Handling Large Data Sets: External Sorting Algorithms
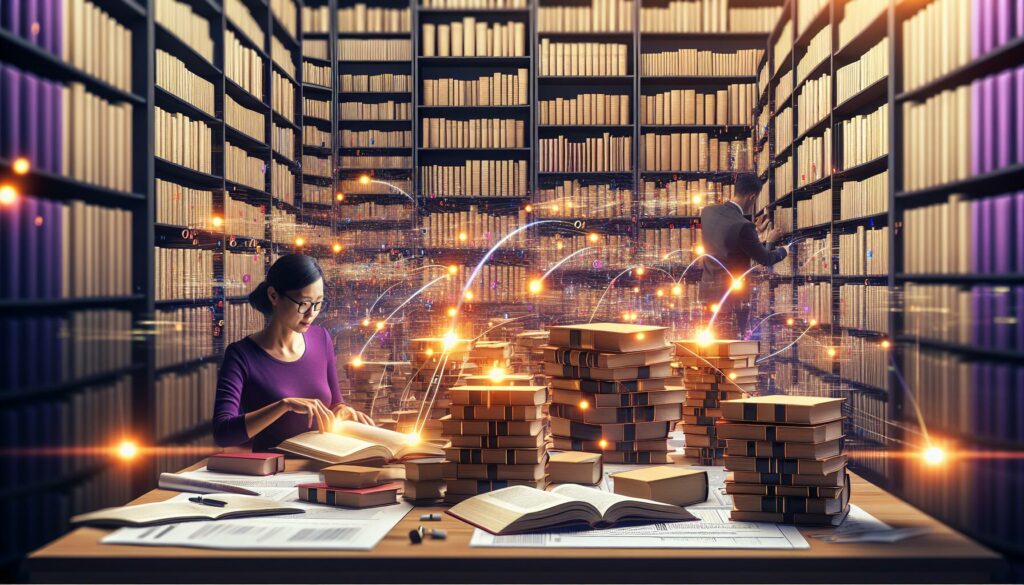
In the world of big data and large-scale computing, efficiently handling and processing massive data sets is a crucial skill for any programmer or data scientist. One of the fundamental operations we often need to perform on large data sets is sorting. However, when the data becomes too large to fit into the main memory of a computer, traditional in-memory sorting algorithms fall short. This is where external sorting algorithms come into play.
In this comprehensive guide, we’ll dive deep into external sorting algorithms, exploring their importance, mechanics, and various implementations. We’ll also discuss real-world applications and provide code examples to help you understand and implement these powerful techniques.
Table of Contents
- Understanding External Sorting
- The Need for External Sorting
- Basic Concepts of External Sorting
- External Merge Sort Algorithm
- Optimizations and Variations
- Implementing External Sort in Python
- Real-world Applications
- Challenges and Considerations
- Comparison with Other Techniques
- Conclusion
1. Understanding External Sorting
External sorting is a class of sorting algorithms designed to handle large volumes of data that exceed the capacity of a computer’s main memory (RAM). These algorithms efficiently sort data that resides in slower external memory, such as hard drives or SSDs, by minimizing the number of I/O operations and maximizing the use of available RAM.
The key principle behind external sorting is to break down the large data set into smaller, manageable chunks that can fit into main memory, sort these chunks individually, and then merge them back together to produce the final sorted output.
2. The Need for External Sorting
As data volumes continue to grow exponentially, many real-world scenarios require processing data sets that are far too large to fit into RAM. Some common situations where external sorting becomes necessary include:
- Processing large log files in system administration
- Sorting massive databases for efficient querying
- Handling big data in data science and machine learning applications
- Managing large-scale distributed systems
- Sorting genome sequencing data in bioinformatics
In these cases, traditional in-memory sorting algorithms like QuickSort or MergeSort are not feasible due to memory constraints. External sorting algorithms provide a solution by efficiently utilizing both RAM and external storage to sort large data sets.
3. Basic Concepts of External Sorting
Before diving into specific algorithms, let’s familiarize ourselves with some key concepts in external sorting:
3.1 Run
A run is a contiguous sequence of sorted elements. In external sorting, we typically create initial runs by reading chunks of data that fit into memory, sorting them, and writing them back to external storage.
3.2 Merge
Merging is the process of combining two or more sorted runs into a single sorted run. This operation is fundamental to many external sorting algorithms.
3.3 K-way Merge
A k-way merge involves combining k sorted runs simultaneously. This technique is often used to reduce the number of passes required in external sorting.
3.4 Replacement Selection
Replacement selection is a technique used to generate initial runs that are typically larger than the available memory, improving the efficiency of the sorting process.
4. External Merge Sort Algorithm
The external merge sort is one of the most common and efficient external sorting algorithms. It consists of two main phases: the run creation phase and the merge phase.
4.1 Run Creation Phase
In this phase, the algorithm reads chunks of data from the input file into memory, sorts them using an efficient in-memory sorting algorithm (like QuickSort), and writes the sorted chunks (runs) back to disk. The process continues until the entire input file has been processed.
4.2 Merge Phase
The merge phase involves combining the sorted runs to produce the final sorted output. This is typically done using a k-way merge, where k is chosen based on the available memory and the number of runs created in the first phase.
Here’s a high-level overview of the external merge sort algorithm:
1. Read chunks of data from the input file
2. Sort each chunk in memory
3. Write sorted chunks (runs) to temporary files
4. Merge the runs using k-way merge
5. Write the final sorted output to a file
5. Optimizations and Variations
Several optimizations and variations can be applied to the basic external merge sort algorithm to improve its performance:
5.1 Polyphase Merge
Polyphase merge is a technique that distributes runs across multiple output tapes (or files) during the run creation phase. This allows for more efficient merging in subsequent phases.
5.2 Cascade Merge
Cascade merge involves merging runs in a hierarchical manner, reducing the number of passes required for large data sets.
5.3 Replacement Selection
As mentioned earlier, replacement selection can be used to generate runs that are larger than the available memory, potentially reducing the number of runs and improving overall efficiency.
5.4 Parallel External Sorting
Leveraging multiple processors or distributed systems can significantly speed up external sorting for extremely large data sets.
6. Implementing External Sort in Python
Let’s implement a basic external merge sort algorithm in Python to demonstrate the concept. This implementation will sort a large file of integers:
import os
import tempfile
def create_runs(input_file, run_size, temp_file_prefix):
runs = []
with open(input_file, 'r') as f:
run = []
for line in f:
run.append(int(line.strip()))
if len(run) == run_size:
run.sort()
temp_file = tempfile.NamedTemporaryFile(mode='w+', delete=False, prefix=temp_file_prefix)
for num in run:
temp_file.write(f"{num}\n")
temp_file.close()
runs.append(temp_file.name)
run = []
if run:
run.sort()
temp_file = tempfile.NamedTemporaryFile(mode='w+', delete=False, prefix=temp_file_prefix)
for num in run:
temp_file.write(f"{num}\n")
temp_file.close()
runs.append(temp_file.name)
return runs
def merge_runs(runs, output_file):
with open(output_file, 'w') as out:
files = [open(run, 'r') for run in runs]
mins = [int(f.readline().strip()) for f in files]
while True:
min_val = min(mins)
min_idx = mins.index(min_val)
out.write(f"{min_val}\n")
next_line = files[min_idx].readline()
if next_line:
mins[min_idx] = int(next_line.strip())
else:
files[min_idx].close()
os.unlink(runs[min_idx])
files.pop(min_idx)
mins.pop(min_idx)
runs.pop(min_idx)
if not mins:
break
def external_sort(input_file, output_file, run_size):
temp_file_prefix = 'temp_run_'
runs = create_runs(input_file, run_size, temp_file_prefix)
merge_runs(runs, output_file)
# Usage
input_file = 'large_unsorted_file.txt'
output_file = 'sorted_output.txt'
run_size = 1000000 # Adjust based on available memory
external_sort(input_file, output_file, run_size)
This implementation demonstrates the basic principles of external sorting:
- The
create_runs
function reads chunks of data from the input file, sorts them in memory, and writes them to temporary files. - The
merge_runs
function performs a k-way merge of the sorted runs to produce the final sorted output. - The
external_sort
function orchestrates the entire process.
Note that this is a basic implementation and can be further optimized for better performance in real-world scenarios.
7. Real-world Applications
External sorting algorithms find applications in various domains where large-scale data processing is required:
7.1 Database Management Systems
External sorting is crucial for efficiently sorting large tables, creating indexes, and performing join operations in database systems.
7.2 Big Data Processing
Frameworks like Hadoop and Spark use external sorting techniques to process and analyze massive data sets across distributed systems.
7.3 Operating Systems
File systems and I/O subsystems in operating systems often employ external sorting for managing large directories and file operations.
7.4 Scientific Computing
Fields like genomics, climate modeling, and particle physics deal with enormous data sets that require external sorting for analysis and processing.
7.5 Log Analysis
System administrators and security analysts use external sorting to process and analyze large log files for troubleshooting and threat detection.
8. Challenges and Considerations
While external sorting algorithms provide powerful solutions for handling large data sets, they come with their own set of challenges:
8.1 I/O Performance
External sorting is heavily dependent on I/O operations, which are significantly slower than in-memory operations. Optimizing I/O performance is crucial for efficient external sorting.
8.2 Memory Management
Effective utilization of available memory is essential. Balancing between run size and the number of runs is important for optimal performance.
8.3 Disk Space Requirements
External sorting typically requires additional disk space for temporary files. Managing this space efficiently is important, especially for very large data sets.
8.4 Complexity
Implementing efficient external sorting algorithms can be more complex than in-memory sorting, requiring careful handling of file I/O and memory management.
8.5 Parallelization and Distribution
For extremely large data sets, parallelizing the sorting process across multiple machines introduces additional complexity in terms of data distribution and result aggregation.
9. Comparison with Other Techniques
While external sorting is powerful, it’s important to understand how it compares to other techniques for handling large data sets:
9.1 In-Memory Sorting
For data sets that fit in memory, traditional algorithms like QuickSort or MergeSort are generally faster due to the absence of I/O overhead.
9.2 Database Indexing
For structured data in databases, creating appropriate indexes can often provide faster access to sorted data than performing external sorts on-demand.
9.3 Distributed Sorting
For extremely large data sets, distributed sorting algorithms that leverage multiple machines can offer better scalability than single-machine external sorting.
9.4 Approximate Sorting
In some applications, approximate sorting techniques like bucket sort or counting sort can provide faster results when exact ordering is not critical.
10. Conclusion
External sorting algorithms are essential tools in the modern programmer’s toolkit for handling large-scale data processing tasks. By understanding the principles behind external sorting and mastering techniques like external merge sort, you’ll be well-equipped to tackle big data challenges in various domains.
As you continue to explore this topic, consider the following areas for further study:
- Advanced optimizations for external sorting algorithms
- Implementing external sorting in distributed systems
- Benchmarking and performance tuning of external sorting implementations
- Exploring specialized external sorting algorithms for specific data types or applications
Remember that efficient handling of large data sets is a valuable skill in today’s data-driven world. Whether you’re preparing for technical interviews at major tech companies or working on real-world big data projects, a solid understanding of external sorting algorithms will serve you well in your programming career.
Keep practicing, experimenting with different implementations, and applying these concepts to real-world problems. With time and experience, you’ll develop the expertise to tackle even the most challenging data processing tasks with confidence and efficiency.