lists:
- Search efficiency: Skip lists are much faster (O(log n) vs. O(n)).
- Memory overhead: Skip lists use more memory for the express lanes.
- Insertion/Deletion: Both support efficient insertions and deletions.
8. Optimization Techniques
To further enhance the performance of skip lists, consider the following optimization techniques:
1. Optimizing Level Generation:
Instead of using random number generation for each level, you can use bit manipulation techniques to generate levels more efficiently:
def optimized_random_level(self):
level = 0
random_bits = random.getrandbits(32)
while random_bits & 1 and level < self.max_level:
level += 1
random_bits >>= 1
return level
2. Memory-Efficient Node Structure:
Use a more compact node structure to reduce memory overhead:
class CompactNode:
__slots__ = ['key', 'value', 'forward']
def __init__(self, key, value, level):
self.key = key
self.value = value
self.forward = [None] * (level + 1)
3. Adaptive Maximum Level:
Dynamically adjust the maximum level based on the number of elements:
def adaptive_max_level(self):
return max(1, int(math.log2(self.size)))
4. Batch Updates:
For scenarios with frequent updates, implement batch insert and delete operations to amortize the cost of level adjustments.
5. Concurrent Skip Lists:
For multi-threaded environments, implement lock-free or fine-grained locking mechanisms to allow concurrent access and modifications.
9. Conclusion
Skip lists are a powerful and elegant data structure that offer an excellent balance between simplicity and performance. Their probabilistic nature and hierarchical structure make them well-suited for a wide range of applications, particularly those requiring fast search operations and frequent insertions/deletions.
By implementing skip lists in your projects, you can achieve logarithmic time complexity for key operations while maintaining a relatively simple codebase. The flexibility and ease of implementation make skip lists an attractive alternative to more complex balanced tree structures in many scenarios.
As you continue to explore advanced data structures and algorithms, consider how skip lists might fit into your toolkit. Their unique properties and performance characteristics could be just what you need to optimize your next search-intensive application or to ace that technical interview at a major tech company.
Remember, the key to mastering data structures like skip lists is practice and experimentation. Try implementing your own skip list, test it with various datasets, and compare its performance against other data structures. This hands-on experience will deepen your understanding and help you make informed decisions about when and how to use skip lists in your future projects.