Algorithms for Anomaly Detection: Unveiling the Unusual in Data
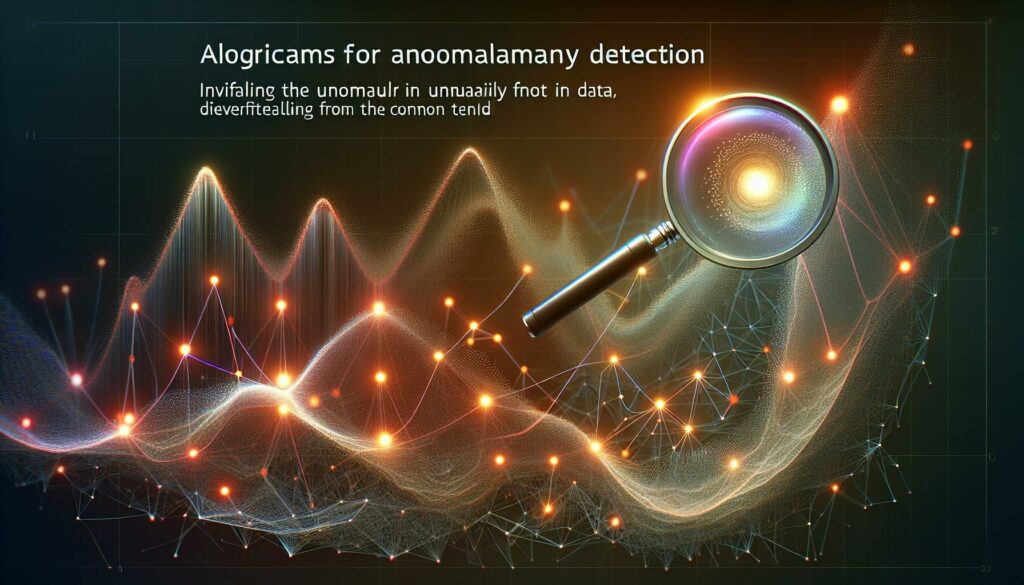
In the vast ocean of data that surrounds us, anomalies are the elusive creatures that often hold the most valuable insights. Whether it’s detecting fraud in financial transactions, identifying network intrusions, or spotting manufacturing defects, the ability to pinpoint anomalies is crucial across various domains. This is where anomaly detection algorithms come into play, serving as the sophisticated nets that catch these outliers in the data sea.
As we dive deep into the world of anomaly detection algorithms, we’ll explore their importance, the different types available, and how they’re implemented. This knowledge is not just theoretical; it’s a practical skill that can set you apart in technical interviews, especially when targeting positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
Understanding Anomaly Detection
Before we delve into specific algorithms, let’s establish what we mean by anomaly detection. In essence, anomaly detection is the process of identifying data points, events, or observations that deviate significantly from the expected pattern in a dataset. These deviations, often called outliers, anomalies, or exceptions, can indicate:
- Potential problems (e.g., a fault in a manufacturing system)
- Rare events (e.g., a security breach)
- Opportunities (e.g., a sudden spike in user engagement)
The challenge lies in distinguishing between normal variations in data and true anomalies. This is where sophisticated algorithms come into play, each with its own strengths and suitable use cases.
Types of Anomaly Detection Algorithms
Anomaly detection algorithms can be broadly categorized into three main types:
- Supervised Anomaly Detection
- Unsupervised Anomaly Detection
- Semi-Supervised Anomaly Detection
Let’s explore each of these in detail.
1. Supervised Anomaly Detection
Supervised anomaly detection algorithms require a labeled dataset where the anomalies are already identified. These algorithms learn from the labeled data to classify new, unseen data points as either normal or anomalous.
Example: Support Vector Machines (SVM) for Anomaly Detection
One popular supervised algorithm for anomaly detection is the Support Vector Machine (SVM). SVMs can be adapted for anomaly detection by using a one-class SVM, which learns a decision boundary that encompasses the normal data points.
Here’s a simple implementation of a one-class SVM for anomaly detection using Python and scikit-learn:
from sklearn import svm
import numpy as np
# Generate some sample data
X = np.random.randn(100, 2) # 100 normal points
X_outliers = np.random.uniform(low=-4, high=4, size=(20, 2)) # 20 outliers
# Fit the model
clf = svm.OneClassSVM(nu=0.1, kernel="rbf", gamma=0.1)
clf.fit(X)
# Predict
y_pred_train = clf.predict(X)
y_pred_outliers = clf.predict(X_outliers)
# Print results
n_error_train = y_pred_train[y_pred_train == -1].size
n_error_outliers = y_pred_outliers[y_pred_outliers == 1].size
print("Number of misclassified normal points: ", n_error_train)
print("Number of misclassified outlier points: ", n_error_outliers)
In this example, we create a dataset with normal points and outliers, train a one-class SVM on the normal data, and then use it to predict anomalies in both the training set and the outlier set.
2. Unsupervised Anomaly Detection
Unsupervised anomaly detection algorithms do not require labeled data. Instead, they assume that normal instances are far more frequent than anomalies in the dataset. These algorithms try to identify patterns and detect data points that don’t conform to these patterns.
Example: Isolation Forest
The Isolation Forest algorithm is a popular unsupervised method for detecting anomalies. It works on the principle that anomalies are few and different, and thus should be easier to isolate than normal points.
Here’s how you can implement an Isolation Forest in Python:
from sklearn.ensemble import IsolationForest
import numpy as np
# Generate sample data
X = np.random.randn(1000, 2) # 1000 normal points
X_outliers = np.random.uniform(low=-4, high=4, size=(100, 2)) # 100 outliers
X = np.r_[X, X_outliers]
# Fit the model
clf = IsolationForest(contamination=0.1, random_state=42)
y_pred = clf.fit_predict(X)
# Print results
n_outliers = len(y_pred[y_pred == -1])
print("Number of detected outliers: ", n_outliers)
In this example, we create a dataset with normal points and outliers, then use the Isolation Forest algorithm to detect anomalies. The algorithm returns -1 for outliers and 1 for inliers.
3. Semi-Supervised Anomaly Detection
Semi-supervised anomaly detection algorithms fall between supervised and unsupervised methods. They typically work with a training dataset that contains only normal instances. The algorithm learns to recognize normal behavior and can then identify anomalies in new data that deviate from this learned normal behavior.
Example: Autoencoder for Anomaly Detection
Autoencoders, a type of neural network, can be used for semi-supervised anomaly detection. The autoencoder is trained on normal data to reconstruct its input. When presented with an anomaly, the reconstruction error will be higher, allowing for detection.
Here’s a simple implementation using TensorFlow and Keras:
import tensorflow as tf
from tensorflow import keras
import numpy as np
# Generate sample data
normal_data = np.random.normal(size=(1000, 10))
anomaly_data = np.random.normal(loc=2, scale=2, size=(100, 10))
# Define and compile the model
model = keras.Sequential([
keras.layers.Dense(5, activation="relu", input_shape=(10,)),
keras.layers.Dense(2, activation="relu"),
keras.layers.Dense(5, activation="relu"),
keras.layers.Dense(10)
])
model.compile(optimizer="adam", loss="mse")
# Train the model on normal data
model.fit(normal_data, normal_data, epochs=50, batch_size=32, validation_split=0.1, verbose=0)
# Predict on normal and anomaly data
normal_pred = model.predict(normal_data)
anomaly_pred = model.predict(anomaly_data)
# Calculate reconstruction error
normal_errors = np.mean(np.abs(normal_pred - normal_data), axis=1)
anomaly_errors = np.mean(np.abs(anomaly_pred - anomaly_data), axis=1)
# Set a threshold (e.g., 3 standard deviations from mean of normal errors)
threshold = np.mean(normal_errors) + 3 * np.std(normal_errors)
# Detect anomalies
print("Normal data points classified as anomalies: ", np.sum(normal_errors > threshold))
print("Anomaly data points classified as anomalies: ", np.sum(anomaly_errors > threshold))
This example trains an autoencoder on normal data, then uses it to reconstruct both normal and anomalous data. The reconstruction error is used to identify anomalies.
Choosing the Right Algorithm
Selecting the appropriate anomaly detection algorithm depends on various factors:
- Data Availability: If you have labeled data with known anomalies, supervised methods might be preferable. If you only have normal data, semi-supervised methods could be ideal. For completely unlabeled data, unsupervised methods are the way to go.
- Data Dimensionality: Some algorithms perform better with high-dimensional data than others. For instance, Isolation Forests handle high-dimensional data well.
- Scalability: If you’re dealing with large datasets, you’ll need to consider the computational efficiency of the algorithm.
- Interpretability: In some cases, you might need to explain why a particular data point was flagged as an anomaly. Some algorithms provide more interpretable results than others.
- Type of Anomalies: Different algorithms are better at detecting different types of anomalies (point anomalies, contextual anomalies, or collective anomalies).
Advanced Techniques in Anomaly Detection
As we progress further into the realm of anomaly detection, it’s worth exploring some more advanced techniques that are gaining traction in the field.
1. Deep Learning for Anomaly Detection
Deep learning models, particularly deep autoencoders and generative adversarial networks (GANs), have shown promising results in anomaly detection tasks.
Variational Autoencoders (VAEs)
VAEs are a probabilistic twist on traditional autoencoders. They learn a probability distribution of the input data, which can be used to generate new samples and detect anomalies.
Here’s a simple implementation of a VAE for anomaly detection:
import tensorflow as tf
from tensorflow import keras
import numpy as np
# Define the encoder
latent_dim = 2
encoder = keras.Sequential([
keras.layers.Dense(64, activation="relu", input_shape=(10,)),
keras.layers.Dense(32, activation="relu"),
keras.layers.Dense(latent_dim + latent_dim)
])
# Define the decoder
decoder = keras.Sequential([
keras.layers.Dense(32, activation="relu", input_shape=(latent_dim,)),
keras.layers.Dense(64, activation="relu"),
keras.layers.Dense(10)
])
# Define the VAE model
class VAE(keras.Model):
def __init__(self, encoder, decoder, **kwargs):
super(VAE, self).__init__(**kwargs)
self.encoder = encoder
self.decoder = decoder
def call(self, x):
z_mean, z_log_var = tf.split(self.encoder(x), num_or_size_splits=2, axis=1)
z = self.reparameterize(z_mean, z_log_var)
return self.decoder(z)
def reparameterize(self, z_mean, z_log_var):
eps = tf.random.normal(shape=tf.shape(z_mean))
return z_mean + tf.exp(0.5 * z_log_var) * eps
vae = VAE(encoder, decoder)
# Define the loss function
def vae_loss(x, x_decoded_mean):
z_mean, z_log_var = tf.split(encoder(x), num_or_size_splits=2, axis=1)
kl_loss = -0.5 * tf.reduce_sum(1 + z_log_var - tf.square(z_mean) - tf.exp(z_log_var), axis=-1)
reconstruction_loss = tf.reduce_sum(keras.losses.mse(x, x_decoded_mean), axis=-1)
return tf.reduce_mean(reconstruction_loss + kl_loss)
# Compile and train the model
vae.compile(optimizer="adam", loss=vae_loss)
vae.fit(normal_data, normal_data, epochs=50, batch_size=32, validation_split=0.1, verbose=0)
# Use for anomaly detection
normal_reconstructed = vae.predict(normal_data)
anomaly_reconstructed = vae.predict(anomaly_data)
normal_errors = np.mean(np.abs(normal_reconstructed - normal_data), axis=1)
anomaly_errors = np.mean(np.abs(anomaly_reconstructed - anomaly_data), axis=1)
threshold = np.mean(normal_errors) + 3 * np.std(normal_errors)
print("Normal data points classified as anomalies: ", np.sum(normal_errors > threshold))
print("Anomaly data points classified as anomalies: ", np.sum(anomaly_errors > threshold))
This VAE learns to reconstruct normal data and can then be used to detect anomalies based on reconstruction error.
2. Ensemble Methods
Ensemble methods combine multiple anomaly detection algorithms to improve overall performance. The idea is that different algorithms might catch different types of anomalies, and combining their outputs can lead to more robust detection.
Example: Simple Ensemble of Isolation Forest and One-Class SVM
from sklearn.ensemble import IsolationForest
from sklearn import svm
import numpy as np
# Generate sample data
X = np.random.randn(1000, 2) # 1000 normal points
X_outliers = np.random.uniform(low=-4, high=4, size=(100, 2)) # 100 outliers
X = np.r_[X, X_outliers]
# Fit Isolation Forest
if_clf = IsolationForest(contamination=0.1, random_state=42)
if_pred = if_clf.fit_predict(X)
# Fit One-Class SVM
svm_clf = svm.OneClassSVM(nu=0.1, kernel="rbf", gamma=0.1)
svm_pred = svm_clf.fit_predict(X)
# Combine predictions (consider a point anomalous if either model flags it)
ensemble_pred = np.where((if_pred == -1) | (svm_pred == -1), -1, 1)
# Print results
n_outliers = len(ensemble_pred[ensemble_pred == -1])
print("Number of detected outliers: ", n_outliers)
This simple ensemble combines the predictions of an Isolation Forest and a One-Class SVM, considering a point anomalous if either model flags it as such.
3. Time Series Anomaly Detection
Time series data presents unique challenges for anomaly detection. Techniques like ARIMA (AutoRegressive Integrated Moving Average) and Prophet are commonly used for this purpose.
Example: Using Prophet for Time Series Anomaly Detection
from fbprophet import Prophet
from fbprophet.plot import plot_plotly
import pandas as pd
import numpy as np
# Generate sample time series data
dates = pd.date_range(start='2020-01-01', end='2021-12-31', freq='D')
y = np.sin(np.arange(len(dates)) * 2 * np.pi / 365) + np.random.normal(0, 0.1, len(dates))
df = pd.DataFrame({'ds': dates, 'y': y})
# Add some anomalies
df.loc[df.index[200:210], 'y'] += 2
df.loc[df.index[400:410], 'y'] -= 2
# Fit the model
model = Prophet()
model.fit(df)
# Make predictions
future = model.make_future_dataframe(periods=0)
forecast = model.predict(future)
# Identify anomalies
df['yhat'] = forecast['yhat']
df['error'] = df['y'] - df['yhat']
threshold = 3 * df['error'].std()
df['anomaly'] = df['error'].abs() > threshold
print("Number of detected anomalies: ", df['anomaly'].sum())
# You can visualize the results using Prophet's built-in plotting function
fig = model.plot(forecast)
fig.show()
This example uses Facebook’s Prophet library to detect anomalies in a time series dataset. It fits a model to the data and then identifies points that deviate significantly from the model’s predictions.
Challenges in Anomaly Detection
While anomaly detection is a powerful tool, it comes with its own set of challenges:
- Defining Normal Behavior: In many real-world scenarios, it’s challenging to define what constitutes “normal” behavior. Normal patterns can evolve over time, making it necessary to update models regularly.
- Handling High-Dimensional Data: As the number of features increases, the space of possible data points grows exponentially. This “curse of dimensionality” can make it harder to distinguish between normal and anomalous points.
- Balancing False Positives and False Negatives: There’s often a trade-off between catching all anomalies (which may lead to more false positives) and minimizing false alarms (which may lead to missing some true anomalies).
- Dealing with Concept Drift: In many applications, the underlying data distribution can change over time. Anomaly detection systems need to adapt to these changes to remain effective.
- Interpretability: While detecting anomalies is valuable, understanding why a particular data point was flagged as anomalous is often crucial for taking appropriate action.
Applications of Anomaly Detection
Anomaly detection finds applications across a wide range of industries and use cases:
- Cybersecurity: Detecting unusual network traffic patterns or user behaviors that could indicate a security breach.
- Finance: Identifying fraudulent transactions or unusual market behavior.
- Manufacturing: Spotting defects in products or anomalies in sensor readings that could indicate equipment failure.
- Healthcare: Detecting anomalies in medical images or patient vital signs that could indicate health issues.
- IoT and Sensor Networks: Identifying faulty sensors or unusual environmental conditions.
- Social Media: Detecting fake accounts or unusual content spreading patterns.
Best Practices for Implementing Anomaly Detection
When implementing anomaly detection systems, consider the following best practices:
- Understand Your Data: Before choosing an algorithm, thoroughly analyze your data to understand its characteristics, distribution, and potential types of anomalies.
- Feature Engineering: Carefully select and engineer features that are likely to be indicative of anomalies in your specific domain.
- Ensemble Approaches: Consider using multiple algorithms and combining their results for more robust detection.
- Continuous Monitoring and Updating: Regularly monitor the performance of your anomaly detection system and update it as needed to adapt to changing data patterns.
- Interpretability: Where possible, use methods that provide insights into why a data point was flagged as anomalous.
- Domain Expert Involvement: Involve domain experts in the process of defining what constitutes an anomaly and in interpreting results.
- Scalability Considerations: Choose algorithms and implementations that can handle your data volume and velocity, especially for real-time applications.
Conclusion
Anomaly detection is a critical component of data analysis and machine learning, with applications spanning numerous industries. From traditional statistical methods to advanced deep learning techniques, the field offers a rich array of algorithms and approaches to tackle this challenging problem.
As you prepare for technical interviews, especially with major tech companies, having a solid understanding of anomaly detection algorithms can set you apart. It demonstrates not only your coding skills but also your ability to think critically about data and solve real-world problems.
Remember, the key to mastering anomaly detection lies not just in understanding the algorithms, but in knowing how to apply them effectively to different types of data and problem domains. Continue to practice implementing these algorithms, experiment with different datasets, and stay updated with the latest advancements in the field.
By honing your skills in anomaly detection, you’re equipping yourself with a powerful tool that’s increasingly valuable in our data-driven world. Whether you’re aiming to detect fraud, improve system reliability, or uncover hidden insights in data, the ability to spot the unusual in the sea of the ordinary is a skill that will serve you well throughout your career in tech.