Cache Algorithms: FIFO vs. LRU vs. LFU – A Comprehensive Guide
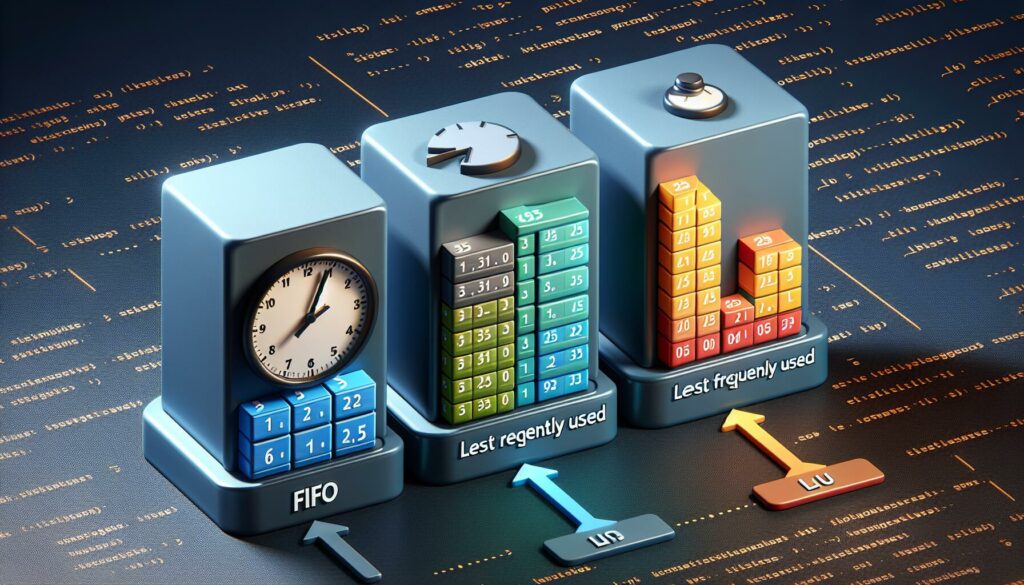
In the world of computer science and software engineering, caching plays a crucial role in improving system performance and reducing latency. At the heart of any caching system are the algorithms that determine which items to keep in the cache and which to evict when the cache reaches its capacity. In this comprehensive guide, we’ll dive deep into three popular cache algorithms: FIFO (First-In-First-Out), LRU (Least Recently Used), and LFU (Least Frequently Used).
Whether you’re preparing for a technical interview at a major tech company or simply looking to enhance your understanding of caching mechanisms, this article will provide you with the knowledge and insights you need to master these important concepts.
Table of Contents
- Introduction to Caching
- FIFO (First-In-First-Out) Algorithm
- LRU (Least Recently Used) Algorithm
- LFU (Least Frequently Used) Algorithm
- Comparison of FIFO, LRU, and LFU
- Implementation Examples
- Real-world Use Cases
- Conclusion
1. Introduction to Caching
Before we delve into the specific cache algorithms, let’s briefly review what caching is and why it’s important in computer systems.
Caching is a technique used to store frequently accessed data in a faster, more easily accessible location. The main purpose of caching is to improve system performance by reducing the time required to access data. When data is requested, the system first checks the cache. If the data is found in the cache (a cache hit), it can be quickly retrieved. If the data is not in the cache (a cache miss), it must be fetched from the slower main memory or storage.
The effectiveness of a cache depends on several factors, including:
- Cache size
- Cache replacement policy (algorithm)
- Access patterns of the data
In this article, we’ll focus on the cache replacement policies, specifically comparing FIFO, LRU, and LFU algorithms.
2. FIFO (First-In-First-Out) Algorithm
The First-In-First-Out (FIFO) algorithm is one of the simplest cache replacement policies. As its name suggests, it operates on the principle that the first item added to the cache is the first one to be removed when the cache reaches its capacity.
How FIFO Works
- When a new item needs to be added to the cache, and the cache is full, the algorithm removes the oldest item (the one that was added first).
- The new item is then added to the cache.
- This process continues, maintaining the order in which items were added to the cache.
Advantages of FIFO
- Simple to implement and understand
- Low overhead in terms of time and space complexity
- Works well for workloads with a consistent access pattern
Disadvantages of FIFO
- Does not consider the frequency or recency of access to items
- Can lead to poor performance if frequently accessed items are evicted simply because they were added earlier
- May not adapt well to changing access patterns
FIFO Implementation Concept
Here’s a conceptual implementation of the FIFO algorithm using a queue data structure:
class FIFOCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.queue = []
def get(self, key):
if key in self.cache:
return self.cache[key]
return -1
def put(self, key, value):
if key not in self.cache:
if len(self.cache) >= self.capacity:
oldest_key = self.queue.pop(0)
del self.cache[oldest_key]
self.queue.append(key)
self.cache[key] = value
In this implementation, we use a dictionary (self.cache
) to store key-value pairs and a list (self.queue
) to keep track of the order in which items were added to the cache.
3. LRU (Least Recently Used) Algorithm
The Least Recently Used (LRU) algorithm is a more sophisticated cache replacement policy that takes into account the recency of access to items in the cache. It operates on the principle that the least recently used item should be the first to be evicted when the cache is full.
How LRU Works
- When an item is accessed (read or updated), it is moved to the front of the cache, marking it as the most recently used.
- When a new item needs to be added to the cache, and the cache is full, the algorithm removes the least recently used item (the one at the back of the cache).
- The new item is then added to the front of the cache.
Advantages of LRU
- Adapts well to changing access patterns
- Performs well for workloads with temporal locality (recently accessed items are likely to be accessed again soon)
- Balances simplicity and effectiveness
Disadvantages of LRU
- Slightly more complex to implement efficiently compared to FIFO
- Does not consider the frequency of access, only recency
- Can perform poorly for cyclic access patterns larger than the cache size
LRU Implementation Concept
Here’s a conceptual implementation of the LRU algorithm using a combination of a dictionary and a doubly linked list:
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.value
return -1
def put(self, key, value):
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self._add(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
lru = self.head.next
self._remove(lru)
del self.cache[lru.key]
def _remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _add(self, node):
node.prev = self.tail.prev
node.next = self.tail
self.tail.prev.next = node
self.tail.prev = node
In this implementation, we use a dictionary (self.cache
) for fast key-value lookups and a doubly linked list to maintain the order of items based on their recency of use.
4. LFU (Least Frequently Used) Algorithm
The Least Frequently Used (LFU) algorithm is another cache replacement policy that focuses on the frequency of access to items in the cache. It operates on the principle that the least frequently used item should be the first to be evicted when the cache is full.
How LFU Works
- Each item in the cache is associated with a counter that tracks how many times it has been accessed.
- When an item is accessed, its counter is incremented.
- When a new item needs to be added to the cache, and the cache is full, the algorithm removes the item with the lowest access count.
- If multiple items have the same lowest count, the algorithm typically uses a secondary criterion (such as least recently used) to break the tie.
Advantages of LFU
- Performs well for workloads with stable access frequencies
- Can be more effective than LRU for certain types of access patterns
- Takes into account long-term popularity of items
Disadvantages of LFU
- More complex to implement efficiently compared to FIFO and LRU
- May not adapt quickly to changing access patterns
- Can lead to “cache pollution” where infrequently used items remain in the cache for a long time due to high initial access counts
LFU Implementation Concept
Here’s a conceptual implementation of the LFU algorithm using dictionaries and a min-heap:
import heapq
class Node:
def __init__(self, key, value, freq=1):
self.key = key
self.value = value
self.freq = freq
self.time = 0 # Used as a tie-breaker
def __lt__(self, other):
if self.freq == other.freq:
return self.time < other.time
return self.freq < other.freq
class LFUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.freq_heap = []
self.time = 0
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._update_freq(node)
return node.value
return -1
def put(self, key, value):
self.time += 1
if key in self.cache:
node = self.cache[key]
node.value = value
self._update_freq(node)
else:
if len(self.cache) >= self.capacity:
while self.freq_heap and self.freq_heap[0].key not in self.cache:
heapq.heappop(self.freq_heap)
if self.freq_heap:
lfu = heapq.heappop(self.freq_heap)
del self.cache[lfu.key]
node = Node(key, value)
node.time = self.time
self.cache[key] = node
heapq.heappush(self.freq_heap, node)
def _update_freq(self, node):
node.freq += 1
node.time = self.time
heapq.heapify(self.freq_heap)
In this implementation, we use a dictionary (self.cache
) for fast key-value lookups and a min-heap (self.freq_heap
) to efficiently track and retrieve the least frequently used items.
5. Comparison of FIFO, LRU, and LFU
Now that we’ve explored each of these cache algorithms in detail, let’s compare them side by side:
Aspect | FIFO | LRU | LFU |
---|---|---|---|
Principle | Evicts oldest item | Evicts least recently used item | Evicts least frequently used item |
Complexity | Low | Medium | High |
Adaptability | Poor | Good | Moderate |
Performance for temporal locality | Poor | Excellent | Good |
Performance for frequency-based patterns | Poor | Moderate | Excellent |
Overhead | Low | Medium | High |
6. Implementation Examples
To further illustrate how these algorithms work in practice, let’s implement a simple cache using each algorithm and run through a series of operations.
FIFO Cache Example
class FIFOCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.queue = []
def get(self, key):
return self.cache.get(key, -1)
def put(self, key, value):
if key not in self.cache:
if len(self.cache) >= self.capacity:
oldest_key = self.queue.pop(0)
del self.cache[oldest_key]
self.queue.append(key)
self.cache[key] = value
# Usage example
fifo_cache = FIFOCache(3)
fifo_cache.put(1, 1)
fifo_cache.put(2, 2)
fifo_cache.put(3, 3)
print(fifo_cache.get(1)) # Output: 1
fifo_cache.put(4, 4)
print(fifo_cache.get(1)) # Output: 1
print(fifo_cache.get(2)) # Output: -1 (evicted)
LRU Cache Example
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
# Usage example
lru_cache = LRUCache(3)
lru_cache.put(1, 1)
lru_cache.put(2, 2)
lru_cache.put(3, 3)
print(lru_cache.get(1)) # Output: 1
lru_cache.put(4, 4)
print(lru_cache.get(1)) # Output: 1
print(lru_cache.get(2)) # Output: -1 (evicted)
LFU Cache Example
from collections import defaultdict
import heapq
class LFUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.freq = defaultdict(set)
self.min_freq = 0
self.time = 0
def get(self, key):
if key not in self.cache:
return -1
self._update_freq(key)
return self.cache[key][0]
def put(self, key, value):
self.time += 1
if self.capacity == 0:
return
if key in self.cache:
self.cache[key] = (value, self.cache[key][1])
self._update_freq(key)
else:
if len(self.cache) >= self.capacity:
self._evict()
self.cache[key] = (value, 1)
self.freq[1].add(key)
self.min_freq = 1
def _update_freq(self, key):
value, freq = self.cache[key]
self.cache[key] = (value, freq + 1)
self.freq[freq].remove(key)
if not self.freq[freq] and freq == self.min_freq:
self.min_freq += 1
self.freq[freq + 1].add(key)
def _evict(self):
key = self.freq[self.min_freq].pop()
if not self.freq[self.min_freq]:
del self.freq[self.min_freq]
del self.cache[key]
# Usage example
lfu_cache = LFUCache(3)
lfu_cache.put(1, 1)
lfu_cache.put(2, 2)
lfu_cache.put(3, 3)
print(lfu_cache.get(1)) # Output: 1
lfu_cache.put(4, 4)
print(lfu_cache.get(1)) # Output: 1
print(lfu_cache.get(2)) # Output: -1 (evicted)
7. Real-world Use Cases
Understanding when to use each cache algorithm is crucial for optimizing system performance. Here are some real-world scenarios where each algorithm might be most appropriate:
FIFO (First-In-First-Out)
- Content Delivery Networks (CDNs): For caching static assets that are updated periodically, FIFO can be effective in ensuring that older versions of assets are removed first.
- Embedded Systems: In resource-constrained environments where simplicity and low overhead are crucial, FIFO can be a good choice.
- Streaming Data: When dealing with time-series data or log processing, where older data becomes less relevant over time, FIFO can be appropriate.
LRU (Least Recently Used)
- Database Query Caches: LRU works well for caching query results, as recently accessed data is likely to be accessed again soon.
- Web Browsers: Browser caches often use LRU to store recently visited web pages and resources.
- File System Caches: Operating systems frequently use LRU for caching file system data, as files accessed recently are likely to be accessed again.
LFU (Least Frequently Used)
- Content Recommendation Systems: LFU can be effective in caching popular items that are frequently accessed by users.
- CDN Edge Caches: For caching content based on long-term popularity, LFU can help optimize storage of frequently requested resources.
- In-memory Databases: When dealing with hot and cold data in memory-constrained environments, LFU can help prioritize frequently accessed data.
8. Conclusion
Cache algorithms play a crucial role in optimizing system performance and resource utilization. FIFO, LRU, and LFU each have their strengths and weaknesses, and the choice of algorithm depends on the specific use case and access patterns of the data being cached.
To summarize:
- FIFO is simple and works well for consistent access patterns but may not adapt to changing workloads.
- LRU is versatile and performs well for workloads with temporal locality, making it a popular choice for many caching scenarios.
- LFU excels at capturing long-term popularity but may be slower to adapt to changing access patterns and more complex to implement efficiently.
When designing or optimizing a caching system, it’s essential to consider the specific requirements of your application, the nature of the data being cached, and the expected access patterns. In some cases, hybrid approaches or more advanced algorithms might be necessary to achieve optimal performance.
As you continue to develop your skills in software engineering and prepare for technical interviews, understanding these cache algorithms and their trade-offs will be invaluable. Practice implementing these algorithms, analyze their behavior with different workloads, and consider how they might be applied to real-world problems you encounter in your projects or interview questions.
Remember, mastering these concepts is not just about memorizing implementations but about understanding the underlying principles and being able to apply them creatively to solve complex problems efficiently. Keep practicing, experimenting, and exploring variations and optimizations of these algorithms to deepen your understanding and sharpen your skills as a software engineer.