Combinatorial Algorithms in Computer Science: Mastering the Art of Efficient Problem-Solving
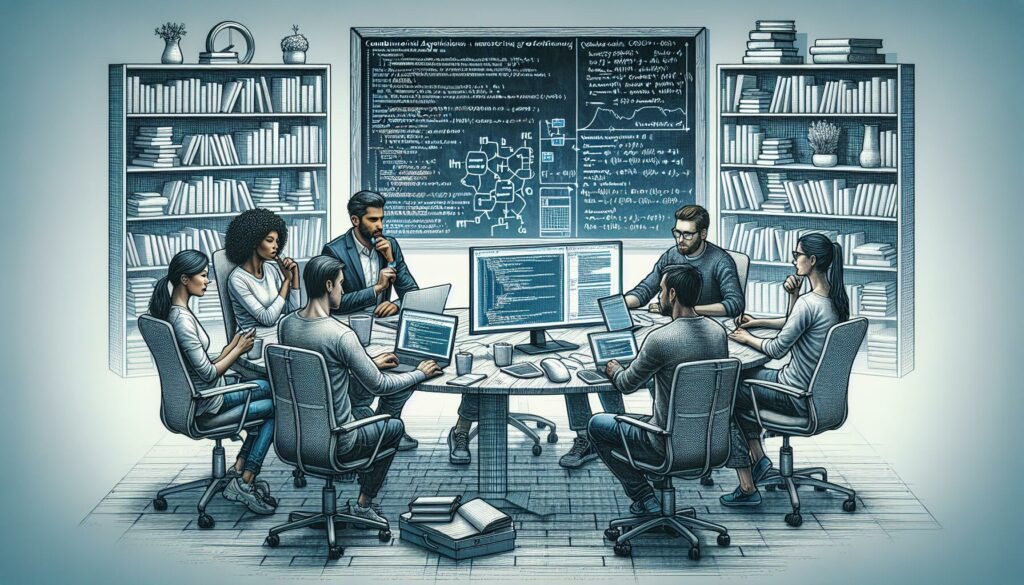
In the vast realm of computer science, combinatorial algorithms play a pivotal role in solving complex problems efficiently. These algorithms are essential for tackling optimization challenges, data analysis, and various computational tasks. As aspiring programmers and seasoned developers alike strive to enhance their skills, understanding combinatorial algorithms becomes crucial for excelling in technical interviews and real-world applications. In this comprehensive guide, we’ll delve deep into the world of combinatorial algorithms, exploring their principles, applications, and implementation techniques.
What are Combinatorial Algorithms?
Combinatorial algorithms are a class of algorithms designed to solve problems involving discrete structures, such as graphs, sets, and arrays. These algorithms focus on finding optimal solutions by exploring combinations and permutations of elements within a given problem space. The primary goal of combinatorial algorithms is to efficiently search through a large number of possibilities to find the best solution or to determine if a solution exists at all.
Some key characteristics of combinatorial algorithms include:
- Dealing with discrete mathematics and finite sets
- Exploring different combinations and arrangements of elements
- Optimizing solutions for complex problems
- Balancing between exhaustive search and heuristic approaches
Types of Combinatorial Algorithms
Combinatorial algorithms encompass a wide range of techniques and approaches. Let’s explore some of the most common types:
1. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. While they don’t always guarantee the best solution, they are often efficient and provide good approximations.
Example: Kruskal’s algorithm for finding the minimum spanning tree of a graph.
function kruskalMST(graph):
edges = sortEdgesByWeight(graph)
mst = []
disjointSet = DisjointSet(graph.vertices)
for edge in edges:
if disjointSet.find(edge.src) != disjointSet.find(edge.dest):
mst.append(edge)
disjointSet.union(edge.src, edge.dest)
return mst
2. Dynamic Programming
Dynamic programming breaks down complex problems into smaller subproblems, solving each subproblem only once and storing the results for future use. This approach is particularly useful for optimization problems with overlapping subproblems.
Example: Fibonacci sequence calculation using dynamic programming.
function fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
3. Backtracking Algorithms
Backtracking algorithms explore all possible solutions by incrementally building candidates and abandoning those that fail to satisfy the problem constraints. This approach is useful for solving puzzles, constraint satisfaction problems, and combinatorial optimization tasks.
Example: N-Queens problem solution using backtracking.
function solveNQueens(n):
board = [['.'] * n for _ in range(n)]
solutions = []
def backtrack(row):
if row == n:
solutions.append([''.join(row) for row in board])
return
for col in range(n):
if isValid(row, col):
board[row][col] = 'Q'
backtrack(row + 1)
board[row][col] = '.'
def isValid(row, col):
# Check column
for i in range(row):
if board[i][col] == 'Q':
return False
# Check upper-left diagonal
for i, j in zip(range(row-1, -1, -1), range(col-1, -1, -1)):
if board[i][j] == 'Q':
return False
# Check upper-right diagonal
for i, j in zip(range(row-1, -1, -1), range(col+1, n)):
if board[i][j] == 'Q':
return False
return True
backtrack(0)
return solutions
4. Branch and Bound Algorithms
Branch and bound algorithms systematically enumerate candidate solutions by means of state space search. They use bounding functions to discard subsets of fruitless candidates, improving efficiency in finding optimal solutions.
Example: Traveling Salesman Problem using branch and bound.
function tspBranchAndBound(graph):
n = len(graph)
best_path = None
best_cost = float('inf')
def bound(path, cost):
# Implement a lower bound estimation
return cost
def branch(path, cost):
nonlocal best_path, best_cost
if len(path) == n:
if cost + graph[path[-1]][path[0]] < best_cost:
best_cost = cost + graph[path[-1]][path[0]]
best_path = path + [path[0]]
return
for next_city in range(n):
if next_city not in path:
new_cost = cost + graph[path[-1]][next_city]
if bound(path + [next_city], new_cost) < best_cost:
branch(path + [next_city], new_cost)
branch([0], 0)
return best_path, best_cost
Applications of Combinatorial Algorithms
Combinatorial algorithms find applications in various domains of computer science and real-world scenarios. Some notable applications include:
1. Graph Theory
Combinatorial algorithms are extensively used in graph theory for solving problems such as:
- Shortest path finding (e.g., Dijkstra’s algorithm)
- Minimum spanning tree construction (e.g., Prim’s algorithm)
- Graph coloring
- Maximum flow in networks
2. Optimization Problems
Many optimization problems rely on combinatorial algorithms to find optimal or near-optimal solutions:
- Knapsack problem
- Traveling Salesman Problem
- Job scheduling
- Resource allocation
3. Cryptography
Combinatorial algorithms play a crucial role in various aspects of cryptography:
- Key generation
- Cryptanalysis
- Hash function design
4. Bioinformatics
In the field of bioinformatics, combinatorial algorithms are used for:
- DNA sequence alignment
- Protein folding prediction
- Genome assembly
5. Machine Learning
Combinatorial algorithms contribute to various machine learning tasks:
- Feature selection
- Decision tree construction
- Ensemble methods
Implementing Combinatorial Algorithms: Best Practices
When implementing combinatorial algorithms, it’s essential to follow best practices to ensure efficiency and correctness. Here are some key considerations:
1. Choose the Right Data Structures
Selecting appropriate data structures can significantly impact the performance of combinatorial algorithms. Common data structures used include:
- Arrays and matrices for graph representation
- Priority queues for greedy algorithms
- Hash tables for efficient lookups
- Disjoint set data structures for union-find operations
2. Optimize for Time and Space Complexity
Analyze the time and space complexity of your algorithms and look for opportunities to optimize:
- Use memoization or tabulation in dynamic programming
- Implement efficient pruning techniques in backtracking algorithms
- Utilize bit manipulation for space-efficient set operations
3. Handle Edge Cases
Ensure your implementations handle various edge cases and input scenarios:
- Empty inputs
- Single-element inputs
- Maximum and minimum possible values
- Invalid inputs
4. Implement Robust Testing
Develop comprehensive test cases to validate the correctness of your algorithms:
- Unit tests for individual functions
- Integration tests for complex algorithms
- Stress tests with large inputs
- Randomized testing for edge cases
5. Document and Comment Your Code
Proper documentation and commenting are crucial for understanding and maintaining combinatorial algorithms:
- Provide clear function descriptions
- Explain the algorithm’s logic and key steps
- Document time and space complexity
- Include usage examples
Advanced Techniques in Combinatorial Algorithms
As you progress in your understanding of combinatorial algorithms, consider exploring these advanced techniques:
1. Approximation Algorithms
For NP-hard problems, approximation algorithms provide near-optimal solutions in polynomial time. These algorithms are crucial when exact solutions are impractical due to time constraints.
Example: Approximation algorithm for the Vertex Cover problem.
function approximateVertexCover(graph):
vertex_cover = set()
edges = list(graph.edges())
while edges:
u, v = edges.pop()
if u not in vertex_cover and v not in vertex_cover:
vertex_cover.add(u)
vertex_cover.add(v)
return vertex_cover
2. Randomized Algorithms
Randomized algorithms incorporate random choices to achieve good average-case performance. They are often simpler and more efficient than deterministic alternatives for certain problems.
Example: Randomized Quicksort algorithm.
import random
def randomized_quicksort(arr):
if len(arr) <= 1:
return arr
pivot = random.choice(arr)
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return randomized_quicksort(left) + middle + randomized_quicksort(right)
3. Parameterized Algorithms
Parameterized algorithms solve hard problems efficiently for small parameter values, even if the input size is large. This approach is useful for problems that are NP-hard in general but become tractable for specific parameter ranges.
Example: Parameterized algorithm for the Vertex Cover problem.
def vertex_cover_parameterized(graph, k):
if k < 0:
return None
if not graph.edges():
return set()
u, v = next(iter(graph.edges()))
# Try including u in the vertex cover
graph_without_u = graph.copy()
graph_without_u.remove_node(u)
vc_with_u = vertex_cover_parameterized(graph_without_u, k - 1)
if vc_with_u is not None:
return vc_with_u.union({u})
# Try including v in the vertex cover
graph_without_v = graph.copy()
graph_without_v.remove_node(v)
vc_with_v = vertex_cover_parameterized(graph_without_v, k - 1)
if vc_with_v is not None:
return vc_with_v.union({v})
return None
Preparing for Technical Interviews
Mastering combinatorial algorithms is crucial for success in technical interviews, especially when targeting positions at major tech companies. Here are some tips to help you prepare:
1. Practice Problem-Solving
Regularly solve problems on platforms like LeetCode, HackerRank, and CodeForces. Focus on problems that involve combinatorial algorithms, such as:
- Graph traversal and shortest path problems
- Dynamic programming challenges
- Backtracking puzzles
- Greedy algorithm applications
2. Analyze Time and Space Complexity
For each problem you solve, practice analyzing the time and space complexity of your solution. Be prepared to discuss trade-offs between different approaches and optimize your code for efficiency.
3. Implement from Scratch
Practice implementing common combinatorial algorithms from scratch, without relying on built-in libraries. This will deepen your understanding of the algorithms and improve your coding skills.
4. Study Algorithm Design Paradigms
Familiarize yourself with various algorithm design paradigms, such as divide-and-conquer, dynamic programming, and greedy approaches. Understand when and how to apply these paradigms to solve different types of problems.
5. Mock Interviews
Participate in mock interviews to simulate the pressure of a real technical interview. Practice explaining your thought process, discussing trade-offs, and optimizing your solutions on the spot.
Conclusion
Combinatorial algorithms form the backbone of efficient problem-solving in computer science. By mastering these algorithms, you’ll be well-equipped to tackle complex challenges in various domains and excel in technical interviews. Remember that proficiency in combinatorial algorithms comes with practice and persistent effort.
As you continue your journey in coding education and skills development, focus on understanding the underlying principles of combinatorial algorithms, implementing them from scratch, and applying them to diverse problem scenarios. With dedication and consistent practice, you’ll develop the algorithmic thinking and problem-solving skills necessary to thrive in the competitive world of software development.
Keep exploring, keep coding, and never stop learning. The world of combinatorial algorithms is vast and exciting, offering endless opportunities for growth and innovation in your programming career.