Exploring the Bellman-Ford Algorithm: A Comprehensive Guide
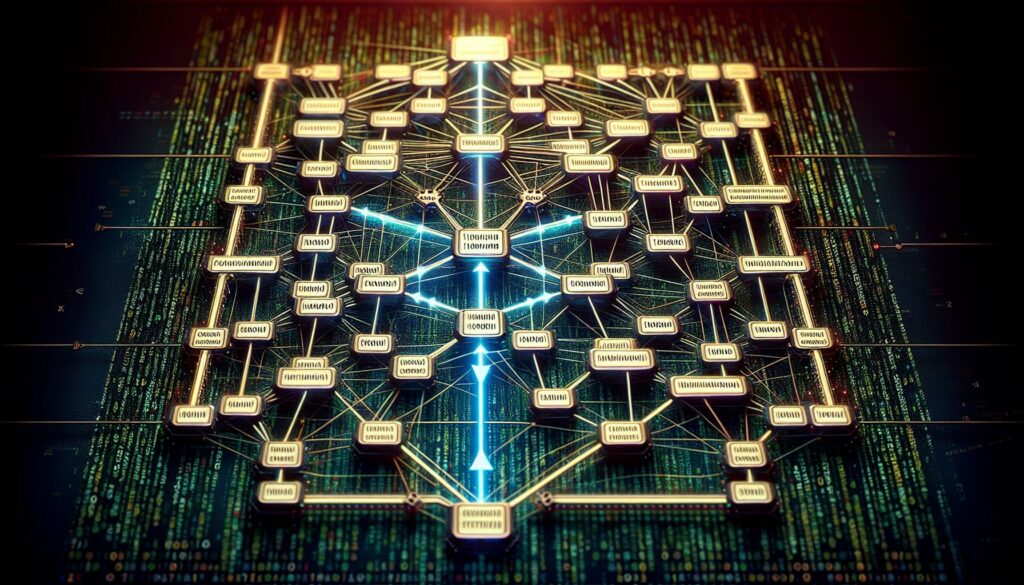
In the world of computer science and graph theory, the Bellman-Ford algorithm stands as a fundamental tool for solving the single-source shortest path problem. Named after its creators, Richard Bellman and Lester Ford Jr., this algorithm has proven to be an essential component in various applications, from network routing to computational biology. In this comprehensive guide, we’ll dive deep into the Bellman-Ford algorithm, exploring its mechanics, implementation, and real-world applications.
Table of Contents
- Introduction to the Bellman-Ford Algorithm
- How the Bellman-Ford Algorithm Works
- Implementing the Bellman-Ford Algorithm
- Time Complexity Analysis
- Comparison with Dijkstra’s Algorithm
- Real-world Applications
- Optimizations and Variations
- Practice Problems and Exercises
- Conclusion
1. Introduction to the Bellman-Ford Algorithm
The Bellman-Ford algorithm is a graph algorithm used to find the shortest paths from a single source vertex to all other vertices in a weighted graph. Unlike Dijkstra’s algorithm, which only works on graphs with non-negative edge weights, the Bellman-Ford algorithm can handle graphs with negative edge weights. This makes it particularly useful in scenarios where negative weights are meaningful, such as in financial modeling or certain types of network flow problems.
Key features of the Bellman-Ford algorithm include:
- Ability to handle negative edge weights
- Detection of negative weight cycles
- Simplicity in implementation
- Guaranteed to find the shortest path if one exists
While the algorithm is less efficient than Dijkstra’s for graphs with non-negative weights, its ability to handle negative weights and detect negative cycles makes it an indispensable tool in certain scenarios.
2. How the Bellman-Ford Algorithm Works
The Bellman-Ford algorithm operates on a simple principle: it repeatedly relaxes all edges of the graph, gradually improving the shortest path estimates until it reaches the optimal solution. Here’s a step-by-step breakdown of how the algorithm works:
- Initialization: Set the distance to the source vertex to 0 and all other vertices to infinity.
- Relaxation: For each edge (u, v) with weight w, if the distance to v is greater than the distance to u plus w, update the distance to v.
- Iteration: Repeat the relaxation step for all edges V-1 times, where V is the number of vertices in the graph.
- Negative Cycle Detection: Perform one more round of relaxation. If any distance is updated, a negative weight cycle exists.
The key insight of the algorithm is that in a graph with V vertices, the shortest path between any two vertices can contain at most V-1 edges. Therefore, by relaxing all edges V-1 times, we guarantee that we’ve found the shortest path to all vertices (if no negative cycles exist).
3. Implementing the Bellman-Ford Algorithm
Let’s implement the Bellman-Ford algorithm in Python. We’ll use a simple graph representation where edges are stored as tuples (source, destination, weight) in a list.
def bellman_ford(graph, source, V):
# Step 1: Initialize distances
dist = [float('inf')] * V
dist[source] = 0
# Step 2: Relax all edges V-1 times
for _ in range(V - 1):
for u, v, w in graph:
if dist[u] != float('inf') and dist[u] + w < dist[v]:
dist[v] = dist[u] + w
# Step 3: Check for negative weight cycles
for u, v, w in graph:
if dist[u] != float('inf') and dist[u] + w < dist[v]:
print("Graph contains negative weight cycle")
return
return dist
# Example usage
graph = [
(0, 1, 4),
(0, 2, 3),
(1, 2, -1),
(1, 3, 2),
(2, 3, 5),
(3, 4, 2)
]
V = 5 # Number of vertices
source = 0
distances = bellman_ford(graph, source, V)
print("Distances from source:", distances)
This implementation follows the steps outlined earlier. It first initializes the distances, then performs V-1 iterations of edge relaxation, and finally checks for negative weight cycles.
4. Time Complexity Analysis
The time complexity of the Bellman-Ford algorithm is O(V * E), where V is the number of vertices and E is the number of edges in the graph. This is because:
- The main loop runs V-1 times
- In each iteration, we relax all E edges
In the worst case, where E is O(V^2) (in a dense graph), the time complexity becomes O(V^3). This makes the Bellman-Ford algorithm less efficient than Dijkstra’s algorithm for graphs with non-negative weights, which has a time complexity of O((V + E) log V) when implemented with a binary heap.
The space complexity of the algorithm is O(V), as we need to store the distance array for all vertices.
5. Comparison with Dijkstra’s Algorithm
While both the Bellman-Ford and Dijkstra’s algorithms solve the single-source shortest path problem, they have some key differences:
Feature | Bellman-Ford | Dijkstra’s |
---|---|---|
Negative edge weights | Can handle | Cannot handle |
Negative cycles | Can detect | Not applicable |
Time complexity | O(V * E) | O((V + E) log V) with binary heap |
Simplicity | Simpler to implement | More complex (requires priority queue) |
Dijkstra’s algorithm is generally preferred when all edge weights are non-negative due to its better time complexity. However, the Bellman-Ford algorithm is the go-to choice when dealing with graphs that may contain negative edge weights or when detecting negative cycles is necessary.
6. Real-world Applications
The Bellman-Ford algorithm finds applications in various real-world scenarios:
- Networking protocols: The algorithm is used in routing protocols like RIP (Routing Information Protocol) to find the best path for data packets.
- Forex arbitrage detection: In financial markets, it can be used to detect arbitrage opportunities in currency exchange rates.
- Traffic routing systems: It can be applied in GPS navigation systems to find the shortest path considering various factors like traffic and road conditions.
- Computational biology: The algorithm is used in sequence alignment problems and protein folding simulations.
- Game development: In pathfinding for non-player characters (NPCs) in games with complex terrain or varying movement costs.
7. Optimizations and Variations
While the basic Bellman-Ford algorithm is powerful, several optimizations and variations have been developed to improve its performance or adapt it to specific scenarios:
7.1 Early Termination
We can optimize the algorithm by adding an early termination condition. If no relaxation occurs in an iteration, we can stop the algorithm as no further improvements are possible.
def optimized_bellman_ford(graph, source, V):
dist = [float('inf')] * V
dist[source] = 0
for i in range(V - 1):
no_change = True
for u, v, w in graph:
if dist[u] != float('inf') and dist[u] + w < dist[v]:
dist[v] = dist[u] + w
no_change = False
if no_change:
break
# Negative cycle check remains the same
for u, v, w in graph:
if dist[u] != float('inf') and dist[u] + w < dist[v]:
print("Graph contains negative weight cycle")
return
return dist
7.2 SPFA (Shortest Path Faster Algorithm)
The SPFA is an optimization of the Bellman-Ford algorithm that uses a queue to only process vertices that have been updated in the previous iteration. This can significantly reduce the number of relaxations in practice, especially for sparse graphs.
from collections import deque
def spfa(graph, source, V):
dist = [float('inf')] * V
dist[source] = 0
in_queue = [False] * V
queue = deque([source])
in_queue[source] = True
while queue:
u = queue.popleft()
in_queue[u] = False
for v, w in graph[u]:
if dist[u] + w < dist[v]:
dist[v] = dist[u] + w
if not in_queue[v]:
queue.append(v)
in_queue[v] = True
return dist
7.3 Yen’s Improvement
Yen’s improvement is a modification that can detect negative cycles earlier in the process. It works by maintaining two distance arrays and comparing them after each iteration.
8. Practice Problems and Exercises
To solidify your understanding of the Bellman-Ford algorithm, try solving these practice problems:
- Basic Implementation: Implement the Bellman-Ford algorithm from scratch and test it on a small graph.
- Negative Cycle Detection: Create a graph with a negative cycle and modify your implementation to detect and report it.
- Path Reconstruction: Extend the algorithm to not only find the shortest distances but also reconstruct the shortest paths.
- Currency Arbitrage: Use the Bellman-Ford algorithm to detect arbitrage opportunities in a set of currency exchange rates.
- Optimized Implementation: Implement the SPFA variation and compare its performance with the basic Bellman-Ford algorithm on various graph types.
Here’s a sample problem to get you started:
def currency_arbitrage(exchange_rates):
# Convert exchange rates to negative logarithms
graph = [(-math.log(rate), i, j) for i, rates in enumerate(exchange_rates)
for j, rate in enumerate(rates) if i != j]
# Run Bellman-Ford
V = len(exchange_rates)
dist = [float('inf')] * V
dist[0] = 0
for _ in range(V - 1):
for w, u, v in graph:
if dist[u] + w < dist[v]:
dist[v] = dist[u] + w
# Check for arbitrage opportunity
for w, u, v in graph:
if dist[u] + w < dist[v]:
return True # Arbitrage exists
return False # No arbitrage
# Example usage
rates = [
[1, 0.23, 0.25, 16.43, 18.21, 4.94],
[4.34, 1, 1.11, 71.40, 79.09, 21.44],
[3.93, 0.90, 1, 64.52, 71.48, 19.37],
[0.061, 0.014, 0.015, 1, 1.11, 0.30],
[0.055, 0.013, 0.014, 0.90, 1, 0.27],
[0.20, 0.047, 0.052, 3.33, 3.69, 1]
]
print("Arbitrage opportunity exists:", currency_arbitrage(rates))
9. Conclusion
The Bellman-Ford algorithm is a powerful tool in the arsenal of any computer scientist or software engineer. Its ability to handle negative edge weights and detect negative cycles makes it invaluable in certain scenarios where other shortest path algorithms fall short. While it may not be the most efficient algorithm for all cases, understanding its mechanics and implementations is crucial for tackling a wide range of graph-related problems.
As you continue your journey in algorithm design and analysis, remember that the Bellman-Ford algorithm is just one piece of the puzzle. It’s often used in conjunction with other algorithms and data structures to solve complex real-world problems. Keep practicing, experimenting with different graph types, and exploring optimizations to deepen your understanding of this fundamental algorithm.
By mastering the Bellman-Ford algorithm and understanding its place in the broader context of graph algorithms, you’ll be well-equipped to tackle challenging problems in areas ranging from network design to financial modeling. Happy coding!