Implementing Hash Functions and Handling Collisions: A Comprehensive Guide
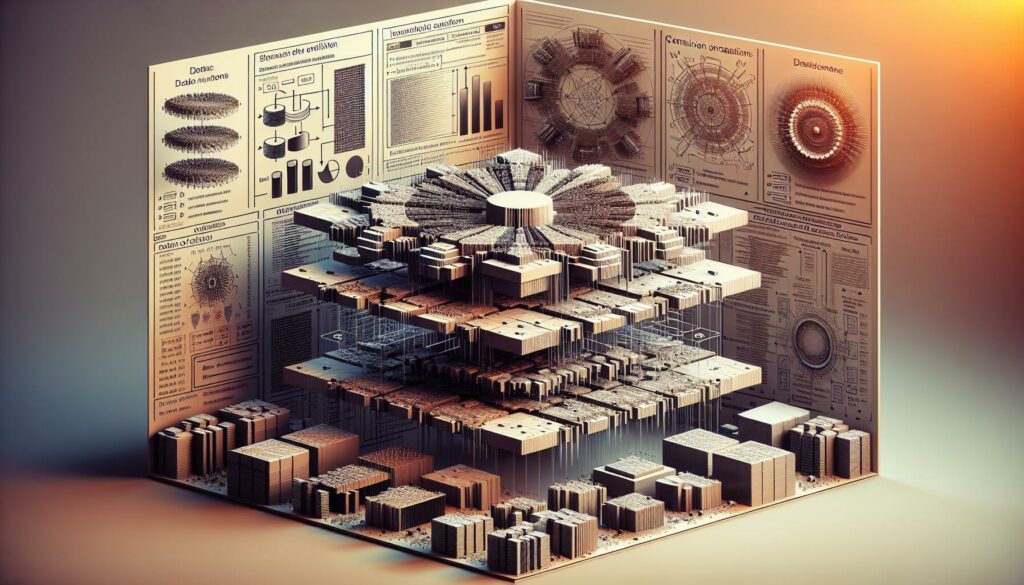
In the world of computer science and programming, hash functions and hash tables play a crucial role in efficiently storing and retrieving data. Whether you’re preparing for technical interviews at major tech companies or simply looking to enhance your coding skills, understanding how to implement hash functions and handle collisions is essential. In this comprehensive guide, we’ll dive deep into the concepts, implementation techniques, and best practices for working with hash functions and managing collisions.
Table of Contents
- What Are Hash Functions?
- Properties of Good Hash Functions
- Implementing Hash Functions
- Understanding Collisions
- Collision Resolution Techniques
- Implementing Collision Resolution
- Performance Analysis
- Real-World Applications
- Best Practices and Tips
- Conclusion
1. What Are Hash Functions?
Hash functions are mathematical functions that take an input (or ‘key’) and return a fixed-size string of bytes. The output is typically a fixed-size integer value called a hash code or hash value. Hash functions are designed to be fast to compute and to minimize collisions, where different inputs produce the same output.
In the context of hash tables or hash maps, hash functions are used to map keys to indices in an array, allowing for efficient storage and retrieval of data. The goal is to distribute the keys as evenly as possible across the available array indices.
2. Properties of Good Hash Functions
A good hash function should possess the following properties:
- Deterministic: The same input should always produce the same output.
- Uniform distribution: The hash function should map the expected inputs as evenly as possible over its output range.
- Efficiency: The hash function should be fast to compute.
- Avalanche effect: A small change in the input should result in a significant change in the output.
- Non-invertible: It should be computationally infeasible to reconstruct the input from the output.
3. Implementing Hash Functions
Let’s explore some common techniques for implementing hash functions:
3.1. Division Method
The division method is one of the simplest hash functions. It involves taking the modulus of the key with the size of the hash table:
h(k) = k % m
Where k
is the key and m
is the size of the hash table. Here’s a Python implementation:
def division_hash(key, table_size):
return key % table_size
3.2. Multiplication Method
The multiplication method involves multiplying the key by a constant A (0 < A < 1) and taking the fractional part. This is then multiplied by the table size and floored:
h(k) = floor(m * (k * A % 1))
Here’s a Python implementation:
import math
def multiplication_hash(key, table_size, A=0.6180339887):
return math.floor(table_size * ((key * A) % 1))
3.3. Universal Hashing
Universal hashing involves selecting a hash function at random from a family of hash functions. This helps to ensure good average-case performance even if the input is chosen by an adversary. Here’s a simple example in Python:
import random
def universal_hash(key, table_size, a, b, p):
return ((a * key + b) % p) % table_size
# Generate random parameters
p = 2**31 - 1 # A large prime number
a = random.randint(1, p - 1)
b = random.randint(0, p - 1)
4. Understanding Collisions
Collisions occur when two different keys hash to the same index in the hash table. This is inevitable due to the pigeonhole principle: if we have more keys than table slots, at least two keys must hash to the same slot.
Collisions can significantly impact the performance of hash tables, potentially degrading lookup times from O(1) to O(n) in the worst case. Therefore, effective collision resolution strategies are crucial for maintaining the efficiency of hash-based data structures.
5. Collision Resolution Techniques
There are two main approaches to handling collisions:
5.1. Chaining (Open Hashing)
In chaining, each slot of the hash table contains a linked list of elements that hash to that slot. When a collision occurs, the new element is simply added to the list at that slot.
Advantages of chaining:
- Simple to implement
- Hash table never fills up
- Less sensitive to the hash function or load factors
Disadvantages of chaining:
- Requires additional memory for linked list pointers
- Cache performance can be poor if chains become long
5.2. Open Addressing (Closed Hashing)
In open addressing, all elements are stored in the hash table itself. When a collision occurs, we probe for the next available slot in the table. There are several probing techniques:
- Linear Probing: Check the next slot sequentially until an empty slot is found.
- Quadratic Probing: Check slots at quadratic intervals.
- Double Hashing: Use a second hash function to determine the interval between probes.
Advantages of open addressing:
- Better cache performance
- No extra memory needed for pointers
Disadvantages of open addressing:
- More sensitive to the hash function and load factor
- Can suffer from primary clustering (in linear probing)
- Deletion is more complicated
6. Implementing Collision Resolution
Let’s implement both chaining and open addressing techniques in Python:
6.1. Chaining Implementation
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.next = None
class HashTableChaining:
def __init__(self, size):
self.size = size
self.table = [None] * size
def hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self.hash_function(key)
if self.table[index] is None:
self.table[index] = Node(key, value)
else:
current = self.table[index]
while current.next:
if current.key == key:
current.value = value
return
current = current.next
if current.key == key:
current.value = value
else:
current.next = Node(key, value)
def get(self, key):
index = self.hash_function(key)
current = self.table[index]
while current:
if current.key == key:
return current.value
current = current.next
raise KeyError(key)
def remove(self, key):
index = self.hash_function(key)
if self.table[index] is None:
raise KeyError(key)
if self.table[index].key == key:
self.table[index] = self.table[index].next
return
current = self.table[index]
while current.next:
if current.next.key == key:
current.next = current.next.next
return
current = current.next
raise KeyError(key)
6.2. Open Addressing Implementation (Linear Probing)
class HashTableOpenAddressing:
def __init__(self, size):
self.size = size
self.keys = [None] * size
self.values = [None] * size
def hash_function(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self.hash_function(key)
while self.keys[index] is not None:
if self.keys[index] == key:
self.values[index] = value
return
index = (index + 1) % self.size
self.keys[index] = key
self.values[index] = value
def get(self, key):
index = self.hash_function(key)
while self.keys[index] is not None:
if self.keys[index] == key:
return self.values[index]
index = (index + 1) % self.size
raise KeyError(key)
def remove(self, key):
index = self.hash_function(key)
while self.keys[index] is not None:
if self.keys[index] == key:
self.keys[index] = None
self.values[index] = None
return
index = (index + 1) % self.size
raise KeyError(key)
7. Performance Analysis
The performance of hash tables depends on several factors:
- Load factor: The ratio of the number of elements to the table size. As the load factor increases, the probability of collisions increases.
- Quality of the hash function: A good hash function distributes keys uniformly, reducing collisions.
- Collision resolution method: Different methods have different trade-offs in terms of memory usage and performance.
Time complexities for hash table operations:
- Average case (good hash function, low load factor):
- Insert: O(1)
- Search: O(1)
- Delete: O(1)
- Worst case (many collisions):
- Insert: O(n)
- Search: O(n)
- Delete: O(n)
To maintain good performance, it’s important to resize the hash table when the load factor exceeds a certain threshold (typically 0.7 or 0.75).
8. Real-World Applications
Hash functions and hash tables have numerous applications in computer science and software development:
- Database indexing: Hash indexes can provide fast access to data in databases.
- Caching: Hash tables are used to implement caches in various systems, from CPU caches to web caches.
- Cryptography: Cryptographic hash functions are used for digital signatures, password storage, and data integrity verification.
- Load balancing: Hash functions can be used to distribute requests or data across multiple servers.
- Duplicate detection: Hash functions can quickly identify duplicate items in large datasets.
- Spell checkers: Hash tables can store dictionaries for fast word lookup.
- Compiler symbol tables: Hash tables are used to store and quickly access variable and function names during compilation.
9. Best Practices and Tips
When implementing hash functions and working with hash tables, keep these best practices in mind:
- Choose an appropriate hash function: Ensure your hash function distributes keys uniformly across the table.
- Monitor the load factor: Resize the hash table when the load factor exceeds a predefined threshold to maintain performance.
- Use prime numbers: When possible, use prime numbers for the table size to reduce collisions.
- Test with various inputs: Verify your hash function’s performance with different types and distributions of input data.
- Consider security: If your hash function will be used in a security-sensitive context, use cryptographic hash functions designed for that purpose.
- Benchmark and profile: Measure the performance of your hash table implementation and identify bottlenecks.
- Use existing implementations: For production code, consider using well-tested hash table implementations provided by standard libraries or frameworks.
10. Conclusion
Understanding and implementing hash functions and collision resolution techniques is crucial for any programmer aiming to work with efficient data structures and algorithms. Hash tables provide near-constant time complexity for insertions, deletions, and lookups, making them indispensable in many applications.
As you prepare for technical interviews or work on real-world projects, remember that mastering these concepts will not only help you solve specific problems but also give you a deeper understanding of how to design efficient algorithms and data structures.
Continue practicing with different hash function implementations and collision resolution techniques. Experiment with various load factors and table sizes to understand their impact on performance. By doing so, you’ll be well-prepared to tackle complex problems and optimize solutions in your future programming endeavors.