Understanding the Traveling Salesman Problem: A Comprehensive Guide
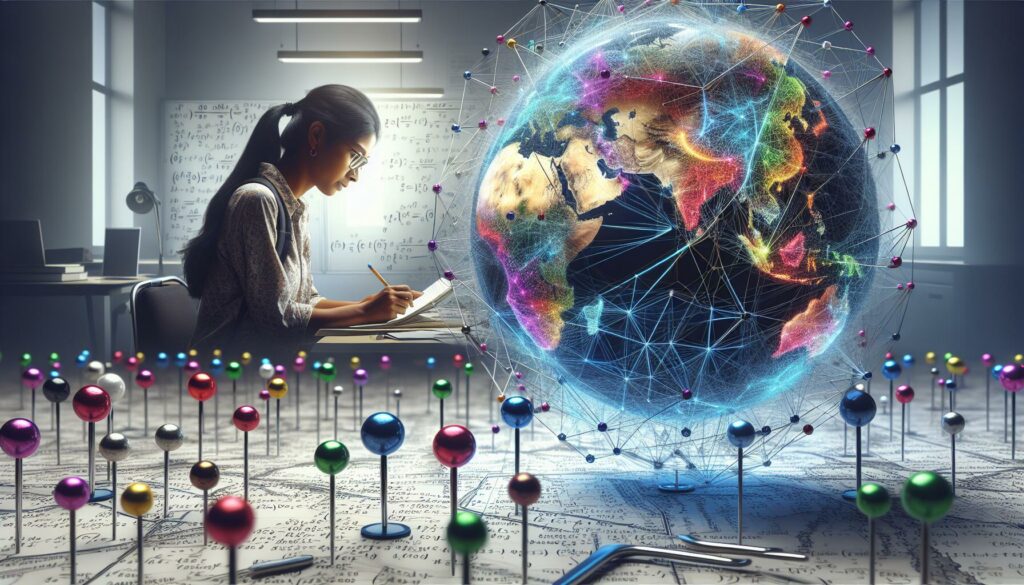
In the world of computer science and optimization, few problems have captivated researchers and practitioners as much as the Traveling Salesman Problem (TSP). This classic algorithmic challenge has been a cornerstone of computational complexity theory and has far-reaching applications in logistics, circuit design, and even DNA sequencing. In this comprehensive guide, we’ll dive deep into the Traveling Salesman Problem, exploring its origins, significance, and the various approaches used to tackle it.
What is the Traveling Salesman Problem?
The Traveling Salesman Problem is a well-known optimization problem in computer science and operations research. It asks the following question: “Given a list of cities and the distances between each pair of cities, what is the shortest possible route that visits each city exactly once and returns to the origin city?”
While this problem might seem straightforward at first glance, it quickly becomes complex as the number of cities increases. The TSP is known to be NP-hard, meaning that there is no known polynomial-time algorithm that can solve it optimally for all instances.
Historical Context and Significance
The origins of the Traveling Salesman Problem can be traced back to the 1800s, but it gained significant attention in the 1930s when mathematicians began studying it more formally. The problem was first formulated as a mathematical problem by Karl Menger in Vienna and Harvard.
The TSP has become a benchmark problem in computational complexity theory and optimization. Its significance lies not only in its practical applications but also in its role as a representative of a larger class of difficult computational problems.
Why is the TSP Important?
- Theoretical Significance: The TSP is a prime example of an NP-hard problem, helping researchers understand the limits of computational efficiency.
- Practical Applications: Solutions to the TSP have real-world applications in logistics, planning, and manufacturing.
- Algorithm Development: Efforts to solve the TSP have led to advancements in algorithm design and optimization techniques.
- Interdisciplinary Research: The problem has attracted attention from various fields, including mathematics, computer science, and operations research.
Formulating the Traveling Salesman Problem
To understand the TSP more formally, let’s break it down into its mathematical components:
Mathematical Formulation
Given a set of n cities and a cost matrix C = (cij) where cij represents the cost (or distance) of traveling from city i to city j, the goal is to find a permutation π of the cities that minimizes the total cost:
minimize Σ cπ(i),π(i+1) + cπ(n),π(1)
i=1 to n-1
This formulation assumes that the problem is symmetric (i.e., the distance from city A to city B is the same as from B to A) and satisfies the triangle inequality (the direct path between two cities is never longer than a path through an intermediate city).
Graph Representation
The TSP can also be represented as a graph problem:
- Cities are represented as vertices in a graph.
- The paths between cities are represented as edges.
- The cost or distance between cities is represented as edge weights.
The goal is to find a Hamiltonian cycle (a cycle that visits each vertex exactly once) with the minimum total weight.
Complexity of the Traveling Salesman Problem
The TSP is notorious for its computational complexity. Let’s explore why this problem is so challenging:
NP-Hardness
The Traveling Salesman Problem is NP-hard, which means:
- There is no known polynomial-time algorithm to solve it optimally for all instances.
- The problem is at least as hard as any problem in the complexity class NP.
- If a polynomial-time algorithm were found for the TSP, it would imply P = NP, solving one of the most important open problems in computer science.
Exponential Growth
The number of possible tours for n cities grows factorially:
- For n cities, there are (n-1)! / 2 possible tours (accounting for symmetry).
- This means that for just 20 cities, there are over 60 quintillion possible tours!
This exponential growth makes brute-force approaches impractical for even moderately sized problems.
Approaches to Solving the TSP
Despite its complexity, researchers and practitioners have developed various approaches to tackle the Traveling Salesman Problem. These methods can be broadly categorized into exact algorithms and heuristic/approximation algorithms.
Exact Algorithms
Exact algorithms guarantee finding the optimal solution but may take exponential time for large instances.
1. Brute Force
The simplest approach is to generate all possible permutations of cities and calculate the total distance for each, selecting the shortest one. While straightforward, this method is impractical for more than a handful of cities due to its factorial time complexity.
2. Dynamic Programming
Held-Karp algorithm is a dynamic programming approach that solves the TSP in O(n2 * 2n) time. While still exponential, it’s a significant improvement over brute force.
3. Branch and Bound
This technique involves systematically enumerating candidate solutions by means of state space search: the set of candidate solutions is thought of as forming a rooted tree with the full set at the root. It uses bounding functions to prune large parts of the search tree.
Heuristic and Approximation Algorithms
These algorithms aim to find good (but not necessarily optimal) solutions in reasonable time.
1. Nearest Neighbor
This greedy algorithm starts from an arbitrary city and repeatedly visits the nearest unvisited city until all cities have been visited. It’s simple and fast but can produce poor results in some cases.
2. Christofides Algorithm
This is a 1.5-approximation algorithm for metric TSP instances. It guarantees that its solution will be no more than 50% longer than the optimal solution.
3. Lin-Kernighan Heuristic
This is one of the most effective heuristics for generating optimal or near-optimal solutions for the TSP. It’s a local search algorithm that swaps edges to improve the tour.
4. Genetic Algorithms
These algorithms use techniques inspired by natural evolution to evolve a population of solutions over time, often producing high-quality results for large instances.
Implementing a Simple TSP Solver
To give you a practical understanding of the TSP, let’s implement a simple nearest neighbor algorithm in Python:
import numpy as np
def nearest_neighbor_tsp(distances):
n = len(distances)
unvisited = set(range(1, n))
tour = [0]
while unvisited:
last = tour[-1]
next_city = min(unvisited, key=lambda city: distances[last][city])
tour.append(next_city)
unvisited.remove(next_city)
tour.append(0)
return tour
# Example usage
distances = np.array([
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
])
tour = nearest_neighbor_tsp(distances)
print(f"Tour: {tour}")
print(f"Tour length: {sum(distances[tour[i]][tour[i+1]] for i in range(len(tour)-1))}")
This implementation uses the nearest neighbor heuristic to construct a tour. While it’s not guaranteed to find the optimal solution, it often produces reasonably good results quickly.
Real-World Applications of the TSP
The Traveling Salesman Problem isn’t just a theoretical exercise; it has numerous practical applications across various industries:
1. Logistics and Transportation
- Route planning for delivery services
- Optimizing supply chain operations
- Planning efficient bus or subway routes
2. Manufacturing
- Optimizing the movement of robotic arms in factories
- Minimizing tool path length in CNC machining
3. Circuit Board Design
- Minimizing the total wire length in printed circuit boards
4. DNA Sequencing
- Determining the order of DNA fragments in genome sequencing
5. Telescope Scheduling
- Optimizing the sequence of observations for large telescopes
Variations of the TSP
The classic TSP has inspired several variations, each with its own unique challenges and applications:
1. Asymmetric TSP
In this variation, the distance between two cities may be different depending on the direction of travel. This is common in real-world scenarios where factors like one-way streets or wind direction can affect travel times.
2. Multiple Traveling Salesmen Problem (mTSP)
This variant involves multiple salesmen who must collectively visit all cities. It’s particularly relevant in scenarios involving multiple vehicles or agents.
3. Capacitated Vehicle Routing Problem (CVRP)
An extension of the TSP where vehicles have limited capacity and must make deliveries to customers. This is highly relevant in logistics and supply chain management.
4. Time-Dependent TSP
In this version, the travel times between cities vary based on the time of day, accounting for factors like traffic congestion.
Advanced Techniques for Solving Large TSP Instances
For very large TSP instances, even heuristic methods may struggle to find good solutions in reasonable time. Researchers have developed advanced techniques to tackle these challenging cases:
1. Concorde TSP Solver
Concorde is a computer code for the symmetric traveling salesman problem and some related network optimization problems. It has been used to solve instances with tens of thousands of cities to optimality.
2. Parallel Computing
Leveraging multiple processors or distributed systems can significantly speed up TSP algorithms, especially for large instances.
3. Machine Learning Approaches
Recent research has explored using neural networks and reinforcement learning to tackle the TSP, showing promising results for certain types of instances.
4. Hybrid Algorithms
Combining multiple approaches, such as using metaheuristics to improve solutions found by construction heuristics, can lead to powerful solvers for large-scale TSPs.
The Future of TSP Research
Despite decades of intense study, the Traveling Salesman Problem continues to be an active area of research:
Quantum Computing
Quantum algorithms, such as quantum approximate optimization algorithm (QAOA), show potential for solving combinatorial optimization problems like the TSP more efficiently than classical algorithms.
Neuromorphic Computing
Brain-inspired computing architectures may offer new ways to approach the TSP and similar optimization problems.
Integration with Machine Learning
Combining traditional TSP algorithms with machine learning techniques is an exciting frontier, potentially leading to more adaptive and efficient solvers.
Conclusion
The Traveling Salesman Problem stands as a testament to the challenges and opportunities in algorithmic problem-solving. Its simple premise belies its computational complexity, making it a fascinating subject for researchers and practitioners alike. From its theoretical significance in complexity theory to its practical applications in logistics and beyond, the TSP continues to drive innovation in algorithm design and optimization techniques.
As we’ve explored in this guide, there’s no one-size-fits-all solution to the TSP. The choice of algorithm depends on the specific requirements of the problem at hand, balancing factors like solution quality, computation time, and problem size. Whether you’re a computer science student diving into algorithmic complexity, a software engineer optimizing delivery routes, or a researcher pushing the boundaries of combinatorial optimization, understanding the Traveling Salesman Problem provides valuable insights into the nature of computational challenges and the creative approaches we use to tackle them.
As technology continues to advance, with developments in areas like quantum computing and artificial intelligence, we may see new breakthroughs in solving the TSP and related problems. The journey of the traveling salesman is far from over, and it continues to lead us to new frontiers in computer science and beyond.