The Art of Algorithm Optimization: Mastering Efficiency in Code
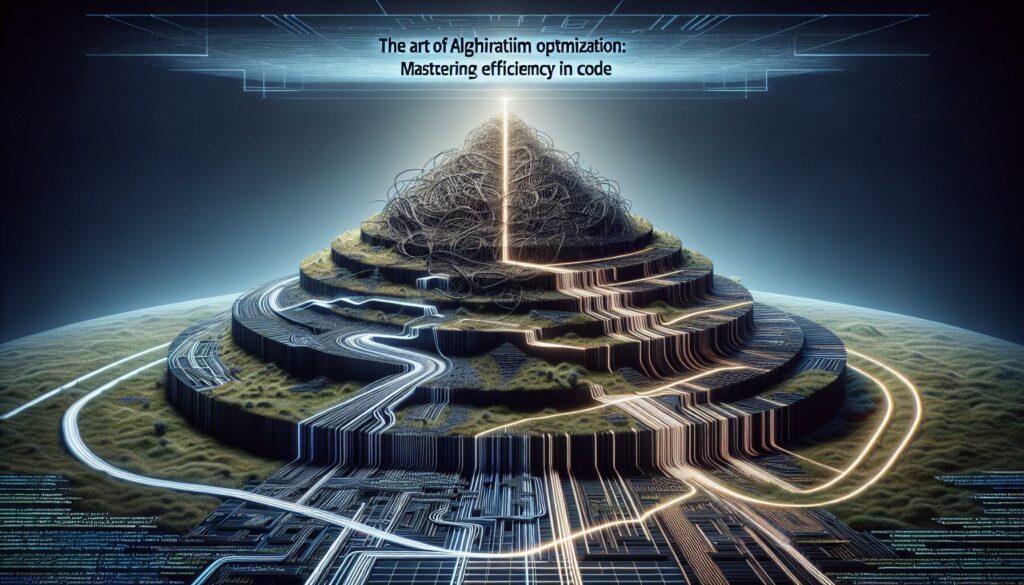
In the world of programming, writing code that works is just the beginning. The true art lies in crafting efficient, optimized algorithms that can handle large-scale problems with grace and speed. Algorithm optimization is a crucial skill for any programmer, especially those aiming to excel in technical interviews at top tech companies. In this comprehensive guide, we’ll dive deep into the art of algorithm optimization, exploring techniques, best practices, and real-world examples to help you elevate your coding skills.
Understanding Algorithm Optimization
Before we delve into specific techniques, it’s essential to understand what algorithm optimization means and why it’s important.
What is Algorithm Optimization?
Algorithm optimization is the process of improving the efficiency of an algorithm in terms of time complexity, space complexity, or both. It involves refining your code to perform tasks faster, use less memory, or ideally, achieve both simultaneously.
Why is Optimization Important?
In an era where data volumes are exploding and real-time processing is often required, optimized algorithms can make the difference between a system that scales gracefully and one that buckles under pressure. Moreover, companies like Google, Amazon, and Facebook place a high premium on candidates who can write efficient code, making optimization skills crucial for acing technical interviews.
Key Concepts in Algorithm Optimization
To master algorithm optimization, you need to be familiar with several fundamental concepts:
1. Time Complexity
Time complexity is a measure of how the runtime of an algorithm grows as the input size increases. It’s typically expressed using Big O notation, which describes the worst-case scenario for the algorithm’s performance.
2. Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s often expressed in Big O notation.
3. Trade-offs
Optimization often involves trade-offs. You might need to sacrifice some space efficiency to gain time efficiency, or vice versa. Understanding these trade-offs is crucial for making informed decisions about optimization strategies.
Common Optimization Techniques
Now that we’ve covered the basics, let’s explore some widely-used optimization techniques:
1. Memoization
Memoization is a technique used to speed up programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. It’s particularly useful in recursive algorithms and dynamic programming problems.
Here’s a simple example of memoization in Python for calculating Fibonacci numbers:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
print(fibonacci(100)) # This would be extremely slow without memoization
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used to optimize recursive algorithms that have overlapping subproblems.
Here’s an example of dynamic programming to solve the classic “Coin Change” problem:
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
coins = [1, 2, 5]
amount = 11
print(coin_change(coins, amount)) # Output: 3 (5 + 5 + 1)
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. While they don’t always produce the best solution, they’re often fast and can be a good approximation in many scenarios.
Here’s a simple example of a greedy algorithm for the “Activity Selection” problem:
def activity_selection(start, finish):
n = len(start)
activities = sorted(zip(start, finish), key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for i in range(1, n):
if activities[i][0] >= last_finish:
selected.append(activities[i])
last_finish = activities[i][1]
return selected
start = [1, 3, 0, 5, 8, 5]
finish = [2, 4, 6, 7, 9, 9]
print(activity_selection(start, finish)) # Output: [(1, 2), (3, 4), (5, 7), (8, 9)]
4. Binary Search
Binary search is a highly efficient algorithm for searching sorted arrays. It repeatedly divides the search interval in half, reducing the time complexity from O(n) to O(log n).
Here’s an implementation of binary search:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
print(binary_search(arr, target)) # Output: 3
5. Two-Pointer Technique
The two-pointer technique is a way to solve problems with less time complexity. It’s often used in array or string problems where you need to find a pair of elements that satisfy certain conditions.
Here’s an example of using two pointers to find a pair of numbers in a sorted array that sum to a target:
def two_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return []
arr = [2, 7, 11, 15]
target = 9
print(two_sum(arr, target)) # Output: [2, 7]
Advanced Optimization Techniques
As you progress in your optimization journey, you’ll encounter more advanced techniques:
1. Bit Manipulation
Bit manipulation can lead to significant optimizations in certain scenarios, especially when dealing with low-level operations or when space efficiency is crucial.
Here’s an example of using bit manipulation to check if a number is a power of two:
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
print(is_power_of_two(16)) # Output: True
print(is_power_of_two(18)) # Output: False
2. Sliding Window
The sliding window technique is used to perform operations on arrays or strings, typically to find subarrays that satisfy certain conditions. It can reduce time complexity from O(n²) to O(n) in many cases.
Here’s an example of using sliding window to find the maximum sum subarray of size k:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(n - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray(arr, k)) # Output: 39
3. Divide and Conquer
Divide and conquer is a paradigm that works by recursively breaking down a problem into smaller subproblems until these become simple enough to be solved directly. The solutions to the subproblems are then combined to give a solution to the original problem.
Here’s an example of using divide and conquer to implement merge sort:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
arr = [38, 27, 43, 3, 9, 82, 10]
print(merge_sort(arr)) # Output: [3, 9, 10, 27, 38, 43, 82]
Best Practices for Algorithm Optimization
While knowing various techniques is important, following best practices can make your optimization efforts more effective:
1. Profile Before Optimizing
Before you start optimizing, use profiling tools to identify the actual bottlenecks in your code. As Donald Knuth famously said, “Premature optimization is the root of all evil.”
2. Understand the Problem Domain
A deep understanding of the problem domain can often lead to more effective optimizations than generic techniques. Sometimes, changing the algorithm entirely based on domain knowledge can yield better results than optimizing an inefficient algorithm.
3. Choose the Right Data Structures
Selecting appropriate data structures can have a significant impact on performance. For example, using a hash table instead of an array can reduce lookup time from O(n) to O(1) in many cases.
4. Write Clean, Readable Code First
Start by writing clear, correct code. Once it’s working, you can optimize it. This approach makes it easier to understand and maintain your code, and often, clean code is naturally more efficient.
5. Use Built-in Functions and Libraries
Many programming languages and libraries offer highly optimized implementations of common algorithms and data structures. Use these when possible instead of reinventing the wheel.
6. Consider Space-Time Trade-offs
Sometimes, using more memory can significantly speed up an algorithm. Other times, you might need to sacrifice some speed to reduce memory usage. Always consider these trade-offs in the context of your specific requirements.
Real-World Optimization Scenarios
Let’s look at some real-world scenarios where algorithm optimization can make a significant difference:
1. Database Query Optimization
In database systems, query optimization is crucial for performance. This might involve:
- Using appropriate indexes
- Rewriting complex queries
- Denormalizing data for read-heavy workloads
2. Web Application Performance
For web applications, optimization often focuses on:
- Minimizing database queries
- Implementing caching strategies
- Optimizing front-end assets (CSS, JavaScript, images)
3. Machine Learning Model Optimization
In machine learning, optimization can involve:
- Feature selection and engineering
- Hyperparameter tuning
- Model compression for deployment on resource-constrained devices
4. Game Development
In game development, optimization is often critical for maintaining smooth gameplay:
- Implementing efficient collision detection algorithms
- Optimizing rendering pipelines
- Using spatial data structures for fast object lookup
Tools for Algorithm Optimization
Several tools can assist you in your optimization efforts:
1. Profilers
Profilers help you identify bottlenecks in your code by measuring the time and memory usage of different parts of your program. Examples include:
- cProfile for Python
- Java VisualVM for Java
- Chrome DevTools for JavaScript
2. Benchmarking Tools
Benchmarking tools allow you to measure and compare the performance of different algorithms or implementations. Examples include:
- Benchmark.js for JavaScript
- Google Benchmark for C++
- Criterion for Rust
3. Code Analysis Tools
Static code analysis tools can help identify potential performance issues before you even run your code. Examples include:
- Pylint for Python
- ESLint for JavaScript
- SonarQube for multiple languages
Conclusion: The Ongoing Journey of Optimization
Algorithm optimization is not a one-time task but an ongoing process of refinement and improvement. As you gain more experience, you’ll develop an intuition for where optimizations are likely to yield the most significant benefits. You’ll also learn to balance the trade-offs between different optimization strategies and to consider factors beyond just time and space complexity, such as code readability and maintainability.
Remember, the goal of optimization is not just to make code run faster or use less memory, but to create efficient, scalable solutions to real-world problems. As you practice and apply these techniques, you’ll not only become better prepared for technical interviews at top tech companies but also develop the skills to build high-performance systems that can handle the challenges of modern computing.
Keep experimenting, keep learning, and never stop optimizing. The art of algorithm optimization is a lifelong journey that will continually challenge and reward you throughout your programming career.