An Overview of Substring Search Algorithms
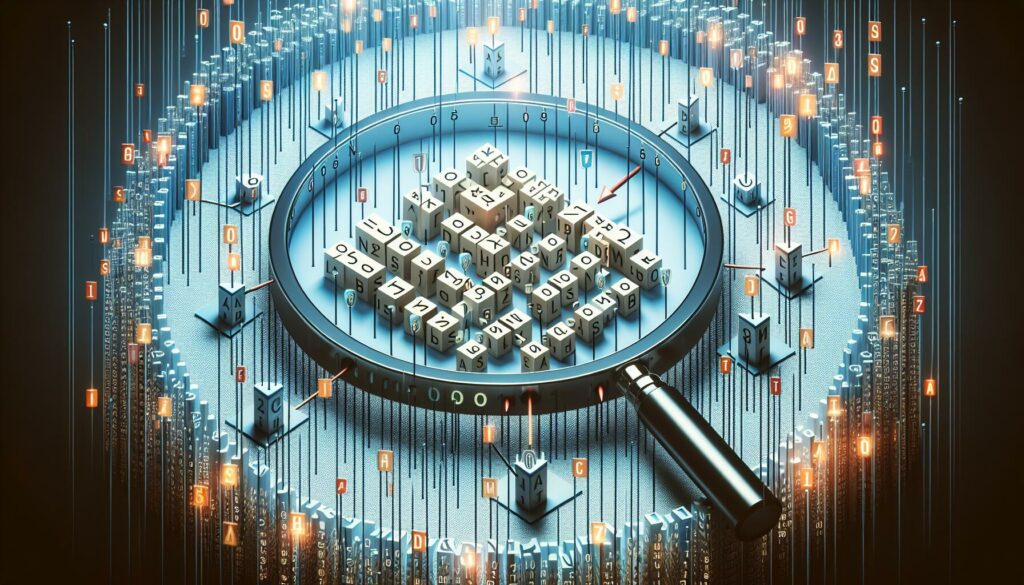
In the world of computer science and programming, efficient string manipulation and searching are crucial skills. Whether you’re developing a text editor, implementing a search function, or working on complex data processing tasks, understanding substring search algorithms is essential. This comprehensive guide will explore various substring search algorithms, their implementations, and their applications in real-world scenarios.
What is Substring Search?
Before diving into the algorithms, let’s clarify what we mean by substring search. A substring is a contiguous sequence of characters within a larger string. For example, in the string “Hello, World!”, “Hello” and “World” are both substrings. Substring search refers to the process of finding occurrences of a particular substring (often called the pattern) within a larger text (often called the text or haystack).
Substring search algorithms are designed to efficiently locate these occurrences, which is a fundamental operation in many applications, including:
- Text editors and word processors
- Search engines
- DNA sequence analysis
- Plagiarism detection
- Data compression
- Network security (e.g., intrusion detection systems)
Naive String Matching Algorithm
Let’s start with the simplest approach to substring searching: the naive or brute-force algorithm. This method involves checking every possible position in the text where the pattern could match.
How it works:
- Align the pattern with the beginning of the text.
- Compare characters one by one.
- If all characters match, we’ve found an occurrence.
- If a mismatch is found or we reach the end of the pattern, shift the pattern one position to the right and repeat from step 2.
Implementation in Python:
def naive_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
print(f"Pattern found at index {i}")
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
naive_search(text, pattern)
While simple to implement, the naive algorithm has a time complexity of O(n*m) in the worst case, where n is the length of the text and m is the length of the pattern. This can be inefficient for large texts or patterns.
Knuth-Morris-Pratt (KMP) Algorithm
The Knuth-Morris-Pratt (KMP) algorithm improves upon the naive approach by utilizing information about the pattern itself to avoid unnecessary comparisons. It preprocesses the pattern to create a “failure function” or “partial match table” that helps skip characters intelligently when a mismatch occurs.
How it works:
- Preprocess the pattern to create the failure function.
- Use the failure function to determine how much to shift the pattern when a mismatch occurs.
- Continue the search process, using the preprocessed information to avoid redundant comparisons.
Implementation in Python:
def kmp_search(text, pattern):
n = len(text)
m = len(pattern)
# Compute the failure function
failure = [0] * m
j = 0
for i in range(1, m):
while j > 0 and pattern[i] != pattern[j]:
j = failure[j - 1]
if pattern[i] == pattern[j]:
j += 1
failure[i] = j
# Perform the search
j = 0
for i in range(n):
while j > 0 and text[i] != pattern[j]:
j = failure[j - 1]
if text[i] == pattern[j]:
j += 1
if j == m:
print(f"Pattern found at index {i - m + 1}")
j = failure[j - 1]
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
kmp_search(text, pattern)
The KMP algorithm has a time complexity of O(n + m), which is a significant improvement over the naive approach, especially for large texts and patterns.
Boyer-Moore Algorithm
The Boyer-Moore algorithm is often considered one of the most efficient string matching algorithms in practice. It uses two main heuristics: the bad character heuristic and the good suffix heuristic. These heuristics allow the algorithm to skip large portions of the text, making it particularly effective for long patterns.
How it works:
- Preprocess the pattern to create bad character and good suffix tables.
- Start matching from the rightmost character of the pattern.
- If a mismatch occurs, use the preprocessed information to shift the pattern as far as possible.
- Continue the search process, utilizing both heuristics for efficient skipping.
Implementation in Python:
def boyer_moore_search(text, pattern):
n = len(text)
m = len(pattern)
# Preprocessing
bad_char = {}
for i in range(m):
bad_char[pattern[i]] = i
# Searching
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
print(f"Pattern found at index {i}")
i += (m - bad_char.get(text[i + m], -1) if i + m < n else 1)
else:
i += max(1, j - bad_char.get(text[i + j], -1))
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
boyer_moore_search(text, pattern)
The Boyer-Moore algorithm has a best-case time complexity of O(n/m) and a worst-case time complexity of O(n*m). However, in practice, it often outperforms other algorithms, especially for larger alphabets and longer patterns.
Rabin-Karp Algorithm
The Rabin-Karp algorithm takes a different approach by using hashing. It calculates hash values for the pattern and substrings of the text, then compares these hash values instead of comparing the strings character by character.
How it works:
- Calculate the hash value of the pattern.
- Calculate the hash value of the first m characters of the text, where m is the length of the pattern.
- Compare the hash values. If they match, perform a character-by-character comparison to confirm.
- Slide the window by one character, update the hash value for the new window, and repeat steps 3-4.
Implementation in Python:
def rabin_karp_search(text, pattern):
n = len(text)
m = len(pattern)
d = 256 # Number of characters in the input alphabet
q = 101 # A prime number
# Calculate hash value for pattern and first window of text
p = 0
t = 0
h = 1
for i in range(m - 1):
h = (h * d) % q
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
# Slide the pattern over text one by one
for i in range(n - m + 1):
if p == t:
if text[i:i+m] == pattern:
print(f"Pattern found at index {i}")
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i + m])) % q
if t < 0:
t += q
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
rabin_karp_search(text, pattern)
The Rabin-Karp algorithm has an average and best-case time complexity of O(n+m), but its worst-case time complexity is O(n*m). It’s particularly useful when searching for multiple patterns simultaneously.
Aho-Corasick Algorithm
While the previous algorithms focus on finding a single pattern, the Aho-Corasick algorithm is designed for efficiently searching for multiple patterns simultaneously. It’s particularly useful in applications like virus scanning, intrusion detection systems, and DNA sequence analysis.
How it works:
- Construct a trie (prefix tree) from the set of patterns.
- Add failure links to the trie, similar to the KMP algorithm’s failure function.
- Add output links to nodes where patterns end.
- Traverse the text, following trie transitions and failure links as needed.
- Report matches when reaching nodes with output links.
Implementation in Python:
from collections import deque
class AhoCorasick:
def __init__(self, patterns):
self.goto = {}
self.output = {}
self.failure = {}
self.build_automaton(patterns)
def build_automaton(self, patterns):
# Construct trie
for i, pattern in enumerate(patterns):
current = 0
for char in pattern:
current = self.goto.setdefault((current, char), len(self.goto))
self.output.setdefault(current, []).append(i)
# Add failure and output links
queue = deque(node for node in self.goto.values() if node != 0)
while queue:
current = queue.popleft()
for char in set(char for _, char in self.goto):
if (current, char) in self.goto:
child = self.goto[(current, char)]
failure = self.failure.get(current, 0)
while failure and (failure, char) not in self.goto:
failure = self.failure.get(failure, 0)
self.failure[child] = self.goto.get((failure, char), 0)
self.output.setdefault(child, []).extend(self.output.get(self.failure[child], []))
queue.append(child)
def search(self, text):
current = 0
for i, char in enumerate(text):
while current and (current, char) not in self.goto:
current = self.failure.get(current, 0)
current = self.goto.get((current, char), 0)
for pattern_index in self.output.get(current, []):
print(f"Pattern {pattern_index} found at index {i - len(patterns[pattern_index]) + 1}")
# Example usage
patterns = ["ABABC", "BABC", "ABCAB"]
text = "ABABDABACDABABCABAB"
ac = AhoCorasick(patterns)
ac.search(text)
The Aho-Corasick algorithm has a time complexity of O(n + m + z), where n is the length of the text, m is the total length of all patterns, and z is the number of output matches. It’s highly efficient for multiple pattern matching scenarios.
Comparing Substring Search Algorithms
Each substring search algorithm has its strengths and weaknesses, making them suitable for different scenarios:
- Naive Algorithm: Simple to implement but inefficient for large texts or patterns. Useful for educational purposes or very small-scale applications.
- KMP Algorithm: Efficient for single pattern matching, especially when the pattern has many repeating substrings. Good for general-purpose string searching.
- Boyer-Moore Algorithm: Often the fastest in practice, especially for longer patterns and larger alphabets. Ideal for applications like text editors where the pattern is typically short compared to the text.
- Rabin-Karp Algorithm: Efficient for multiple pattern searching and in scenarios where hash comparisons are faster than character comparisons. Useful in plagiarism detection systems.
- Aho-Corasick Algorithm: Excellent for multiple pattern matching scenarios. Ideal for applications like virus scanning, intrusion detection, and DNA sequence analysis.
Real-world Applications
Understanding these substring search algorithms is crucial for many real-world applications:
- Text Editors and Word Processors: Efficient search and replace functionality often relies on algorithms like Boyer-Moore.
- Bioinformatics: DNA sequence analysis frequently uses Aho-Corasick for matching multiple patterns (e.g., gene sequences) in large genomic databases.
- Search Engines: While modern search engines use more complex algorithms, basic substring searching is still a fundamental component.
- Plagiarism Detection: Systems may use Rabin-Karp to efficiently compare documents against a large database of known texts.
- Network Security: Intrusion Detection Systems (IDS) often employ Aho-Corasick to scan network traffic for multiple suspicious patterns simultaneously.
- Data Compression: Some compression algorithms use substring searching as part of their process to identify repeating patterns.
- Spell Checkers: These tools may use various string matching algorithms to suggest corrections for misspelled words.
Conclusion
Substring search algorithms are a fundamental part of computer science and have wide-ranging applications in software development. By understanding these algorithms, you’ll be better equipped to choose the right tool for your specific use case, whether you’re developing a simple text editor or working on complex bioinformatics problems.
As you continue your journey in coding education and programming skills development, remember that mastering these algorithms is not just about memorizing implementations. It’s about understanding the underlying principles, the trade-offs between different approaches, and how to apply them in real-world scenarios.
Practice implementing these algorithms, analyze their performance with different inputs, and consider how you might optimize them for specific use cases. This hands-on experience will deepen your understanding and prepare you for technical interviews at major tech companies, where string manipulation and efficient algorithms are often key topics.
Remember, the field of string algorithms is vast and constantly evolving. While these classic algorithms form a strong foundation, stay curious and keep exploring new developments in this exciting area of computer science!