Algorithmic Approaches to Clustering Data: A Comprehensive Guide
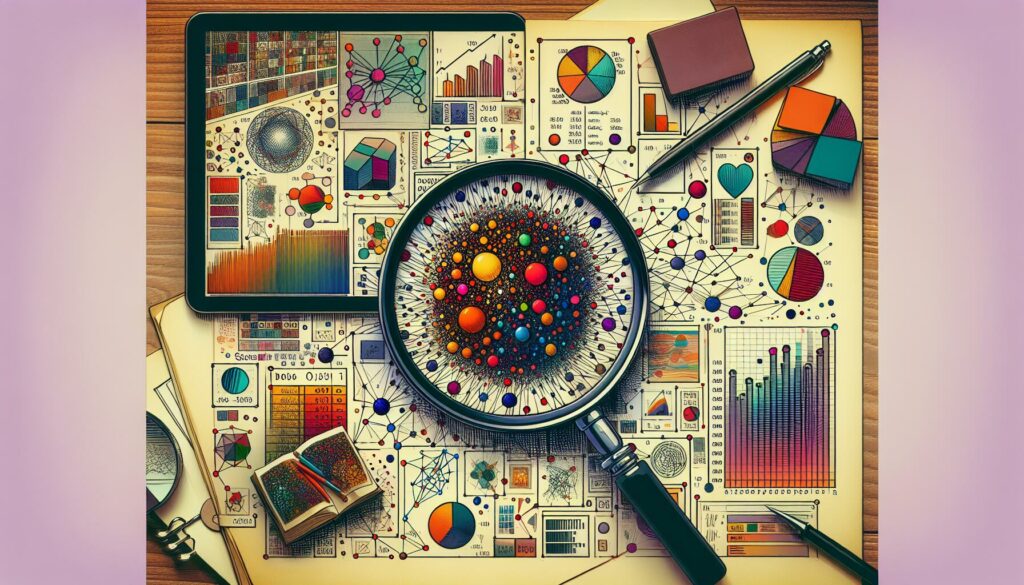
In the world of data science and machine learning, clustering is a fundamental technique used to group similar data points together. This process of organizing data into meaningful clusters is essential for various applications, from customer segmentation in marketing to anomaly detection in cybersecurity. In this comprehensive guide, we’ll explore different algorithmic approaches to clustering data, their implementations, and their practical applications in the field of computer science.
Table of Contents
- Introduction to Clustering
- K-Means Clustering
- Hierarchical Clustering
- DBSCAN (Density-Based Spatial Clustering of Applications with Noise)
- Gaussian Mixture Models
- Spectral Clustering
- Evaluating Clustering Results
- Real-world Applications of Clustering
- Challenges and Considerations in Clustering
- Conclusion
1. Introduction to Clustering
Clustering is an unsupervised learning technique that aims to group data points based on their similarities. Unlike supervised learning, clustering doesn’t rely on pre-labeled data. Instead, it discovers patterns and structures within the data itself. The goal is to maximize intra-cluster similarity while minimizing inter-cluster similarity.
Before diving into specific algorithms, it’s important to understand some key concepts:
- Distance Metrics: These measure the similarity or dissimilarity between data points. Common metrics include Euclidean distance, Manhattan distance, and cosine similarity.
- Feature Space: The multidimensional space where each dimension represents a feature of the data points.
- Centroid: The center point of a cluster, often calculated as the mean of all points in the cluster.
- Inertia: The sum of squared distances of samples to their closest cluster center.
Now, let’s explore some popular clustering algorithms and their implementations.
2. K-Means Clustering
K-Means is one of the most widely used clustering algorithms due to its simplicity and efficiency. It aims to partition n observations into k clusters, where each observation belongs to the cluster with the nearest mean (centroid).
Algorithm Steps:
- Choose the number of clusters, k.
- Randomly initialize k centroids.
- Assign each data point to the nearest centroid.
- Recalculate the centroids based on the current cluster assignments.
- Repeat steps 3 and 4 until convergence or a maximum number of iterations is reached.
Python Implementation:
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.random.rand(100, 2)
# Create KMeans instance
kmeans = KMeans(n_clusters=3, random_state=42)
# Fit the model
kmeans.fit(X)
# Get cluster assignments and centroids
labels = kmeans.labels_
centroids = kmeans.cluster_centers_
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.scatter(centroids[:, 0], centroids[:, 1], marker='x', s=200, linewidths=3, color='r')
plt.title('K-Means Clustering')
plt.show()
This implementation uses scikit-learn’s KMeans class to cluster 100 random 2D points into 3 clusters. The resulting plot shows the data points colored by their cluster assignments and the centroids marked with red X’s.
Advantages and Disadvantages:
- Advantages:
- Simple and easy to implement
- Scales well to large datasets
- Guarantees convergence
- Disadvantages:
- Requires specifying the number of clusters in advance
- Sensitive to initial centroid positions
- Assumes spherical cluster shapes
- May converge to local optima
3. Hierarchical Clustering
Hierarchical clustering creates a tree-like hierarchy of clusters, known as a dendrogram. There are two main approaches: agglomerative (bottom-up) and divisive (top-down). We’ll focus on the more common agglomerative approach.
Algorithm Steps (Agglomerative):
- Start with each data point as a separate cluster.
- Compute the distance between all pairs of clusters.
- Merge the two closest clusters.
- Update the distances between the new cluster and the remaining clusters.
- Repeat steps 3 and 4 until only one cluster remains or a stopping criterion is met.
Python Implementation:
import numpy as np
from scipy.cluster.hierarchy import dendrogram, linkage
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.random.rand(50, 2)
# Perform hierarchical clustering
Z = linkage(X, method='ward')
# Plot the dendrogram
plt.figure(figsize=(10, 7))
dendrogram(Z)
plt.title('Hierarchical Clustering Dendrogram')
plt.xlabel('Sample Index')
plt.ylabel('Distance')
plt.show()
This implementation uses SciPy’s linkage function to perform hierarchical clustering on 50 random 2D points. The resulting dendrogram visualizes the hierarchical structure of the clusters.
Advantages and Disadvantages:
- Advantages:
- No need to specify the number of clusters in advance
- Produces a hierarchical representation of the data
- Can uncover underlying structure at different scales
- Disadvantages:
- Computationally expensive for large datasets (O(n^2) space complexity, O(n^3) time complexity)
- Sensitive to outliers
- Can be difficult to determine the optimal number of clusters
4. DBSCAN (Density-Based Spatial Clustering of Applications with Noise)
DBSCAN is a density-based clustering algorithm that groups together points that are closely packed together, marking points that lie alone in low-density regions as outliers. It can discover clusters of arbitrary shape and is particularly useful when the number of clusters is unknown.
Algorithm Concepts:
- ε (eps): The maximum distance between two samples for one to be considered as in the neighborhood of the other.
- MinPts: The minimum number of samples in a neighborhood for a point to be considered as a core point.
- Core Point: A point that has at least MinPts points within distance ε of it.
- Border Point: A point that is within distance ε of a core point but is not a core point itself.
- Noise Point: Any point that is neither a core point nor a border point.
Python Implementation:
import numpy as np
from sklearn.cluster import DBSCAN
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.random.rand(100, 2)
# Create DBSCAN instance
dbscan = DBSCAN(eps=0.3, min_samples=5)
# Fit the model and predict clusters
labels = dbscan.fit_predict(X)
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.title('DBSCAN Clustering')
plt.show()
This implementation uses scikit-learn’s DBSCAN class to cluster 100 random 2D points. The resulting plot shows the data points colored by their cluster assignments, with noise points typically labeled as -1.
Advantages and Disadvantages:
- Advantages:
- Does not require specifying the number of clusters beforehand
- Can find arbitrarily shaped clusters
- Robust to outliers
- Only requires two parameters (ε and MinPts)
- Disadvantages:
- Sensitive to the choice of ε and MinPts
- Not suitable for datasets with varying densities
- Can struggle with high-dimensional data due to the “curse of dimensionality”
5. Gaussian Mixture Models
Gaussian Mixture Models (GMMs) are probabilistic models that assume the data is generated from a mixture of a finite number of Gaussian distributions with unknown parameters. GMMs can be viewed as a soft clustering method, where each data point has a probability of belonging to each cluster.
Algorithm Concepts:
- Mixture Components: Each Gaussian distribution in the mixture.
- Expectation-Maximization (EM) Algorithm: Used to estimate the parameters of the GMM.
- Responsibilities: The probability of a data point belonging to each mixture component.
Python Implementation:
import numpy as np
from sklearn.mixture import GaussianMixture
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.concatenate([np.random.normal(0, 1, (100, 2)),
np.random.normal(3, 1.5, (100, 2))])
# Create GaussianMixture instance
gmm = GaussianMixture(n_components=2, random_state=42)
# Fit the model and predict clusters
labels = gmm.fit_predict(X)
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.title('Gaussian Mixture Model Clustering')
plt.show()
This implementation uses scikit-learn’s GaussianMixture class to cluster data generated from two Gaussian distributions. The resulting plot shows the data points colored by their most likely cluster assignments.
Advantages and Disadvantages:
- Advantages:
- Provides soft cluster assignments (probabilities)
- Can model clusters with different sizes and shapes
- Naturally handles uncertainty in cluster assignments
- Disadvantages:
- Requires specifying the number of components in advance
- Sensitive to initialization
- May converge to local optima
- Assumes Gaussian distributions, which may not always be appropriate
6. Spectral Clustering
Spectral clustering is a technique that uses the eigenvalues of the similarity matrix of the data to perform dimensionality reduction before clustering in fewer dimensions. It’s particularly effective for data that can be represented as a graph.
Algorithm Steps:
- Construct a similarity graph between data points.
- Compute the Laplacian matrix of the graph.
- Compute the first k eigenvectors of the Laplacian matrix.
- Form a matrix with these k eigenvectors as columns.
- Cluster the rows of this matrix using K-means or another algorithm.
Python Implementation:
import numpy as np
from sklearn.cluster import SpectralClustering
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.concatenate([np.random.normal(0, 1, (100, 2)),
np.random.normal(4, 1, (100, 2))])
# Create SpectralClustering instance
spectral = SpectralClustering(n_clusters=2, random_state=42)
# Fit the model and get cluster labels
labels = spectral.fit_predict(X)
# Plot the results
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='viridis')
plt.title('Spectral Clustering')
plt.show()
This implementation uses scikit-learn’s SpectralClustering class to cluster data generated from two normal distributions. The resulting plot shows the data points colored by their cluster assignments.
Advantages and Disadvantages:
- Advantages:
- Can find clusters with complex shapes
- Works well when the data has a graph-like structure
- Can be more effective than traditional clustering algorithms in certain scenarios
- Disadvantages:
- Computationally expensive for large datasets
- Sensitive to the choice of similarity measure and the number of eigenvectors
- Can be challenging to interpret the results
7. Evaluating Clustering Results
Evaluating the quality of clustering results can be challenging, especially in unsupervised settings where there are no ground truth labels. However, several metrics and techniques can be used to assess clustering performance:
Internal Evaluation Metrics:
- Silhouette Score: Measures how similar an object is to its own cluster compared to other clusters.
- Calinski-Harabasz Index: Ratio of between-cluster dispersion to within-cluster dispersion.
- Davies-Bouldin Index: The average similarity between each cluster and its most similar cluster.
External Evaluation Metrics (when ground truth is available):
- Adjusted Rand Index (ARI): Measures the similarity between two clusterings, adjusted for chance.
- Normalized Mutual Information (NMI): Measures the mutual information between the clustering and the ground truth, normalized to scale between 0 and 1.
- V-measure: Harmonic mean of homogeneity and completeness.
Python Implementation of Silhouette Score:
from sklearn.metrics import silhouette_score
from sklearn.cluster import KMeans
import numpy as np
# Generate sample data
np.random.seed(42)
X = np.random.rand(100, 2)
# Perform K-means clustering
kmeans = KMeans(n_clusters=3, random_state=42)
labels = kmeans.fit_predict(X)
# Calculate silhouette score
score = silhouette_score(X, labels)
print(f"Silhouette Score: {score:.3f}")
This code calculates the silhouette score for a K-means clustering of random 2D data. A higher silhouette score indicates better-defined clusters.
8. Real-world Applications of Clustering
Clustering algorithms have a wide range of applications across various domains:
- Customer Segmentation: Grouping customers based on purchasing behavior, demographics, or preferences to tailor marketing strategies.
- Image Segmentation: Partitioning digital images into multiple segments or objects for analysis or compression.
- Anomaly Detection: Identifying unusual patterns in data, useful in fraud detection, network security, and medical diagnosis.
- Document Clustering: Organizing large collections of texts into topically related groups for information retrieval or summarization.
- Recommender Systems: Grouping similar items or users to make personalized recommendations.
- Bioinformatics: Clustering gene expression data to identify functionally related genes or to study evolutionary relationships.
- Social Network Analysis: Identifying communities or influential nodes in social networks.
- Urban Planning: Analyzing spatial data to identify areas with similar characteristics for zoning or resource allocation.
9. Challenges and Considerations in Clustering
While clustering is a powerful technique, it comes with several challenges and considerations:
- Choosing the Right Algorithm: Different algorithms are suited for different types of data and clustering objectives.
- Determining the Number of Clusters: Many algorithms require specifying the number of clusters in advance, which can be difficult to determine.
- Handling High-Dimensional Data: The “curse of dimensionality” can make distance-based clustering less effective in high-dimensional spaces.
- Scalability: Some algorithms struggle with very large datasets.
- Interpreting Results: Understanding and validating the meaning of discovered clusters can be challenging, especially in high-dimensional spaces.
- Dealing with Outliers: Outliers can significantly impact the results of some clustering algorithms.
- Feature Selection and Preprocessing: Choosing relevant features and properly scaling or normalizing data can greatly affect clustering results.
- Cluster Stability: Ensuring that clustering results are stable and not overly sensitive to small changes in the data or algorithm parameters.
10. Conclusion
Clustering is a fundamental technique in data analysis and machine learning, offering powerful ways to uncover structure in unlabeled data. From the simple and widely-used K-means algorithm to more sophisticated methods like DBSCAN and Spectral Clustering, each approach has its strengths and limitations.
As we’ve explored in this guide, the choice of clustering algorithm depends on various factors, including the nature of the data, the specific problem at hand, and computational constraints. It’s crucial for data scientists and machine learning practitioners to understand the underlying principles of these algorithms, their implementations, and how to evaluate their results.
Moreover, the real-world applications of clustering span across numerous domains, from business analytics to scientific research, demonstrating its versatility and importance. As data continues to grow in volume and complexity, clustering techniques will undoubtedly play an increasingly vital role in extracting meaningful insights and patterns.
By mastering these algorithmic approaches to clustering data, you’ll be well-equipped to tackle a wide range of data analysis challenges and contribute to the ever-evolving field of data science and machine learning.