Algorithmic Approaches to String Matching: Mastering Essential Techniques for Coding Interviews
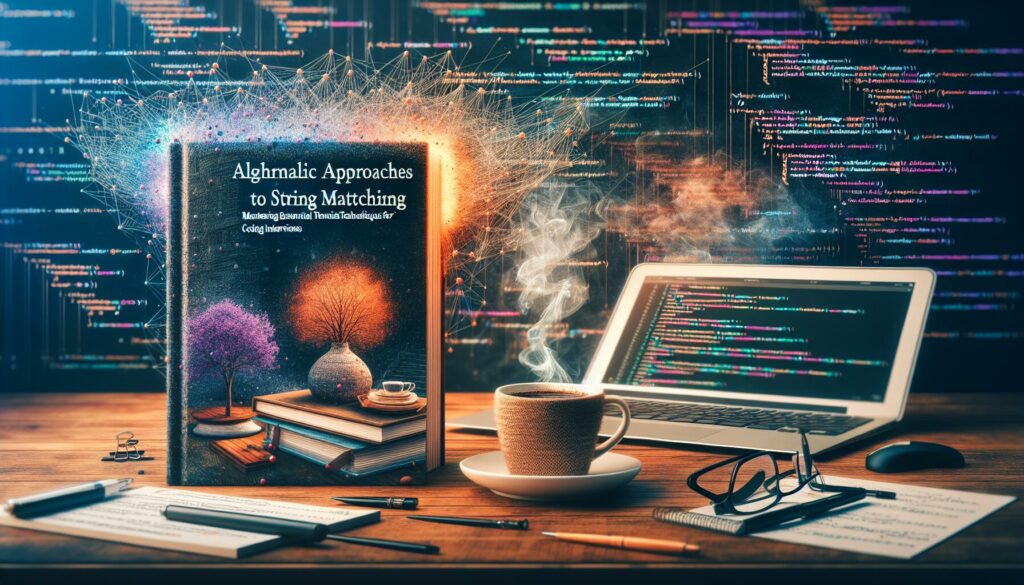
String matching is a fundamental problem in computer science that plays a crucial role in various applications, from text editors to bioinformatics. As aspiring programmers and those preparing for technical interviews, particularly with major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and implementing efficient string matching algorithms is essential. In this comprehensive guide, we’ll explore various algorithmic approaches to string matching, their implementations, and their applications in real-world scenarios.
1. Introduction to String Matching
String matching, also known as string searching, is the process of finding occurrences of a pattern string within a larger text string. This seemingly simple task becomes complex when dealing with large amounts of data or when requiring high-performance solutions.
The problem can be formally defined as follows:
- Given a text string T of length n and a pattern string P of length m
- Find all occurrences of P in T
- Return the starting indices of all matches
For example, if T = “ABABDABACDABABCABAB” and P = “ABABC”, the algorithm should return [10] as the pattern occurs at index 10 in the text.
2. Naive String Matching Algorithm
The naive approach to string matching involves checking every possible position in the text for a match with the pattern. While simple to implement, this method is not efficient for large texts or patterns.
Algorithm:
- Iterate through each position i in the text T from 0 to n – m
- For each position, compare the substring T[i:i+m] with the pattern P
- If a match is found, add i to the result list
Implementation in Python:
def naive_string_match(text, pattern):
n, m = len(text), len(pattern)
results = []
for i in range(n - m + 1):
if text[i:i+m] == pattern:
results.append(i)
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(naive_string_match(text, pattern)) # Output: [10]
Time Complexity: O(n * m), where n is the length of the text and m is the length of the pattern.
3. Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm improves upon the naive approach by utilizing information about the pattern itself to avoid unnecessary comparisons. It preprocesses the pattern to create a failure function (or prefix function) that helps skip characters that are known not to lead to a match.
Key Concepts:
- Prefix Function: An array that stores the length of the longest proper prefix that is also a suffix for each substring of the pattern
- Partial Match Table: Used to determine how many characters to skip when a mismatch occurs
Algorithm:
- Preprocess the pattern to create the failure function
- Iterate through the text, comparing characters with the pattern
- When a mismatch occurs, use the failure function to determine how many characters to skip
- Continue until the end of the text is reached
Implementation in Python:
def kmp_string_match(text, pattern):
n, m = len(text), len(pattern)
# Compute failure function
failure = [0] * m
j = 0
for i in range(1, m):
while j > 0 and pattern[i] != pattern[j]:
j = failure[j-1]
if pattern[i] == pattern[j]:
j += 1
failure[i] = j
# Perform string matching
results = []
j = 0
for i in range(n):
while j > 0 and text[i] != pattern[j]:
j = failure[j-1]
if text[i] == pattern[j]:
j += 1
if j == m:
results.append(i - m + 1)
j = failure[j-1]
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(kmp_string_match(text, pattern)) # Output: [10]
Time Complexity: O(n + m), where n is the length of the text and m is the length of the pattern.
4. Boyer-Moore Algorithm
The Boyer-Moore algorithm is considered one of the most efficient string matching algorithms, especially for large alphabets. It uses two heuristics to improve performance: the bad character heuristic and the good suffix heuristic.
Key Concepts:
- Bad Character Heuristic: Skips characters based on the rightmost occurrence of a mismatched character in the pattern
- Good Suffix Heuristic: Utilizes information about the occurrence of suffixes in the pattern to skip comparisons
Algorithm:
- Preprocess the pattern to create bad character and good suffix tables
- Align the pattern at the beginning of the text
- Compare characters from right to left
- If a mismatch occurs, use the maximum of bad character and good suffix heuristics to shift the pattern
- Repeat until the end of the text is reached
Implementation in Python:
def boyer_moore_string_match(text, pattern):
n, m = len(text), len(pattern)
# Preprocessing
bad_char = {c: max(1, m - i - 1) for i, c in enumerate(pattern)}
# String matching
results = []
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
results.append(i)
i += 1
else:
i += max(1, j - bad_char.get(text[i + j], m))
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(boyer_moore_string_match(text, pattern)) # Output: [10]
Time Complexity: O(n + m) in the best and average cases, O(n * m) in the worst case.
5. Rabin-Karp Algorithm
The Rabin-Karp algorithm uses hashing to find pattern matches in the text. It calculates hash values for the pattern and substrings of the text, comparing only when hash values match.
Key Concepts:
- Rolling Hash: Efficiently compute hash values for substrings of the text
- Modular Arithmetic: Used to prevent integer overflow in hash calculations
Algorithm:
- Compute the hash value of the pattern
- Compute the hash value of the first m characters of the text
- For each subsequent position in the text:
- If the hash values match, compare the actual characters
- If a match is found, add the index to the result list
- Update the rolling hash for the next substring
Implementation in Python:
def rabin_karp_string_match(text, pattern):
n, m = len(text), len(pattern)
p, t = 0, 0 # hash values for pattern and text
results = []
d = 256 # number of characters in the alphabet
q = 101 # a prime number
h = pow(d, m-1) % q
# Compute initial hash values
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
# Sliding window
for i in range(n - m + 1):
if p == t:
if text[i:i+m] == pattern:
results.append(i)
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i+m])) % q
if t < 0:
t += q
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(rabin_karp_string_match(text, pattern)) # Output: [10]
Time Complexity: O(n + m) average and best case, O(n * m) worst case.
6. Aho-Corasick Algorithm
The Aho-Corasick algorithm is particularly useful for multiple pattern matching. It constructs a finite state machine from the set of patterns and then uses it to process the text in a single pass.
Key Concepts:
- Trie: A tree-like data structure used to store the patterns
- Failure Links: Similar to KMP’s failure function, used for efficient transitions
- Output Function: Keeps track of matched patterns at each state
Algorithm:
- Construct a trie from the set of patterns
- Build failure links and output function for the trie
- Process the text using the constructed automaton
- Report matches as they are found
Implementation in Python:
from collections import deque
class AhoCorasick:
def __init__(self, patterns):
self.trie = {}
self.failure = {}
self.output = {}
self.build_trie(patterns)
self.build_failure_and_output()
def build_trie(self, patterns):
for i, pattern in enumerate(patterns):
node = self.trie
for char in pattern:
node = node.setdefault(char, {})
node["#"] = i
def build_failure_and_output(self):
queue = deque(self.trie.values())
while queue:
node = queue.popleft()
for char, child in node.items():
if char != "#":
queue.append(child)
failure = self.failure.get(node, self.trie)
while char not in failure and failure:
failure = self.failure.get(failure, self.trie)
self.failure[child] = failure.get(char, self.trie)
self.output[child] = self.output.get(self.failure[child], [])
if "#" in self.failure[child]:
self.output[child].append(self.failure[child]["#"])
def search(self, text):
node = self.trie
results = []
for i, char in enumerate(text):
while char not in node and node != self.trie:
node = self.failure[node]
node = node.get(char, self.trie)
for pattern_index in self.output.get(node, []):
results.append((i - len(patterns[pattern_index]) + 1, pattern_index))
if "#" in node:
results.append((i - len(patterns[node["#"]]) + 1, node["#"]))
return results
# Example usage
patterns = ["ABABC", "ABC", "BC"]
text = "ABABDABACDABABCABAB"
ac = AhoCorasick(patterns)
matches = ac.search(text)
print(matches) # Output: [(10, 0), (11, 1), (12, 2)]
Time Complexity: O(n + m + z), where n is the length of the text, m is the total length of all patterns, and z is the number of output matches.
7. Applications and Real-world Scenarios
String matching algorithms find applications in various domains:
- Text Editors: Implementing search and replace functionality
- Bioinformatics: DNA sequence analysis and protein pattern matching
- Network Security: Intrusion detection systems and packet inspection
- Information Retrieval: Search engines and document indexing
- Spam Filters: Identifying patterns in email content
- Plagiarism Detection: Comparing documents for similarities
Example: Implementing a Simple Plagiarism Detector
Let’s use the Rabin-Karp algorithm to create a basic plagiarism detector that finds common substrings between two documents:
def plagiarism_detector(doc1, doc2, k):
"""
Detect potential plagiarism by finding common substrings of length k
"""
def hash_func(s):
return sum(ord(c) for c in s)
n1, n2 = len(doc1), len(doc2)
common_substrings = set()
# Create hash tables for both documents
hash1 = {hash_func(doc1[i:i+k]): i for i in range(n1-k+1)}
# Check for matches in doc2
for i in range(n2-k+1):
h = hash_func(doc2[i:i+k])
if h in hash1:
if doc2[i:i+k] == doc1[hash1[h]:hash1[h]+k]:
common_substrings.add(doc2[i:i+k])
return common_substrings
# Example usage
doc1 = "The quick brown fox jumps over the lazy dog"
doc2 = "A quick brown dog jumps over the lazy fox"
k = 5 # Length of substrings to check
common = plagiarism_detector(doc1, doc2, k)
print(f"Common substrings of length {k}:")
for substring in common:
print(substring)
This simple plagiarism detector finds common substrings of a specified length between two documents, which could indicate potential copied content.
8. Performance Comparison and Choosing the Right Algorithm
When selecting a string matching algorithm for a specific problem, consider the following factors:
- Text and pattern sizes
- Alphabet size
- Expected frequency of matches
- Memory constraints
- Preprocessing time vs. matching time trade-offs
Here’s a comparison of the algorithms discussed:
Algorithm | Preprocessing Time | Matching Time | Space Complexity | Best For |
---|---|---|---|---|
Naive | O(1) | O(n*m) | O(1) | Short patterns, small texts |
KMP | O(m) | O(n) | O(m) | Long patterns, large texts |
Boyer-Moore | O(m + σ) | O(n/m) to O(n*m) | O(σ) | Large alphabets, long patterns |
Rabin-Karp | O(m) | O(n) average, O(n*m) worst | O(1) | Multiple pattern matching |
Aho-Corasick | O(m) | O(n + z) | O(m) | Multiple pattern matching |
Where n is the text length, m is the pattern length, σ is the alphabet size, and z is the number of matches.
9. Optimizations and Variations
Several optimizations and variations of these algorithms exist:
- Suffix Arrays: Efficient data structure for multiple string matching
- Bit-parallel Algorithms: Utilize bitwise operations for faster matching
- Approximate String Matching: Allow for a certain number of errors or differences
- Compressed String Matching: Perform matching directly on compressed text
Example: Bit-parallel Algorithm for Exact String Matching
Here’s an implementation of the Shift-Or algorithm, a bit-parallel approach to string matching:
def shift_or_string_match(text, pattern):
n, m = len(text), len(pattern)
if m > 63: # Limit due to 64-bit integers
raise ValueError("Pattern too long for this implementation")
# Preprocessing
char_mask = {}
all_ones = (1 << m) - 1
for i, char in enumerate(pattern):
char_mask[char] = all_ones ^ (1 << i)
# Matching
state = all_ones
results = []
for i, char in enumerate(text):
state = ((state << 1) | 1) & char_mask.get(char, all_ones)
if not state & (1 << (m - 1)):
results.append(i - m + 1)
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABC"
print(shift_or_string_match(text, pattern)) # Output: [10]
This algorithm uses bitwise operations to perform string matching, which can be very efficient on modern processors.
10. Conclusion
String matching is a fundamental problem in computer science with numerous applications. Understanding and implementing these algorithms is crucial for aspiring programmers and those preparing for technical interviews, especially with major tech companies.
Key takeaways:
- Different algorithms suit different scenarios based on text and pattern characteristics
- Preprocessing can significantly improve matching efficiency
- Advanced techniques like bit-parallelism can further optimize performance
- Real-world applications span various domains, from text processing to bioinformatics
As you prepare for coding interviews and develop your programming skills, practice implementing and analyzing these string matching algorithms. They not only demonstrate your understanding of fundamental computer science concepts but also showcase your ability to optimize solutions for specific problem constraints.
Remember that mastering these algorithms is just one aspect of becoming a proficient programmer. Continue to explore other areas of algorithm design, data structures, and problem-solving techniques to build a well-rounded skill set that will serve you well in your coding journey and future technical interviews.