Algorithms Behind Modern Encryption Techniques
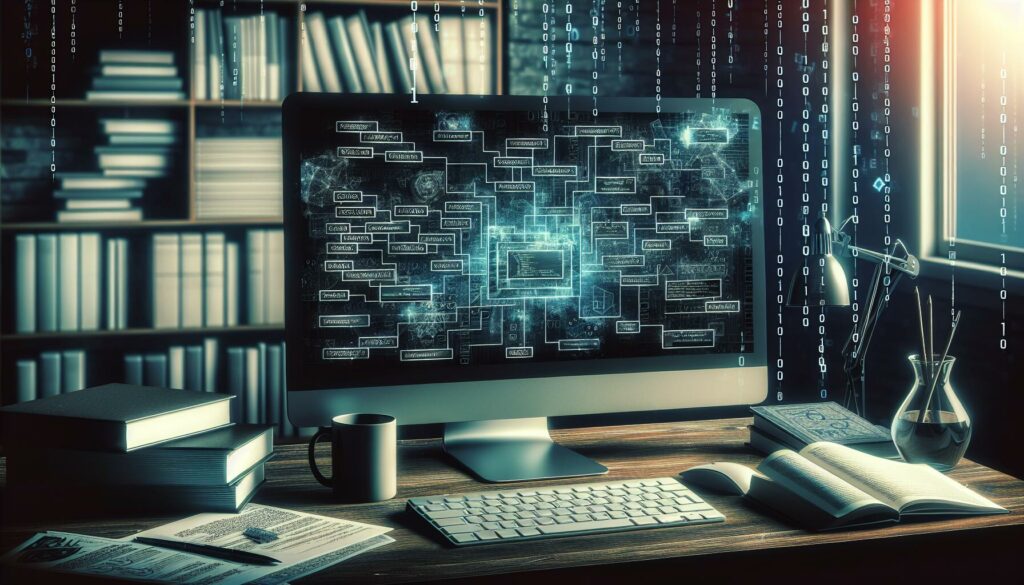
In today’s digital age, where data breaches and cyber threats are increasingly common, encryption plays a crucial role in protecting sensitive information. As we dive into the world of modern encryption techniques, we’ll explore the sophisticated algorithms that form the backbone of secure communication and data storage. This article will provide an in-depth look at the most widely used encryption algorithms, their inner workings, and their applications in various sectors of technology.
1. Introduction to Encryption
Encryption is the process of encoding information in such a way that only authorized parties can access it. The primary goal of encryption is to protect the confidentiality of digital data, whether it’s stored on computer systems or transmitted over networks like the internet.
At its core, encryption involves two main components:
- Plaintext: The original, readable data
- Ciphertext: The encrypted, unreadable version of the data
The process of converting plaintext to ciphertext is called encryption, while the reverse process is called decryption. Both processes typically rely on a key, which is a piece of information (usually a string of numbers or letters) that determines the output of the encryption algorithm.
2. Symmetric Encryption Algorithms
Symmetric encryption algorithms use the same key for both encryption and decryption. These algorithms are generally faster and more efficient for large amounts of data, but they require secure key exchange between parties.
2.1 Advanced Encryption Standard (AES)
AES is one of the most widely used symmetric encryption algorithms. It was established by the U.S. National Institute of Standards and Technology (NIST) in 2001 and is now used worldwide.
Key features of AES:
- Block cipher with fixed block size of 128 bits
- Supports key sizes of 128, 192, and 256 bits
- Uses a series of linked operations, including substitutions, permutations, and mixing of input data
The AES encryption process involves several rounds of transformations, with the number of rounds depending on the key size:
- 128-bit key: 10 rounds
- 192-bit key: 12 rounds
- 256-bit key: 14 rounds
Each round consists of several processing steps, including:
- SubBytes: A non-linear substitution step where each byte is replaced with another according to a lookup table
- ShiftRows: A transposition step where each row of the state is shifted cyclically a certain number of steps
- MixColumns: A mixing operation which operates on the columns of the state, combining the four bytes in each column
- AddRoundKey: Each byte of the state is combined with the round key using bitwise XOR
Here’s a simplified representation of the AES encryption process in Python:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_aes(plaintext, key):
cipher = AES.new(key, AES.MODE_ECB)
return cipher.encrypt(plaintext)
def decrypt_aes(ciphertext, key):
cipher = AES.new(key, AES.MODE_ECB)
return cipher.decrypt(ciphertext)
# Generate a random 256-bit key
key = get_random_bytes(32)
# Plaintext must be a multiple of 16 bytes
plaintext = b'This is a secret message padded to 32 bytes'
ciphertext = encrypt_aes(plaintext, key)
decrypted_text = decrypt_aes(ciphertext, key)
print(f"Plaintext: {plaintext}")
print(f"Ciphertext: {ciphertext.hex()}")
print(f"Decrypted: {decrypted_text}")
2.2 Data Encryption Standard (DES) and Triple DES
DES was one of the earliest encryption standards, developed in the 1970s. While it’s now considered insecure due to its small key size (56 bits), its successor, Triple DES, is still in use in some legacy systems.
Triple DES applies the DES algorithm three times to each data block, effectively increasing the key size and security. The process typically uses three different keys, resulting in a total key length of 168 bits.
3. Asymmetric Encryption Algorithms
Asymmetric encryption, also known as public-key cryptography, uses two different keys: a public key for encryption and a private key for decryption. This approach solves the key distribution problem inherent in symmetric encryption but is generally slower and more computationally intensive.
3.1 RSA (Rivest-Shamir-Adleman)
RSA is one of the first and most widely used asymmetric encryption algorithms. Its security is based on the difficulty of factoring the product of two large prime numbers.
The RSA algorithm involves the following steps:
- Key generation:
- Choose two large prime numbers, p and q
- Compute n = p * q
- Compute φ(n) = (p-1) * (q-1)
- Choose an integer e such that 1 < e < φ(n) and gcd(e, φ(n)) = 1
- Compute d to satisfy the congruence relation de ≡ 1 (mod φ(n))
- Encryption: c = m^e mod n, where m is the plaintext message
- Decryption: m = c^d mod n
Here’s a simple implementation of RSA in Python:
import random
def is_prime(n, k=5):
if n < 2: return False
for p in [2,3,5,7,11,13,17,19,23,29]:
if n % p == 0: return n == p
s, d = 0, n-1
while d % 2 == 0:
s, d = s+1, d//2
for i in range(k):
x = pow(random.randint(2, n-1), d, n)
if x == 1 or x == n-1: continue
for r in range(s-1):
x = pow(x, 2, n)
if x == n-1: break
else: return False
return True
def generate_prime(bits):
while True:
p = random.getrandbits(bits)
if is_prime(p):
return p
def generate_keypair(bits):
p = generate_prime(bits)
q = generate_prime(bits)
n = p * q
phi = (p-1) * (q-1)
e = 65537
d = pow(e, -1, phi)
return ((e, n), (d, n))
def encrypt(pk, plaintext):
e, n = pk
cipher = [pow(ord(char), e, n) for char in plaintext]
return cipher
def decrypt(pk, ciphertext):
d, n = pk
plain = [chr(pow(char, d, n)) for char in ciphertext]
return ''.join(plain)
# Generate keypair
public, private = generate_keypair(1024)
# Encrypt and decrypt a message
message = "Hello, RSA!"
encrypted = encrypt(public, message)
decrypted = decrypt(private, encrypted)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
3.2 Elliptic Curve Cryptography (ECC)
ECC is a more recent approach to public-key cryptography based on the algebraic structure of elliptic curves over finite fields. It offers equivalent security to RSA with much smaller key sizes, making it particularly suitable for resource-constrained environments.
The security of ECC is based on the elliptic curve discrete logarithm problem (ECDLP), which is believed to be more difficult than the integer factorization problem used in RSA.
Key components of ECC:
- Elliptic curve: Defined by an equation of the form y^2 = x^3 + ax + b
- Base point: A point on the curve that generates a subgroup
- Private key: A randomly selected integer
- Public key: The result of multiplying the base point by the private key
ECC encryption typically involves these steps:
- Choose a random number k
- Compute the point R = k * G, where G is the base point
- Compute the shared secret S = k * Q, where Q is the recipient’s public key
- Use a key derivation function to derive an encryption key from S
- Encrypt the message using a symmetric encryption algorithm with the derived key
4. Hash Functions in Cryptography
While not encryption algorithms per se, cryptographic hash functions play a crucial role in many security protocols and are often used in conjunction with encryption algorithms.
4.1 SHA-256 (Secure Hash Algorithm 256-bit)
SHA-256 is part of the SHA-2 family of cryptographic hash functions, designed by the U.S. National Security Agency (NSA). It produces a 256-bit (32-byte) hash value, typically rendered as a 64-digit hexadecimal number.
Key features of SHA-256:
- One-way function: It’s computationally infeasible to reverse the hash to obtain the original input
- Deterministic: The same input always produces the same hash
- Avalanche effect: A small change in the input results in a significantly different hash
- Collision-resistant: It’s extremely unlikely to find two different inputs that produce the same hash
Here’s an example of using SHA-256 in Python:
import hashlib
def sha256_hash(input_string):
return hashlib.sha256(input_string.encode()).hexdigest()
# Example usage
message = "Hello, World!"
hashed = sha256_hash(message)
print(f"Original message: {message}")
print(f"SHA-256 hash: {hashed}")
5. Encryption Modes
Encryption modes define how the encryption algorithm is applied to data, especially when dealing with multiple blocks of data. Some common modes include:
5.1 Electronic Codebook (ECB)
ECB is the simplest mode, where each block of plaintext is encrypted independently. While easy to implement, it’s generally not recommended for most applications due to its vulnerability to certain types of attacks.
5.2 Cipher Block Chaining (CBC)
In CBC mode, each block of plaintext is XORed with the previous ciphertext block before being encrypted. This creates a dependency between blocks, making the encryption more secure than ECB.
5.3 Galois/Counter Mode (GCM)
GCM is a mode of operation for symmetric key cryptographic block ciphers that provides authenticated encryption. It’s widely used due to its performance and security properties.
6. Practical Applications of Encryption Algorithms
6.1 Secure Communication Protocols
Encryption algorithms form the backbone of secure communication protocols such as:
- HTTPS (HTTP Secure): Uses TLS/SSL, which typically employs a combination of asymmetric encryption (for key exchange) and symmetric encryption (for data transfer)
- SSH (Secure Shell): Uses various encryption algorithms to secure remote login and other network services
- VPNs (Virtual Private Networks): Utilize encryption to create secure tunnels over public networks
6.2 File and Disk Encryption
Encryption algorithms are used to protect data at rest:
- Full-disk encryption: Protects all data on a storage device
- File-level encryption: Encrypts individual files or folders
- Database encryption: Protects sensitive data within databases
6.3 Digital Signatures
Asymmetric encryption algorithms are used to create digital signatures, which provide authentication, integrity, and non-repudiation for digital documents and transactions.
7. Quantum Cryptography and Post-Quantum Algorithms
As quantum computers advance, they pose a potential threat to many current encryption algorithms, particularly those based on integer factorization and discrete logarithm problems. This has led to the development of quantum-resistant or post-quantum cryptographic algorithms.
7.1 Lattice-based Cryptography
Lattice-based cryptography is one of the most promising approaches for post-quantum cryptography. It’s based on the hardness of certain lattice problems, which are believed to be difficult even for quantum computers.
7.2 Hash-based Signatures
These are digital signature schemes that only rely on the security of cryptographic hash functions, making them resistant to attacks by quantum computers.
8. Implementing Encryption in Programming
When implementing encryption in your programs, it’s crucial to use well-vetted libraries rather than trying to implement the algorithms yourself. Here are some popular cryptographic libraries for different programming languages:
- Python: PyCryptodome, cryptography
- Java: Java Cryptography Architecture (JCA), Bouncy Castle
- JavaScript: Web Crypto API, Node.js crypto module
- C++: OpenSSL, Crypto++
Here’s an example of using the Web Crypto API in JavaScript to perform AES encryption:
async function encryptAES(plaintext, key) {
const encoder = new TextEncoder();
const data = encoder.encode(plaintext);
const iv = crypto.getRandomValues(new Uint8Array(12));
const alg = { name: 'AES-GCM', iv: iv };
const cryptoKey = await crypto.subtle.importKey('raw', key, alg, false, ['encrypt']);
const ciphertext = await crypto.subtle.encrypt(alg, cryptoKey, data);
return { ciphertext, iv };
}
async function decryptAES(ciphertext, key, iv) {
const alg = { name: 'AES-GCM', iv: iv };
const cryptoKey = await crypto.subtle.importKey('raw', key, alg, false, ['decrypt']);
const plainBuffer = await crypto.subtle.decrypt(alg, cryptoKey, ciphertext);
const decoder = new TextDecoder();
return decoder.decode(plainBuffer);
}
// Example usage
(async () => {
const key = await crypto.subtle.generateKey(
{ name: 'AES-GCM', length: 256 },
true,
['encrypt', 'decrypt']
);
const message = 'Secret message';
const { ciphertext, iv } = await encryptAES(message, key);
const decrypted = await decryptAES(ciphertext, key, iv);
console.log(`Original: ${message}`);
console.log(`Encrypted: ${new Uint8Array(ciphertext)}`);
console.log(`Decrypted: ${decrypted}`);
})();
9. Best Practices in Encryption
When working with encryption, it’s important to follow these best practices:
- Use strong, randomly generated keys
- Implement proper key management (secure storage, rotation, and destruction)
- Use authenticated encryption modes (like AES-GCM) when possible
- Keep encryption algorithms and implementations up to date
- Don’t roll your own cryptography – use well-vetted libraries and protocols
- Understand and properly implement initialization vectors (IVs) and nonces
- Use appropriate key sizes (e.g., at least 256 bits for symmetric encryption)
- Implement perfect forward secrecy in communication protocols
Conclusion
The algorithms behind modern encryption techniques form the foundation of digital security in our interconnected world. From symmetric ciphers like AES to asymmetric systems like RSA and ECC, these mathematical constructs protect our data, communications, and digital identities.
As technology evolves, so too must our encryption methods. The looming threat of quantum computing is driving innovation in post-quantum cryptography, ensuring that our digital lives remain secure in the face of advancing computational power.
For developers and IT professionals, a solid understanding of these encryption algorithms and their proper implementation is crucial. By leveraging well-tested libraries and following best practices, we can harness the power of these algorithms to build secure, robust systems that protect sensitive data and maintain user trust.
As we continue to push the boundaries of technology, the importance of strong encryption will only grow. By staying informed about the latest developments in cryptography and implementing them thoughtfully, we can ensure that our digital future remains secure and private.