The Science Behind Compression Algorithms: Unraveling Data Efficiency
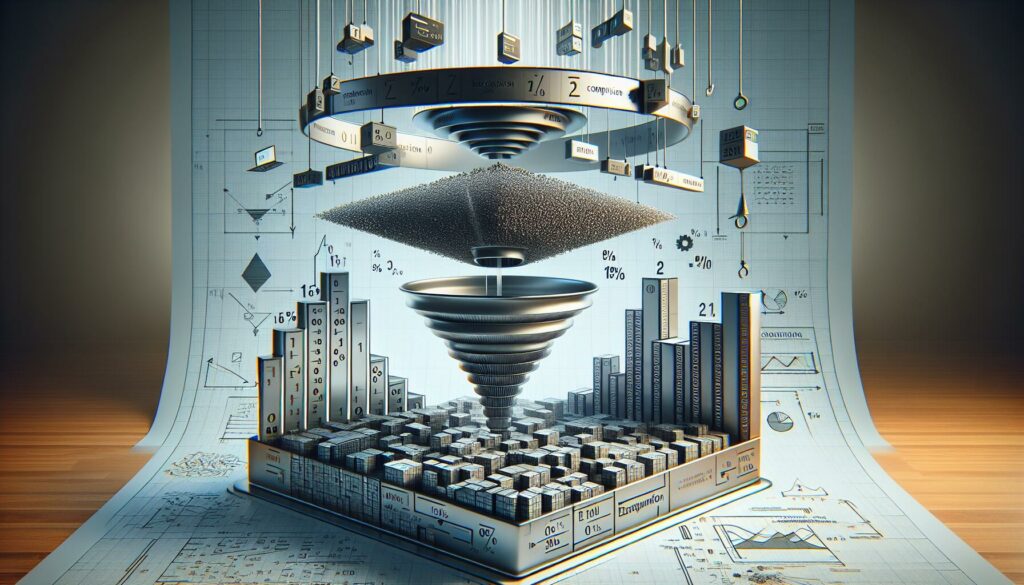
In the vast landscape of computer science and data management, compression algorithms stand as unsung heroes, silently working behind the scenes to make our digital world more efficient. These algorithms are the backbone of file compression, data transmission, and storage optimization, playing a crucial role in how we interact with and manage information in the digital age. In this comprehensive exploration, we’ll dive deep into the science behind compression algorithms, unraveling their inner workings, and understanding their significance in modern computing.
Understanding Compression: The Basics
At its core, compression is the process of encoding information using fewer bits than the original representation. This reduction in data size serves two primary purposes:
- Reducing storage requirements
- Decreasing transmission times
Compression algorithms achieve these goals by identifying and eliminating redundancies in data, essentially finding more efficient ways to represent the same information. But how exactly do they accomplish this feat?
Types of Compression Algorithms
Compression algorithms can be broadly categorized into two main types:
1. Lossless Compression
Lossless compression algorithms reduce file size without losing any data. When the compressed file is decompressed, it is identical to the original. This type of compression is crucial for applications where data integrity is paramount, such as:
- Text documents
- Executable files
- Source code
2. Lossy Compression
Lossy compression algorithms achieve higher compression ratios by selectively discarding some data. While this results in a loss of information, it’s often imperceptible or acceptable for certain types of data, such as:
- Images
- Audio
- Video
The choice between lossless and lossy compression depends on the specific use case and the nature of the data being compressed.
The Science Behind Lossless Compression
Let’s delve into some of the most common lossless compression techniques and the scientific principles behind them:
Run-Length Encoding (RLE)
Run-Length Encoding is one of the simplest forms of data compression. It works by replacing sequences of identical data elements (runs) with a single data value and count.
For example, the string “AABBBCCCC” could be encoded as “2A3B4C”.
Here’s a simple implementation of RLE in Python:
def run_length_encode(data):
encoded = ''
count = 1
for i in range(1, len(data)):
if data[i] == data[i-1]:
count += 1
else:
encoded += str(count) + data[i-1]
count = 1
encoded += str(count) + data[-1]
return encoded
# Example usage
original = "AABBBCCCC"
compressed = run_length_encode(original)
print(f"Original: {original}")
print(f"Compressed: {compressed}")
RLE is particularly effective for data with long runs of repeated values, such as simple graphics or certain types of scientific data.
Huffman Coding
Huffman coding is a more sophisticated lossless compression technique that assigns variable-length codes to characters based on their frequency of occurrence. More frequent characters are assigned shorter codes, while less frequent characters get longer codes.
The algorithm works as follows:
- Count the frequency of each character in the data.
- Build a binary tree where each leaf represents a character, and the path from the root to a leaf represents its code.
- Assign shorter paths to more frequent characters.
Here’s a simplified implementation of Huffman coding in Python:
import heapq
from collections import Counter
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(data):
# Count frequency of each character
frequency = Counter(data)
# Create a priority queue of nodes
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
# Build the Huffman tree
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
internal = Node(None, left.freq + right.freq)
internal.left = left
internal.right = right
heapq.heappush(heap, internal)
return heap[0]
def generate_codes(root, current_code, codes):
if root is None:
return
if root.char is not None:
codes[root.char] = current_code
return
generate_codes(root.left, current_code + '0', codes)
generate_codes(root.right, current_code + '1', codes)
# Example usage
data = "this is an example for huffman encoding"
root = build_huffman_tree(data)
codes = {}
generate_codes(root, '', codes)
print("Huffman Codes:")
for char, code in codes.items():
print(f"{char}: {code}")
Huffman coding is widely used in various compression algorithms and file formats, including JPEG and MP3.
Lempel-Ziv-Welch (LZW) Compression
The Lempel-Ziv-Welch (LZW) algorithm is a dictionary-based compression technique that builds a dictionary of substrings as it processes the data. It replaces repeated occurrences of substrings with references to their earlier occurrences in the dictionary.
The LZW algorithm follows these steps:
- Initialize a dictionary with single-character strings.
- Read input data character by character.
- Find the longest match in the dictionary.
- Output the code for the match and add the match plus the next character to the dictionary.
- Repeat steps 3-4 until all data is processed.
Here’s a basic implementation of LZW compression in Python:
def lzw_compress(data):
dictionary = {chr(i): i for i in range(256)}
next_code = 256
result = []
current_string = ""
for char in data:
current_string += char
if current_string not in dictionary:
result.append(dictionary[current_string[:-1]])
dictionary[current_string] = next_code
next_code += 1
current_string = char
if current_string:
result.append(dictionary[current_string])
return result
# Example usage
data = "TOBEORNOTTOBEORTOBEORNOT"
compressed = lzw_compress(data)
print(f"Original: {data}")
print(f"Compressed: {compressed}")
LZW compression is used in various file formats, including GIF and TIFF, and forms the basis for many modern compression algorithms.
The Science Behind Lossy Compression
Lossy compression algorithms take a different approach, sacrificing some data fidelity for higher compression ratios. Let’s explore some key techniques used in lossy compression:
Transform Coding
Transform coding is a fundamental technique in lossy compression, particularly for images and audio. It involves transforming the data from one domain (e.g., spatial or time) to another (e.g., frequency), where it can be more efficiently represented and compressed.
One of the most common transform coding techniques is the Discrete Cosine Transform (DCT), which is used in JPEG image compression. The DCT converts spatial image data into frequency coefficients, allowing the compression algorithm to prioritize more important visual information.
Here’s a simplified example of how DCT might be applied to image compression:
import numpy as np
from scipy.fftpack import dct, idct
def dct2(block):
return dct(dct(block.T, norm='ortho').T, norm='ortho')
def idct2(block):
return idct(idct(block.T, norm='ortho').T, norm='ortho')
# Example usage
block = np.random.randn(8, 8) # 8x8 block of image data
dct_block = dct2(block)
# Quantization (simulating lossy compression)
quantization_matrix = np.array([
[16, 11, 10, 16, 24, 40, 51, 61],
[12, 12, 14, 19, 26, 58, 60, 55],
[14, 13, 16, 24, 40, 57, 69, 56],
[14, 17, 22, 29, 51, 87, 80, 62],
[18, 22, 37, 56, 68, 109, 103, 77],
[24, 35, 55, 64, 81, 104, 113, 92],
[49, 64, 78, 87, 103, 121, 120, 101],
[72, 92, 95, 98, 112, 100, 103, 99]
])
quantized_dct = np.round(dct_block / quantization_matrix)
# Reconstruction
reconstructed_block = idct2(quantized_dct * quantization_matrix)
print("Original block:")
print(block)
print("\nReconstructed block:")
print(reconstructed_block)
This example demonstrates how DCT can be used to transform image data, allowing for more efficient compression through quantization of frequency coefficients.
Vector Quantization
Vector Quantization (VQ) is another lossy compression technique that works by dividing the data into vectors and mapping them to a finite set of representative vectors (a codebook). This technique is particularly useful for compressing multidimensional data, such as images or audio spectrograms.
The VQ process involves these steps:
- Divide the data into vectors.
- Create a codebook of representative vectors.
- Map each input vector to the closest representative vector in the codebook.
- Store or transmit the indices of the representative vectors instead of the original data.
Here’s a simple implementation of Vector Quantization using K-means clustering:
import numpy as np
from sklearn.cluster import KMeans
def vector_quantization(data, n_clusters):
# Reshape data into vectors
vectors = data.reshape(-1, data.shape[-1])
# Perform K-means clustering
kmeans = KMeans(n_clusters=n_clusters, random_state=42)
kmeans.fit(vectors)
# Get cluster centers (codebook)
codebook = kmeans.cluster_centers_
# Map each vector to the nearest cluster center
quantized_indices = kmeans.predict(vectors)
return codebook, quantized_indices
# Example usage
data = np.random.rand(64, 64, 3) # 64x64 RGB image
n_clusters = 16 # Number of colors in the compressed image
codebook, quantized_indices = vector_quantization(data, n_clusters)
# Reconstruct the image
reconstructed_data = codebook[quantized_indices].reshape(data.shape)
print(f"Original data shape: {data.shape}")
print(f"Codebook shape: {codebook.shape}")
print(f"Quantized indices shape: {quantized_indices.shape}")
print(f"Reconstructed data shape: {reconstructed_data.shape}")
Vector Quantization is used in various applications, including image and speech compression.
Advanced Compression Techniques
As technology advances, new compression algorithms continue to emerge, pushing the boundaries of data efficiency. Some advanced techniques include:
1. Wavelet Compression
Wavelet compression uses mathematical functions to decompose data into different frequency components. This allows for more flexible and efficient compression, especially for images and audio. The JPEG 2000 format, for example, uses wavelet compression to achieve better compression ratios and quality compared to standard JPEG.
2. Fractal Compression
Fractal compression exploits self-similarity within data, particularly images. It represents an image as a collection of transformed copies of parts of itself. While computationally intensive, fractal compression can achieve high compression ratios for certain types of images.
3. Machine Learning-based Compression
Recent advancements in machine learning have led to novel compression techniques. Neural networks can be trained to compress and decompress data, potentially outperforming traditional algorithms in specific scenarios. For example, Google’s JPEG-XL format incorporates machine learning techniques to achieve better compression ratios while maintaining image quality.
The Impact of Compression Algorithms
The science behind compression algorithms has far-reaching implications across various domains:
1. Data Storage and Transmission
Compression algorithms enable efficient storage and transmission of vast amounts of data. This is crucial for everything from cloud storage services to streaming platforms.
2. Mobile Computing
In the era of mobile devices, compression algorithms play a vital role in optimizing data usage and improving user experience, especially in areas with limited bandwidth.
3. Big Data and Analytics
Compression techniques are essential for managing and analyzing large datasets, allowing organizations to extract insights from massive volumes of data more efficiently.
4. Artificial Intelligence and Machine Learning
Compression algorithms are increasingly important in AI and ML, helping to reduce the size of large models and datasets, making them more deployable and efficient.
Conclusion
The science behind compression algorithms is a fascinating intersection of mathematics, computer science, and information theory. From simple techniques like Run-Length Encoding to complex methods involving machine learning, compression algorithms continue to evolve, driving innovations in data management and digital communication.
As we generate and consume ever-increasing amounts of data, the importance of efficient compression techniques only grows. Understanding the principles behind these algorithms not only provides insight into how our digital world operates but also opens doors to new possibilities in data processing and storage.
Whether you’re a software developer working on optimizing data transmission, a data scientist dealing with large datasets, or simply a curious individual interested in the inner workings of technology, the world of compression algorithms offers a rich field of study and application. As we look to the future, it’s clear that the science of compression will continue to play a crucial role in shaping our digital landscape, enabling new technologies and pushing the boundaries of what’s possible in data management and communication.