Algorithms in Machine Learning: An Introduction
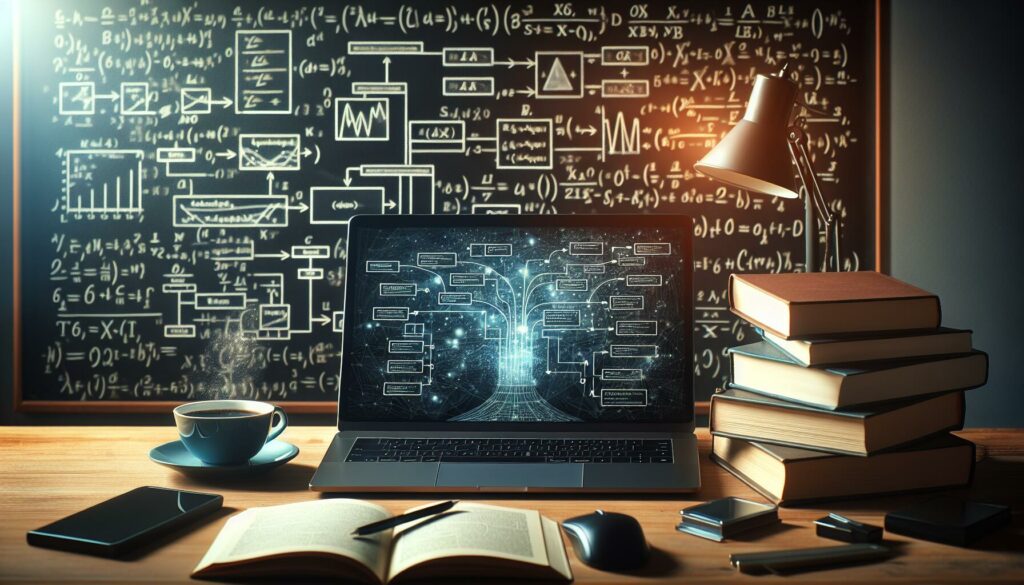
In the ever-evolving world of technology, machine learning has emerged as a cornerstone of innovation, powering everything from recommendation systems to autonomous vehicles. At the heart of machine learning lie algorithms – the step-by-step procedures that enable computers to learn from data and make intelligent decisions. This comprehensive guide will introduce you to the fascinating world of algorithms in machine learning, exploring their types, applications, and importance in shaping the future of artificial intelligence.
What Are Machine Learning Algorithms?
Machine learning algorithms are computational methods that allow computer systems to improve their performance on a specific task through experience. Unlike traditional programming, where explicit instructions are given to solve a problem, machine learning algorithms use data to learn patterns and make predictions or decisions without being explicitly programmed for the task.
These algorithms can be broadly categorized into three main types:
- Supervised Learning
- Unsupervised Learning
- Reinforcement Learning
Each type serves different purposes and is suited for specific kinds of problems. Let’s delve deeper into each category and explore some popular algorithms within them.
Supervised Learning Algorithms
Supervised learning algorithms work with labeled data, where both the input and the desired output are provided. The algorithm learns to map the input to the output, allowing it to make predictions on new, unseen data. Some common supervised learning algorithms include:
1. Linear Regression
Linear regression is one of the simplest and most widely used algorithms for predictive modeling. It’s used to predict a continuous outcome variable based on one or more input variables. The algorithm finds the best-fitting straight line through the points in the data.
Here’s a simple example of implementing linear regression in Python using the scikit-learn library:
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
# Create and train the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
new_X = np.array([[6]])
prediction = model.predict(new_X)
print(f"Prediction for X=6: {prediction[0]}")
2. Logistic Regression
Despite its name, logistic regression is used for classification problems. It predicts the probability of an instance belonging to a particular class. It’s widely used in binary classification problems, such as spam detection or disease diagnosis.
3. Decision Trees
Decision trees are versatile algorithms that can be used for both classification and regression tasks. They work by creating a tree-like model of decisions based on features in the data. Each internal node represents a “test” on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or a numerical value.
4. Random Forests
Random forests are an ensemble learning method that operates by constructing multiple decision trees during training and outputting the class that is the mode of the classes (classification) or mean prediction (regression) of the individual trees. This approach helps to reduce overfitting, a common problem with individual decision trees.
5. Support Vector Machines (SVM)
SVMs are powerful algorithms used for classification and regression tasks. They work by finding the hyperplane that best divides a dataset into classes. SVMs are particularly effective in high-dimensional spaces and are widely used in image classification and bioinformatics.
Unsupervised Learning Algorithms
Unsupervised learning algorithms work with unlabeled data, trying to find patterns or structures within the data without any predefined output. These algorithms are useful for exploratory data analysis, dimensionality reduction, and finding hidden patterns. Some popular unsupervised learning algorithms include:
1. K-Means Clustering
K-means is a widely used clustering algorithm that aims to partition n observations into k clusters, where each observation belongs to the cluster with the nearest mean (cluster centroid). It’s commonly used for customer segmentation, image compression, and anomaly detection.
Here’s a basic implementation of K-means clustering using scikit-learn:
from sklearn.cluster import KMeans
import numpy as np
# Sample data
X = np.array([[1, 2], [1.5, 1.8], [5, 8], [8, 8], [1, 0.6], [9, 11]])
# Create and fit the model
kmeans = KMeans(n_clusters=2)
kmeans.fit(X)
# Get cluster assignments
labels = kmeans.labels_
print(f"Cluster assignments: {labels}")
2. Hierarchical Clustering
Hierarchical clustering creates a tree of clusters, known as a dendrogram. It can be either agglomerative (bottom-up approach) or divisive (top-down approach). This method is particularly useful when you want to visualize the hierarchy of clusters in your data.
3. Principal Component Analysis (PCA)
PCA is a dimensionality reduction technique that transforms the data into a new coordinate system where the variables are uncorrelated. It’s widely used for feature extraction, noise reduction, and visualization of high-dimensional data.
4. t-SNE (t-Distributed Stochastic Neighbor Embedding)
t-SNE is another dimensionality reduction technique, particularly useful for visualizing high-dimensional data. Unlike PCA, t-SNE is capable of preserving local structures in the data, making it excellent for visualizing clusters or groups in complex datasets.
Reinforcement Learning Algorithms
Reinforcement learning is a type of machine learning where an agent learns to make decisions by interacting with an environment. The agent receives rewards or penalties for its actions and aims to maximize the cumulative reward over time. Some popular reinforcement learning algorithms include:
1. Q-Learning
Q-Learning is a model-free reinforcement learning algorithm that learns the value of an action in a particular state. It’s widely used in game playing and robotics. The “Q” in Q-Learning stands for the quality of an action taken in a given state.
2. SARSA (State-Action-Reward-State-Action)
SARSA is an on-policy reinforcement learning algorithm for learning a Markov decision process policy. Unlike Q-Learning, which is an off-policy method, SARSA takes into account the action performed in the next state when updating the Q-value of the current state-action pair.
3. Deep Q-Network (DQN)
DQN combines Q-Learning with deep neural networks to handle high-dimensional state spaces. It was famously used by DeepMind to achieve human-level performance on Atari games.
4. Policy Gradient Methods
Policy gradient methods work by optimizing the policy directly, rather than maintaining a value function. These methods are particularly useful in continuous action spaces and have been successful in robotic control tasks.
The Importance of Algorithms in Machine Learning
Understanding and implementing machine learning algorithms is crucial for several reasons:
- Problem Solving: Different problems require different algorithmic approaches. Knowing a variety of algorithms allows you to choose the most appropriate one for your specific task.
- Performance Optimization: Understanding how algorithms work enables you to fine-tune their parameters and improve their performance on your data.
- Interpretability: Some algorithms, like decision trees, provide interpretable models that can help explain the reasoning behind predictions.
- Efficiency: Choosing the right algorithm can significantly impact the computational resources required and the speed of model training and inference.
- Innovation: A deep understanding of existing algorithms can inspire the development of new, more efficient algorithms or hybrid approaches.
Challenges in Implementing Machine Learning Algorithms
While machine learning algorithms offer powerful solutions to complex problems, their implementation comes with several challenges:
1. Data Quality and Quantity
The performance of machine learning algorithms heavily depends on the quality and quantity of the data they’re trained on. Insufficient, biased, or noisy data can lead to poor model performance.
2. Overfitting and Underfitting
Overfitting occurs when a model learns the training data too well, including its noise and outliers, leading to poor generalization on new data. Underfitting, on the other hand, happens when the model is too simple to capture the underlying patterns in the data.
3. Feature Selection and Engineering
Choosing the right features and creating new, informative features from existing data (feature engineering) can significantly impact model performance. This process often requires domain expertise and can be time-consuming.
4. Hyperparameter Tuning
Many machine learning algorithms have hyperparameters that need to be set before training. Finding the optimal combination of these hyperparameters can be challenging and often requires techniques like grid search, random search, or Bayesian optimization.
5. Interpretability vs. Performance Trade-off
Some of the most powerful algorithms, like deep neural networks, are often criticized for being “black boxes” that are difficult to interpret. Balancing model performance with interpretability is an ongoing challenge in the field.
Future Trends in Machine Learning Algorithms
As the field of machine learning continues to evolve, several exciting trends are emerging:
1. Automated Machine Learning (AutoML)
AutoML aims to automate the process of selecting, configuring, and optimizing machine learning models. This technology has the potential to make machine learning more accessible to non-experts and increase the efficiency of data scientists.
2. Federated Learning
Federated learning allows for training models on distributed datasets without centralizing the data. This approach addresses privacy concerns and enables learning from data that can’t be centralized due to regulatory or practical constraints.
3. Quantum Machine Learning
As quantum computing technology advances, researchers are exploring ways to leverage quantum algorithms for machine learning tasks. Quantum machine learning has the potential to solve certain problems exponentially faster than classical algorithms.
4. Explainable AI (XAI)
There’s a growing emphasis on developing machine learning models that can explain their decisions in human-understandable terms. This is particularly important in high-stakes applications like healthcare and finance.
5. Edge AI
Edge AI involves running machine learning algorithms on edge devices (like smartphones or IoT devices) rather than in the cloud. This approach reduces latency and addresses privacy concerns by keeping data on the device.
Conclusion
Algorithms are the backbone of machine learning, enabling computers to learn from data and make intelligent decisions. From supervised learning algorithms like linear regression and random forests to unsupervised methods like K-means clustering and reinforcement learning approaches like Q-learning, each algorithm has its strengths and suitable applications.
As you embark on your journey in machine learning, remember that understanding these algorithms is just the beginning. The real challenge – and excitement – lies in applying them to real-world problems, overcoming the inherent challenges, and pushing the boundaries of what’s possible with artificial intelligence.
Whether you’re a beginner just starting out or an experienced practitioner looking to deepen your knowledge, the world of machine learning algorithms offers endless opportunities for learning and innovation. As the field continues to evolve, staying updated with the latest algorithmic developments and trends will be crucial for anyone looking to make an impact in this exciting domain.
Remember, the best way to truly understand these algorithms is through hands-on practice. Start experimenting with different algorithms on various datasets, participate in machine learning competitions, and don’t be afraid to dive deep into the mathematical foundations of these methods. With persistence and curiosity, you’ll be well on your way to mastering the art and science of machine learning algorithms.