Cache-Friendly Algorithms and Data Structures: Optimizing Performance Through Efficient Memory Access
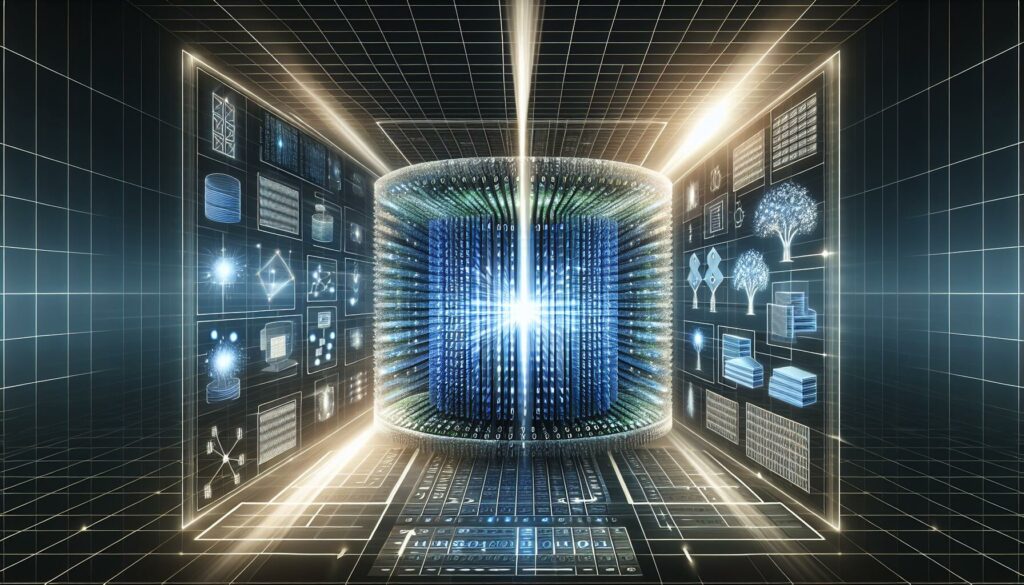
In the world of computer science and software engineering, performance optimization is a crucial aspect of creating efficient and scalable applications. One of the most significant factors affecting performance is how well our algorithms and data structures interact with the computer’s memory hierarchy, particularly the cache. In this comprehensive guide, we’ll explore cache-friendly algorithms and data structures, understanding their importance, and learning how to implement them to boost your application’s performance.
Table of Contents
- Understanding Cache and Its Importance
- Principles of Cache-Friendly Design
- Cache-Friendly Algorithms
- Cache-Friendly Data Structures
- Implementing Cache-Friendly Techniques
- Measuring Cache Performance
- Real-World Applications
- Conclusion
1. Understanding Cache and Its Importance
Before diving into cache-friendly algorithms and data structures, it’s essential to understand what cache is and why it’s crucial for performance.
What is Cache?
Cache is a small, fast memory that sits between the CPU and main memory (RAM). It stores frequently accessed data and instructions to reduce the time required to fetch them from slower main memory. There are typically multiple levels of cache (L1, L2, L3) with varying sizes and speeds.
Why is Cache Important?
The importance of cache lies in the significant speed difference between cache and main memory:
- L1 cache access: ~1 nanosecond
- L2 cache access: ~4 nanoseconds
- L3 cache access: ~10 nanoseconds
- Main memory access: ~100 nanoseconds
As we can see, accessing data from cache is orders of magnitude faster than from main memory. Therefore, designing algorithms and data structures that maximize cache utilization can lead to substantial performance improvements.
2. Principles of Cache-Friendly Design
To create cache-friendly algorithms and data structures, we need to understand and apply certain principles:
Spatial Locality
Spatial locality refers to the tendency of a program to access memory locations that are near each other. When a memory location is accessed, nearby locations are also loaded into the cache. To take advantage of this:
- Arrange data in contiguous memory locations
- Access data sequentially whenever possible
- Use array-based structures instead of linked structures when appropriate
Temporal Locality
Temporal locality is the principle that recently accessed items are likely to be accessed again in the near future. To leverage this:
- Reuse data that’s already in the cache
- Organize computations to maximize data reuse
- Use techniques like loop tiling to improve temporal locality
Minimize Cache Misses
A cache miss occurs when the requested data is not found in the cache and must be fetched from main memory. To reduce cache misses:
- Prefetch data before it’s needed
- Align data structures to cache line boundaries
- Avoid unnecessary data movement between cache and main memory
3. Cache-Friendly Algorithms
Let’s explore some algorithms that are designed to be cache-friendly and compare them with their less cache-efficient counterparts.
Matrix Multiplication
Consider the naive approach to matrix multiplication:
for (i = 0; i < n; i++)
for (j = 0; j < n; j++)
for (k = 0; k < n; k++)
C[i][j] += A[i][k] * B[k][j];
This algorithm accesses B in a column-wise manner, which is cache-unfriendly for row-major languages like C. A cache-friendly version would be:
for (i = 0; i < n; i++)
for (k = 0; k < n; k++)
for (j = 0; j < n; j++)
C[i][j] += A[i][k] * B[k][j];
This version accesses both matrices in a row-wise manner, improving spatial locality.
Cache-Oblivious Algorithms
Cache-oblivious algorithms are designed to be efficient on any cache hierarchy without knowing the specific cache parameters. The Strassen algorithm for matrix multiplication is an example of a cache-oblivious algorithm.
Merge Sort vs. Quicksort
While Quicksort is often faster in practice, Merge Sort can be more cache-friendly due to its sequential access patterns. However, a cache-aware implementation of Quicksort can outperform Merge Sort by using techniques like:
- Choosing pivot elements to align with cache lines
- Using insertion sort for small subarrays
- Employing cache-conscious partitioning strategies
4. Cache-Friendly Data Structures
The way we organize and access data can significantly impact cache performance. Let’s examine some cache-friendly data structures and their benefits.
Arrays vs. Linked Lists
Arrays are generally more cache-friendly than linked lists due to their contiguous memory layout. Consider the following comparison:
// Array traversal
int array[1000000];
for (int i = 0; i < 1000000; i++) {
sum += array[i];
}
// Linked list traversal
struct Node {
int data;
Node* next;
};
Node* head;
while (head != nullptr) {
sum += head->data;
head = head->next;
}
The array traversal will have better cache performance due to spatial locality, while the linked list may cause more cache misses.
Cache-Friendly Trees
Traditional binary search trees can be cache-unfriendly due to their pointer-based nature. Some cache-friendly alternatives include:
- B-Trees: By storing multiple keys in each node, B-Trees reduce the tree height and improve cache utilization.
- Van Emde Boas Trees: These trees offer O(log log n) operations and are inherently cache-oblivious.
- Cache-Oblivious B-Trees: Combining the benefits of B-Trees and cache-oblivious design.
Flat Data Structures
Flat data structures store related data in contiguous memory, improving cache performance. For example, instead of an array of pointers to objects, consider using a struct-of-arrays approach:
// Cache-unfriendly
struct Person {
char* name;
int age;
float height;
};
Person* people[1000000];
// Cache-friendly
struct People {
char* names[1000000];
int ages[1000000];
float heights[1000000];
} people;
The cache-friendly version allows for better spatial locality when accessing specific attributes across multiple records.
5. Implementing Cache-Friendly Techniques
Now that we’ve covered the principles and some examples, let’s look at how to implement cache-friendly techniques in your code.
Loop Tiling
Loop tiling (or blocking) is a technique to improve cache utilization by breaking large loops into smaller chunks that fit in the cache. Here’s an example of matrix multiplication with loop tiling:
const int TILE_SIZE = 32;
for (int i = 0; i < n; i += TILE_SIZE)
for (int j = 0; j < n; j += TILE_SIZE)
for (int k = 0; k < n; k += TILE_SIZE)
for (int ii = i; ii < min(i + TILE_SIZE, n); ii++)
for (int jj = j; jj < min(j + TILE_SIZE, n); jj++)
for (int kk = k; kk < min(k + TILE_SIZE, n); kk++)
C[ii][jj] += A[ii][kk] * B[kk][jj];
This technique improves temporal locality by ensuring that data loaded into the cache is reused before being evicted.
Data Alignment
Aligning data structures to cache line boundaries can improve performance by reducing the number of cache lines accessed. In C++, you can use alignment specifiers:
struct alignas(64) CacheAlignedStruct {
int data[16];
};
This ensures that each instance of CacheAlignedStruct
starts at a 64-byte boundary, which is a common cache line size.
Prefetching
Prefetching involves loading data into the cache before it’s needed. Many compilers perform automatic prefetching, but you can also do it manually:
#include <xmmintrin.h>
void process_data(int* data, int size) {
for (int i = 0; i < size; i++) {
_mm_prefetch((char*)&data[i + 16], _MM_HINT_T0);
// Process data[i]
}
}
This example prefetches data 16 elements ahead of the current processing position.
6. Measuring Cache Performance
To optimize for cache performance, it’s crucial to measure and analyze cache behavior. Here are some tools and techniques to help you do that:
Hardware Performance Counters
Modern CPUs have built-in performance counters that can measure cache-related events. Tools like perf
on Linux can access these counters:
perf stat -e cache-misses,cache-references ./your_program
This command will run your program and report the number of cache misses and references.
Valgrind and Cachegrind
Valgrind is a powerful tool for memory debugging and profiling. Its Cachegrind tool simulates the cache hierarchy and provides detailed statistics:
valgrind --tool=cachegrind ./your_program
This will generate a report with information about cache misses, accesses, and more.
Intel VTune Profiler
For Intel processors, the VTune Profiler provides advanced performance analysis capabilities, including detailed cache performance metrics and visualizations.
7. Real-World Applications
Cache-friendly algorithms and data structures have significant impacts in various domains:
Database Systems
Database management systems heavily rely on cache-friendly techniques to optimize query performance. For example:
- Column-oriented storage for analytical workloads
- Cache-conscious index structures like CSS-Trees
- Buffer pool management algorithms that consider cache behavior
Graphics and Game Development
In graphics programming and game development, cache-friendly techniques are crucial for performance:
- Texture atlases to improve spatial locality in texture access
- Vertex cache optimization for efficient rendering
- Data-oriented design for game engine architecture
Scientific Computing
Large-scale scientific simulations benefit greatly from cache optimizations:
- Cache-oblivious algorithms for linear algebra operations
- Tiled algorithms for stencil computations in fluid dynamics
- Optimized FFT implementations considering cache behavior
8. Conclusion
Cache-friendly algorithms and data structures are essential tools in a programmer’s arsenal for achieving high performance in modern computing systems. By understanding the principles of cache behavior and applying techniques like spatial and temporal locality, loop tiling, and appropriate data structure selection, you can significantly improve the efficiency of your code.
Remember that cache optimization is often a balancing act between different performance factors, and what works best can depend on the specific hardware, workload, and problem size. Always measure and profile your code to ensure that your optimizations are having the desired effect.
As you continue to develop your skills in algorithm design and implementation, keep cache-friendliness in mind. It’s a powerful concept that can set your code apart in terms of performance and efficiency, especially when working on large-scale systems or performance-critical applications.
By mastering cache-friendly techniques, you’ll be better equipped to tackle complex programming challenges and optimize systems for real-world use cases. Whether you’re preparing for technical interviews at top tech companies or building high-performance applications, understanding and implementing cache-friendly algorithms and data structures will be a valuable skill in your programming journey.