An Introduction to A* Pathfinding Algorithm
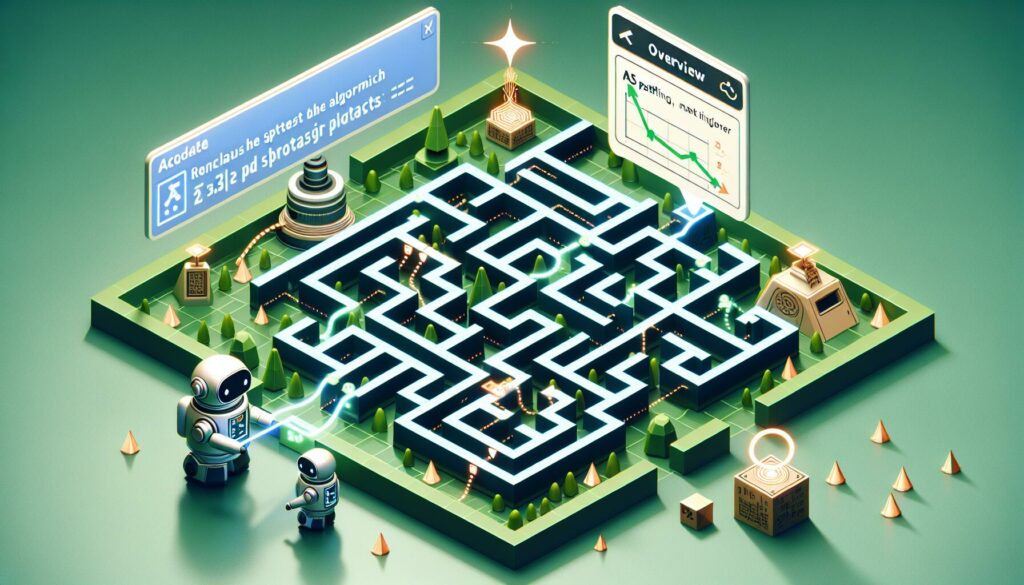
In the world of computer science and artificial intelligence, pathfinding algorithms play a crucial role in solving various problems, from navigating robots through complex environments to finding the shortest route in GPS systems. Among these algorithms, the A* (pronounced “A-star”) algorithm stands out as one of the most efficient and widely used pathfinding techniques. In this comprehensive guide, we’ll dive deep into the A* algorithm, exploring its principles, implementation, and applications.
What is the A* Algorithm?
The A* algorithm is a graph traversal and pathfinding algorithm that is commonly used in computer science due to its completeness, optimality, and efficiency. Developed as an extension of Edsger Dijkstra’s algorithm, A* was first described by Peter Hart, Nils Nilsson, and Bertram Raphael of Stanford Research Institute in 1968. It is an informed search algorithm, meaning it uses heuristic functions to guide its search and find the least-cost path from a given starting node to a goal node.
A* combines features of uniform-cost search and pure heuristic search to efficiently compute optimal paths. It uses a best-first search and finds a least-cost path from a given initial node to one goal node (out of one or more possible goals).
How Does A* Work?
The A* algorithm works by maintaining a tree of paths originating at the start node and extending those paths one edge at a time until its termination criterion is satisfied. At each iteration of its main loop, A* needs to determine which of its paths to extend. It does so based on the cost of the path and an estimate of the cost required to extend the path all the way to the goal.
Specifically, A* selects the path that minimizes:
f(n) = g(n) + h(n)
where:
n
is the next node on the pathg(n)
is the cost of the path from the start node ton
h(n)
is a heuristic function that estimates the cost of the cheapest path fromn
to the goal
The algorithm maintains two lists: an open list and a closed list. The open list contains all the nodes that are candidates for exploration, while the closed list contains the nodes that have already been explored.
The A* Algorithm Step by Step
Let’s break down the A* algorithm into steps:
- Initialize the open list with the starting node.
- Initialize the closed list as empty.
- While the open list is not empty:
- Find the node with the lowest f(n) on the open list, call it “q”.
- Pop q off the open list.
- Generate q’s 8 successors and set their parents to q.
- For each successor:
- If successor is the goal, stop search.
- Else, compute both g and h for successor.
- If a node with the same position as successor is in the open list which has a lower f than successor, skip this successor.
- If a node with the same position as successor is in the closed list which has a lower f than successor, skip this successor.
- Otherwise, add the node to the open list.
- Push q on the closed list.
Implementing A* in Python
Now that we understand the theory behind A*, let’s implement it in Python. We’ll create a simple grid-based pathfinding scenario.
import heapq
class Node:
def __init__(self, position, g=0, h=0, parent=None):
self.position = position
self.g = g
self.h = h
self.f = g + h
self.parent = parent
def __lt__(self, other):
return self.f < other.f
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def get_neighbors(grid, node):
neighbors = []
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
new_position = (node.position[0] + dx, node.position[1] + dy)
if 0 <= new_position[0] < len(grid) and 0 <= new_position[1] < len(grid[0]) and grid[new_position[0]][new_position[1]] == 0:
neighbors.append(new_position)
return neighbors
def astar(grid, start, goal):
start_node = Node(start, h=heuristic(start, goal))
open_list = [start_node]
closed_set = set()
while open_list:
current_node = heapq.heappop(open_list)
if current_node.position == goal:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
closed_set.add(current_node.position)
for neighbor_pos in get_neighbors(grid, current_node):
if neighbor_pos in closed_set:
continue
neighbor = Node(neighbor_pos,
g=current_node.g + 1,
h=heuristic(neighbor_pos, goal),
parent=current_node)
if neighbor not in open_list:
heapq.heappush(open_list, neighbor)
else:
idx = open_list.index(neighbor)
if open_list[idx].g > neighbor.g:
open_list[idx] = neighbor
heapq.heapify(open_list)
return None # No path found
# Example usage
grid = [
[0, 0, 0, 0, 1],
[1, 1, 0, 0, 0],
[0, 0, 0, 1, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
start = (0, 0)
goal = (4, 4)
path = astar(grid, start, goal)
print(path)
In this implementation, we use a grid where 0 represents walkable cells and 1 represents obstacles. The astar
function returns the optimal path from the start to the goal, if one exists.
Time and Space Complexity
The time complexity of A* depends on the heuristic function. In the worst case, the number of nodes expanded is exponential in the length of the solution (the shortest path), but it is polynomial when the search space is a tree, there is a single goal state, and the heuristic function h meets the following condition:
|h(x) - h*(x)| = O(log h*(x))
where h* is the optimal heuristic, the exact cost to get from x to the goal.
The space complexity of A* is roughly the same as its time complexity, as it needs to keep all generated nodes in memory.
Advantages and Disadvantages of A*
Like any algorithm, A* has its strengths and weaknesses:
Advantages:
- Optimal: A* is guaranteed to find the shortest path if one exists.
- Efficient: With a good heuristic, A* can be much faster than other pathfinding algorithms.
- Flexible: A* can be adapted to work with various types of graphs and heuristics.
Disadvantages:
- Memory intensive: A* stores all generated nodes in memory, which can be problematic for large search spaces.
- Heuristic dependent: The efficiency of A* heavily depends on the quality of the heuristic function.
- Not suitable for all scenarios: In some cases, simpler algorithms like Dijkstra’s might be more appropriate.
Applications of A*
A* has a wide range of applications in various fields:
- Video Games: A* is commonly used for pathfinding in video games, helping NPCs navigate game worlds.
- Robotics: A* can be used to plan paths for robots in both known and unknown environments.
- GPS and Navigation Systems: A* helps find the shortest route between two points on a map.
- Artificial Intelligence: A* is used in various AI applications, including puzzle-solving and strategic planning.
- Network Routing: A* can be applied to find optimal routes in computer networks.
Variations and Improvements of A*
Several variations of A* have been developed to address specific challenges or improve performance in certain scenarios:
1. Jump Point Search (JPS)
JPS is an optimization of A* for uniform-cost grids. It can significantly reduce the number of nodes expanded, especially in open areas.
2. Iterative Deepening A* (IDA*)
IDA* is a variant that uses less memory than A* by running a depth-first search to a limited depth, iteratively increasing this limit until a solution is found.
3. Weighted A*
This variant inflates the heuristic by a factor greater than 1, which can lead to faster but potentially suboptimal solutions.
4. Bidirectional A*
This approach runs two simultaneous searches: one forward from the start and one backward from the goal, potentially finding a solution faster.
Optimizing A* Performance
To get the best performance out of A*, consider the following tips:
- Choose an appropriate heuristic: The heuristic function should be admissible (never overestimate the cost) and as close to the actual cost as possible.
- Use efficient data structures: Implement the open list as a priority queue (heap) for faster operations.
- Prune unnecessary nodes: Implement techniques like Jump Point Search to reduce the number of nodes expanded.
- Optimize memory usage: Use memory-efficient data structures and consider variants like IDA* for large search spaces.
- Precompute data: If possible, precompute and store heuristic values or other relevant data to speed up runtime calculations.
Comparing A* with Other Pathfinding Algorithms
While A* is a powerful algorithm, it’s important to understand how it compares to other pathfinding algorithms:
Dijkstra’s Algorithm
Dijkstra’s algorithm is essentially A* with a heuristic function that always returns zero. It guarantees the shortest path but explores more nodes than A* in most cases.
Breadth-First Search (BFS)
BFS finds the shortest path in unweighted graphs but can be less efficient than A* in large or complex search spaces.
Depth-First Search (DFS)
DFS is memory-efficient but doesn’t guarantee the shortest path and can get stuck in infinite loops without proper precautions.
Best-First Search
Best-First Search is like A* but only considers the heuristic cost. It’s faster but doesn’t guarantee the optimal path.
Challenges in Implementing A*
While implementing A*, you might encounter several challenges:
- Choosing the right heuristic: The efficiency of A* heavily depends on the heuristic function. A poor heuristic can lead to suboptimal performance.
- Handling ties: When multiple paths have the same f-score, the choice of which to explore first can impact performance.
- Memory management: For large search spaces, managing memory usage can be challenging.
- Dealing with dynamic environments: If the environment changes during pathfinding, additional logic is needed to handle these changes.
- Balancing speed and optimality: Sometimes, you might need to sacrifice guarantees of optimality for faster performance, especially in real-time applications.
Conclusion
The A* algorithm is a powerful and flexible pathfinding tool that has stood the test of time since its introduction in 1968. Its ability to efficiently find optimal paths makes it invaluable in various fields, from video game development to robotics and beyond.
By understanding the principles behind A*, implementing it effectively, and knowing its strengths and limitations, you can leverage this algorithm to solve complex pathfinding problems in your own projects. Whether you’re developing a game, planning robot movements, or optimizing network routes, A* provides a solid foundation for tackling these challenges.
As you continue to explore algorithms and data structures, remember that A* is just one tool in a vast toolkit. The key to becoming a proficient programmer and problem solver is to understand when and how to apply different algorithms to various scenarios. Keep practicing, experimenting, and learning, and you’ll be well on your way to mastering not just A*, but a wide range of algorithmic techniques.