Introduction to Monte Carlo Algorithms: Harnessing Randomness for Problem-Solving
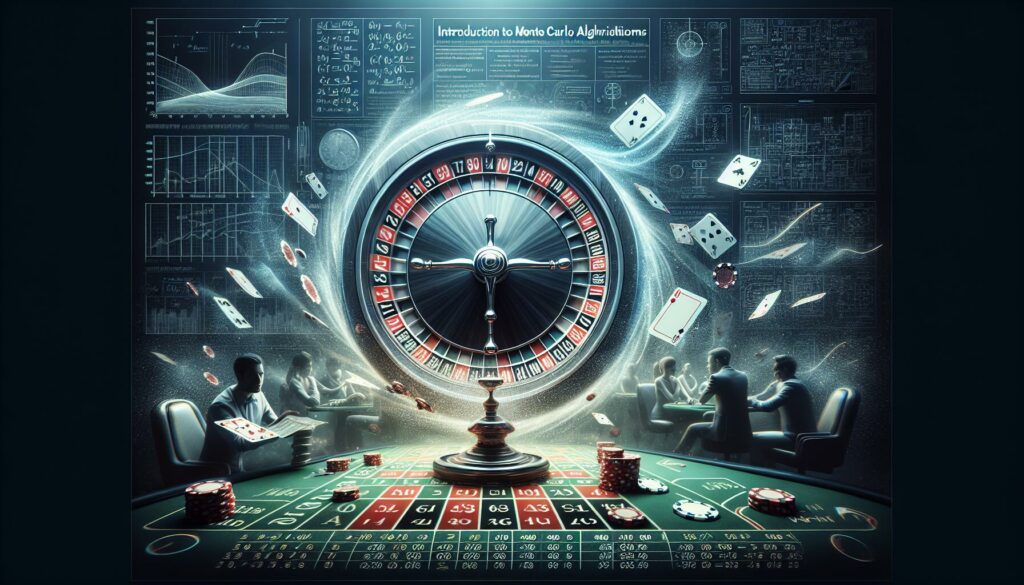
In the vast landscape of computer science and algorithmic problem-solving, Monte Carlo algorithms stand out as a fascinating and powerful approach. These algorithms harness the power of randomness to tackle complex problems that might otherwise be computationally infeasible. In this comprehensive guide, we’ll dive deep into the world of Monte Carlo algorithms, exploring their principles, applications, and implementation in various programming languages.
What Are Monte Carlo Algorithms?
Monte Carlo algorithms are a class of computational algorithms that rely on repeated random sampling to obtain numerical results. The name “Monte Carlo” comes from the famous casino in Monaco, alluding to the element of chance involved in these methods. At their core, Monte Carlo algorithms use randomness to solve problems that might be deterministic in principle.
These algorithms are particularly useful for solving problems with the following characteristics:
- High dimensionality
- Complex systems with many coupled degrees of freedom
- Problems where a deterministic algorithm is not known or is too computationally expensive
The Basic Principle of Monte Carlo Methods
The fundamental idea behind Monte Carlo methods is to use random samples to approximate solutions to quantitative problems. This approach can be broken down into a few key steps:
- Define the domain of possible inputs
- Generate random inputs from the domain
- Perform deterministic computations on the inputs
- Aggregate the results of the individual computations
By repeating this process many times, Monte Carlo algorithms can provide approximate solutions to problems that might be difficult or impossible to solve analytically.
Common Applications of Monte Carlo Algorithms
Monte Carlo algorithms find applications in a wide range of fields, including:
1. Finance and Risk Analysis
In finance, Monte Carlo methods are used for portfolio evaluation, option pricing, and risk management. They help in simulating various market scenarios and assessing potential outcomes.
2. Physics and Chemistry
Scientists use Monte Carlo algorithms to simulate complex physical systems, from particle physics to molecular dynamics.
3. Artificial Intelligence and Machine Learning
Monte Carlo tree search is a popular algorithm in AI, particularly in game-playing algorithms like those used in chess and Go.
4. Computer Graphics
Monte Carlo methods are used in rendering algorithms for realistic lighting and shading in computer-generated imagery.
5. Optimization Problems
Many optimization problems, especially those with multiple local optima, can be tackled using Monte Carlo techniques.
Implementing a Simple Monte Carlo Algorithm
Let’s implement a classic example of a Monte Carlo algorithm: estimating the value of Ï€ (pi). We’ll use the fact that the probability of a random point falling inside a quarter circle inscribed in a square is Ï€/4.
Python Implementation
Here’s a Python implementation of the Monte Carlo method to estimate Ï€:
import random
import math
def estimate_pi(num_points):
inside_circle = 0
total_points = num_points
for _ in range(total_points):
x = random.uniform(0, 1)
y = random.uniform(0, 1)
distance = math.sqrt(x**2 + y**2)
if distance <= 1:
inside_circle += 1
pi_estimate = 4 * inside_circle / total_points
return pi_estimate
# Run the estimation
num_points = 1000000
estimated_pi = estimate_pi(num_points)
print(f"Estimated value of π: {estimated_pi}")
print(f"Actual value of π: {math.pi}")
print(f"Error: {abs(estimated_pi - math.pi)}")
This implementation generates random points within a 1×1 square and checks if they fall within a quarter circle of radius 1. The ratio of points inside the circle to the total number of points, multiplied by 4, gives us an estimate of Ï€.
JavaScript Implementation
Here’s the same algorithm implemented in JavaScript:
function estimatePi(numPoints) {
let insideCircle = 0;
const totalPoints = numPoints;
for (let i = 0; i < totalPoints; i++) {
const x = Math.random();
const y = Math.random();
const distance = Math.sqrt(x**2 + y**2);
if (distance <= 1) {
insideCircle++;
}
}
const piEstimate = 4 * insideCircle / totalPoints;
return piEstimate;
}
// Run the estimation
const numPoints = 1000000;
const estimatedPi = estimatePi(numPoints);
console.log(`Estimated value of π: ${estimatedPi}`);
console.log(`Actual value of π: ${Math.PI}`);
console.log(`Error: ${Math.abs(estimatedPi - Math.PI)}`);
Advanced Monte Carlo Techniques
While the Ï€ estimation example provides a good introduction, Monte Carlo methods can be much more sophisticated. Let’s explore some advanced techniques:
1. Importance Sampling
Importance sampling is a variance reduction technique used to make Monte Carlo simulations more efficient. Instead of sampling uniformly from the input space, we sample from a distribution that focuses on the most “important” regions of the input space.
2. Markov Chain Monte Carlo (MCMC)
MCMC methods are a class of algorithms for sampling from probability distributions. They are particularly useful for high-dimensional problems and are widely used in Bayesian statistics.
3. Metropolis-Hastings Algorithm
This is a specific MCMC method used to obtain a sequence of random samples from a probability distribution for which direct sampling is difficult.
4. Gibbs Sampling
Gibbs sampling is another MCMC method, particularly useful when the joint distribution is not known explicitly, but the conditional distribution of each variable is known.
Monte Carlo Tree Search (MCTS)
Monte Carlo Tree Search is a heuristic search algorithm for decision processes, notably used in game-playing AI. It combines the precision of tree search with the generality of random sampling. Here’s a basic outline of the MCTS algorithm:
- Selection: Start from the root node and select successive child nodes down to a leaf node.
- Expansion: If the leaf node is not a terminal node, create one or more child nodes.
- Simulation: From the new node(s), play out the game randomly until a result is achieved.
- Backpropagation: Use the result of the playout to update information in the nodes on the path from the leaf to the root.
Here’s a simplified Python implementation of MCTS for the game of Tic-Tac-Toe:
import math
import random
class Node:
def __init__(self, state, parent=None):
self.state = state
self.parent = parent
self.children = []
self.visits = 0
self.score = 0
def ucb1(node, parent_visits):
if node.visits == 0:
return float('inf')
return (node.score / node.visits) + math.sqrt(2 * math.log(parent_visits) / node.visits)
def select(node):
while node.children:
node = max(node.children, key=lambda n: ucb1(n, node.visits))
return node
def expand(node):
if not node.children and not is_terminal(node.state):
for move in get_legal_moves(node.state):
new_state = make_move(node.state, move)
new_node = Node(new_state, parent=node)
node.children.append(new_node)
return random.choice(node.children) if node.children else node
def simulate(state):
while not is_terminal(state):
move = random.choice(get_legal_moves(state))
state = make_move(state, move)
return get_result(state)
def backpropagate(node, result):
while node:
node.visits += 1
node.score += result
node = node.parent
def mcts(root_state, num_iterations):
root = Node(root_state)
for _ in range(num_iterations):
node = select(root)
node = expand(node)
result = simulate(node.state)
backpropagate(node, result)
return max(root.children, key=lambda n: n.visits).state
# Helper functions (to be implemented)
def is_terminal(state):
# Check if the game is over
pass
def get_legal_moves(state):
# Return list of legal moves
pass
def make_move(state, move):
# Apply move to state and return new state
pass
def get_result(state):
# Return the result of the game (1 for win, 0 for draw, -1 for loss)
pass
# Usage
initial_state = [0] * 9 # Represent empty 3x3 board
best_move = mcts(initial_state, 1000)
print(f"Best move: {best_move}")
This implementation provides a framework for MCTS in Tic-Tac-Toe. You would need to implement the helper functions (is_terminal, get_legal_moves, make_move, get_result) specific to Tic-Tac-Toe rules.
Advantages and Limitations of Monte Carlo Algorithms
Advantages:
- Can handle high-dimensional problems efficiently
- Often simpler to implement than deterministic algorithms for complex problems
- Can provide good approximate solutions when exact solutions are not feasible
- Naturally parallelizable, making them suitable for distributed computing
Limitations:
- Results are approximate and have statistical errors
- May require a large number of samples for accurate results, which can be computationally expensive
- The quality of results can be sensitive to the quality of random number generation
- May struggle with rare events or tail probabilities
Optimizing Monte Carlo Algorithms
To get the most out of Monte Carlo algorithms, consider these optimization strategies:
1. Variance Reduction Techniques
Use methods like stratified sampling, control variates, or antithetic variates to reduce the variance of your estimates and improve efficiency.
2. Parallel Implementation
Take advantage of the inherently parallel nature of Monte Carlo methods by implementing them on multi-core CPUs or GPUs.
3. Adaptive Sampling
Dynamically adjust the sampling strategy based on intermediate results to focus computational effort where it’s most needed.
4. Quasi-Monte Carlo Methods
Use low-discrepancy sequences instead of pseudo-random numbers to potentially achieve faster convergence rates.
Conclusion
Monte Carlo algorithms represent a powerful and versatile approach to problem-solving in computer science and beyond. By harnessing the power of randomness, these methods can tackle complex problems that would be intractable with deterministic approaches. From estimating mathematical constants to powering sophisticated AI systems, Monte Carlo techniques continue to play a crucial role in advancing the frontiers of computational problem-solving.
As you continue your journey in algorithmic thinking and coding education, keep Monte Carlo methods in your toolkit. They offer a unique perspective on problem-solving and can be invaluable in situations where traditional deterministic algorithms fall short. Whether you’re preparing for technical interviews at major tech companies or simply expanding your programming skills, understanding and implementing Monte Carlo algorithms will undoubtedly enhance your capabilities as a developer and problem-solver.
Remember, the key to mastering Monte Carlo methods lies in practice and experimentation. Try implementing these algorithms for different problems, analyze their performance, and explore ways to optimize them. As you gain experience, you’ll develop an intuition for when and how to apply these powerful techniques effectively.