An Introduction to Approximate String Matching: Techniques and Applications
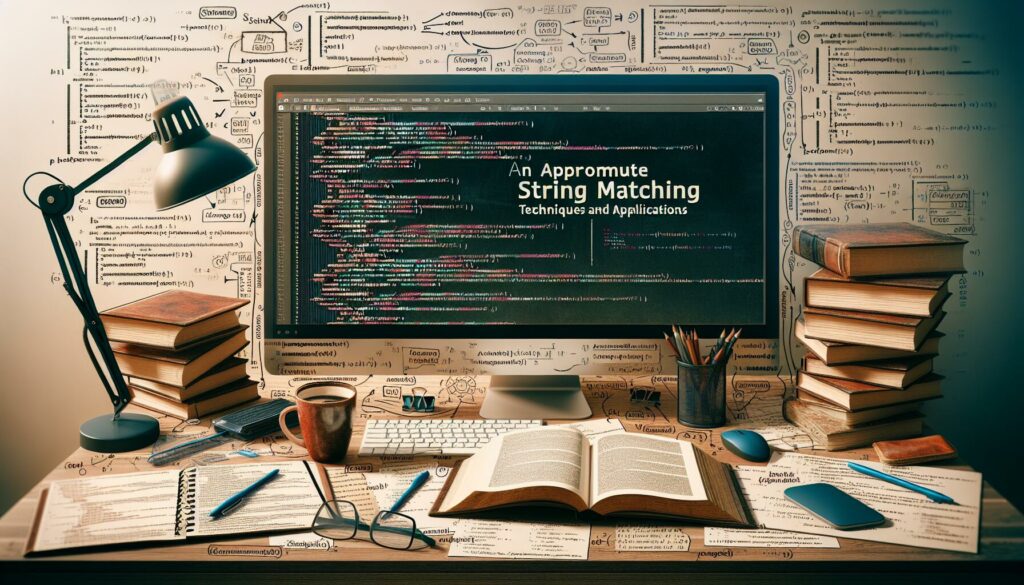
In the vast realm of computer science and data processing, the ability to compare and match strings is a fundamental operation. However, real-world data is often messy, inconsistent, or prone to errors. This is where approximate string matching comes into play, offering a powerful set of techniques to find similarities between strings even when they’re not exactly the same. In this comprehensive guide, we’ll dive deep into the world of approximate string matching, exploring its concepts, algorithms, and practical applications.
What is Approximate String Matching?
Approximate string matching, also known as fuzzy string searching, is the technique of finding strings that match a pattern approximately (rather than exactly). This means identifying strings that are similar but not necessarily identical to a given pattern, allowing for some degree of variation or error.
Unlike exact string matching, which requires a perfect match between the pattern and the text, approximate string matching allows for:
- Character insertions
- Character deletions
- Character substitutions
- Character transpositions (in some cases)
The goal is to find matches that are “close enough” to the pattern, based on a predefined similarity threshold or distance metric.
Why is Approximate String Matching Important?
Approximate string matching has numerous applications across various domains:
- Spell checking and correction: Identifying and suggesting corrections for misspelled words.
- DNA sequence analysis: Finding similar genetic sequences in bioinformatics.
- Information retrieval: Improving search results by matching similar terms.
- Data cleansing: Identifying and merging duplicate records in databases.
- Plagiarism detection: Finding similar text passages in documents.
- Optical Character Recognition (OCR): Improving accuracy in text recognition from images.
Key Concepts in Approximate String Matching
1. Edit Distance
Edit distance is a fundamental concept in approximate string matching. It quantifies the similarity between two strings by counting the minimum number of operations required to transform one string into another. The most common types of edit distance are:
- Levenshtein distance: Allows insertions, deletions, and substitutions.
- Hamming distance: Only allows substitutions (applicable to strings of equal length).
- Damerau-Levenshtein distance: Extends Levenshtein distance to include transpositions.
2. Similarity Metrics
While edit distance measures the difference between strings, similarity metrics provide a normalized measure of how alike two strings are. Common similarity metrics include:
- Jaccard similarity: Measures the overlap between character sets of two strings.
- Cosine similarity: Treats strings as vectors and measures the cosine of the angle between them.
- Jaro-Winkler similarity: Designed for short strings like names, giving more weight to matching prefixes.
3. N-grams
N-grams are contiguous sequences of n items (characters or words) from a given string. They are often used in approximate string matching to break down strings into smaller, comparable units. For example, the trigrams (3-grams) of “hello” would be “hel”, “ell”, and “llo”.
Algorithms for Approximate String Matching
Let’s explore some of the most popular algorithms used in approximate string matching:
1. Dynamic Programming for Edit Distance
The most straightforward approach to calculate edit distance (specifically, Levenshtein distance) is using dynamic programming. This algorithm builds a matrix to compute the minimum number of edits required to transform one string into another.
Here’s a Python implementation of the Levenshtein distance algorithm:
def levenshtein_distance(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(m + 1):
dp[i][0] = i
for j in range(n + 1):
dp[0][j] = j
for i in range(1, m + 1):
for j in range(1, n + 1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = 1 + min(dp[i-1][j], # deletion
dp[i][j-1], # insertion
dp[i-1][j-1]) # substitution
return dp[m][n]
# Example usage
print(levenshtein_distance("kitten", "sitting")) # Output: 3
This algorithm has a time complexity of O(mn) and a space complexity of O(mn), where m and n are the lengths of the input strings.
2. Rabin-Karp Algorithm with Fuzzy Matching
The Rabin-Karp algorithm, typically used for exact string matching, can be adapted for approximate string matching by using a rolling hash function and allowing for a certain number of mismatches.
Here’s a simplified Python implementation that demonstrates the concept:
def rabin_karp_fuzzy(text, pattern, k):
n, m = len(text), len(pattern)
matches = []
pattern_hash = sum(ord(c) for c in pattern)
text_hash = sum(ord(text[i]) for i in range(m))
for i in range(n - m + 1):
if abs(text_hash - pattern_hash) <= k * 255: # Allow k mismatches
mismatches = sum(a != b for a, b in zip(text[i:i+m], pattern))
if mismatches <= k:
matches.append(i)
if i < n - m:
text_hash = text_hash - ord(text[i]) + ord(text[i+m])
return matches
# Example usage
text = "The quick brown fox jumps over the lazy dog"
pattern = "brwn"
k = 1 # Allow 1 mismatch
print(rabin_karp_fuzzy(text, pattern, k)) # Output: [10]
This implementation has a time complexity of O(n) on average, but can degrade to O(nm) in the worst case.
3. Bitap Algorithm (Shift-Or Algorithm)
The Bitap algorithm, also known as the Shift-Or algorithm, uses bitwise operations to perform fast approximate string matching. It’s particularly efficient for short patterns and small alphabets.
Here’s a Python implementation of the Bitap algorithm for fuzzy matching:
def bitap_fuzzy(text, pattern, k):
m = len(pattern)
alphabet = set(text + pattern)
mask = {c: ~(1 << i) for i, c in enumerate(pattern)}
R = [~0] * (k + 1)
matches = []
for i, c in enumerate(text):
for d in range(k, 0, -1):
R[d] = ((R[d] << 1) | 1) & (R[d-1] << 1) & mask.get(c, ~0)
R[0] = ((R[0] << 1) | 1) & mask.get(c, ~0)
if R[k] & (1 << (m - 1)) == 0:
matches.append(i - m + 1)
return matches
# Example usage
text = "The quick brown fox jumps over the lazy dog"
pattern = "brwn"
k = 1 # Allow 1 error
print(bitap_fuzzy(text, pattern, k)) # Output: [10]
The Bitap algorithm has a time complexity of O(kn) and is very efficient for small patterns and error thresholds.
Practical Applications and Implementations
Now that we’ve covered the theoretical aspects and some basic implementations, let’s explore how approximate string matching is used in real-world applications and discuss some popular libraries and tools.
1. Spell Checking and Autocorrection
Spell checkers use approximate string matching to suggest corrections for misspelled words. They typically combine edit distance calculations with a dictionary of known words to find the most likely correct spelling.
Here’s a simple example using the `difflib` module in Python:
import difflib
def spell_check(word, dictionary):
return difflib.get_close_matches(word, dictionary, n=1, cutoff=0.6)
# Example usage
dictionary = ["apple", "banana", "cherry", "date", "elderberry"]
misspelled = "banan"
print(spell_check(misspelled, dictionary)) # Output: ['banana']
2. Fuzzy Search in Databases
Many database systems support fuzzy matching capabilities, allowing users to find records that approximately match a given query. For example, PostgreSQL provides the `pg_trgm` extension for trigram-based similarity searches:
-- Enable the pg_trgm extension
CREATE EXTENSION pg_trgm;
-- Create a table with a trigram index
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name TEXT
);
CREATE INDEX idx_users_name_trgm ON users USING gin (name gin_trgm_ops);
-- Perform a fuzzy search
SELECT name, similarity(name, 'John Doe') AS sim
FROM users
WHERE name % 'John Doe'
ORDER BY sim DESC
LIMIT 10;
3. Duplicate Detection in Data Cleansing
Data cleansing often involves identifying and merging duplicate records that may have slight variations. Approximate string matching techniques are crucial for this task.
Here’s an example using the `fuzzywuzzy` library in Python:
from fuzzywuzzy import fuzz
from fuzzywuzzy import process
def find_duplicates(names, threshold=80):
duplicates = []
for i, name1 in enumerate(names):
for name2 in names[i+1:]:
similarity = fuzz.ratio(name1, name2)
if similarity >= threshold:
duplicates.append((name1, name2, similarity))
return duplicates
# Example usage
names = ["John Doe", "Jane Doe", "Jon Doe", "John D.", "Mary Smith"]
print(find_duplicates(names))
4. Bioinformatics: DNA Sequence Alignment
In bioinformatics, approximate string matching is used to align DNA sequences, allowing for mutations, insertions, and deletions. The Smith-Waterman algorithm is a popular choice for local sequence alignment.
Here’s a simplified implementation of the Smith-Waterman algorithm:
def smith_waterman(seq1, seq2, match_score=2, mismatch_score=-1, gap_penalty=-1):
m, n = len(seq1), len(seq2)
score_matrix = [[0] * (n + 1) for _ in range(m + 1)]
max_score = 0
max_pos = (0, 0)
for i in range(1, m + 1):
for j in range(1, n + 1):
match = score_matrix[i-1][j-1] + (match_score if seq1[i-1] == seq2[j-1] else mismatch_score)
delete = score_matrix[i-1][j] + gap_penalty
insert = score_matrix[i][j-1] + gap_penalty
score_matrix[i][j] = max(0, match, delete, insert)
if score_matrix[i][j] > max_score:
max_score = score_matrix[i][j]
max_pos = (i, j)
return max_score, max_pos
# Example usage
seq1 = "ACGTACGT"
seq2 = "ACGTACGG"
score, position = smith_waterman(seq1, seq2)
print(f"Alignment score: {score}, End position: {position}")
Advanced Techniques and Optimizations
As we delve deeper into approximate string matching, there are several advanced techniques and optimizations worth exploring:
1. Indexing for Faster Matching
For large datasets, precomputing indexes can significantly speed up approximate string matching. Some popular indexing techniques include:
- Inverted Index: Maps n-grams to the documents or strings containing them.
- BK-tree: A tree structure that allows for efficient searching of strings within a certain edit distance.
- Locality-Sensitive Hashing (LSH): Hashes similar items into the same buckets with high probability.
2. Phonetic Algorithms
Phonetic algorithms encode strings based on their pronunciation, which can be useful for matching names or words that sound similar but are spelled differently. Common phonetic algorithms include:
- Soundex: Encodes consonants while preserving the first letter.
- Metaphone: An improvement over Soundex that handles various linguistic rules.
- Double Metaphone: Produces two encodings to account for multiple possible pronunciations.
Here’s an example implementation of the Soundex algorithm in Python:
def soundex(name):
name = name.upper()
soundex = name[0]
# Mapping of consonants to Soundex codes
code_map = {
'BFPV': '1', 'CGJKQSXZ': '2', 'DT': '3',
'L': '4', 'MN': '5', 'R': '6'
}
for char in name[1:]:
for key in code_map:
if char in key:
code = code_map[key]
if code != soundex[-1]: # Avoid adjacent duplicates
soundex += code
soundex = soundex.replace('0', '') # Remove zeros
soundex = soundex.ljust(4, '0')[:4] # Pad with zeros and truncate
return soundex
# Example usage
print(soundex("Robert")) # Output: R163
print(soundex("Rupert")) # Output: R163
3. Machine Learning Approaches
Machine learning techniques can be applied to approximate string matching to improve accuracy and efficiency:
- Learned Edit Distance: Using neural networks to learn optimal edit costs based on training data.
- Siamese Networks: Training neural networks to produce similar embeddings for similar strings.
- Transformer Models: Leveraging pre-trained language models for contextual string similarity.
4. Approximate String Matching in Streaming Data
For real-time applications dealing with streaming data, traditional algorithms may not be sufficient. Techniques for approximate matching in streams include:
- Sliding Window Algorithms: Maintaining a fixed-size window of recent data for matching.
- Sketch Algorithms: Using probabilistic data structures like Count-Min Sketch for approximate matching.
- Online Learning: Continuously updating matching models as new data arrives.
Challenges and Considerations
While approximate string matching is a powerful technique, it comes with its own set of challenges and considerations:
1. Performance vs. Accuracy Trade-offs
More accurate matching often comes at the cost of increased computational complexity. It’s crucial to find the right balance between performance and accuracy for your specific use case.
2. Handling Large-Scale Data
As data volumes grow, efficient indexing and distributed computing techniques become essential for maintaining reasonable performance.
3. Language and Domain Specificity
Different languages and domains may require specialized algorithms or tuning. For example, matching Chinese characters may need different approaches compared to matching English words.
4. Privacy and Security Concerns
In some applications, such as healthcare or finance, approximate matching must be done in a privacy-preserving manner, potentially using techniques like secure multi-party computation or homomorphic encryption.
Conclusion
Approximate string matching is a fascinating and essential technique in the world of computer science and data processing. From simple edit distance calculations to advanced machine learning approaches, the field offers a rich set of tools and algorithms to tackle the challenge of finding similar strings in various contexts.
As we’ve explored in this guide, the applications of approximate string matching are vast and diverse, ranging from everyday spell-checking to cutting-edge bioinformatics research. By understanding the fundamental concepts, algorithms, and practical implementations, you’re now equipped to apply these techniques to your own projects and challenges.
Remember that the choice of algorithm and approach depends heavily on your specific use case, data characteristics, and performance requirements. As with any powerful tool, it’s essential to use approximate string matching judiciously, always considering the trade-offs between accuracy, performance, and complexity.
As data continues to grow in volume and importance, the field of approximate string matching will undoubtedly continue to evolve, with new algorithms, optimizations, and applications emerging. By mastering these techniques, you’ll be well-prepared to tackle the data challenges of today and tomorrow, unlocking new insights and possibilities in the world of information processing.