Algorithms Behind Recommender Systems: Powering Personalized Experiences
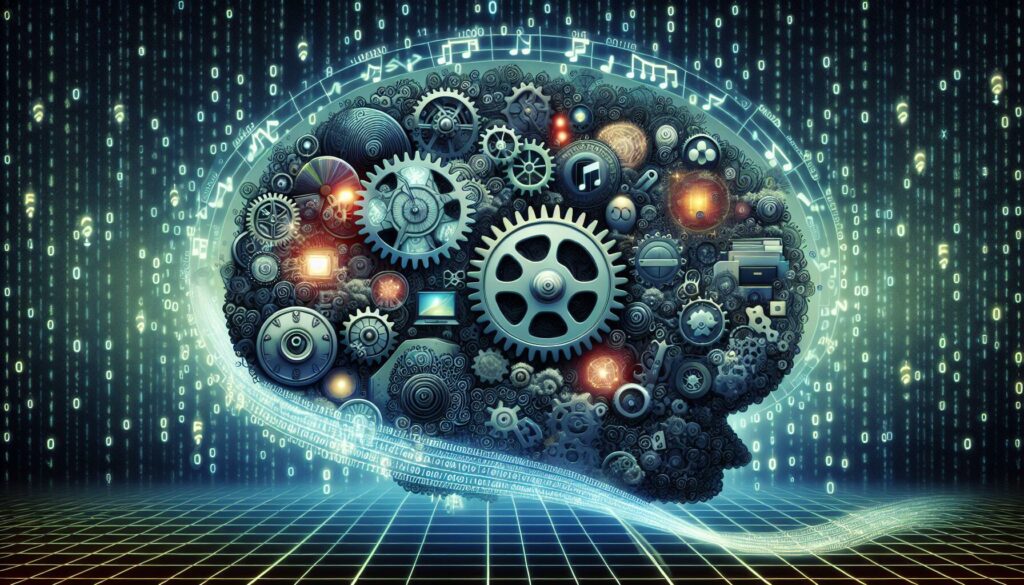
In today’s digital age, recommender systems have become an integral part of our online experiences. From Netflix suggesting your next binge-worthy series to Amazon recommending products you might like, these intelligent systems are working tirelessly behind the scenes to personalize our interactions with technology. But have you ever wondered about the algorithms that power these recommendation engines? In this comprehensive guide, we’ll dive deep into the world of recommender systems, exploring the key algorithms and techniques that make them tick.
Understanding Recommender Systems
Before we delve into the algorithms, let’s first understand what recommender systems are and why they’re so important in today’s digital landscape.
What are Recommender Systems?
Recommender systems are a subclass of information filtering systems that seek to predict the preferences or ratings a user would give to an item. These systems are designed to suggest relevant items to users based on their past behavior, preferences, and other factors.
Why are Recommender Systems Important?
Recommender systems play a crucial role in various domains:
- E-commerce: Helping users discover products they might be interested in
- Streaming services: Suggesting movies, TV shows, or music based on viewing/listening history
- Social media: Recommending content, friends, or groups to follow
- News aggregators: Personalizing news feeds based on reading habits
- Online advertising: Targeting ads to the most relevant audience
Now that we understand the importance of recommender systems, let’s explore the algorithms that make them work.
Key Algorithms in Recommender Systems
1. Collaborative Filtering
Collaborative Filtering (CF) is one of the most popular and widely used algorithms in recommender systems. It works on the principle that users who agreed in the past will likely agree in the future.
Types of Collaborative Filtering:
- User-Based Collaborative Filtering: This approach finds users with similar tastes and recommends items that these similar users have liked.
- Item-Based Collaborative Filtering: This method focuses on finding similar items based on user ratings and recommends these similar items.
Implementing User-Based Collaborative Filtering:
Here’s a simple example of how user-based collaborative filtering might be implemented in Python:
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
def user_based_cf(user_item_matrix, target_user, k=5):
# Calculate user similarity
user_similarity = cosine_similarity(user_item_matrix)
# Find k most similar users
similar_users = np.argsort(user_similarity[target_user])[-k-1:-1][::-1]
# Get items rated by similar users but not by target user
recommendations = []
for item in range(user_item_matrix.shape[1]):
if user_item_matrix[target_user][item] == 0: # Item not rated by target user
score = np.mean([user_item_matrix[user][item] for user in similar_users])
recommendations.append((item, score))
# Sort recommendations by score
recommendations.sort(key=lambda x: x[1], reverse=True)
return recommendations
# Example usage
user_item_matrix = np.array([
[4, 3, 0, 5, 0],
[5, 0, 4, 0, 2],
[3, 1, 2, 5, 0],
[0, 0, 0, 4, 4],
[1, 0, 3, 0, 5]
])
target_user = 0
recommendations = user_based_cf(user_item_matrix, target_user)
print(f"Recommendations for user {target_user}: {recommendations}")
2. Content-Based Filtering
Content-Based Filtering recommends items similar to those that a user has liked in the past. This approach analyzes the attributes of items to identify similarities.
Key Steps in Content-Based Filtering:
- Item Representation: Convert item attributes into feature vectors
- User Profile Creation: Build user profiles based on the items they’ve interacted with
- Similarity Calculation: Compute similarity between user profiles and item features
- Recommendation Generation: Recommend items with the highest similarity scores
Implementing Content-Based Filtering:
Here’s a simple example of content-based filtering using TF-IDF and cosine similarity:
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
def content_based_filtering(item_descriptions, user_profile, top_n=5):
# Create TF-IDF vectors for item descriptions
tfidf = TfidfVectorizer(stop_words='english')
item_vectors = tfidf.fit_transform(item_descriptions)
# Create user profile vector
user_vector = tfidf.transform([user_profile])
# Calculate similarity between user profile and items
similarities = cosine_similarity(user_vector, item_vectors).flatten()
# Get top N recommendations
top_indices = similarities.argsort()[-top_n:][::-1]
recommendations = [(i, similarities[i]) for i in top_indices]
return recommendations
# Example usage
item_descriptions = [
"Action movie with lots of explosions",
"Romantic comedy about a wedding",
"Sci-fi thriller set in space",
"Historical drama about World War II",
"Animated family movie with talking animals"
]
user_profile = "I like action movies and sci-fi thrillers"
recommendations = content_based_filtering(item_descriptions, user_profile)
print(f"Recommendations: {recommendations}")
3. Matrix Factorization
Matrix Factorization is a latent factor model that aims to decompose the user-item interaction matrix into two lower-dimensional matrices. This technique is particularly useful for handling large, sparse datasets.
Key Concepts in Matrix Factorization:
- User-Item Interaction Matrix: A matrix R where R[i][j] represents the rating of user i for item j
- Latent Factors: Hidden characteristics that influence user preferences and item attributes
- Factorization: Decomposing R into two matrices P (user factors) and Q (item factors)
Implementing Matrix Factorization:
Here’s a simple implementation of matrix factorization using gradient descent:
import numpy as np
def matrix_factorization(R, P, Q, K, steps=5000, alpha=0.0002, beta=0.02):
Q = Q.T
for step in range(steps):
for i in range(len(R)):
for j in range(len(R[i])):
if R[i][j] > 0:
eij = R[i][j] - np.dot(P[i,:], Q[:,j])
for k in range(K):
P[i][k] += alpha * (2 * eij * Q[k][j] - beta * P[i][k])
Q[k][j] += alpha * (2 * eij * P[i][k] - beta * Q[k][j])
e = 0
for i in range(len(R)):
for j in range(len(R[i])):
if R[i][j] > 0:
e += pow(R[i][j] - np.dot(P[i,:], Q[:,j]), 2)
for k in range(K):
e += (beta/2) * (pow(P[i][k], 2) + pow(Q[k][j], 2))
if e < 0.001:
break
return P, Q.T
# Example usage
R = np.array([
[5, 3, 0, 1],
[4, 0, 0, 1],
[1, 1, 0, 5],
[1, 0, 0, 4],
[0, 1, 5, 4],
])
N = len(R)
M = len(R[0])
K = 2
P = np.random.rand(N, K)
Q = np.random.rand(M, K)
nP, nQ = matrix_factorization(R, P, Q, K)
nR = np.dot(nP, nQ.T)
print("Original Matrix:")
print(R)
print("\nPredicted Matrix:")
print(nR)
4. Hybrid Approaches
Hybrid recommender systems combine multiple recommendation techniques to leverage the strengths of different approaches and mitigate their individual weaknesses.
Common Hybrid Strategies:
- Weighted Hybrid: Combine scores from multiple recommenders
- Switching Hybrid: Choose between different recommenders based on certain criteria
- Feature Combination: Use features from one technique as input to another
- Cascade Hybrid: Apply recommenders in a sequential manner, refining recommendations at each step
Implementing a Simple Weighted Hybrid:
Here’s an example of a weighted hybrid approach combining collaborative and content-based filtering:
def weighted_hybrid_recommender(user_id, item_id, collaborative_score, content_based_score, w1=0.7, w2=0.3):
return w1 * collaborative_score + w2 * content_based_score
# Example usage
collaborative_score = 0.8
content_based_score = 0.6
hybrid_score = weighted_hybrid_recommender(1, 1, collaborative_score, content_based_score)
print(f"Hybrid recommendation score: {hybrid_score}")
Advanced Techniques in Recommender Systems
1. Deep Learning for Recommender Systems
Deep learning has revolutionized many areas of machine learning, and recommender systems are no exception. Neural networks can capture complex patterns and non-linear relationships in user-item interactions.
Key Deep Learning Approaches:
- Neural Collaborative Filtering (NCF): Combines matrix factorization with neural networks
- Autoencoders: Learn compact representations of user-item interactions
- Recurrent Neural Networks (RNNs): Model sequential patterns in user behavior
- Graph Neural Networks (GNNs): Capture relationships in user-item interaction graphs
2. Context-Aware Recommender Systems
Context-aware recommender systems take into account additional contextual information, such as time, location, or user mood, to provide more relevant recommendations.
Key Aspects of Context-Aware Systems:
- Contextual Pre-filtering: Filter data based on context before applying traditional recommender algorithms
- Contextual Post-filtering: Apply context-based rules after generating recommendations
- Contextual Modeling: Incorporate context directly into the recommendation model
3. Reinforcement Learning for Recommendations
Reinforcement Learning (RL) approaches treat the recommendation process as a sequential decision-making problem, aiming to maximize long-term user satisfaction.
Key Concepts in RL for Recommendations:
- State: User’s current context and history
- Action: Recommending an item
- Reward: User’s feedback (e.g., clicks, ratings)
- Policy: Strategy for selecting recommendations
Challenges and Considerations in Recommender Systems
1. Cold Start Problem
The cold start problem occurs when the system lacks sufficient information about new users or items to make accurate recommendations.
Strategies to Address Cold Start:
- Content-based approaches for new items
- Demographic information for new users
- Hybrid methods combining multiple data sources
- Active learning techniques to gather initial preferences
2. Scalability
As the number of users and items grows, recommender systems must be able to handle large-scale data efficiently.
Approaches to Improve Scalability:
- Dimensionality reduction techniques
- Distributed computing frameworks (e.g., Apache Spark)
- Approximate nearest neighbor search algorithms
- Incremental learning and model updating
3. Privacy and Security
Recommender systems often rely on personal user data, raising concerns about privacy and data protection.
Privacy-Preserving Techniques:
- Federated learning
- Differential privacy
- Homomorphic encryption
- Local differential privacy
4. Diversity and Serendipity
Balancing accuracy with diversity and serendipity is crucial to avoid filter bubbles and provide a satisfying user experience.
Approaches to Enhance Diversity:
- Re-ranking algorithms
- Exploration-exploitation trade-offs
- Multi-objective optimization
- Diversity-aware evaluation metrics
Evaluation Metrics for Recommender Systems
Evaluating the performance of recommender systems is crucial for understanding their effectiveness and guiding improvements. Here are some common evaluation metrics:
1. Accuracy Metrics
- Mean Absolute Error (MAE): Measures the average absolute difference between predicted and actual ratings
- Root Mean Square Error (RMSE): Similar to MAE but gives higher weight to larger errors
- Precision: The fraction of recommended items that are relevant
- Recall: The fraction of relevant items that are recommended
- F1 Score: Harmonic mean of precision and recall
2. Ranking Metrics
- Mean Average Precision (MAP): Measures the quality of the ranking of recommended items
- Normalized Discounted Cumulative Gain (NDCG): Evaluates the ranking quality with emphasis on top-ranked items
- Mean Reciprocal Rank (MRR): Measures the rank of the first relevant item in the recommendation list
3. Diversity and Novelty Metrics
- Intra-List Diversity: Measures the diversity within a single recommendation list
- Coverage: The proportion of items that the system is able to recommend
- Novelty: The ability of the system to recommend items that are new or unexpected to the user
- Serendipity: The ability to make surprising and valuable recommendations
Implementing a Simple Recommender System
To bring everything together, let’s implement a simple hybrid recommender system that combines collaborative filtering and content-based filtering using Python and the Surprise library.
from surprise import Dataset, Reader, SVD
from surprise.model_selection import train_test_split
from surprise import accuracy
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.metrics.pairwise import cosine_similarity
import pandas as pd
import numpy as np
# Load movie data
movies_df = pd.read_csv('movies.csv')
ratings_df = pd.read_csv('ratings.csv')
# Prepare data for Surprise
reader = Reader(rating_scale=(1, 5))
data = Dataset.load_from_df(ratings_df[['userId', 'movieId', 'rating']], reader)
# Split the data
trainset, testset = train_test_split(data, test_size=0.25)
# Train SVD model (Collaborative Filtering)
svd = SVD()
svd.fit(trainset)
# Content-Based Filtering
tfidf = TfidfVectorizer(stop_words='english')
tfidf_matrix = tfidf.fit_transform(movies_df['genres'])
def get_content_based_recommendations(movie_id, top_n=10):
idx = movies_df.index[movies_df['movieId'] == movie_id].tolist()[0]
sim_scores = list(enumerate(cosine_similarity(tfidf_matrix[idx], tfidf_matrix)[0]))
sim_scores = sorted(sim_scores, key=lambda x: x[1], reverse=True)
sim_scores = sim_scores[1:top_n+1]
movie_indices = [i[0] for i in sim_scores]
return movies_df['movieId'].iloc[movie_indices].tolist()
# Hybrid Recommender
def hybrid_recommender(user_id, movie_id, w1=0.7, w2=0.3):
cf_score = svd.predict(user_id, movie_id).est
cb_recs = get_content_based_recommendations(movie_id)
cb_score = 1 if movie_id in cb_recs else 0
return w1 * cf_score + w2 * cb_score
# Example usage
user_id = 1
movie_id = 1
hybrid_score = hybrid_recommender(user_id, movie_id)
print(f"Hybrid recommendation score for user {user_id} and movie {movie_id}: {hybrid_score}")
# Evaluate the model
predictions = svd.test(testset)
rmse = accuracy.rmse(predictions)
mae = accuracy.mae(predictions)
print(f"RMSE: {rmse}")
print(f"MAE: {mae}")
Conclusion
Recommender systems have become an indispensable part of our digital experiences, helping us navigate the vast sea of information and choices available to us. From collaborative filtering to deep learning approaches, the field of recommender systems continues to evolve, driven by advancements in machine learning and the increasing availability of data.
As we’ve explored in this article, there’s no one-size-fits-all solution when it comes to building recommender systems. The choice of algorithm depends on various factors, including the nature of the data, the specific application domain, and the desired trade-offs between accuracy, scalability, and other considerations.
For developers and data scientists looking to implement recommender systems, it’s crucial to understand the strengths and limitations of different approaches and to continually evaluate and refine your models based on user feedback and performance metrics.
As recommender systems continue to play a vital role in shaping our online experiences, it’s exciting to imagine the future possibilities and innovations that lie ahead in this field. Whether you’re building the next big e-commerce platform or simply looking to enhance user engagement in your application, mastering the algorithms behind recommender systems is a valuable skill in today’s data-driven world.