Implementing Dynamic Connectivity in Graphs: A Comprehensive Guide
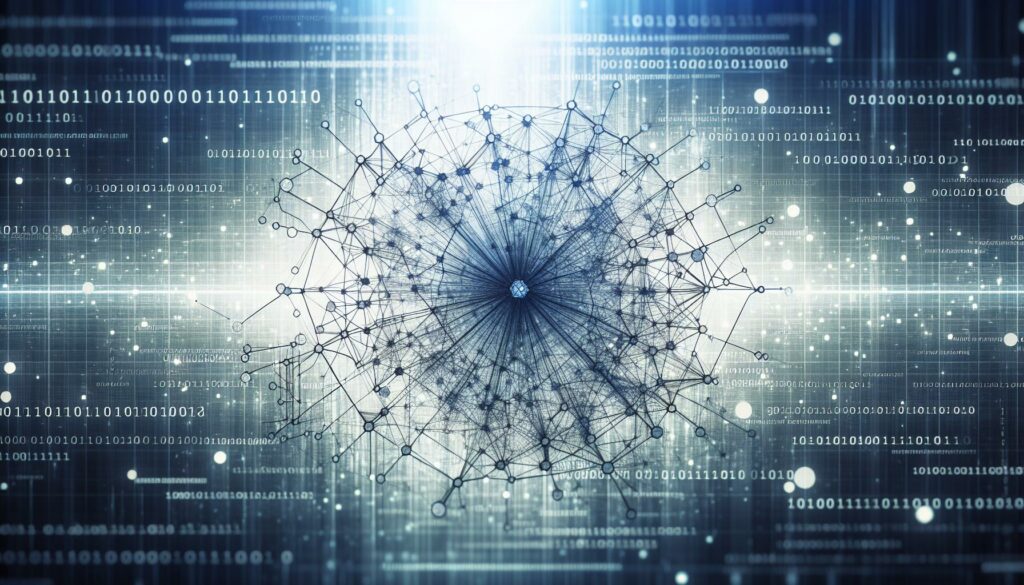
In the world of computer science and algorithmic problem-solving, graph theory plays a crucial role. One of the fundamental problems in graph theory is dynamic connectivity. This concept is essential for various applications, from social network analysis to computer networks and beyond. In this comprehensive guide, we’ll dive deep into the implementation of dynamic connectivity in graphs, exploring different approaches and their efficiency.
Table of Contents
- Introduction to Dynamic Connectivity
- Naive Approach: Depth-First Search (DFS)
- Union-Find Data Structure
- Quick Find Algorithm
- Quick Union Algorithm
- Weighted Quick Union Algorithm
- Path Compression Optimization
- Implementing Dynamic Operations
- Real-world Applications
- Advanced Techniques and Further Optimizations
- Conclusion
1. Introduction to Dynamic Connectivity
Dynamic connectivity is a problem in graph theory that deals with maintaining information about connected components in a graph as it undergoes a series of edge additions or deletions. The primary operations we need to support are:
- connect(p, q): Add an edge between nodes p and q
- isConnected(p, q): Determine if nodes p and q are in the same connected component
The challenge lies in efficiently performing these operations, especially when dealing with large graphs that change frequently. Let’s explore various approaches to solve this problem, starting from the most basic to more sophisticated algorithms.
2. Naive Approach: Depth-First Search (DFS)
The most straightforward approach to check if two nodes are connected is to perform a depth-first search (DFS) from one node and see if we can reach the other. Here’s a simple implementation in Python:
class Graph:
def __init__(self, n):
self.n = n
self.adj_list = [[] for _ in range(n)]
def connect(self, p, q):
self.adj_list[p].append(q)
self.adj_list[q].append(p)
def is_connected(self, p, q):
visited = set()
self.dfs(p, visited)
return q in visited
def dfs(self, node, visited):
visited.add(node)
for neighbor in self.adj_list[node]:
if neighbor not in visited:
self.dfs(neighbor, visited)
# Usage
graph = Graph(5)
graph.connect(0, 1)
graph.connect(1, 2)
graph.connect(3, 4)
print(graph.is_connected(0, 2)) # True
print(graph.is_connected(0, 4)) # False
While this approach works, it’s inefficient for large graphs, especially when we need to perform many connectivity queries. The time complexity for each is_connected
operation is O(V + E), where V is the number of vertices and E is the number of edges.
3. Union-Find Data Structure
To improve upon the naive approach, we can use a data structure called Union-Find (also known as Disjoint Set Union). This data structure is specifically designed to handle dynamic connectivity efficiently. The basic idea is to maintain a set of disjoint sets, where each set represents a connected component in the graph.
The Union-Find data structure supports two main operations:
- union(p, q): Merge the sets containing p and q
- find(p): Find the representative element of the set containing p
Using these operations, we can implement connect
and is_connected
as follows:
connect(p, q)
: Callunion(p, q)
is_connected(p, q)
: Check iffind(p) == find(q)
Let’s explore different implementations of the Union-Find data structure, starting with the simplest one.
4. Quick Find Algorithm
The Quick Find algorithm is the simplest implementation of Union-Find. It maintains an array where the index represents the element, and the value represents the set it belongs to.
class QuickFind:
def __init__(self, n):
self.id = list(range(n))
def find(self, p):
return self.id[p]
def union(self, p, q):
pid, qid = self.find(p), self.find(q)
if pid == qid:
return
for i in range(len(self.id)):
if self.id[i] == pid:
self.id[i] = qid
def is_connected(self, p, q):
return self.find(p) == self.find(q)
# Usage
qf = QuickFind(5)
qf.union(0, 1)
qf.union(1, 2)
qf.union(3, 4)
print(qf.is_connected(0, 2)) # True
print(qf.is_connected(0, 4)) # False
The Quick Find algorithm has a time complexity of O(1) for find
and is_connected
operations, but O(n) for union
operations. This makes it inefficient for graphs with many edge additions.
5. Quick Union Algorithm
The Quick Union algorithm improves upon Quick Find by representing each set as a tree. Each element points to its parent, and the root of the tree is the representative element of the set.
class QuickUnion:
def __init__(self, n):
self.parent = list(range(n))
def find(self, p):
while p != self.parent[p]:
p = self.parent[p]
return p
def union(self, p, q):
root_p, root_q = self.find(p), self.find(q)
if root_p != root_q:
self.parent[root_p] = root_q
def is_connected(self, p, q):
return self.find(p) == self.find(q)
# Usage
qu = QuickUnion(5)
qu.union(0, 1)
qu.union(1, 2)
qu.union(3, 4)
print(qu.is_connected(0, 2)) # True
print(qu.is_connected(0, 4)) # False
The Quick Union algorithm has a worst-case time complexity of O(n) for all operations, but it performs better than Quick Find in practice, especially for union
operations.
6. Weighted Quick Union Algorithm
To further improve the Quick Union algorithm, we can implement a weighted version. The idea is to always attach the smaller tree to the root of the larger tree during union operations. This ensures that the trees remain balanced, reducing the worst-case scenario.
class WeightedQuickUnion:
def __init__(self, n):
self.parent = list(range(n))
self.size = [1] * n
def find(self, p):
while p != self.parent[p]:
p = self.parent[p]
return p
def union(self, p, q):
root_p, root_q = self.find(p), self.find(q)
if root_p == root_q:
return
if self.size[root_p] < self.size[root_q]:
self.parent[root_p] = root_q
self.size[root_q] += self.size[root_p]
else:
self.parent[root_q] = root_p
self.size[root_p] += self.size[root_q]
def is_connected(self, p, q):
return self.find(p) == self.find(q)
# Usage
wqu = WeightedQuickUnion(5)
wqu.union(0, 1)
wqu.union(1, 2)
wqu.union(3, 4)
print(wqu.is_connected(0, 2)) # True
print(wqu.is_connected(0, 4)) # False
The Weighted Quick Union algorithm has a time complexity of O(log n) for all operations in the worst case, which is a significant improvement over the previous algorithms.
7. Path Compression Optimization
We can further optimize the Weighted Quick Union algorithm by implementing path compression. The idea is to flatten the tree structure during find operations by making every node on the path point directly to the root.
class WeightedQuickUnionWithPathCompression:
def __init__(self, n):
self.parent = list(range(n))
self.size = [1] * n
def find(self, p):
if p != self.parent[p]:
self.parent[p] = self.find(self.parent[p])
return self.parent[p]
def union(self, p, q):
root_p, root_q = self.find(p), self.find(q)
if root_p == root_q:
return
if self.size[root_p] < self.size[root_q]:
self.parent[root_p] = root_q
self.size[root_q] += self.size[root_p]
else:
self.parent[root_q] = root_p
self.size[root_p] += self.size[root_q]
def is_connected(self, p, q):
return self.find(p) == self.find(q)
# Usage
wqupc = WeightedQuickUnionWithPathCompression(5)
wqupc.union(0, 1)
wqupc.union(1, 2)
wqupc.union(3, 4)
print(wqupc.is_connected(0, 2)) # True
print(wqupc.is_connected(0, 4)) # False
This optimized version has a time complexity that is nearly constant for all practical purposes. The amortized time complexity for all operations is O(α(n)), where α(n) is the inverse Ackermann function, which grows extremely slowly and is effectively constant for all practical values of n.
8. Implementing Dynamic Operations
Now that we have an efficient Union-Find data structure, let’s implement the dynamic connectivity operations for a graph:
class DynamicConnectivityGraph:
def __init__(self, n):
self.uf = WeightedQuickUnionWithPathCompression(n)
def connect(self, p, q):
self.uf.union(p, q)
def is_connected(self, p, q):
return self.uf.is_connected(p, q)
def add_edge(self, p, q):
self.connect(p, q)
def remove_edge(self, p, q):
# Note: Edge removal is not efficiently supported in the basic Union-Find structure
# For edge removal, more advanced data structures like Euler Tour Trees are needed
raise NotImplementedError("Edge removal is not supported in this implementation")
# Usage
graph = DynamicConnectivityGraph(5)
graph.add_edge(0, 1)
graph.add_edge(1, 2)
graph.add_edge(3, 4)
print(graph.is_connected(0, 2)) # True
print(graph.is_connected(0, 4)) # False
This implementation efficiently supports edge addition and connectivity queries. However, it’s important to note that the basic Union-Find structure does not support efficient edge removal. For applications requiring edge removal, more advanced data structures like Euler Tour Trees or Link-Cut Trees are needed.
9. Real-world Applications
Dynamic connectivity in graphs has numerous real-world applications:
- Social Networks: Tracking friend connections and suggesting mutual friends.
- Computer Networks: Monitoring network connectivity and detecting network partitions.
- Image Processing: Connected component labeling in image segmentation.
- Percolation Theory: Studying the behavior of connected clusters in a random graph.
- Automated Reasoning Systems: Maintaining equivalence classes of expressions.
- Least Common Ancestor (LCA) in Trees: Solving LCA queries using the Union-Find data structure.
10. Advanced Techniques and Further Optimizations
While the Weighted Quick Union with Path Compression algorithm is highly efficient for most practical purposes, there are advanced techniques and data structures for even more specialized scenarios:
- Randomized Algorithms: Randomized linking can provide expected logarithmic time complexity without the need for maintaining size information.
- Dynamic Trees: Data structures like Link-Cut Trees or Euler Tour Trees can support both edge insertion and deletion in logarithmic time.
- Incremental Connectivity: For scenarios where edges are only added (not removed), there are algorithms that can achieve optimal amortized time complexity.
- Parallel and Distributed Algorithms: For extremely large graphs or distributed systems, parallel Union-Find algorithms can be employed.
Here’s an example of a randomized linking algorithm:
import random
class RandomizedQuickUnion:
def __init__(self, n):
self.parent = list(range(n))
def find(self, p):
if p != self.parent[p]:
self.parent[p] = self.find(self.parent[p])
return self.parent[p]
def union(self, p, q):
root_p, root_q = self.find(p), self.find(q)
if root_p != root_q:
if random.random() < 0.5:
self.parent[root_p] = root_q
else:
self.parent[root_q] = root_p
def is_connected(self, p, q):
return self.find(p) == self.find(q)
# Usage
rqu = RandomizedQuickUnion(5)
rqu.union(0, 1)
rqu.union(1, 2)
rqu.union(3, 4)
print(rqu.is_connected(0, 2)) # True
print(rqu.is_connected(0, 4)) # False
This randomized approach achieves expected logarithmic time complexity without the need for maintaining size information, which can be beneficial in certain scenarios.
11. Conclusion
Implementing dynamic connectivity in graphs is a fundamental problem with wide-ranging applications. We’ve explored various approaches, from the naive DFS method to highly optimized Union-Find data structures. The Weighted Quick Union with Path Compression algorithm provides an excellent balance of simplicity and efficiency for most practical applications.
Key takeaways from this comprehensive guide:
- Dynamic connectivity deals with maintaining connected components in a changing graph.
- The Union-Find data structure is central to efficient dynamic connectivity implementations.
- Optimizations like weighting and path compression significantly improve performance.
- The most efficient implementation (Weighted Quick Union with Path Compression) achieves near-constant time complexity for practical purposes.
- Advanced techniques exist for specialized scenarios, including randomized algorithms and dynamic trees.
As you work on graph-related problems or design systems that require dynamic connectivity, consider the trade-offs between implementation complexity and performance requirements. For most applications, the Weighted Quick Union with Path Compression algorithm will provide excellent performance. However, always be aware of the specific needs of your project, as more advanced techniques may be necessary for certain specialized scenarios.
By mastering these concepts and implementations, you’ll be well-equipped to handle a wide range of graph-related problems and contribute to the development of efficient, scalable systems in various domains of computer science and software engineering.