Algorithms in Virtual Reality and Gaming: Powering Immersive Experiences
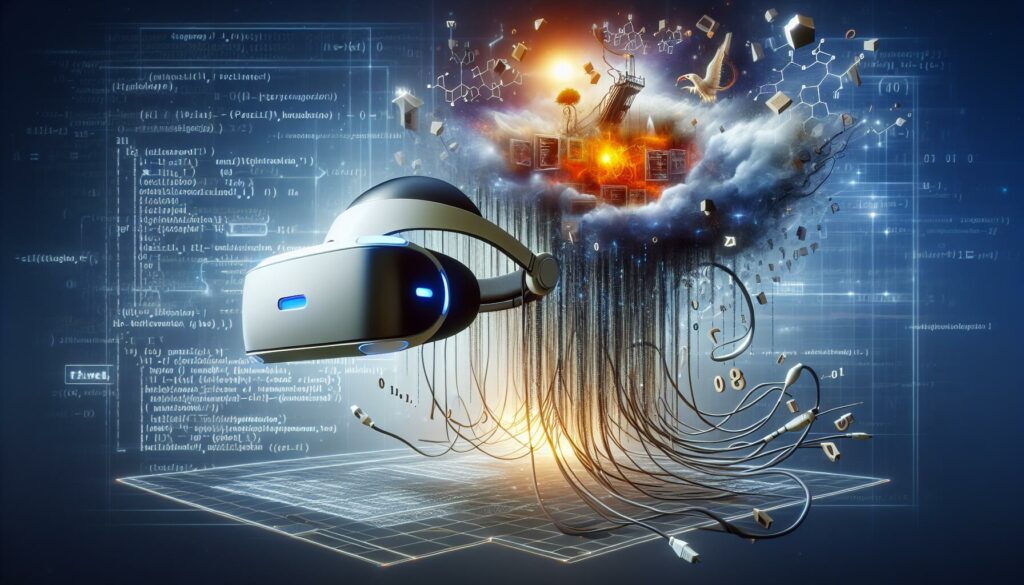
In the ever-evolving landscape of technology, virtual reality (VR) and gaming have emerged as two of the most exciting and rapidly advancing fields. At the heart of these immersive experiences lie complex algorithms that power everything from realistic graphics to intelligent non-player characters (NPCs). As the gaming industry continues to push the boundaries of what’s possible, understanding the role of algorithms in VR and gaming becomes increasingly important for developers, enthusiasts, and aspiring programmers alike.
In this comprehensive guide, we’ll explore the fascinating world of algorithms in virtual reality and gaming. We’ll delve into the key concepts, techniques, and challenges that define this cutting-edge field, and examine how algorithms are shaping the future of interactive entertainment.
Table of Contents
- Introduction to Algorithms in VR and Gaming
- Graphics and Rendering Algorithms
- Physics Simulation Algorithms
- Artificial Intelligence and Game AI Algorithms
- Procedural Generation Algorithms
- Networking and Multiplayer Algorithms
- Optimization Algorithms for VR and Gaming
- Audio Processing Algorithms
- Input Processing and Motion Tracking Algorithms
- Challenges and Future Directions
- Conclusion
1. Introduction to Algorithms in VR and Gaming
Algorithms are the backbone of modern virtual reality and gaming experiences. They are responsible for transforming raw data and user inputs into the immersive, interactive worlds we explore in games and VR applications. From rendering lifelike graphics to simulating complex physics, algorithms play a crucial role in creating believable and engaging virtual environments.
The use of algorithms in VR and gaming spans a wide range of applications, including:
- Graphics rendering and real-time 3D visualization
- Physics simulations for realistic object interactions
- Artificial intelligence for non-player characters and game logic
- Procedural content generation for diverse and dynamic environments
- Networking and multiplayer synchronization
- Performance optimization for smooth gameplay
- Audio processing for immersive soundscapes
- Input processing and motion tracking for VR interactions
As we explore each of these areas in detail, we’ll see how algorithms are pushing the boundaries of what’s possible in virtual reality and gaming.
2. Graphics and Rendering Algorithms
Graphics and rendering algorithms are at the forefront of creating visually stunning and immersive experiences in VR and gaming. These algorithms are responsible for transforming 3D models, textures, and lighting information into the 2D images we see on our screens or in VR headsets.
Key Graphics Algorithms:
- Rasterization: The process of converting 3D geometry into 2D pixels on the screen.
- Ray Tracing: A technique that simulates the path of light rays to create highly realistic lighting and reflections.
- Tessellation: The process of subdividing 3D models to increase detail and smoothness.
- Texture Mapping: Applying 2D images to 3D surfaces to add detail and realism.
- Ambient Occlusion: Simulating soft shadows and indirect lighting for added depth and realism.
- Anti-Aliasing: Techniques to reduce jagged edges and improve image quality.
One of the most significant challenges in graphics rendering for VR and gaming is achieving high frame rates while maintaining visual quality. This is particularly crucial in VR, where low frame rates can lead to motion sickness and a poor user experience.
To address this challenge, developers often employ various optimization techniques and algorithms, such as:
- Level of Detail (LOD) algorithms: Dynamically adjusting the complexity of 3D models based on their distance from the camera.
- Occlusion culling: Identifying and skipping the rendering of objects that are not visible to the camera.
- Frustum culling: Determining which objects are within the camera’s field of view and only rendering those.
- Spatial data structures: Using techniques like octrees or BVH (Bounding Volume Hierarchy) to efficiently organize and query 3D scene data.
Here’s a simple example of how frustum culling might be implemented in pseudocode:
function isObjectVisible(object, camera):
frustum = camera.getFrustum()
boundingBox = object.getBoundingBox()
for each plane in frustum:
if boundingBox is entirely behind plane:
return false
return true
function renderScene(scene, camera):
for each object in scene:
if isObjectVisible(object, camera):
render(object)
As graphics hardware and algorithms continue to evolve, we’re seeing increasingly sophisticated techniques being employed in VR and gaming. Real-time ray tracing, for example, is becoming more prevalent, allowing for incredibly realistic lighting and reflections that were previously only possible in pre-rendered scenes.
3. Physics Simulation Algorithms
Physics simulation is a crucial component of creating believable and interactive virtual environments. In both VR and gaming, physics algorithms are used to simulate the behavior of objects, fluids, cloth, and even entire worlds. These simulations add a layer of realism and interactivity that greatly enhances the user’s immersion.
Key Physics Simulation Algorithms:
- Rigid Body Dynamics: Simulating the motion of solid objects that don’t deform.
- Soft Body Dynamics: Modeling deformable objects like cloth, rubber, or flesh.
- Particle Systems: Simulating phenomena like fire, smoke, or water using large numbers of individual particles.
- Collision Detection: Identifying when objects intersect or come into contact with each other.
- Constraint Solvers: Enforcing physical constraints between objects, such as joints or hinges.
- Fluid Dynamics: Simulating the behavior of liquids and gases.
One of the main challenges in physics simulation for VR and gaming is balancing accuracy with performance. While we want our simulations to be as realistic as possible, we also need them to run in real-time, often at high frame rates.
To achieve this balance, developers often use simplified physics models and various optimization techniques. For example, instead of simulating every single particle in a fluid, we might use a grid-based approach that approximates the fluid’s behavior at a larger scale.
Here’s a simple example of how a basic particle system might be implemented:
class Particle:
def __init__(self, position, velocity):
self.position = position
self.velocity = velocity
self.acceleration = Vector3(0, -9.8, 0) # Gravity
def update(self, dt):
self.velocity += self.acceleration * dt
self.position += self.velocity * dt
class ParticleSystem:
def __init__(self):
self.particles = []
def emit_particle(self, position, velocity):
self.particles.append(Particle(position, velocity))
def update(self, dt):
for particle in self.particles:
particle.update(dt)
# Remove particles that have fallen below a certain height
self.particles = [p for p in self.particles if p.position.y > -10]
def render(self):
for particle in self.particles:
render_particle(particle.position)
In VR applications, physics simulations take on added importance as they directly impact the user’s sense of presence and immersion. For example, when a user reaches out to grab an object in VR, the physics simulation needs to accurately model the object’s weight, inertia, and how it responds to the user’s actions.
4. Artificial Intelligence and Game AI Algorithms
Artificial Intelligence (AI) plays a crucial role in creating dynamic, responsive, and challenging experiences in both VR and gaming. Game AI encompasses a wide range of techniques and algorithms designed to create intelligent behavior in non-player characters (NPCs), control game systems, and adapt to player actions.
Key AI Algorithms in Gaming and VR:
- Pathfinding: Algorithms like A* for finding optimal routes through complex environments.
- Behavior Trees: A hierarchical structure for defining complex AI behaviors.
- State Machines: A simple but effective way to model different AI states and transitions.
- Decision Trees: Used for making decisions based on various game conditions.
- Neural Networks: For learning and adapting AI behaviors based on player actions.
- Genetic Algorithms: Used for evolving and optimizing AI strategies over time.
- Fuzzy Logic: Allowing for more nuanced decision-making in uncertain conditions.
One of the most common and important AI algorithms in gaming is pathfinding. The A* (A-star) algorithm is widely used for finding the shortest path between two points in a game world. Here’s a simplified implementation of A* in Python:
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(start, goal, graph):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {start: None}
cost_so_far = {start: 0}
while frontier:
current = heapq.heappop(frontier)[1]
if current == goal:
break
for next in graph[current]:
new_cost = cost_so_far[current] + 1
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
return came_from, cost_so_far
In VR applications, AI can be used to create more immersive and interactive environments. For example, AI-driven NPCs can respond realistically to the player’s presence and actions, enhancing the sense of being in a living, breathing world.
Another important aspect of AI in gaming and VR is procedural content generation. This involves using algorithms to create game content such as levels, quests, or even entire worlds. Procedural generation can greatly increase the variety and replayability of games, as well as reduce development time and costs.
5. Procedural Generation Algorithms
Procedural generation is a technique used in both gaming and VR to create vast amounts of content algorithmically, rather than manually. This approach can create diverse and seemingly infinite worlds, levels, textures, and even music, all generated on-the-fly.
Key Procedural Generation Techniques:
- Noise Functions: Such as Perlin noise or Simplex noise, used for generating natural-looking terrains and textures.
- L-Systems: Used for generating plant-like structures and fractals.
- Cellular Automata: For creating complex patterns from simple rules, often used for cave systems or mazes.
- Wave Function Collapse: A constraint-based algorithm for generating structured content.
- Grammars and Rule Systems: For generating structured content like quests or narratives.
Here’s a simple example of using Perlin noise to generate a 2D heightmap for terrain:
import numpy as np
from noise import pnoise2
def generate_terrain(width, height, scale=50.0, octaves=6, persistence=0.5, lacunarity=2.0):
terrain = np.zeros((width, height))
for i in range(width):
for j in range(height):
terrain[i][j] = pnoise2(i/scale,
j/scale,
octaves=octaves,
persistence=persistence,
lacunarity=lacunarity)
return terrain
# Generate a 256x256 terrain
terrain = generate_terrain(256, 256)
# You can now use this terrain data to create a 3D mesh or 2D map
Procedural generation is particularly valuable in VR applications, where creating large, detailed environments manually can be prohibitively time-consuming and resource-intensive. By using procedural techniques, developers can create vast, explorable worlds that feel unique and varied.
However, procedural generation also comes with challenges. Ensuring that generated content is balanced, interesting, and meets specific design criteria can be difficult. Developers often need to carefully tune their algorithms and combine procedural techniques with hand-crafted content to achieve the best results.
6. Networking and Multiplayer Algorithms
Networking is a crucial aspect of many modern games and VR experiences, enabling multiplayer interactions and shared virtual worlds. Implementing efficient and reliable networking systems requires specialized algorithms to handle issues like latency, packet loss, and synchronization.
Key Networking Algorithms and Techniques:
- Client-Server Architecture: The most common model for multiplayer games, where a central server manages the game state.
- Peer-to-Peer Networking: An alternative model where players connect directly to each other.
- State Synchronization: Algorithms for keeping game state consistent across multiple clients.
- Lag Compensation: Techniques to mitigate the effects of network latency on gameplay.
- Dead Reckoning: Predicting the position of objects based on their last known state and velocity.
- Delta Compression: Reducing network traffic by only sending changes in game state.
One common challenge in networked games is implementing smooth movement for remote players. Here’s a simple example of client-side prediction and reconciliation:
class Player:
def __init__(self):
self.position = Vector3(0, 0, 0)
self.velocity = Vector3(0, 0, 0)
self.input_sequence = 0
def apply_input(self, input, dt):
# Apply input to change velocity
self.velocity += input * dt
# Update position
self.position += self.velocity * dt
def update(self, dt):
self.apply_input(self.current_input, dt)
self.input_sequence += 1
class ClientPlayer(Player):
def reconcile(self, server_state):
if server_state.sequence > self.last_acknowledged_sequence:
# Rewind to last acknowledged state
self.position = server_state.position
self.velocity = server_state.velocity
# Re-apply all inputs since then
for input in self.unacknowledged_inputs:
self.apply_input(input, dt)
self.last_acknowledged_sequence = server_state.sequence
In VR multiplayer experiences, networking becomes even more critical. The high frame rates and low latency requirements of VR make it challenging to create smooth, synchronized experiences across multiple users. Techniques like local rendering and prediction become essential to maintain the illusion of a shared space.
7. Optimization Algorithms for VR and Gaming
Optimization is a crucial aspect of both VR and gaming development. With the high performance demands of these applications, developers need to employ a wide range of optimization techniques to ensure smooth, responsive experiences.
Key Optimization Techniques:
- Spatial Partitioning: Techniques like quadtrees or octrees to efficiently organize and query spatial data.
- Level of Detail (LOD): Dynamically adjusting the complexity of objects based on their distance from the camera.
- Occlusion Culling: Avoiding the rendering of objects that are not visible to the camera.
- Texture Atlasing: Combining multiple textures into a single large texture to reduce draw calls.
- Instancing: Rendering multiple copies of the same object efficiently.
- Multithreading: Utilizing multiple CPU cores for parallel processing.
Here’s a simple example of implementing a quadtree for 2D spatial partitioning:
class QuadTree:
def __init__(self, boundary, capacity):
self.boundary = boundary
self.capacity = capacity
self.points = []
self.divided = False
def insert(self, point):
if not self.boundary.contains(point):
return False
if len(self.points) < self.capacity:
self.points.append(point)
return True
if not self.divided:
self.subdivide()
return (self.northeast.insert(point) or
self.northwest.insert(point) or
self.southeast.insert(point) or
self.southwest.insert(point))
def subdivide(self):
x = self.boundary.x
y = self.boundary.y
w = self.boundary.w / 2
h = self.boundary.h / 2
ne = Rectangle(x + w, y - h, w, h)
self.northeast = QuadTree(ne, self.capacity)
nw = Rectangle(x - w, y - h, w, h)
self.northwest = QuadTree(nw, self.capacity)
se = Rectangle(x + w, y + h, w, h)
self.southeast = QuadTree(se, self.capacity)
sw = Rectangle(x - w, y + h, w, h)
self.southwest = QuadTree(sw, self.capacity)
self.divided = True
def query(self, range):
found = []
if not self.boundary.intersects(range):
return found
for p in self.points:
if range.contains(p):
found.append(p)
if self.divided:
found.extend(self.northwest.query(range))
found.extend(self.northeast.query(range))
found.extend(self.southwest.query(range))
found.extend(self.southeast.query(range))
return found
In VR applications, optimization becomes even more critical due to the need for high frame rates and low latency. Techniques like foveated rendering, which reduces detail in the peripheral vision, can significantly improve performance in VR without noticeably impacting visual quality.
8. Audio Processing Algorithms
Audio plays a crucial role in creating immersive experiences in both VR and gaming. Advanced audio processing algorithms are used to create realistic 3D soundscapes, simulate acoustic environments, and enhance the overall audio experience.
Key Audio Processing Techniques:
- 3D Sound Positioning: Algorithms for accurately placing sounds in 3D space.
- Head-Related Transfer Functions (HRTF): Used to simulate how sound is perceived by the human ear based on its direction.
- Reverb and Echo Simulation: Creating realistic acoustic environments.
- Doppler Effect Simulation: Mimicking the change in pitch of moving sound sources.
- Adaptive Audio: Dynamically adjusting audio based on game state or player actions.
- Procedural Audio Generation: Creating sound effects algorithmically.
Here’s a simple example of how you might implement basic 3D sound positioning:
import math
def calculate_3d_audio(listener_pos, sound_pos, sound_volume):
# Calculate distance between listener and sound source
dx = sound_pos[0] - listener_pos[0]
dy = sound_pos[1] - listener_pos[1]
dz = sound_pos[2] - listener_pos[2]
distance = math.sqrt(dx*dx + dy*dy + dz*dz)
# Calculate attenuation based on distance
attenuation = 1.0 / (1.0 + distance)
# Calculate final volume
final_volume = sound_volume * attenuation
# Calculate stereo panning (simplified)
pan = dx / (abs(dx) + abs(dz))
return final_volume, pan
# Example usage
listener_position = (0, 0, 0)
sound_position = (5, 0, 5)
sound_volume = 1.0
volume, pan = calculate_3d_audio(listener_position, sound_position, sound_volume)
print(f"Volume: {volume}, Pan: {pan}")
In VR applications, accurate and responsive audio is essential for maintaining presence and immersion. Techniques like binaural audio rendering become particularly important, as they can create a convincing sense of sound directionality and space.
9. Input Processing and Motion Tracking Algorithms
Input processing and motion tracking are critical components of both gaming and VR experiences. These algorithms are responsible for interpreting user inputs, tracking body movements, and translating them into in-game actions or VR interactions.
Key Input Processing and Motion Tracking Techniques:
- Gesture Recognition: Algorithms for identifying specific hand or body movements.
- Inverse Kinematics (IK): Used for realistic body and limb movements in VR.
- Kalman Filters: For smoothing and predicting motion in tracking systems.
- Sensor Fusion: Combining data from multiple sensors (e.g., accelerometers, gyroscopes) for accurate tracking.
- Machine Learning Models: For advanced gesture recognition and prediction.
Here’s a simple example of how you might implement basic gesture recognition using a distance-based approach:
import numpy as np
def dtw_distance(s1, s2):
dtw = np.zeros((len(s1) + 1, len(s2) + 1))
for i in range(1, len(s1) + 1):
for j in range(1, len(s2) + 1):
cost = abs(s1[i-1] - s2[j-1])
dtw[i, j] = cost + min(dtw[i-1, j], dtw[i, j-1], dtw[i-1, j-1])
return dtw[-1, -1]
def recognize_gesture(input_gesture, gesture_database):
min_distance = float('inf')
recognized_gesture = None
for name, gesture in gesture_database.items():
distance = dtw_distance(input_gesture, gesture)
if distance < min_distance:
min_distance = distance
recognized_gesture = name
return recognized_gesture
# Example usage
gesture_database = {
"swipe_right": [0, 1, 2, 3, 4],
"swipe_left": [4, 3, 2, 1, 0],
"circle": [0, 1, 2, 3, 2, 1, 0]
}
input_gesture = [0, 1, 2, 3, 3, 4]
result = recognize_gesture(input_gesture, gesture_database)
print(f"Recognized gesture: {result}")
In VR applications, accurate and low-latency motion tracking is crucial for maintaining presence and preventing motion sickness. Advanced techniques like simultaneous localization and mapping (SLAM) are often used to track the user’s position and orientation in space.
10. Challenges and Future Directions
As VR and gaming technologies continue to evolve, developers face numerous challenges and exciting opportunities for innovation. Some of the key areas of focus include:
- Improving Performance: Continually optimizing algorithms to achieve higher frame rates and lower latency, especially for VR applications.
- Enhancing Realism: Developing more sophisticated physics simulations, AI behaviors, and graphics rendering techniques to create increasingly lifelike virtual worlds.
- Scaling Virtual Worlds: Creating larger, more detailed, and more dynamic environments while maintaining performance.
- Improving Accessibility: Developing algorithms and techniques to make VR and gaming experiences more accessible to users with different abilities.
- Advancing AI: Creating more intelligent and responsive NPCs and game systems using advanced machine learning techniques.
- Enhancing Haptic Feedback: Developing algorithms for more realistic and immersive tactile sensations in VR.
- Improving Motion Sickness Mitigation: Refining techniques to reduce discomfort in VR experiences.
As these challenges are addressed, we can expect to see increasingly immersive, realistic, and engaging VR and gaming experiences in the future.
11. Conclusion
Algorithms play a fundamental role in shaping the future of virtual reality and gaming. From graphics rendering and physics simulations to AI and procedural generation, these computational techniques are the building blocks of immersive digital experiences.
As we’ve explored in this article, the field of VR and gaming algorithms is vast and multifaceted, encompassing a wide range of disciplines from computer graphics and artificial intelligence to networking and audio processing. By understanding and leveraging these algorithms, developers can create increasingly sophisticated, realistic, and engaging virtual worlds.
The future of VR and gaming is bright, with ongoing advancements in hardware capabilities and software techniques continually pushing the boundaries of what’s possible. As these technologies evolve, so too will the algorithms that power them, opening up new possibilities for immersive storytelling, interactive entertainment, and virtual experiences.
Whether you’re a developer, a gamer, or simply someone interested in the cutting edge of technology, the world of algorithms in VR and gaming offers endless opportunities for exploration and innovation. As we look to the future, it’s clear that these algorithms will continue to play a crucial role in shaping the virtual worlds of tomorrow.