The Role of Algorithms in Autonomous Vehicles: Driving the Future of Transportation
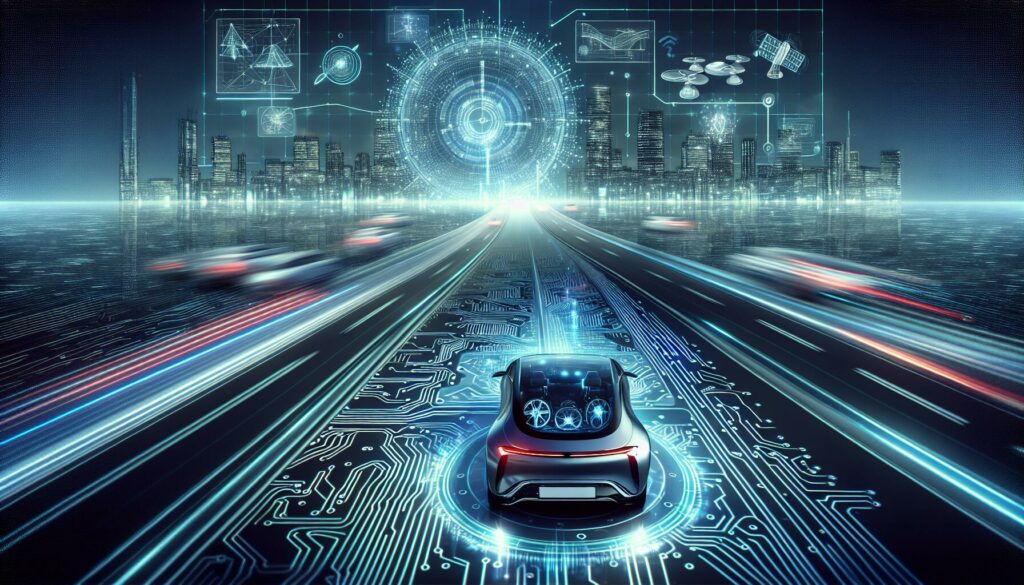
In recent years, the automotive industry has been undergoing a revolutionary transformation with the advent of autonomous vehicles. These self-driving cars are not just a futuristic concept anymore; they are becoming a reality on our roads. At the heart of this technological marvel lies a complex network of algorithms that work tirelessly to ensure safe, efficient, and intelligent navigation. In this comprehensive guide, we’ll explore the crucial role that algorithms play in autonomous vehicles and how they are shaping the future of transportation.
Understanding Autonomous Vehicles
Before diving into the algorithms that power self-driving cars, it’s essential to understand what autonomous vehicles are and how they operate. Autonomous vehicles, also known as self-driving cars or driverless cars, are vehicles capable of sensing their environment and navigating without human input. They use a variety of sensors, actuators, and complex algorithms to perceive their surroundings and make decisions about where to go and how to get there safely.
The Society of Automotive Engineers (SAE) has defined six levels of driving automation, ranging from Level 0 (no automation) to Level 5 (full automation). Most current autonomous vehicle projects aim for Level 4 or 5 automation, where the vehicle can handle all driving tasks under specific conditions or in all conditions, respectively.
The Algorithmic Foundation of Autonomous Vehicles
Algorithms are the backbone of autonomous vehicle technology. They process vast amounts of data from various sensors and make split-second decisions to navigate the vehicle safely. Let’s explore some of the key algorithmic components that make self-driving cars possible:
1. Perception Algorithms
Perception algorithms are responsible for interpreting the data collected by the vehicle’s sensors, such as cameras, LiDAR (Light Detection and Ranging), radar, and ultrasonic sensors. These algorithms must accurately identify and classify objects in the vehicle’s environment, including:
- Other vehicles
- Pedestrians
- Cyclists
- Road signs and traffic signals
- Lane markings
- Obstacles and road hazards
One of the most common algorithms used for object detection and classification is the Convolutional Neural Network (CNN). CNNs are particularly effective at processing image data and have been widely adopted in computer vision tasks.
Here’s a simplified example of how a CNN might be implemented for object detection in Python using the popular deep learning library TensorFlow:
import tensorflow as tf
from tensorflow.keras import layers, models
def create_cnn_model(input_shape, num_classes):
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=input_shape),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(num_classes, activation='softmax')
])
return model
# Example usage
input_shape = (224, 224, 3) # Assuming RGB images of size 224x224
num_classes = 10 # Number of object classes to detect
model = create_cnn_model(input_shape, num_classes)
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train the model with your dataset
# model.fit(...)
This CNN model can be trained on a large dataset of labeled images to recognize different objects that an autonomous vehicle might encounter on the road.
2. Localization and Mapping Algorithms
For an autonomous vehicle to navigate effectively, it needs to know its precise location and have an accurate map of its surroundings. This is achieved through a combination of GPS data, sensor information, and advanced algorithms such as Simultaneous Localization and Mapping (SLAM).
SLAM algorithms allow the vehicle to build a map of an unknown environment while simultaneously keeping track of its location within that environment. One popular implementation of SLAM is the Extended Kalman Filter (EKF) SLAM algorithm.
Here’s a basic outline of how an EKF SLAM algorithm might be implemented:
import numpy as np
class EKF_SLAM:
def __init__(self, initial_state, initial_covariance):
self.state = initial_state
self.covariance = initial_covariance
def predict(self, control_input, noise_covariance):
# Predict the new state based on control input
self.state = self.motion_model(self.state, control_input)
# Update the covariance
jacobian = self.motion_model_jacobian(self.state, control_input)
self.covariance = jacobian @ self.covariance @ jacobian.T + noise_covariance
def update(self, measurement, measurement_covariance):
# Calculate the expected measurement
expected_measurement = self.measurement_model(self.state)
# Calculate the innovation
innovation = measurement - expected_measurement
# Calculate the Kalman gain
jacobian = self.measurement_model_jacobian(self.state)
S = jacobian @ self.covariance @ jacobian.T + measurement_covariance
K = self.covariance @ jacobian.T @ np.linalg.inv(S)
# Update the state and covariance
self.state = self.state + K @ innovation
self.covariance = (np.eye(len(self.state)) - K @ jacobian) @ self.covariance
def motion_model(self, state, control_input):
# Implement the motion model
pass
def motion_model_jacobian(self, state, control_input):
# Implement the Jacobian of the motion model
pass
def measurement_model(self, state):
# Implement the measurement model
pass
def measurement_model_jacobian(self, state):
# Implement the Jacobian of the measurement model
pass
This EKF_SLAM class provides a framework for implementing the Extended Kalman Filter SLAM algorithm. The specific implementation details of the motion and measurement models would depend on the sensors and dynamics of the particular autonomous vehicle system.
3. Path Planning Algorithms
Once the vehicle has a clear understanding of its environment and location, it needs to plan a safe and efficient path to its destination. Path planning algorithms are responsible for generating a trajectory that avoids obstacles, obeys traffic rules, and optimizes for factors such as time, fuel efficiency, and passenger comfort.
Some common path planning algorithms used in autonomous vehicles include:
- A* Algorithm
- Rapidly-exploring Random Trees (RRT)
- Model Predictive Control (MPC)
Let’s take a closer look at the A* algorithm, which is widely used for finding the optimal path in a graph or grid-based environment:
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(graph, start, goal):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
current = heapq.heappop(frontier)[1]
if current == goal:
break
for next in graph.neighbors(current):
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
return came_from, cost_so_far
class Graph:
def __init__(self):
self.edges = {}
def neighbors(self, id):
return self.edges[id]
def cost(self, from_node, to_node):
return 1 # Assuming uniform cost
# Example usage
graph = Graph()
graph.edges = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
start = 'A'
goal = 'F'
came_from, cost_so_far = a_star(graph, start, goal)
# Reconstruct path
path = []
current = goal
while current != start:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
print(f"Optimal path from {start} to {goal}: {' -> '.join(path)}")
print(f"Total cost: {cost_so_far[goal]}")
This implementation of the A* algorithm can be adapted for use in autonomous vehicles by incorporating more complex cost functions that take into account factors such as road conditions, traffic, and vehicle dynamics.
4. Decision-Making Algorithms
Autonomous vehicles must make countless decisions in real-time, such as when to change lanes, how to respond to unexpected obstacles, or how to handle complex traffic scenarios. Decision-making algorithms use the information from perception, localization, and path planning to determine the best course of action at any given moment.
These algorithms often employ techniques from artificial intelligence and machine learning, such as:
- Reinforcement Learning
- Behavior Trees
- Finite State Machines
Reinforcement Learning (RL) is particularly promising for autonomous vehicle decision-making because it allows the system to learn optimal behaviors through trial and error in simulated environments. Here’s a simple example of how a Q-learning algorithm (a type of RL) might be implemented for a basic driving scenario:
import numpy as np
import random
class QLearning:
def __init__(self, states, actions, learning_rate=0.1, discount_factor=0.9, epsilon=0.1):
self.q_table = np.zeros((states, actions))
self.lr = learning_rate
self.gamma = discount_factor
self.epsilon = epsilon
def choose_action(self, state):
if random.uniform(0, 1) < self.epsilon:
return random.randint(0, self.q_table.shape[1] - 1) # Explore
else:
return np.argmax(self.q_table[state]) # Exploit
def learn(self, state, action, reward, next_state):
predict = self.q_table[state, action]
target = reward + self.gamma * np.max(self.q_table[next_state])
self.q_table[state, action] += self.lr * (target - predict)
# Example usage
num_states = 10 # Number of discretized states
num_actions = 3 # e.g., 0: maintain speed, 1: accelerate, 2: brake
agent = QLearning(num_states, num_actions)
# Training loop (simplified)
num_episodes = 1000
for episode in range(num_episodes):
state = 0 # Initial state
done = False
while not done:
action = agent.choose_action(state)
# Simulate the environment (simplified)
next_state = min(state + action - 1, num_states - 1) # Simplified state transition
reward = -1 if next_state == state else 0 # Penalize staying in the same state
done = (next_state == num_states - 1) # Goal state reached
agent.learn(state, action, reward, next_state)
state = next_state
print("Q-table after training:")
print(agent.q_table)
This simplified Q-learning example demonstrates how an autonomous vehicle could learn to make decisions about speed control. In a real-world application, the state space would be much larger and more complex, incorporating various sensor inputs and traffic conditions.
Challenges and Future Developments
While algorithms have made tremendous strides in enabling autonomous vehicles, several challenges remain:
1. Handling Edge Cases
Autonomous vehicles must be prepared to handle rare and unexpected situations, known as edge cases. These could include unusual road conditions, extreme weather, or complex traffic scenarios that weren’t encountered during training. Developing algorithms that can generalize well to these edge cases is an ongoing challenge.
2. Ethical Decision-Making
Autonomous vehicles may encounter situations where they need to make ethical decisions, such as choosing between two potentially harmful outcomes. Programming these ethical considerations into algorithms is a complex and controversial topic that requires careful consideration.
3. Cybersecurity
As autonomous vehicles become more connected and reliant on software, ensuring their security against cyber attacks becomes crucial. Developing robust security algorithms to protect against hacking and unauthorized access is a critical area of research.
4. Interpretability and Explainability
Many of the advanced algorithms used in autonomous vehicles, particularly deep learning models, are often seen as “black boxes.” Improving the interpretability and explainability of these algorithms is important for building trust in autonomous vehicle technology and for debugging and improving the systems.
The Future of Algorithms in Autonomous Vehicles
As technology continues to advance, we can expect to see further developments in the algorithms powering autonomous vehicles:
1. Advanced AI and Machine Learning
Future algorithms will likely incorporate more sophisticated AI and machine learning techniques, such as meta-learning and transfer learning, allowing autonomous vehicles to adapt more quickly to new environments and situations.
2. Improved Sensor Fusion
Algorithms that can more effectively combine data from multiple sensors (sensor fusion) will lead to more accurate and robust perception systems.
3. Collaborative Learning
As more autonomous vehicles hit the roads, there’s potential for collaborative learning algorithms that allow vehicles to share knowledge and experiences, leading to faster improvements across entire fleets.
4. Human-AI Collaboration
Future algorithms may focus on better collaboration between human drivers and AI systems, creating smoother transitions between different levels of autonomy.
Conclusion
Algorithms play a crucial role in the development and operation of autonomous vehicles, forming the intelligence that allows these vehicles to perceive their environment, make decisions, and navigate safely. From perception and localization to path planning and decision-making, each component relies on sophisticated algorithms to process vast amounts of data and make split-second decisions.
As we continue to refine and develop these algorithms, we move closer to a future where autonomous vehicles are a common sight on our roads, promising increased safety, efficiency, and accessibility in transportation. However, challenges remain, particularly in areas such as handling edge cases, ethical decision-making, and cybersecurity.
For aspiring programmers and AI enthusiasts, the field of autonomous vehicles offers exciting opportunities to work on cutting-edge algorithms that have the potential to revolutionize transportation. Whether you’re interested in computer vision, machine learning, or robotics, there’s a place for your skills in the autonomous vehicle industry.
As we look to the future, it’s clear that the role of algorithms in autonomous vehicles will only grow in importance. By staying informed about these developments and continually honing your programming and problem-solving skills, you’ll be well-positioned to contribute to this exciting field and shape the future of transportation.