An Introduction to Synchronization Algorithms: Ensuring Harmony in Concurrent Systems
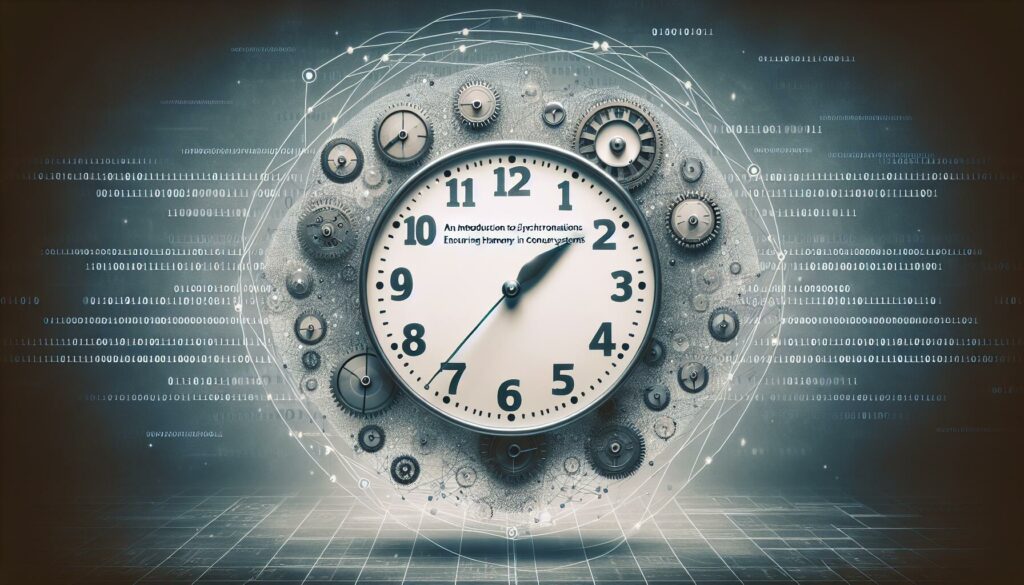
In the world of computer science and software engineering, synchronization algorithms play a crucial role in managing concurrent processes and ensuring the smooth operation of complex systems. As we dive deeper into the realm of multi-threaded applications and distributed computing, understanding these algorithms becomes increasingly important. This comprehensive guide will introduce you to the concept of synchronization algorithms, explore their significance, and delve into some of the most common implementations used in modern software development.
What are Synchronization Algorithms?
Synchronization algorithms are a set of techniques and protocols designed to coordinate the execution of multiple processes or threads in a concurrent computing environment. These algorithms aim to prevent race conditions, deadlocks, and other issues that can arise when multiple processes compete for shared resources or need to communicate with each other.
The primary goals of synchronization algorithms include:
- Ensuring mutual exclusion: Preventing multiple processes from simultaneously accessing shared resources
- Maintaining data consistency: Guaranteeing that shared data remains in a valid state
- Avoiding deadlocks: Preventing situations where processes are unable to proceed due to circular dependencies
- Facilitating inter-process communication: Enabling processes to exchange information and coordinate their actions
The Importance of Synchronization in Concurrent Systems
As modern computing systems become increasingly parallel and distributed, the need for effective synchronization mechanisms grows. Here are some key reasons why synchronization algorithms are crucial:
- Resource Management: In multi-threaded applications, multiple processes often need to access shared resources such as memory, files, or hardware devices. Synchronization algorithms ensure that these resources are accessed in an orderly manner, preventing conflicts and data corruption.
- Performance Optimization: Proper synchronization can help maximize system throughput by efficiently managing concurrent operations and minimizing unnecessary waiting times.
- Reliability: By preventing race conditions and maintaining data consistency, synchronization algorithms contribute to the overall reliability and correctness of software systems.
- Scalability: As systems grow in complexity and scale, synchronization becomes even more critical for maintaining performance and preventing bottlenecks.
- Distributed Systems: In distributed computing environments, synchronization algorithms play a vital role in coordinating actions across multiple nodes and ensuring consistency in distributed data stores.
Common Synchronization Primitives
Before diving into specific synchronization algorithms, it’s essential to understand some fundamental synchronization primitives that form the building blocks of more complex algorithms:
1. Mutex (Mutual Exclusion)
A mutex is a synchronization primitive that provides exclusive access to a shared resource. It ensures that only one thread can access the protected resource at a time.
// Pseudo-code for using a mutex
mutex.lock()
// Critical section (access shared resource)
mutex.unlock()
2. Semaphore
A semaphore is a synchronization primitive that can be used to control access to a set of resources. It maintains a count of available resources and allows threads to acquire or release them.
// Pseudo-code for using a semaphore
semaphore.wait() // Decrease the count
// Access the resource
semaphore.signal() // Increase the count
3. Monitor
A monitor is a high-level synchronization construct that combines mutual exclusion with the ability to wait for specific conditions. It encapsulates shared data and the operations that manipulate that data.
// Pseudo-code for a monitor
monitor ResourceManager {
// Shared data
// Methods to manipulate shared data
method1() {
// Implementation
}
method2() {
// Implementation
}
}
Classic Synchronization Algorithms
Now that we’ve covered the basic primitives, let’s explore some classic synchronization algorithms that are widely used in concurrent programming:
1. Producer-Consumer Problem
The Producer-Consumer problem is a classic synchronization scenario where one or more producer threads generate data items and add them to a shared buffer, while one or more consumer threads remove items from the buffer for processing.
// Pseudo-code for Producer-Consumer problem
class Buffer {
private Queue<Item> queue;
private int capacity;
private Semaphore full, empty, mutex;
public Buffer(int capacity) {
this.capacity = capacity;
queue = new Queue<>();
full = new Semaphore(0);
empty = new Semaphore(capacity);
mutex = new Semaphore(1);
}
public void produce(Item item) {
empty.wait();
mutex.wait();
queue.add(item);
mutex.signal();
full.signal();
}
public Item consume() {
full.wait();
mutex.wait();
Item item = queue.remove();
mutex.signal();
empty.signal();
return item;
}
}
2. Readers-Writers Problem
The Readers-Writers problem involves managing access to a shared resource that can be read by multiple threads simultaneously but must be written to by only one thread at a time.
// Pseudo-code for Readers-Writers problem
class ReadWriteLock {
private int readers = 0;
private boolean isWriting = false;
private Semaphore mutex = new Semaphore(1);
private Semaphore writeLock = new Semaphore(1);
public void acquireReadLock() {
mutex.wait();
readers++;
if (readers == 1) {
writeLock.wait();
}
mutex.signal();
}
public void releaseReadLock() {
mutex.wait();
readers--;
if (readers == 0) {
writeLock.signal();
}
mutex.signal();
}
public void acquireWriteLock() {
writeLock.wait();
isWriting = true;
}
public void releaseWriteLock() {
isWriting = false;
writeLock.signal();
}
}
3. Dining Philosophers Problem
The Dining Philosophers problem is a classic synchronization scenario that illustrates the challenges of resource allocation and deadlock prevention. It involves a group of philosophers sitting around a table, alternating between thinking and eating, with shared chopsticks between them.
// Pseudo-code for Dining Philosophers problem
class DiningPhilosophers {
private int numPhilosophers;
private Semaphore[] chopsticks;
public DiningPhilosophers(int numPhilosophers) {
this.numPhilosophers = numPhilosophers;
chopsticks = new Semaphore[numPhilosophers];
for (int i = 0; i
Advanced Synchronization Algorithms
As concurrent systems become more complex, advanced synchronization algorithms have been developed to address specific challenges and optimize performance. Let’s explore some of these algorithms:
1. Lock-Free Algorithms
Lock-free algorithms aim to provide thread-safe access to shared data structures without using traditional locks. These algorithms use atomic operations and careful design to ensure progress even in the presence of contention.
One example of a lock-free algorithm is the implementation of a lock-free queue:
// Pseudo-code for a lock-free queue
class LockFreeQueue<T> {
private AtomicReference<Node<T>> head, tail;
public LockFreeQueue() {
Node<T> dummy = new Node<>(null);
head = new AtomicReference<>(dummy);
tail = new AtomicReference<>(dummy);
}
public void enqueue(T item) {
Node<T> newNode = new Node<>(item);
while (true) {
Node<T> curTail = tail.get();
Node<T> tailNext = curTail.next.get();
if (curTail == tail.get()) {
if (tailNext != null) {
tail.compareAndSet(curTail, tailNext);
} else {
if (curTail.next.compareAndSet(null, newNode)) {
tail.compareAndSet(curTail, newNode);
return;
}
}
}
}
}
public T dequeue() {
while (true) {
Node<T> curHead = head.get();
Node<T> curTail = tail.get();
Node<T> headNext = curHead.next.get();
if (curHead == head.get()) {
if (curHead == curTail) {
if (headNext == null) {
return null;
}
tail.compareAndSet(curTail, headNext);
} else {
T value = headNext.value;
if (head.compareAndSet(curHead, headNext)) {
return value;
}
}
}
}
}
private static class Node<T> {
final T value;
final AtomicReference<Node<T>> next;
Node(T value) {
this.value = value;
this.next = new AtomicReference<>(null);
}
}
}
2. Read-Copy-Update (RCU)
Read-Copy-Update (RCU) is a synchronization mechanism that allows for efficient read-mostly workloads. It provides a way to read shared data without acquiring locks, while updates are made by creating new versions of the data.
Here’s a simplified example of RCU in action:
// Pseudo-code for RCU
class RCUProtectedData {
private AtomicReference<Data> dataRef;
public RCUProtectedData(Data initialData) {
dataRef = new AtomicReference<>(initialData);
}
public Data read() {
return dataRef.get();
}
public void update(Function<Data, Data> updateFunction) {
while (true) {
Data oldData = dataRef.get();
Data newData = updateFunction.apply(oldData);
if (dataRef.compareAndSet(oldData, newData)) {
break;
}
}
}
}
3. Transactional Memory
Transactional Memory is a concurrency control mechanism that allows programmers to define sections of code as transactions. These transactions are executed atomically and in isolation, simplifying the process of writing concurrent programs.
While hardware support for transactional memory is becoming more common, software transactional memory (STM) implementations are also available. Here’s a simplified example of how STM might be used:
// Pseudo-code for Software Transactional Memory
@Transactional
public void transferMoney(Account from, Account to, double amount) {
if (from.getBalance() >= amount) {
from.withdraw(amount);
to.deposit(amount);
}
}
Challenges and Considerations in Synchronization
While synchronization algorithms are essential for managing concurrent systems, they come with their own set of challenges and considerations:
1. Performance Overhead
Synchronization mechanisms often introduce performance overhead due to locking, context switching, and potential contention. Careful design and optimization are necessary to minimize this overhead.
2. Deadlocks and Livelocks
Improper use of synchronization primitives can lead to deadlocks (where processes are unable to proceed) or livelocks (where processes are actively executing but unable to make progress). Techniques such as lock ordering and timeout mechanisms can help mitigate these issues.
3. Scalability
As systems scale, synchronization can become a bottleneck. Techniques like fine-grained locking, lock-free algorithms, and distributed synchronization protocols are often employed to improve scalability.
4. Complexity
Implementing correct and efficient synchronization can be complex and error-prone. Tools like formal verification, model checking, and extensive testing are crucial for ensuring the correctness of synchronization algorithms.
5. Fairness
Ensuring fair access to shared resources can be challenging, especially in systems with varying priorities or workloads. Algorithms must be designed to prevent starvation and provide adequate responsiveness to all processes.
Conclusion
Synchronization algorithms are a fundamental aspect of concurrent and distributed systems, playing a crucial role in ensuring the correct and efficient operation of modern software. From basic primitives like mutexes and semaphores to advanced techniques like lock-free algorithms and transactional memory, the field of synchronization continues to evolve to meet the demands of increasingly complex and parallel computing environments.
As a software developer or computer science enthusiast, understanding these algorithms and their applications is essential for building robust, scalable, and high-performance systems. By mastering synchronization techniques, you’ll be better equipped to tackle the challenges of concurrent programming and contribute to the development of next-generation software systems.
Remember that while this introduction provides a solid foundation, the world of synchronization algorithms is vast and constantly evolving. Continued learning and practical experience will be key to mastering these concepts and applying them effectively in real-world scenarios.