Exploring Neural Network Algorithms: A Comprehensive Guide for Aspiring Programmers
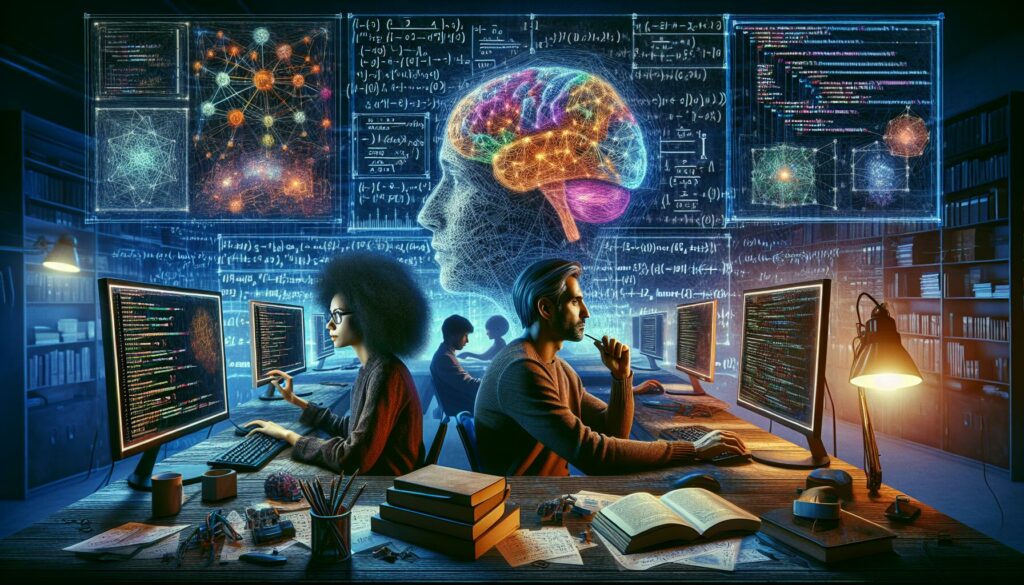
In the ever-evolving landscape of artificial intelligence and machine learning, neural networks have emerged as a powerful tool for solving complex problems and making accurate predictions. As aspiring programmers and coding enthusiasts, understanding the intricacies of neural network algorithms is crucial for staying ahead in the tech industry. In this comprehensive guide, we’ll dive deep into the world of neural networks, exploring their fundamental concepts, various types, and practical applications.
What are Neural Networks?
Neural networks, also known as artificial neural networks (ANNs), are computational models inspired by the human brain’s structure and function. They consist of interconnected nodes, or “neurons,” organized in layers that process and transmit information. The primary goal of neural networks is to learn patterns from input data and make predictions or decisions based on that learning.
The basic structure of a neural network typically includes:
- Input layer: Receives the initial data
- Hidden layer(s): Processes the information
- Output layer: Produces the final result or prediction
Key Components of Neural Networks
1. Neurons
Neurons, or nodes, are the fundamental units of a neural network. Each neuron receives input, processes it, and produces an output. The processing typically involves applying a weighted sum of inputs and an activation function.
2. Weights and Biases
Weights determine the strength of connections between neurons, while biases add a constant value to the weighted sum. These parameters are adjusted during the training process to improve the network’s performance.
3. Activation Functions
Activation functions introduce non-linearity into the network, allowing it to learn complex patterns. Common activation functions include:
- Sigmoid
- ReLU (Rectified Linear Unit)
- Tanh (Hyperbolic Tangent)
- Softmax
4. Loss Function
The loss function measures the difference between the network’s predictions and the actual target values. It quantifies the network’s performance and guides the learning process.
5. Optimization Algorithm
Optimization algorithms, such as Stochastic Gradient Descent (SGD) or Adam, are used to update the network’s weights and biases to minimize the loss function.
Types of Neural Networks
There are several types of neural networks, each designed for specific tasks and data structures. Let’s explore some of the most common types:
1. Feedforward Neural Networks (FNN)
Feedforward neural networks are the simplest type of artificial neural network. Information flows in one direction, from the input layer through hidden layers to the output layer, without any loops or cycles.
Key characteristics:
- Unidirectional flow of information
- Suitable for simple classification and regression tasks
- Easy to implement and understand
Example implementation in Python using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(64, activation='relu', input_shape=(input_dim,)),
Dense(32, activation='relu'),
Dense(output_dim, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
2. Convolutional Neural Networks (CNN)
Convolutional Neural Networks are specialized for processing grid-like data, such as images. They use convolutional layers to automatically learn spatial hierarchies of features.
Key characteristics:
- Effective for image and video processing
- Utilizes convolutional and pooling layers
- Reduces the number of parameters compared to fully connected networks
Example implementation in Python using TensorFlow:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(height, width, channels)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation='relu'),
Dense(num_classes, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
3. Recurrent Neural Networks (RNN)
Recurrent Neural Networks are designed to work with sequential data, such as time series or natural language. They have loops that allow information to persist, making them suitable for tasks that involve context and memory.
Key characteristics:
- Handles variable-length sequences
- Suitable for time series analysis and natural language processing
- Can suffer from vanishing or exploding gradients
Example implementation in Python using TensorFlow:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import SimpleRNN, Dense
model = Sequential([
SimpleRNN(64, input_shape=(sequence_length, input_dim), return_sequences=True),
SimpleRNN(32),
Dense(output_dim, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
4. Long Short-Term Memory Networks (LSTM)
LSTM networks are a type of RNN that addresses the vanishing gradient problem. They use a more complex structure with gates to control the flow of information, allowing them to capture long-term dependencies.
Key characteristics:
- Better at capturing long-term dependencies compared to simple RNNs
- Uses gates to control information flow
- Widely used in speech recognition and machine translation
Example implementation in Python using TensorFlow:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
model = Sequential([
LSTM(64, input_shape=(sequence_length, input_dim), return_sequences=True),
LSTM(32),
Dense(output_dim, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
5. Generative Adversarial Networks (GAN)
GANs consist of two neural networks, a generator and a discriminator, that compete against each other. The generator creates fake data, while the discriminator tries to distinguish between real and fake data.
Key characteristics:
- Used for generating new, synthetic data
- Consists of a generator and a discriminator network
- Applications include image generation and style transfer
Example implementation in Python using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Reshape, Conv2D, Conv2DTranspose, Flatten
def build_generator():
model = Sequential([
Dense(7*7*256, use_bias=False, input_shape=(100,)),
Reshape((7, 7, 256)),
Conv2DTranspose(128, (5, 5), strides=(1, 1), padding='same', use_bias=False),
Conv2DTranspose(64, (5, 5), strides=(2, 2), padding='same', use_bias=False),
Conv2DTranspose(1, (5, 5), strides=(2, 2), padding='same', use_bias=False, activation='tanh')
])
return model
def build_discriminator():
model = Sequential([
Conv2D(64, (5, 5), strides=(2, 2), padding='same', input_shape=[28, 28, 1]),
Conv2D(128, (5, 5), strides=(2, 2), padding='same'),
Flatten(),
Dense(1)
])
return model
generator = build_generator()
discriminator = build_discriminator()
cross_entropy = tf.keras.losses.BinaryCrossentropy(from_logits=True)
def generator_loss(fake_output):
return cross_entropy(tf.ones_like(fake_output), fake_output)
def discriminator_loss(real_output, fake_output):
real_loss = cross_entropy(tf.ones_like(real_output), real_output)
fake_loss = cross_entropy(tf.zeros_like(fake_output), fake_output)
total_loss = real_loss + fake_loss
return total_loss
generator_optimizer = tf.keras.optimizers.Adam(1e-4)
discriminator_optimizer = tf.keras.optimizers.Adam(1e-4)
Training Neural Networks
Training a neural network involves the following steps:
- Data preparation: Collect and preprocess the data, splitting it into training, validation, and test sets.
- Model architecture: Design the network structure, choosing the appropriate type and number of layers.
- Forward propagation: Pass the input data through the network to generate predictions.
- Loss calculation: Compute the difference between predictions and actual values using a loss function.
- Backpropagation: Calculate gradients of the loss with respect to the network’s parameters.
- Parameter update: Adjust weights and biases using an optimization algorithm to minimize the loss.
- Iteration: Repeat steps 3-6 for multiple epochs until the model converges or reaches satisfactory performance.
Challenges in Training Neural Networks
Training neural networks can be challenging due to several factors:
- Overfitting: When the model learns the training data too well, it may perform poorly on unseen data.
- Underfitting: When the model is too simple to capture the underlying patterns in the data.
- Vanishing/exploding gradients: Issues that can occur in deep networks, especially RNNs, where gradients become extremely small or large during backpropagation.
- Choosing hyperparameters: Selecting the right learning rate, batch size, and network architecture can be difficult and time-consuming.
Techniques to Improve Neural Network Performance
To address these challenges and improve neural network performance, consider the following techniques:
- Regularization: Use methods like L1/L2 regularization or dropout to prevent overfitting.
- Batch normalization: Normalize the inputs of each layer to reduce internal covariate shift and improve training stability.
- Transfer learning: Utilize pre-trained models and fine-tune them for specific tasks to leverage learned features and reduce training time.
- Data augmentation: Artificially increase the size of the training dataset by applying transformations to existing data.
- Ensemble methods: Combine multiple models to improve overall performance and reduce overfitting.
- Hyperparameter tuning: Use techniques like grid search, random search, or Bayesian optimization to find optimal hyperparameters.
Applications of Neural Networks
Neural networks have a wide range of applications across various industries and domains:
- Computer Vision: Image classification, object detection, facial recognition
- Natural Language Processing: Machine translation, sentiment analysis, chatbots
- Speech Recognition: Voice assistants, transcription services
- Recommendation Systems: Personalized content suggestions, product recommendations
- Financial Forecasting: Stock price prediction, risk assessment
- Healthcare: Disease diagnosis, drug discovery, medical image analysis
- Autonomous Vehicles: Self-driving cars, drones
- Gaming: AI opponents, procedural content generation
Future Trends in Neural Networks
As the field of artificial intelligence continues to evolve, several exciting trends are emerging in neural network research and development:
- Explainable AI (XAI): Developing techniques to make neural network decisions more interpretable and transparent.
- Neuromorphic computing: Creating hardware architectures that more closely mimic the structure and function of biological neural networks.
- Federated learning: Training models on decentralized data while maintaining privacy and security.
- Few-shot and zero-shot learning: Improving the ability of neural networks to learn from limited examples or adapt to new tasks without extensive retraining.
- Quantum neural networks: Exploring the potential of quantum computing to enhance neural network performance and capabilities.
- Energy-efficient neural networks: Developing more sustainable and environmentally friendly AI models with reduced computational requirements.
Conclusion
Neural networks have revolutionized the field of artificial intelligence and continue to push the boundaries of what’s possible in machine learning. As aspiring programmers and coding enthusiasts, understanding the fundamentals of neural network algorithms is crucial for staying competitive in the tech industry.
By exploring different types of neural networks, understanding their training process, and keeping up with emerging trends, you’ll be well-equipped to tackle complex problems and develop innovative solutions using these powerful tools. As you continue your journey in coding education and programming skills development, remember that practical experience and hands-on projects are key to mastering neural network algorithms and their applications.
Keep experimenting, stay curious, and don’t hesitate to dive deeper into specific areas of neural networks that interest you. With dedication and continuous learning, you’ll be well-prepared to face the challenges of technical interviews and contribute to the exciting world of artificial intelligence and machine learning.