Implementing MapReduce Algorithms: A Comprehensive Guide
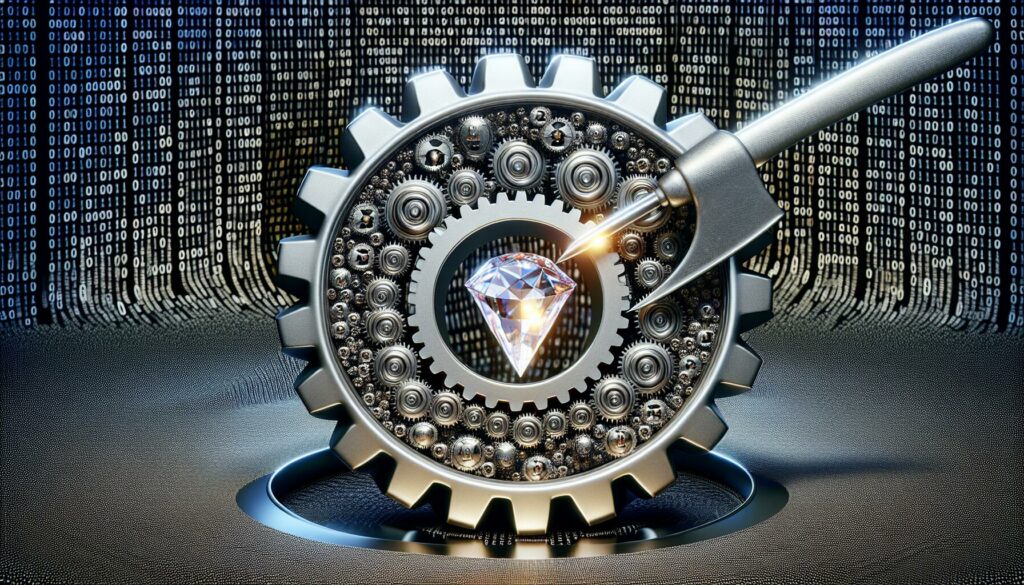
In the world of big data processing and distributed computing, MapReduce has emerged as a powerful paradigm for handling large-scale data analysis tasks. Originally developed by Google, MapReduce has become a cornerstone of many modern data processing frameworks. In this comprehensive guide, we’ll dive deep into the concept of MapReduce, explore its implementation, and walk through practical examples to help you master this essential technique in the realm of distributed computing.
Table of Contents
- Understanding MapReduce
- The MapReduce Workflow
- Implementing MapReduce Algorithms
- Practical MapReduce Examples
- Popular MapReduce Frameworks
- Best Practices for MapReduce Implementation
- Challenges and Limitations of MapReduce
- The Future of MapReduce
- Conclusion
1. Understanding MapReduce
MapReduce is a programming model and processing technique designed for distributed computing on large datasets. It allows developers to write scalable, fault-tolerant applications that can process vast amounts of data in parallel across a cluster of machines.
The core idea behind MapReduce is to break down a complex computation into two main phases:
- Map: This phase processes input data and generates intermediate key-value pairs.
- Reduce: This phase aggregates the intermediate results to produce the final output.
By dividing the computation into these two phases, MapReduce enables efficient parallel processing and distribution of work across multiple nodes in a cluster.
2. The MapReduce Workflow
To better understand how MapReduce works, let’s break down its workflow into steps:
- Input Splitting: The input data is divided into fixed-size chunks, called splits or input splits.
- Map Phase: The map function is applied to each split, processing the data and emitting intermediate key-value pairs.
- Shuffling and Sorting: The intermediate key-value pairs are grouped by key and sorted.
- Reduce Phase: The reduce function is applied to each group of values associated with a key, producing the final output.
- Output: The results are written to the output location, typically a distributed file system.
This workflow allows for efficient parallel processing of large datasets, as each step can be executed independently on different nodes in the cluster.
3. Implementing MapReduce Algorithms
To implement a MapReduce algorithm, you need to define two main functions: the map function and the reduce function. Let’s look at the general structure of these functions:
Map Function
The map function takes an input key-value pair and produces a set of intermediate key-value pairs. Its general signature is:
map(input_key, input_value) -> list(intermediate_key, intermediate_value)
Reduce Function
The reduce function takes an intermediate key and a list of values associated with that key, and produces a final output. Its general signature is:
reduce(intermediate_key, list(intermediate_value)) -> list(output_value)
Now, let’s implement a simple MapReduce algorithm to count the occurrences of words in a large text corpus using Python:
def map_function(_, line):
words = line.split()
for word in words:
yield (word.lower(), 1)
def reduce_function(word, counts):
yield (word, sum(counts))
# Example usage
input_data = [
"Hello World",
"Hello MapReduce",
"MapReduce is awesome"
]
# Simulate MapReduce execution
mapped_data = []
for line in input_data:
mapped_data.extend(map_function(None, line))
# Group by key
grouped_data = {}
for key, value in mapped_data:
if key not in grouped_data:
grouped_data[key] = []
grouped_data[key].append(value)
# Reduce phase
result = []
for word, counts in grouped_data.items():
result.extend(reduce_function(word, counts))
print(result)
This example demonstrates a simple word count implementation using MapReduce. The map function splits each line into words and emits a key-value pair for each word, with the word as the key and a count of 1 as the value. The reduce function then sums up the counts for each word to produce the final result.
4. Practical MapReduce Examples
Let’s explore a few more practical examples of MapReduce algorithms to solidify our understanding:
Example 1: Calculating Average Temperature
Suppose we have a large dataset of temperature readings from various weather stations. We want to calculate the average temperature for each station.
def map_function(station_id, temperature):
yield (station_id, (temperature, 1))
def reduce_function(station_id, temperature_data):
total_temp = sum(temp for temp, _ in temperature_data)
count = sum(count for _, count in temperature_data)
average_temp = total_temp / count
yield (station_id, average_temp)
# Example usage
input_data = [
("Station1", 25),
("Station2", 30),
("Station1", 27),
("Station2", 28),
("Station1", 26)
]
# Simulate MapReduce execution
mapped_data = []
for station_id, temperature in input_data:
mapped_data.extend(map_function(station_id, temperature))
# Group by key
grouped_data = {}
for key, value in mapped_data:
if key not in grouped_data:
grouped_data[key] = []
grouped_data[key].append(value)
# Reduce phase
result = []
for station_id, temperature_data in grouped_data.items():
result.extend(reduce_function(station_id, temperature_data))
print(result)
Example 2: Finding Maximum Value
Let’s implement a MapReduce algorithm to find the maximum value in a large dataset of numbers.
def map_function(_, number):
yield ("max", number)
def reduce_function(key, numbers):
yield (key, max(numbers))
# Example usage
input_data = [10, 5, 8, 12, 3, 7, 9, 15, 1]
# Simulate MapReduce execution
mapped_data = []
for number in input_data:
mapped_data.extend(map_function(None, number))
# Group by key
grouped_data = {}
for key, value in mapped_data:
if key not in grouped_data:
grouped_data[key] = []
grouped_data[key].append(value)
# Reduce phase
result = []
for key, numbers in grouped_data.items():
result.extend(reduce_function(key, numbers))
print(result)
5. Popular MapReduce Frameworks
While we’ve implemented simple MapReduce algorithms in Python, real-world applications often use specialized frameworks designed for distributed computing. Here are some popular MapReduce frameworks:
Apache Hadoop
Hadoop is an open-source framework that allows for distributed processing of large datasets across clusters of computers. It includes the Hadoop Distributed File System (HDFS) for storage and the MapReduce programming model for processing.
Apache Spark
Spark is a fast and general-purpose cluster computing system that provides high-level APIs in Java, Scala, Python, and R. While not strictly a MapReduce framework, Spark can efficiently perform MapReduce-like operations and offers additional functionality for stream processing and machine learning.
Google Cloud Dataflow
Dataflow is a fully managed service for executing Apache Beam pipelines within the Google Cloud Platform ecosystem. It provides a programming model that generalizes the MapReduce paradigm and can be used for both batch and streaming data processing.
Amazon EMR
Amazon Elastic MapReduce (EMR) is a cloud-based big data platform that allows you to process vast amounts of data using open-source tools such as Apache Spark, Apache Hive, Apache HBase, Apache Flink, and Presto.
6. Best Practices for MapReduce Implementation
When implementing MapReduce algorithms, consider the following best practices to optimize performance and ensure reliability:
- Minimize data transfer: Design your map and reduce functions to minimize the amount of data transferred between nodes.
- Use combiners: Implement a combiner function to perform local aggregation before the shuffle and sort phase, reducing network traffic.
- Partition data effectively: Choose appropriate partitioning strategies to ensure even distribution of work across reducers.
- Handle skewed data: Be aware of potential data skew and implement strategies to mitigate its impact on performance.
- Optimize I/O operations: Minimize disk I/O by using in-memory processing where possible and compressing intermediate data.
- Test thoroughly: Validate your MapReduce jobs on smaller datasets before running them on large-scale production data.
- Monitor and tune: Use monitoring tools to identify bottlenecks and optimize your MapReduce jobs iteratively.
7. Challenges and Limitations of MapReduce
While MapReduce is a powerful paradigm for distributed computing, it does have some challenges and limitations:
- Complexity: Writing efficient MapReduce algorithms can be challenging, especially for complex computations that don’t naturally fit the MapReduce model.
- Performance overhead: The MapReduce framework introduces some overhead due to data serialization, network transfer, and disk I/O operations.
- Iterative algorithms: MapReduce is not well-suited for iterative algorithms that require multiple passes over the data, as each iteration typically requires a new MapReduce job.
- Real-time processing: Traditional MapReduce is designed for batch processing and may not be suitable for real-time or low-latency applications.
- Lack of built-in state management: MapReduce doesn’t provide built-in mechanisms for maintaining state between jobs, which can complicate certain types of computations.
8. The Future of MapReduce
As the field of big data and distributed computing continues to evolve, new paradigms and technologies are emerging that build upon or complement the MapReduce model:
- Stream processing: Frameworks like Apache Flink and Apache Kafka Streams enable real-time data processing, addressing some of the limitations of batch-oriented MapReduce.
- In-memory computing: Technologies like Apache Spark leverage in-memory processing to improve performance for iterative algorithms and interactive data analysis.
- Serverless computing: Cloud providers are offering serverless big data processing services that abstract away the complexities of cluster management and scaling.
- Machine learning integration: Many modern data processing frameworks are incorporating machine learning capabilities, allowing for seamless integration of ML models into data pipelines.
While these advancements are expanding the possibilities of distributed computing, the core principles of MapReduce remain relevant and continue to influence the design of new systems and algorithms.
9. Conclusion
MapReduce has revolutionized the way we process and analyze large-scale datasets. By breaking down complex computations into map and reduce phases, it enables efficient parallel processing across distributed systems. While implementing MapReduce algorithms can be challenging, mastering this paradigm opens up a world of possibilities in big data processing and analysis.
As you continue your journey in distributed computing and big data, remember that MapReduce is just one tool in your arsenal. Familiarize yourself with modern frameworks and technologies that build upon MapReduce principles, and always consider the specific requirements of your use case when choosing the right approach for your data processing needs.
By understanding the fundamentals of MapReduce and staying up-to-date with emerging trends in distributed computing, you’ll be well-equipped to tackle the challenges of processing and analyzing large-scale datasets in today’s data-driven world.