Algorithms for Handling Streaming Data: Mastering Real-Time Data Processing
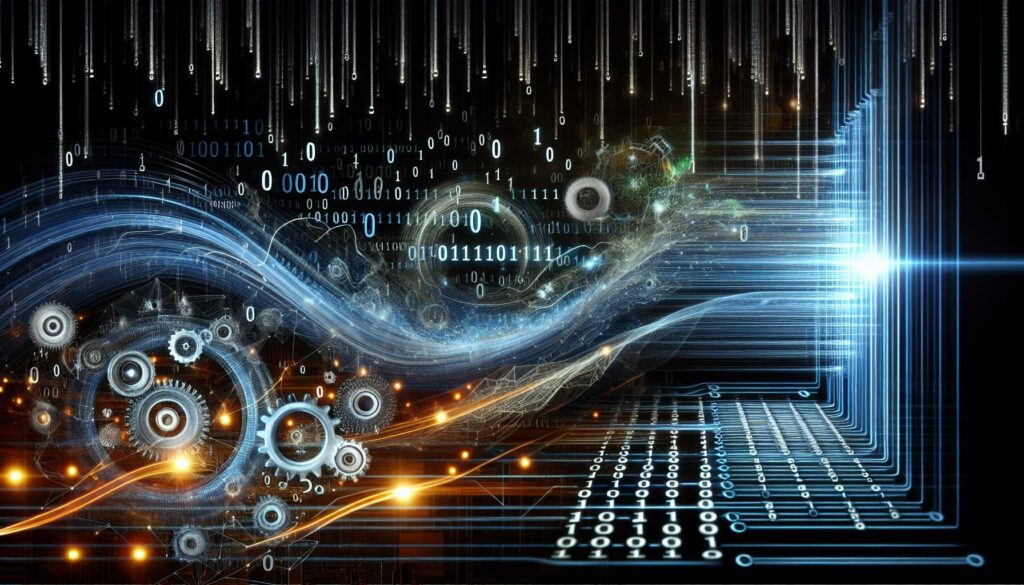
In today’s data-driven world, the ability to process and analyze large volumes of data in real-time has become increasingly important. This is where streaming data algorithms come into play. These algorithms are designed to handle continuous streams of data, allowing for quick decision-making and insights. In this comprehensive guide, we’ll explore various algorithms for handling streaming data, their applications, and how they can be implemented in your coding projects.
Table of Contents
- Introduction to Streaming Data
- Challenges in Handling Streaming Data
- Key Algorithms for Streaming Data
- Reservoir Sampling
- Count-Min Sketch
- HyperLogLog
- Bloom Filters
- Sliding Window Algorithms
- Hoeffding Trees
- Real-World Applications
- Implementing Streaming Algorithms
- Conclusion
1. Introduction to Streaming Data
Streaming data refers to data that is generated continuously, typically in high volumes and at high velocity. Examples include social media feeds, sensor data from IoT devices, financial market data, and log files from web servers. Unlike traditional batch processing, where data is collected over time and then processed, streaming data requires real-time or near-real-time processing.
The key characteristics of streaming data include:
- Continuous flow: Data arrives in a never-ending stream
- High velocity: Data is generated at a rapid pace
- Unbounded size: The total volume of data is potentially infinite
- Real-time processing: Data needs to be processed as it arrives
2. Challenges in Handling Streaming Data
Processing streaming data presents several unique challenges:
- Limited memory: It’s often impractical or impossible to store all the data in memory
- Single-pass constraint: Algorithms must process each data point only once
- Real-time requirements: Processing must keep up with the incoming data rate
- Evolving data distributions: The nature of the data may change over time (concept drift)
- Out-of-order data: Data points may arrive out of sequence
- Fault tolerance: Systems must be robust to failures and data loss
To address these challenges, specialized algorithms have been developed that can efficiently process streaming data while maintaining accuracy and scalability.
3. Key Algorithms for Streaming Data
Let’s dive into some of the most important algorithms used for handling streaming data. These algorithms are designed to provide approximate solutions to various problems while using limited memory and processing each data point only once.
4. Reservoir Sampling
Reservoir sampling is a family of randomized algorithms for selecting a random sample of k items from a list of n items, where n is either a very large or unknown number. This is particularly useful when dealing with streaming data where the total number of items is not known in advance.
How It Works
- Create a “reservoir” array of size k and fill it with the first k items of the stream.
- For each subsequent item i (where i > k):
- Generate a random number j between 1 and i (inclusive).
- If j ≤ k, replace the j-th item in the reservoir with the i-th item from the stream.
This algorithm ensures that at any point, each item in the stream has an equal probability of being in the reservoir.
Implementation
Here’s a simple implementation of reservoir sampling in Python:
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if i < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Example usage
stream = range(1000000) # Simulating a large stream of data
sample = reservoir_sampling(stream, 10)
print(sample)
This implementation allows you to sample k items from a potentially infinite stream while maintaining a uniform distribution of samples.
5. Count-Min Sketch
The Count-Min Sketch is a probabilistic data structure used for summarizing streaming data. It’s particularly useful for estimating the frequency of items in a data stream using sub-linear space.
How It Works
- Initialize a 2D array of counters with d rows and w columns, all set to zero.
- Choose d hash functions, each mapping items to a column in its respective row.
- For each item in the stream:
- Apply each hash function to the item.
- Increment the corresponding counter in each row.
- To estimate the frequency of an item:
- Apply the hash functions to the item.
- Return the minimum value among the corresponding counters.
The Count-Min Sketch provides a frequency estimate that is always greater than or equal to the true frequency, with the error decreasing as more space is allocated.
Implementation
Here’s a basic implementation of a Count-Min Sketch in Python:
import mmh3 # MurmurHash3 implementation
class CountMinSketch:
def __init__(self, width, depth):
self.width = width
self.depth = depth
self.sketch = [[0] * width for _ in range(depth)]
def add(self, item, count=1):
for i in range(self.depth):
column = mmh3.hash(item, i) % self.width
self.sketch[i][column] += count
def estimate(self, item):
return min(self.sketch[i][mmh3.hash(item, i) % self.width]
for i in range(self.depth))
# Example usage
cms = CountMinSketch(width=1000, depth=5)
stream = ['apple', 'banana', 'apple', 'cherry', 'banana', 'date', 'apple']
for item in stream:
cms.add(item)
print(cms.estimate('apple')) # Should be close to 3
print(cms.estimate('banana')) # Should be close to 2
print(cms.estimate('cherry')) # Should be close to 1
This implementation uses the MurmurHash3 algorithm for hashing, which provides good distribution and speed. The width and depth parameters control the trade-off between space usage and estimation accuracy.
6. HyperLogLog
HyperLogLog is an algorithm used for estimating the number of distinct elements (cardinality) in a multiset. It’s particularly useful when dealing with very large datasets where storing all unique elements is impractical.
How It Works
- Initialize m registers, each set to -1.
- For each item in the stream:
- Hash the item to get a binary string.
- Find the index of the leftmost 1-bit in the hash (let’s call it r).
- Update the corresponding register with max(current value, r).
- To estimate cardinality:
- Calculate the harmonic mean of 2^r for all registers.
- Apply bias correction.
HyperLogLog provides an estimate with a typical error rate of about 2%, using much less memory than would be required to store all unique elements.
Implementation
Here’s a simplified implementation of HyperLogLog in Python:
import mmh3
class HyperLogLog:
def __init__(self, p):
self.p = p
self.m = 1 << p
self.registers = [0] * self.m
self.alpha = self._get_alpha()
def _get_alpha(self):
if self.p == 4:
return 0.673
elif self.p == 5:
return 0.697
elif self.p == 6:
return 0.709
else:
return 0.7213 / (1 + 1.079 / self.m)
def add(self, item):
x = mmh3.hash(item)
j = x & (self.m - 1)
w = x >> self.p
self.registers[j] = max(self.registers[j], self._rho(w))
def _rho(self, w):
return len(bin(w)) - 3
def estimate(self):
Z = sum(2 ** -r for r in self.registers)
E = self.alpha * self.m * self.m / Z
return int(E)
# Example usage
hll = HyperLogLog(p=14) # 2^14 registers
stream = ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig', 'grape']
for item in stream:
hll.add(item)
print(f"Estimated cardinality: {hll.estimate()}")
print(f"Actual cardinality: {len(set(stream))}")
This implementation uses 2^14 registers. The choice of p (determining the number of registers) affects the accuracy and memory usage of the algorithm.
7. Bloom Filters
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set. It can have false positives but no false negatives, making it useful for many applications in streaming data processing.
How It Works
- Initialize a bit array of m bits, all set to 0.
- Choose k different hash functions.
- To add an element:
- Feed it to each of the k hash functions.
- Set the bits at the resulting k positions to 1.
- To query for an element:
- Feed it to each of the k hash functions.
- If any of the bits at the resulting positions is 0, the element is not in the set.
- If all are 1, the element is probably in the set.
Bloom filters are particularly useful when you need to quickly check if an item has been seen before in a stream, with a small probability of false positives.
Implementation
Here’s a simple implementation of a Bloom filter in Python:
import mmh3
class BloomFilter:
def __init__(self, size, hash_count):
self.size = size
self.hash_count = hash_count
self.bit_array = [0] * size
def add(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
self.bit_array[index] = 1
def check(self, item):
for seed in range(self.hash_count):
index = mmh3.hash(item, seed) % self.size
if self.bit_array[index] == 0:
return False
return True
# Example usage
bf = BloomFilter(size=100, hash_count=3)
stream = ['apple', 'banana', 'cherry', 'date', 'elderberry']
for item in stream:
bf.add(item)
print(bf.check('apple')) # Should be True
print(bf.check('banana')) # Should be True
print(bf.check('grape')) # Should be False (probably)
This implementation uses MurmurHash3 with different seed values to simulate multiple hash functions. The size and hash_count parameters affect the trade-off between memory usage and false positive rate.
8. Sliding Window Algorithms
Sliding window algorithms are a class of techniques used to process streaming data over a fixed-size “window” of recent elements. These algorithms are particularly useful for analyzing trends, detecting patterns, or computing statistics over the most recent data points.
Types of Sliding Windows
- Fixed-size window: Maintains a fixed number of most recent elements.
- Time-based window: Keeps elements within a specific time range (e.g., last 5 minutes).
- Landmark window: Starts at a fixed point and grows indefinitely.
- Tumbling window: Non-overlapping fixed-size windows.
Implementation
Here’s an example of a simple fixed-size sliding window algorithm for computing the moving average:
from collections import deque
class MovingAverage:
def __init__(self, window_size):
self.window = deque(maxlen=window_size)
self.window_size = window_size
self.sum = 0
def next(self, val):
if len(self.window) == self.window_size:
self.sum -= self.window[0]
self.window.append(val)
self.sum += val
return self.sum / len(self.window)
# Example usage
ma = MovingAverage(3)
stream = [1, 10, 3, 5, 2, 6]
for value in stream:
print(f"Current value: {value}, Moving average: {ma.next(value)}")
This implementation efficiently maintains a moving average over a fixed-size window, updating in O(1) time for each new element.
9. Hoeffding Trees
Hoeffding Trees, also known as Very Fast Decision Trees (VFDT), are a class of decision tree algorithms designed for streaming data. They allow for incremental learning and can make split decisions based on a small subset of the data, making them suitable for high-speed data streams.
How It Works
- Start with a single leaf node (the root).
- For each incoming instance:
- Sort it to a leaf.
- Update sufficient statistics at the leaf.
- If the leaf has seen enough instances:
- Evaluate possible split attributes.
- Use the Hoeffding bound to decide if the best attribute is significantly better.
- If yes, split on that attribute.
The Hoeffding bound provides a statistical guarantee on the quality of the split decision, allowing the tree to grow incrementally with high confidence.
Implementation
Implementing a full Hoeffding Tree is complex, but here’s a simplified example to illustrate the concept:
import math
from collections import Counter
class HoeffdingTreeNode:
def __init__(self, attribute=None):
self.attribute = attribute
self.children = {}
self.class_counts = Counter()
self.attribute_counts = {}
def update(self, instance, label):
self.class_counts[label] += 1
for attr, value in instance.items():
if attr not in self.attribute_counts:
self.attribute_counts[attr] = Counter()
self.attribute_counts[attr][value] += 1
def should_split(self, n, delta):
# Simplified split decision based on information gain
if len(self.attribute_counts) < 2:
return False
total = sum(self.class_counts.values())
class_entropy = self._entropy(self.class_counts.values())
best_attr = None
best_gain = 0
second_best_gain = 0
for attr, counts in self.attribute_counts.items():
attr_entropy = sum((sum(v.values()) / total) * self._entropy(v.values()) for v in counts.values())
gain = class_entropy - attr_entropy
if gain > best_gain:
second_best_gain = best_gain
best_gain = gain
best_attr = attr
elif gain > second_best_gain:
second_best_gain = gain
hoeffding_bound = math.sqrt(math.log(1/delta) / (2 * n))
return best_gain - second_best_gain > hoeffding_bound, best_attr
def _entropy(self, counts):
total = sum(counts)
return -sum((c/total) * math.log2(c/total) for c in counts if c > 0)
class HoeffdingTree:
def __init__(self, delta=0.01):
self.root = HoeffdingTreeNode()
self.delta = delta
self.n_samples = 0
def update(self, instance, label):
self.n_samples += 1
node = self.root
while True:
node.update(instance, label)
should_split, best_attr = node.should_split(self.n_samples, self.delta)
if should_split:
if not node.children:
node.attribute = best_attr
for value in node.attribute_counts[best_attr]:
node.children[value] = HoeffdingTreeNode()
if not node.children:
break
node = node.children[instance[node.attribute]]
def predict(self, instance):
node = self.root
while node.children:
node = node.children[instance[node.attribute]]
return node.class_counts.most_common(1)[0][0]
# Example usage
ht = HoeffdingTree()
stream = [
({'color': 'red', 'shape': 'circle'}, 'fruit'),
({'color': 'yellow', 'shape': 'circle'}, 'fruit'),
({'color': 'green', 'shape': 'rectangle'}, 'vegetable'),
({'color': 'red', 'shape': 'circle'}, 'fruit'),
({'color': 'green', 'shape': 'rectangle'}, 'vegetable'),
]
for instance, label in stream:
ht.update(instance, label)
print(ht.predict({'color': 'red', 'shape': 'circle'})) # Should predict 'fruit'
print(ht.predict({'color': 'green', 'shape': 'rectangle'})) # Should predict 'vegetable'
This implementation is a simplified version of a Hoeffding Tree and doesn’t include all optimizations found in production-ready implementations. It demonstrates the basic concept of incremental learning and split decisions based on the Hoeffding bound.
10. Real-World Applications
Streaming data algorithms find applications in various domains:
- Network monitoring: Detecting anomalies, DDoS attacks, and traffic patterns
- Financial markets: Real-time trading algorithms, fraud detection
- Social media analysis: Trending topics, sentiment analysis
- IoT and sensor networks: Processing data from multiple sensors in real-time
- Log analysis: Monitoring system logs for errors or security breaches
- Recommendation systems: Updating user preferences in real-time
- Clickstream analysis: Understanding user behavior on websites
11. Implementing Streaming Algorithms
When implementing streaming algorithms in practice, consider the following tips:
- Choose the right algorithm: Select an algorithm that fits your specific problem and data characteristics.
- Optimize for performance: Use efficient data structures and algorithms to handle high-velocity data.
- Handle out-of-order data: Implement mechanisms to deal with late-arriving or out-of-sequence data points.
- Ensure fault tolerance: Design your system to be resilient to failures and data loss.
- Scale horizontally: Use distributed computing frameworks like Apache Flink or Apache Spark Streaming for large-scale data processing.
- Monitor and adapt: Implement monitoring and adjust your algorithms as data characteristics change over time.
- Consider approximate computing: Many streaming algorithms provide approximate results. Understand the trade-offs between accuracy and performance.
12. Conclusion
Algorithms for handling streaming data are crucial in today’s data-driven world. They enable us to process and analyze vast amounts of data in real-time, providing valuable insights and enabling quick decision-making. From simple techniques like reservoir sampling to more complex algorithms like Hoeffding Trees, these methods allow us to overcome the challenges posed by high-velocity, unbounded data streams.
As you continue your journey in coding education and programming skills development, mastering these streaming data algorithms will be invaluable. They not only prepare you for technical interviews at major tech companies but also equip you with the skills to tackle real-world big data problems.
Remember that the field of streaming data processing is continuously evolving. Stay curious, keep practicing, and always be on the lookout for new algorithms and techniques. With a solid understanding of these foundational concepts, you’ll be well-prepared to handle the challenges of real-time data processing in your future projects and career.