Understanding and Implementing Dijkstra’s Algorithm: A Comprehensive Guide
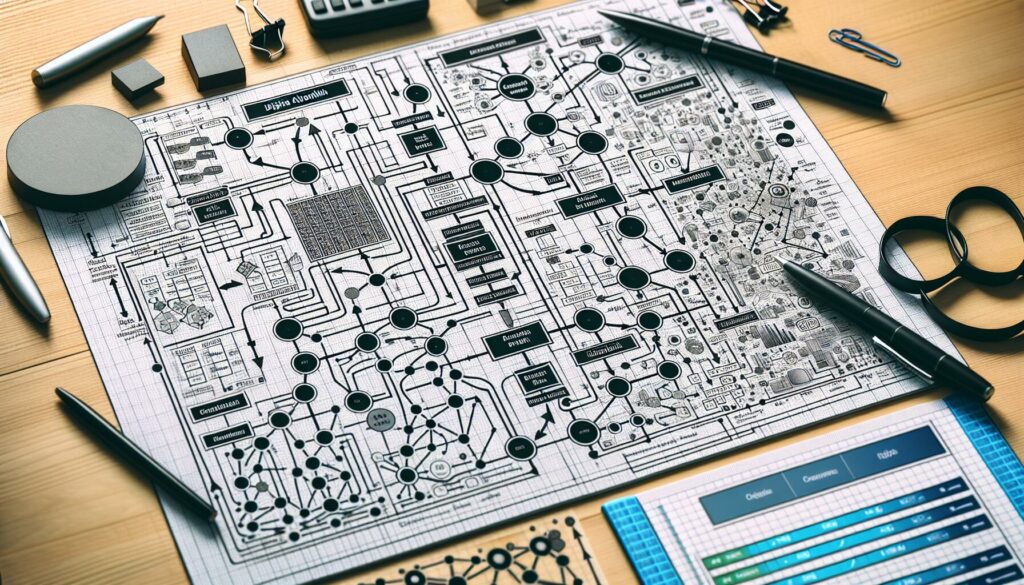
In the world of computer science and algorithms, Dijkstra’s algorithm stands out as a fundamental and powerful tool for solving shortest path problems in weighted graphs. Named after its creator, Dutch computer scientist Edsger W. Dijkstra, this algorithm has found applications in various fields, from network routing to GPS navigation systems. In this comprehensive guide, we’ll dive deep into understanding Dijkstra’s algorithm, its implementation, and its practical applications.
Table of Contents
- Introduction to Dijkstra’s Algorithm
- How Dijkstra’s Algorithm Works
- Implementing Dijkstra’s Algorithm
- Time Complexity Analysis
- Optimizations and Variations
- Real-world Applications
- Comparison with Other Shortest Path Algorithms
- Practice Problems and Exercises
- Conclusion
1. Introduction to Dijkstra’s Algorithm
Dijkstra’s algorithm is a graph search algorithm that solves the single-source shortest path problem for a graph with non-negative edge weights. It was conceived by computer scientist Edsger W. Dijkstra in 1956 and published three years later.
The algorithm finds the shortest path between a given start node and all other nodes in the graph, producing a shortest-path tree. This makes it ideal for navigation tasks, network routing protocols, and as a subroutine in other graph algorithms.
Key Concepts
- Weighted Graph: A graph where each edge has an associated weight or cost.
- Shortest Path: The path between two nodes with the minimum total weight.
- Relaxation: The process of updating the distance to a node if a shorter path is found.
2. How Dijkstra’s Algorithm Works
Dijkstra’s algorithm works on the principle of relaxation, progressively finding shorter paths from the start node to all other nodes in the graph. Here’s a step-by-step explanation of how the algorithm operates:
- Initialization:
- Set the distance to the start node to 0.
- Set the distance to all other nodes to infinity.
- Create a set of unvisited nodes containing all nodes in the graph.
- Selection: Select the unvisited node with the smallest tentative distance.
- Relaxation: For the current node, consider all of its unvisited neighbors. Calculate their tentative distances through the current node. If this calculated distance is less than the previously recorded tentative distance, update it.
- Marking: After considering all neighbors, mark the current node as visited and remove it from the unvisited set.
- Repeat: If the destination node has been marked visited or if the smallest tentative distance among the unvisited nodes is infinity, then stop. Otherwise, repeat from step 2.
This process ensures that the algorithm always selects the node with the smallest known distance to process first, and once a node is visited, its distance from the start node is final and minimal.
3. Implementing Dijkstra’s Algorithm
Let’s implement Dijkstra’s algorithm in Python. We’ll use a simple adjacency list representation for the graph and a priority queue to efficiently select the node with the smallest tentative distance.
import heapq
from typing import List, Tuple, Dict
def dijkstra(graph: Dict[int, List[Tuple[int, int]]], start: int) -> Dict[int, int]:
# Initialize distances and priority queue
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
# If we've found a longer path, skip
if current_distance > distances[current_node]:
continue
# Check all neighbors of the current node
for neighbor, weight in graph[current_node]:
distance = current_distance + weight
# If we've found a shorter path, update and add to queue
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
0: [(1, 4), (2, 1)],
1: [(3, 1)],
2: [(1, 2), (3, 5)],
3: [(4, 3)],
4: []
}
start_node = 0
shortest_distances = dijkstra(graph, start_node)
print(f"Shortest distances from node {start_node}: {shortest_distances}")
This implementation uses a min-heap (priority queue) to efficiently select the node with the smallest tentative distance. The heapq
module in Python provides an implementation of the heap queue algorithm.
Explanation of the Implementation
- We initialize all distances to infinity except for the start node, which is set to 0.
- We use a priority queue (
pq
) to store nodes and their tentative distances, always selecting the node with the smallest distance. - For each node, we explore its neighbors and update their distances if we find a shorter path.
- We continue this process until the priority queue is empty, meaning we’ve explored all reachable nodes.
4. Time Complexity Analysis
The time complexity of Dijkstra’s algorithm depends on the data structures used for its implementation. Let’s analyze the complexity for different implementations:
1. Basic Implementation (with array)
If we use an array to store the distances and perform a linear search to find the minimum distance node:
- Time Complexity: O(V^2), where V is the number of vertices
- This implementation is suitable for dense graphs
2. Binary Heap Implementation
Using a binary heap (priority queue) to efficiently find the minimum distance node:
- Time Complexity: O((V + E) log V), where E is the number of edges
- This is more efficient for sparse graphs
3. Fibonacci Heap Implementation
Using a Fibonacci heap can further improve the theoretical time complexity:
- Time Complexity: O(E + V log V)
- While theoretically faster, the constant factors and implementation complexity often make binary heaps more practical
The space complexity of Dijkstra’s algorithm is O(V), as we need to store the distance and visited status for each vertex.
5. Optimizations and Variations
While the basic Dijkstra’s algorithm is powerful, several optimizations and variations can improve its performance or adapt it to specific scenarios:
1. Bidirectional Search
For finding the shortest path between two specific nodes, we can run two simultaneous searches: one from the start node and one from the end node. This can significantly reduce the search space.
2. A* Algorithm
A* is a variation of Dijkstra’s algorithm that uses heuristics to guide the search towards the goal, often resulting in faster pathfinding for specific start and end nodes.
3. Delta Stepping
This is a parallel version of Dijkstra’s algorithm that can efficiently utilize multiple processors to speed up the computation for large graphs.
4. Pruning
If we’re only interested in paths up to a certain length, we can prune the search by not exploring paths that exceed this length.
5. Landmark-based Triangle Inequality
By precomputing distances to a set of landmark nodes, we can obtain lower bounds on distances between arbitrary nodes, potentially speeding up the search.
6. Real-world Applications
Dijkstra’s algorithm and its variations find applications in numerous real-world scenarios:
1. GPS Navigation Systems
Dijkstra’s algorithm is at the heart of many GPS navigation systems, helping find the shortest or fastest route between two locations.
2. Network Routing Protocols
Routing protocols like OSPF (Open Shortest Path First) use Dijkstra’s algorithm to determine the best path for data packets in computer networks.
3. Social Network Analysis
In social networks, Dijkstra’s algorithm can be used to find the shortest connection between two individuals.
4. Robotics
Path planning for robots often involves finding the shortest path while avoiding obstacles, a problem well-suited for Dijkstra’s algorithm.
5. Telecommunications
In telephone networks, Dijkstra’s algorithm can be used to find the cheapest way to route calls.
6. Biology
In biological networks, such as protein-protein interaction networks, Dijkstra’s algorithm can help analyze the relationships between different biological entities.
7. Comparison with Other Shortest Path Algorithms
While Dijkstra’s algorithm is widely used, it’s important to understand how it compares to other shortest path algorithms:
Dijkstra’s vs. Bellman-Ford
- Dijkstra’s Algorithm:
- Works only on graphs with non-negative edge weights
- More efficient for sparse graphs
- Time complexity: O((V + E) log V) with a binary heap
- Bellman-Ford Algorithm:
- Can handle graphs with negative edge weights
- Can detect negative cycles
- Time complexity: O(VE)
Dijkstra’s vs. Floyd-Warshall
- Dijkstra’s Algorithm:
- Solves single-source shortest path problem
- More efficient for sparse graphs
- Floyd-Warshall Algorithm:
- Solves all-pairs shortest path problem
- Works well for dense graphs
- Time complexity: O(V^3)
Dijkstra’s vs. A*
- Dijkstra’s Algorithm:
- Explores nodes in all directions
- Guarantees the shortest path
- A* Algorithm:
- Uses heuristics to guide the search towards the goal
- Often faster for specific start and end nodes
- May not always find the shortest path if the heuristic is not admissible
8. Practice Problems and Exercises
To solidify your understanding of Dijkstra’s algorithm, here are some practice problems and exercises:
1. Basic Implementation
Implement Dijkstra’s algorithm from scratch using an adjacency matrix representation of a graph.
2. Path Reconstruction
Modify the Dijkstra’s algorithm implementation to not only find the shortest distances but also reconstruct the actual shortest paths.
3. Network Routing
Given a network of routers and the latencies between them, use Dijkstra’s algorithm to find the path with the lowest total latency between two specific routers.
4. City Navigation
Implement a simple city navigation system using Dijkstra’s algorithm. Given a map of cities and roads with distances, find the shortest route between any two cities.
5. Optimize for Multiple Criteria
Modify Dijkstra’s algorithm to find the best path considering multiple criteria (e.g., distance and time) using a weighted sum approach.
6. Bidirectional Dijkstra
Implement a bidirectional version of Dijkstra’s algorithm and compare its performance with the standard version for finding paths between specific pairs of nodes.
7. Large Graph Performance
Generate a large random graph (e.g., 1 million nodes) and measure the performance of your Dijkstra’s implementation. Optimize it to handle such large graphs efficiently.
9. Conclusion
Dijkstra’s algorithm is a fundamental tool in the field of graph theory and algorithm design. Its elegant approach to finding shortest paths has made it an essential component in numerous applications, from everyday GPS navigation to complex network routing protocols.
By understanding the core principles of Dijkstra’s algorithm, its implementation details, and its various optimizations and applications, you’ve gained valuable insight into an algorithm that continues to play a crucial role in computer science and beyond.
As you continue your journey in algorithm design and problem-solving, remember that Dijkstra’s algorithm is just one piece of the puzzle. Its principles of greedy selection and progressive refinement can be found in many other algorithms and can inspire novel solutions to complex problems.
Keep practicing, exploring variations, and applying Dijkstra’s algorithm to diverse problems. With time and experience, you’ll develop an intuitive understanding of when and how to apply this powerful algorithm, and you’ll be well-equipped to tackle a wide range of graph-related challenges in your programming career.