Exploring Consistent Hashing in Distributed Systems
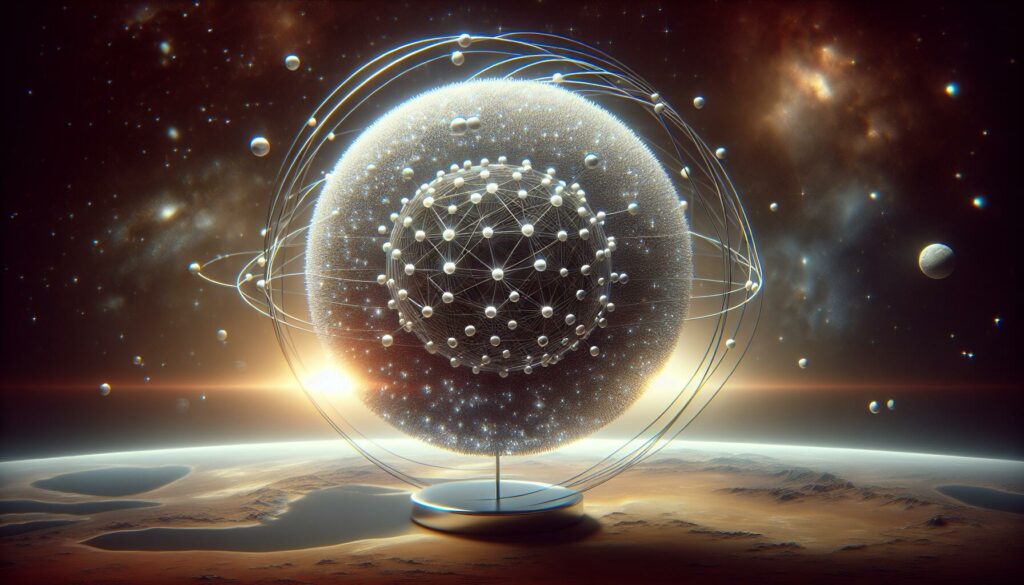
In the world of distributed systems and large-scale applications, efficient data distribution and load balancing are crucial for maintaining performance and reliability. One technique that has gained significant popularity in addressing these challenges is consistent hashing. This powerful algorithm plays a vital role in various distributed systems, including content delivery networks (CDNs), distributed caches, and distributed databases. In this comprehensive guide, we’ll dive deep into the concept of consistent hashing, explore its benefits, and examine its practical applications in modern distributed systems.
What is Consistent Hashing?
Consistent hashing is a distributed hashing scheme that provides a way to distribute data or requests across a cluster of nodes in a way that minimizes reorganization when nodes are added or removed. It was first introduced in 1997 by David Karger et al. in their paper “Consistent Hashing and Random Trees: Distributed Caching Protocols for Relieving Hot Spots on the World Wide Web”.
The primary goal of consistent hashing is to balance load across multiple nodes while maintaining a stable distribution when the number of nodes changes. This is particularly useful in scenarios where the set of nodes in a distributed system is dynamic, such as in cloud environments or peer-to-peer networks.
How Does Consistent Hashing Work?
To understand consistent hashing, let’s break down its key components and mechanisms:
1. Hash Space
Consistent hashing uses a circular hash space or “ring”. This space is typically represented by the range of a hash function, such as [0, 2^32 – 1] for a 32-bit hash function.
2. Mapping Nodes to the Hash Space
Each node in the distributed system is mapped to one or more points on this hash ring. This is done by hashing the node’s identifier (e.g., IP address or name) using the chosen hash function.
3. Mapping Keys to Nodes
When a key needs to be assigned to a node:
- The key is hashed using the same hash function.
- The resulting hash value determines a position on the ring.
- The key is assigned to the first node encountered when moving clockwise from this position on the ring.
4. Virtual Nodes
To improve load distribution, each physical node is often represented by multiple virtual nodes on the ring. This technique helps to spread the load more evenly across the system.
Implementing Consistent Hashing
Let’s look at a basic implementation of consistent hashing in Python to illustrate the concept:
import hashlib
class ConsistentHash:
def __init__(self, nodes=None, virtual_nodes=100):
self.virtual_nodes = virtual_nodes
self.ring = {}
self.sorted_keys = []
if nodes:
for node in nodes:
self.add_node(node)
def add_node(self, node):
for i in range(self.virtual_nodes):
key = self._hash(f"{node}:{i}")
self.ring[key] = node
self.sorted_keys.append(key)
self.sorted_keys.sort()
def remove_node(self, node):
for i in range(self.virtual_nodes):
key = self._hash(f"{node}:{i}")
del self.ring[key]
self.sorted_keys.remove(key)
def get_node(self, key):
if not self.ring:
return None
hash_key = self._hash(key)
for node_key in self.sorted_keys:
if node_key >= hash_key:
return self.ring[node_key]
return self.ring[self.sorted_keys[0]]
def _hash(self, key):
return hashlib.md5(key.encode()).hexdigest()
# Usage example
nodes = ["node1", "node2", "node3"]
ch = ConsistentHash(nodes)
print(ch.get_node("key1")) # Output: node2
print(ch.get_node("key2")) # Output: node1
print(ch.get_node("key3")) # Output: node3
ch.add_node("node4")
print(ch.get_node("key1")) # Output: node2 (unchanged)
ch.remove_node("node2")
print(ch.get_node("key1")) # Output: node4 (reassigned)
This implementation demonstrates the basic principles of consistent hashing, including the use of virtual nodes to improve distribution. It’s important to note that in a production environment, you’d want to use a more sophisticated implementation with additional features and optimizations.
Benefits of Consistent Hashing
Consistent hashing offers several advantages over traditional hash-based distribution methods:
1. Minimal Redistribution
When nodes are added or removed, only a small fraction of keys need to be remapped. This is in contrast to traditional modulo-based hashing, where a change in the number of nodes would require remapping almost all keys.
2. Scalability
Consistent hashing makes it easier to scale distributed systems horizontally by adding or removing nodes with minimal disruption.
3. Load Balancing
With the use of virtual nodes, consistent hashing can achieve a more uniform distribution of load across nodes.
4. Fault Tolerance
In the event of node failures, consistent hashing allows for graceful degradation by redistributing the load to the remaining nodes.
Applications of Consistent Hashing
Consistent hashing finds applications in various distributed systems and scenarios:
1. Distributed Caches
Systems like Memcached and Redis use consistent hashing to distribute cache entries across multiple cache servers. This allows for easy scaling and resilience to server failures.
2. Content Delivery Networks (CDNs)
CDNs use consistent hashing to determine which edge server should serve content for a particular request, ensuring efficient content distribution and load balancing.
3. Distributed Databases
NoSQL databases like Apache Cassandra and Amazon DynamoDB employ consistent hashing for data partitioning and load balancing across nodes.
4. Load Balancers
Consistent hashing can be used in load balancers to distribute incoming requests across multiple backend servers while maintaining session affinity.
Challenges and Considerations
While consistent hashing is a powerful technique, there are some challenges and considerations to keep in mind:
1. Hotspots
Even with virtual nodes, it’s possible for certain keys to become hotspots, leading to uneven load distribution. Techniques like bounded loads can help mitigate this issue.
2. Data Rebalancing
Although consistent hashing minimizes data movement when nodes are added or removed, some data still needs to be rebalanced. This process needs to be managed carefully in production systems.
3. Complexity
Implementing and maintaining a consistent hashing system can be more complex than simpler distribution methods. It’s important to weigh the benefits against the added complexity for your specific use case.
4. Hash Function Selection
The choice of hash function can impact the distribution quality. It’s crucial to select a hash function with good uniformity and performance characteristics.
Advanced Techniques and Variations
As consistent hashing has evolved, several advanced techniques and variations have been developed to address specific challenges or improve performance:
1. Jump Consistent Hash
Introduced by Google, Jump Consistent Hash is a fast, memory-efficient consistent hashing algorithm that doesn’t require a ring data structure. It’s particularly useful for systems with a large number of buckets.
def jump_consistent_hash(key, num_buckets):
b = -1
j = 0
while j < num_buckets:
b = j
key = ((key * 2862933555777941757) + 1) & 0xffffffffffffffff
j = int((b + 1) * (float(1 << 31) / float((key >> 33) + 1)))
return b
2. Rendezvous Hashing
Also known as Highest Random Weight (HRW) hashing, Rendezvous hashing is another approach to distributed hashing that achieves similar goals to consistent hashing but with a different mechanism.
3. Bounded Loads
This technique, introduced by Vimeo, aims to address the issue of load imbalance in consistent hashing by setting upper bounds on the load of each node and redirecting requests when these bounds are exceeded.
4. Consistent Hashing with Bounded Loads
This approach combines consistent hashing with the bounded loads technique to achieve better load distribution while maintaining the benefits of consistent hashing.
Implementing Consistent Hashing in Real-World Systems
When implementing consistent hashing in production systems, several factors need to be considered:
1. Performance Optimization
In high-throughput systems, the performance of the consistent hashing algorithm becomes critical. Techniques like caching hash calculations and using efficient data structures for the hash ring can significantly improve performance.
2. Monitoring and Rebalancing
Implementing a monitoring system to track the load distribution across nodes is crucial. When imbalances are detected, automated or manual rebalancing procedures may need to be triggered.
3. Fault Tolerance and Redundancy
In distributed systems, node failures are inevitable. Implementing redundancy through techniques like data replication and using backup nodes can enhance the system’s fault tolerance.
4. Consistency Models
Depending on the application, different consistency models may be required. Strong consistency might be necessary for certain use cases, while eventual consistency might be sufficient for others. The consistent hashing implementation should be designed to support the required consistency model.
Case Studies: Consistent Hashing in Action
Let’s explore how some well-known systems use consistent hashing:
1. Apache Cassandra
Cassandra, a distributed NoSQL database, uses consistent hashing to distribute data across the cluster. It employs a technique called virtual nodes (vnodes) to improve load distribution and make data rebalancing more efficient when nodes are added or removed.
2. Amazon DynamoDB
DynamoDB, Amazon’s managed NoSQL database service, uses consistent hashing for data partitioning. It automatically manages the distribution of data across multiple servers to provide seamless scalability.
3. Akamai Content Delivery Network
Akamai, one of the world’s largest CDN providers, uses consistent hashing to determine which edge server should serve content for a particular request. This allows for efficient content distribution and load balancing across their global network of servers.
4. Discord
The popular communication platform Discord uses consistent hashing in their Redis architecture to manage user sessions across multiple Redis instances. This allows them to scale their infrastructure efficiently while maintaining session consistency.
Future Trends and Research
As distributed systems continue to evolve and scale, research in consistent hashing and related techniques is ongoing. Some areas of active research and development include:
1. Machine Learning-Based Approaches
Researchers are exploring the use of machine learning techniques to optimize data distribution and load balancing in consistent hashing systems, potentially leading to more adaptive and efficient solutions.
2. Quantum-Resistant Hashing
With the advent of quantum computing, there’s growing interest in developing quantum-resistant hashing algorithms that can maintain the properties of consistent hashing in a post-quantum world.
3. Edge Computing Optimizations
As edge computing becomes more prevalent, there’s a need for consistent hashing techniques optimized for highly distributed and heterogeneous edge environments.
4. Integration with Blockchain Technologies
Researchers are exploring how consistent hashing can be applied in blockchain systems to improve scalability and data distribution in decentralized networks.
Conclusion
Consistent hashing has become an indispensable tool in the design and implementation of large-scale distributed systems. Its ability to provide efficient data distribution, load balancing, and scalability makes it a crucial technique for developers working on distributed caches, databases, content delivery networks, and other distributed applications.
As we’ve explored in this article, consistent hashing offers significant advantages over traditional hash-based distribution methods, particularly in dynamic environments where nodes are frequently added or removed. However, it’s important to understand its challenges and limitations, and to consider advanced techniques and optimizations when implementing consistent hashing in production systems.
The field of distributed systems continues to evolve, and with it, the techniques and applications of consistent hashing. As developers and system architects, staying informed about these developments and understanding how to effectively implement and optimize consistent hashing will be key to building robust, scalable, and efficient distributed systems in the future.
Whether you’re designing a new distributed system, optimizing an existing one, or preparing for technical interviews at major tech companies, a solid understanding of consistent hashing and its practical applications is invaluable. By mastering this powerful technique, you’ll be well-equipped to tackle the challenges of modern distributed computing and contribute to the next generation of scalable, resilient systems.