Implementing Fenwick Trees for Efficient Range Queries
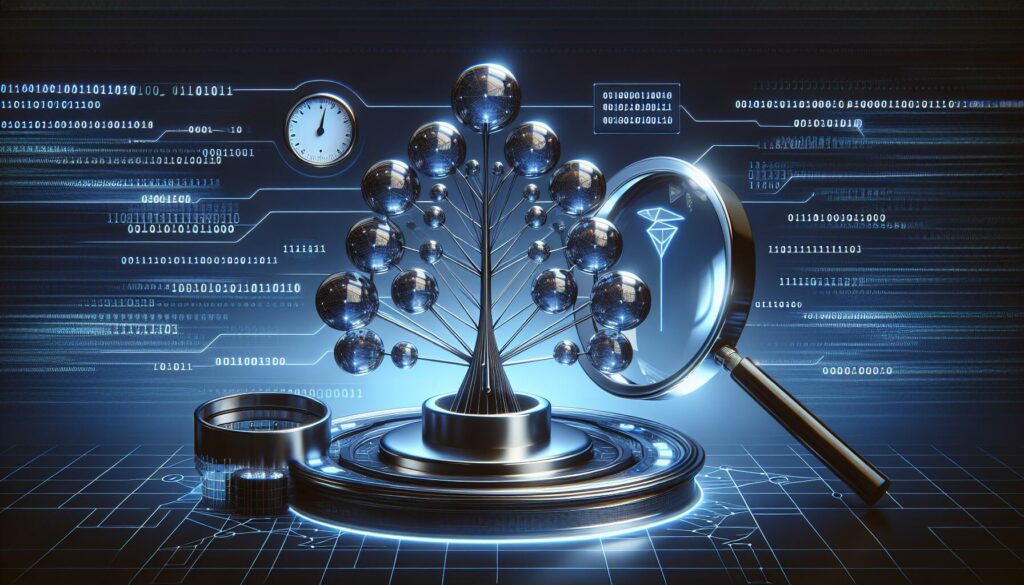
In the realm of competitive programming and advanced data structures, Fenwick Trees (also known as Binary Indexed Trees or BITs) stand out as a powerful tool for handling range queries and updates efficiently. This data structure, invented by Peter M. Fenwick in 1994, offers a clever way to perform cumulative frequency calculations and updates in logarithmic time complexity. In this comprehensive guide, we’ll dive deep into the world of Fenwick Trees, exploring their implementation, applications, and advantages over other data structures.
What are Fenwick Trees?
Fenwick Trees are a data structure designed to efficiently handle two types of operations on an array of numbers:
- Update the value at a specific index
- Calculate the sum of a range of elements
Unlike traditional arrays where these operations would take O(1) and O(n) time respectively, Fenwick Trees allow both operations to be performed in O(log n) time, where n is the size of the array. This significant improvement in time complexity makes Fenwick Trees invaluable for scenarios involving frequent updates and range sum queries.
The Intuition Behind Fenwick Trees
To understand Fenwick Trees, it’s helpful to visualize the array as a binary representation. Each index in the Fenwick Tree is responsible for storing the sum of a certain range of elements. The key insight is that we can represent any index as a sum of powers of 2, and use this property to efficiently store and retrieve partial sums.
For example, let’s consider an 8-element array:
Index: 1 2 3 4 5 6 7 8
Value: 3 2 4 5 1 1 3 3
In a Fenwick Tree representation:
- Index 1 (001 in binary) stores the sum of element 1
- Index 2 (010 in binary) stores the sum of elements 1-2
- Index 4 (100 in binary) stores the sum of elements 1-4
- Index 6 (110 in binary) stores the sum of elements 5-6
- Index 8 (1000 in binary) stores the sum of elements 1-8
This clever arrangement allows us to compute any range sum by combining these partial sums efficiently.
Implementing a Fenwick Tree in C++
Let’s implement a basic Fenwick Tree class in C++. We’ll start with the core structure and then add methods for updating values and querying range sums.
#include <vector>
class FenwickTree {
private:
std::vector<int> tree;
int n;
public:
FenwickTree(int size) : n(size + 1) {
tree.resize(n, 0);
}
// Update value at index i
void update(int i, int delta) {
while (i < n) {
tree[i] += delta;
i += i & (-i); // Add the least significant bit
}
}
// Get sum of range [1, i]
int sum(int i) {
int result = 0;
while (i > 0) {
result += tree[i];
i -= i & (-i); // Remove the least significant bit
}
return result;
}
// Get sum of range [left, right]
int rangeSum(int left, int right) {
return sum(right) - sum(left - 1);
}
};
Let’s break down the key components of this implementation:
1. Constructor
The constructor initializes the Fenwick Tree with a given size. We add 1 to the size because Fenwick Trees are typically 1-indexed for simplicity in calculations.
2. Update Method
The update
method is used to modify the value at a specific index. It updates all the relevant partial sums in the tree. The key operation here is i += i & (-i)
, which adds the least significant bit of i to itself. This operation effectively moves to the next index that needs to be updated in the tree.
3. Sum Method
The sum
method calculates the cumulative sum from index 1 to the given index. It traverses the tree, accumulating partial sums. The operation i -= i & (-i)
removes the least significant bit of i, moving to the next relevant partial sum in the tree.
4. Range Sum Method
The rangeSum
method calculates the sum of elements in a given range [left, right]. It does this by subtracting the sum up to left - 1
from the sum up to right
.
Using the Fenwick Tree
Now that we have implemented our Fenwick Tree, let’s see how to use it in practice:
#include <iostream>
int main() {
FenwickTree ft(8);
// Initialize the tree with values
ft.update(1, 3);
ft.update(2, 2);
ft.update(3, 4);
ft.update(4, 5);
ft.update(5, 1);
ft.update(6, 1);
ft.update(7, 3);
ft.update(8, 3);
// Query range sums
std::cout << "Sum of range [1, 5]: " << ft.rangeSum(1, 5) << std::endl;
std::cout << "Sum of range [3, 7]: " << ft.rangeSum(3, 7) << std::endl;
// Update a value
ft.update(4, 2); // Add 2 to the value at index 4
// Query again after update
std::cout << "Sum of range [1, 5] after update: " << ft.rangeSum(1, 5) << std::endl;
return 0;
}
This example demonstrates how to initialize a Fenwick Tree, perform range sum queries, and update values. The output would be:
Sum of range [1, 5]: 15
Sum of range [3, 7]: 14
Sum of range [1, 5] after update: 17
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of Fenwick Trees:
Time Complexity:
- Update Operation: O(log n)
- Sum Query: O(log n)
- Range Sum Query: O(log n)
Both update and query operations involve traversing the tree by manipulating the least significant bit, which takes logarithmic time in the worst case.
Space Complexity:
- Storage: O(n)
The Fenwick Tree uses a single array of size n to store all partial sums, resulting in linear space complexity.
Advantages of Fenwick Trees
Fenwick Trees offer several advantages over other data structures:
- Efficiency: Both updates and queries are performed in O(log n) time, making them suitable for scenarios with frequent updates and queries.
- Simplicity: Fenwick Trees are relatively simple to implement compared to more complex structures like Segment Trees.
- Memory Efficiency: They use less memory than Segment Trees, as they only require a single array.
- Versatility: While primarily used for range sum queries, Fenwick Trees can be adapted for other range operations like minimum, maximum, or XOR.
Applications of Fenwick Trees
Fenwick Trees find applications in various areas of computer science and competitive programming:
- Cumulative Frequency Tables: Efficiently maintain and query cumulative frequency distributions.
- Range Sum Queries in Databases: Optimize range sum calculations in large datasets.
- Computational Geometry: Solve problems involving counting points in 2D space.
- Inverted Index in Information Retrieval: Efficiently update and query document frequencies in search engines.
- Dynamic Programming Optimizations: Speed up certain DP algorithms that require range sum calculations.
Advanced Techniques with Fenwick Trees
While we’ve covered the basics, there are several advanced techniques and variations of Fenwick Trees worth exploring:
1. 2D Fenwick Trees
Fenwick Trees can be extended to two dimensions, allowing for efficient updates and queries on 2D grids. This is particularly useful in problems involving matrices or 2D coordinate systems.
2. Range Updates
With some modifications, Fenwick Trees can support range updates (updating a range of elements at once) while maintaining logarithmic time complexity for both updates and queries.
3. Fenwick Trees for Non-Commutative Operations
While typically used for sum operations, Fenwick Trees can be adapted for non-commutative operations like matrix multiplication, opening up new possibilities for range queries.
4. Persistent Fenwick Trees
By incorporating persistence techniques, we can create versions of Fenwick Trees that allow querying previous states, which is useful in certain algorithmic problems.
Comparison with Other Data Structures
To fully appreciate Fenwick Trees, it’s worth comparing them to other data structures used for similar purposes:
Fenwick Trees vs. Segment Trees
- Complexity: Both have O(log n) time for updates and queries.
- Memory: Fenwick Trees use less memory (n elements vs. 4n for Segment Trees).
- Flexibility: Segment Trees are more flexible and can handle a wider range of operations.
- Implementation: Fenwick Trees are generally simpler to implement.
Fenwick Trees vs. Square Root Decomposition
- Complexity: Fenwick Trees offer better time complexity (O(log n) vs. O(√n)).
- Simplicity: Square Root Decomposition is conceptually simpler for some problems.
- Flexibility: Square Root Decomposition can be more flexible for certain types of problems.
Common Pitfalls and Best Practices
When working with Fenwick Trees, keep these points in mind:
- 1-Indexing: Fenwick Trees typically use 1-based indexing. Be careful when converting between 0-based and 1-based indices.
- Initialization: Ensure the tree is properly initialized before performing operations.
- Range Limits: Be mindful of the valid range for queries and updates (1 to n).
- Integer Overflow: For large sums, consider using long long instead of int to prevent overflow.
- Memory Management: In languages without automatic memory management, ensure proper allocation and deallocation of memory.
Practice Problems
To solidify your understanding of Fenwick Trees, try solving these problems:
Conclusion
Fenwick Trees are a powerful and efficient data structure for handling range queries and updates. Their simplicity, coupled with logarithmic time complexity for operations, makes them an invaluable tool in a programmer’s arsenal. Whether you’re tackling competitive programming challenges or optimizing real-world applications, understanding and implementing Fenwick Trees can significantly enhance your problem-solving capabilities.
As you continue to explore advanced data structures and algorithms, remember that mastering tools like Fenwick Trees not only improves your coding skills but also sharpens your ability to think critically about efficiency and optimization in software development. Keep practicing, exploring variations, and applying Fenwick Trees to diverse problems to fully grasp their potential and limitations.
Happy coding, and may your range queries always be swift and efficient!