Balancing Trees: AVL vs. Red-Black Trees
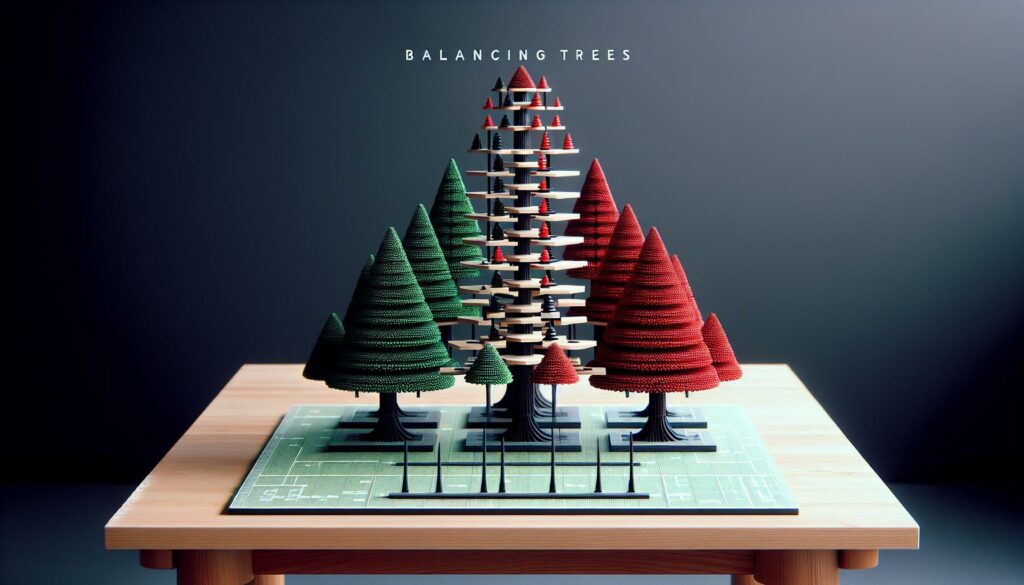
In the world of data structures and algorithms, balanced trees play a crucial role in maintaining efficient operations, especially when dealing with large datasets. Two of the most popular self-balancing binary search tree structures are AVL trees and Red-Black trees. Both of these structures aim to keep the tree balanced, ensuring that operations like insertion, deletion, and search remain efficient, even as the tree grows. In this comprehensive guide, we’ll dive deep into the world of AVL and Red-Black trees, exploring their similarities, differences, and use cases.
Understanding Self-Balancing Trees
Before we delve into the specifics of AVL and Red-Black trees, it’s essential to understand the concept of self-balancing trees and why they’re important in computer science and software engineering.
A self-balancing tree is a type of binary search tree that automatically keeps its height (maximal number of levels below the root) small in the face of arbitrary insertions and deletions. These structures are designed to prevent the tree from becoming skewed or unbalanced, which can lead to degraded performance in basic operations like search, insert, and delete.
The primary goal of self-balancing trees is to ensure that the tree remains approximately balanced at all times, maintaining a height of O(log n), where n is the number of nodes in the tree. This logarithmic height guarantee ensures that basic operations can be performed in O(log n) time, which is crucial for maintaining efficiency in large-scale applications.
AVL Trees: Strictly Balanced
AVL trees, named after their inventors Adelson-Velsky and Landis, were the first self-balancing binary search trees to be invented. Let’s explore the key characteristics and operations of AVL trees.
Balance Factor
The core concept in AVL trees is the balance factor. For each node in an AVL tree, the heights of the left and right subtrees can differ by at most one. This difference is called the balance factor:
Balance Factor = Height(Left Subtree) - Height(Right Subtree)
In a valid AVL tree, the balance factor for every node must be -1, 0, or 1. If at any time the balance factor becomes less than -1 or greater than 1, the tree must be rebalanced through rotations.
Rotations in AVL Trees
AVL trees use four types of rotations to maintain balance:
- Left Rotation
- Right Rotation
- Left-Right Rotation
- Right-Left Rotation
These rotations are performed after insertions or deletions that cause the tree to become unbalanced. Let’s look at a simple example of a right rotation:
A B
/ \ / \
B C => D A
/ \ / \
D E E C
Insertion in AVL Trees
When inserting a new node into an AVL tree, we follow these steps:
- Perform standard BST insertion
- Update height of ancestors
- Check balance factor of ancestors
- If unbalanced, perform appropriate rotation(s)
Here’s a Python implementation of the AVL tree insertion:
class AVLNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def __init__(self):
self.root = None
def height(self, node):
if not node:
return 0
return node.height
def balance_factor(self, node):
if not node:
return 0
return self.height(node.left) - self.height(node.right)
def update_height(self, node):
if not node:
return
node.height = 1 + max(self.height(node.left), self.height(node.right))
def right_rotate(self, y):
x = y.left
T2 = x.right
x.right = y
y.left = T2
self.update_height(y)
self.update_height(x)
return x
def left_rotate(self, x):
y = x.right
T2 = y.left
y.left = x
x.right = T2
self.update_height(x)
self.update_height(y)
return y
def insert(self, root, key):
if not root:
return AVLNode(key)
if key < root.key:
root.left = self.insert(root.left, key)
else:
root.right = self.insert(root.right, key)
self.update_height(root)
balance = self.balance_factor(root)
# Left Left Case
if balance > 1 and key < root.left.key:
return self.right_rotate(root)
# Right Right Case
if balance < -1 and key > root.right.key:
return self.left_rotate(root)
# Left Right Case
if balance > 1 and key > root.left.key:
root.left = self.left_rotate(root.left)
return self.right_rotate(root)
# Right Left Case
if balance < -1 and key < root.right.key:
root.right = self.right_rotate(root.right)
return self.left_rotate(root)
return root
def insert_key(self, key):
self.root = self.insert(self.root, key)
Deletion in AVL Trees
Deletion in AVL trees follows a similar process to insertion:
- Perform standard BST deletion
- Update height of ancestors
- Check balance factor of ancestors
- If unbalanced, perform appropriate rotation(s)
The deletion operation is more complex than insertion because a deletion can cause imbalances further up the tree.
Red-Black Trees: Approximately Balanced
Red-Black trees are another type of self-balancing binary search tree, but they use a different approach to maintain balance. Instead of strictly balancing the tree like AVL trees, Red-Black trees aim for approximate balance, which can lead to faster insertion and deletion operations in practice.
Properties of Red-Black Trees
A Red-Black tree must satisfy the following properties:
- Every node is either red or black.
- The root is always black.
- Every leaf (NIL) is black.
- If a node is red, then both its children are black.
- For each node, all simple paths from the node to descendant leaves contain the same number of black nodes.
These properties ensure that the tree remains approximately balanced. The longest path from the root to a leaf is no more than twice as long as the shortest path, guaranteeing O(log n) time for basic operations.
Rotations and Color Changes
Red-Black trees use a combination of rotations (similar to AVL trees) and color changes to maintain their properties. The specific operations used depend on the “case” encountered during insertion or deletion.
Insertion in Red-Black Trees
When inserting a new node into a Red-Black tree, we follow these steps:
- Perform standard BST insertion
- Color the new node red
- Fix any violations of Red-Black properties
Here’s a Python implementation of Red-Black tree insertion:
class RBNode:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.parent = None
self.color = 1 # 1 for red, 0 for black
class RedBlackTree:
def __init__(self):
self.TNULL = RBNode(0)
self.TNULL.color = 0
self.TNULL.left = None
self.TNULL.right = None
self.root = self.TNULL
def insert(self, key):
node = RBNode(key)
node.parent = None
node.left = self.TNULL
node.right = self.TNULL
node.color = 1 # new node must be red
y = None
x = self.root
while x != self.TNULL:
y = x
if node.key < x.key:
x = x.left
else:
x = x.right
node.parent = y
if y == None:
self.root = node
elif node.key < y.key:
y.left = node
else:
y.right = node
if node.parent == None:
node.color = 0
return
if node.parent.parent == None:
return
self.fix_insert(node)
def fix_insert(self, k):
while k.parent.color == 1:
if k.parent == k.parent.parent.right:
u = k.parent.parent.left
if u.color == 1:
u.color = 0
k.parent.color = 0
k.parent.parent.color = 1
k = k.parent.parent
else:
if k == k.parent.left:
k = k.parent
self.right_rotate(k)
k.parent.color = 0
k.parent.parent.color = 1
self.left_rotate(k.parent.parent)
else:
u = k.parent.parent.right
if u.color == 1:
u.color = 0
k.parent.color = 0
k.parent.parent.color = 1
k = k.parent.parent
else:
if k == k.parent.right:
k = k.parent
self.left_rotate(k)
k.parent.color = 0
k.parent.parent.color = 1
self.right_rotate(k.parent.parent)
if k == self.root:
break
self.root.color = 0
def left_rotate(self, x):
y = x.right
x.right = y.left
if y.left != self.TNULL:
y.left.parent = x
y.parent = x.parent
if x.parent == None:
self.root = y
elif x == x.parent.left:
x.parent.left = y
else:
x.parent.right = y
y.left = x
x.parent = y
def right_rotate(self, x):
y = x.left
x.left = y.right
if y.right != self.TNULL:
y.right.parent = x
y.parent = x.parent
if x.parent == None:
self.root = y
elif x == x.parent.right:
x.parent.right = y
else:
x.parent.left = y
y.right = x
x.parent = y
Deletion in Red-Black Trees
Deletion in Red-Black trees is more complex than insertion and involves several cases to handle. The process involves:
- Perform standard BST deletion
- If the deleted node was red, we’re done
- If the deleted node was black, we need to fix violations of Red-Black properties
Comparing AVL and Red-Black Trees
Now that we’ve explored both AVL and Red-Black trees, let’s compare their characteristics and performance:
Balance
AVL trees maintain a stricter balance, with the heights of subtrees differing by at most one. Red-Black trees allow for a more relaxed balance, where the longest path from root to leaf is no more than twice the length of the shortest path.
Height
AVL trees typically have a lower height compared to Red-Black trees. The height of an AVL tree with n nodes is bounded by approximately 1.44 log n, while for Red-Black trees, it’s bounded by 2 log n.
Insertion and Deletion
Red-Black trees generally perform faster insertions and deletions, as they require fewer rotations on average. AVL trees might require more rotations to maintain their stricter balance.
Search Performance
Due to their lower height, AVL trees can provide slightly faster search operations compared to Red-Black trees.
Memory Usage
Red-Black trees require an extra bit per node to store the color, while AVL trees typically store balance factors or heights. In practice, this difference is usually negligible.
Use Cases
AVL trees are preferred in scenarios where lookup operations are more frequent than insertions and deletions. Examples include in-memory databases or systems that perform many searches.
Red-Black trees are often used in scenarios with frequent insertions and deletions, such as in the implementation of associative arrays in many programming language standard libraries.
Implementing Balancing Trees in Practice
When implementing balanced trees in real-world applications, several factors should be considered:
Language and Library Support
Many programming languages provide built-in implementations of balanced trees. For example:
- C++: std::map and std::set are typically implemented as Red-Black trees
- Java: java.util.TreeMap and java.util.TreeSet use Red-Black trees
- Python: While Python’s built-in dict is not a balanced tree, the sortedcontainers library provides SortedDict and SortedSet implementations
Custom Implementations
If you need to implement a balanced tree yourself, consider the following tips:
- Start with a clear understanding of the tree’s properties and invariants
- Implement and thoroughly test basic BST operations before adding balancing logic
- Use recursive implementations for simplicity, but be aware of potential stack overflow issues with very large trees
- Consider using sentinel nodes (like TNULL in our Red-Black tree example) to simplify edge cases
- Implement comprehensive unit tests, especially for edge cases and rebalancing scenarios
Performance Considerations
When working with large datasets, consider the following performance aspects:
- Memory usage: For very large trees, consider using external storage or memory-mapped files
- Concurrency: If multiple threads need to access the tree, consider using concurrent versions of balanced trees or appropriate locking mechanisms
- Bulk operations: For large batches of insertions or deletions, consider specialized bulk update algorithms that can be more efficient than individual operations
Conclusion
Balanced trees, particularly AVL and Red-Black trees, are fundamental data structures in computer science and software engineering. They provide efficient solutions for maintaining sorted data with logarithmic-time operations, making them invaluable in numerous applications.
While AVL trees offer slightly better search performance due to their stricter balance, Red-Black trees often perform better in scenarios with frequent modifications. The choice between the two depends on the specific requirements of your application and the expected usage patterns.
As you continue your journey in algorithm and data structure mastery, understanding these balanced tree structures will prove invaluable. They not only serve as powerful tools in your programming toolkit but also provide insights into the trade-offs and design decisions involved in creating efficient data structures.
Remember, the key to mastering these concepts is practice. Implement both AVL and Red-Black trees from scratch, experiment with different datasets, and analyze their performance in various scenarios. This hands-on experience will deepen your understanding and prepare you for tackling complex problems in your coding journey and technical interviews.