Advanced Dynamic Programming Problems and Solutions
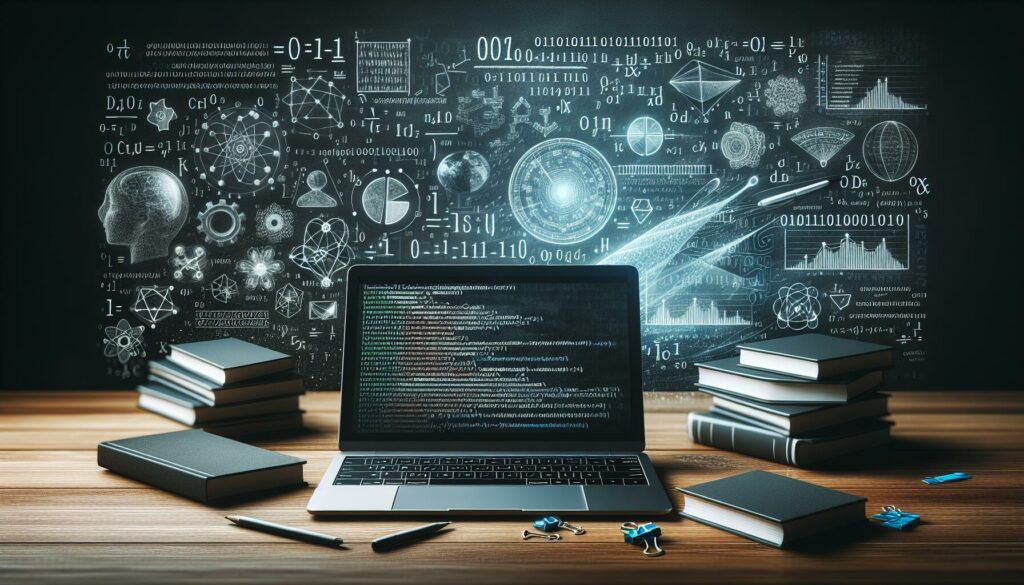
Dynamic Programming (DP) is a powerful algorithmic technique used to solve complex problems by breaking them down into simpler subproblems. It’s a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore advanced dynamic programming problems and their solutions, providing you with the tools to tackle even the most challenging coding questions.
Table of Contents
- Introduction to Advanced Dynamic Programming
- Problem 1: Longest Increasing Subsequence
- Problem 2: Edit Distance
- Problem 3: Knapsack Problem
- Problem 4: Matrix Chain Multiplication
- Problem 5: Palindrome Partitioning
- Tips for Solving Advanced DP Problems
- Conclusion
Introduction to Advanced Dynamic Programming
Advanced Dynamic Programming problems often require a deeper understanding of DP principles and more complex state representations. These problems typically involve:
- Multiple dimensions in the DP table
- Non-trivial state transitions
- Optimization of multiple parameters simultaneously
- Combination with other algorithmic techniques
As we dive into each problem, we’ll break down the solution process, discuss the intuition behind the approach, and provide optimized code implementations.
Problem 1: Longest Increasing Subsequence
The Longest Increasing Subsequence (LIS) problem is a classic DP problem that asks us to find the length of the longest subsequence of a given sequence such that all elements of the subsequence are sorted in increasing order.
Problem Statement
Given an unsorted array of integers, find the length of the longest increasing subsequence.
Example
Input: [10, 9, 2, 5, 3, 7, 101, 18]
Output: 4
Explanation: The longest increasing subsequence is [2, 5, 7, 101], therefore the length is 4.
Solution Approach
We can solve this problem using DP with the following approach:
- Create a DP array of the same length as the input array, initialized with 1s (as each element is an increasing subsequence of length 1 by itself).
- For each element, look at all previous elements:
- If the current element is greater than the previous element, we can potentially extend the increasing subsequence.
- Update the DP value for the current element to be the maximum of its current value and 1 plus the DP value of the smaller element.
Implementation
class Solution {
public:
int lengthOfLIS(vector<int>& nums) {
int n = nums.size();
if (n == 0) return 0;
vector<int> dp(n, 1);
int maxLen = 1;
for (int i = 1; i < n; i++) {
for (int j = 0; j < i; j++) {
if (nums[i] > nums[j]) {
dp[i] = max(dp[i], dp[j] + 1);
}
}
maxLen = max(maxLen, dp[i]);
}
return maxLen;
}
};
Time Complexity: O(n^2), where n is the length of the input array.
Space Complexity: O(n) for the DP array.
Optimization
We can optimize this solution to O(n log n) time complexity using a binary search approach, but that’s beyond the scope of this DP discussion.
Problem 2: Edit Distance
The Edit Distance problem, also known as the Levenshtein Distance problem, is another classic DP problem. It asks us to find the minimum number of operations required to transform one string into another.
Problem Statement
Given two strings word1 and word2, return the minimum number of operations required to convert word1 to word2. You have the following three operations permitted on a word:
- Insert a character
- Delete a character
- Replace a character
Example
Input: word1 = "horse", word2 = "ros"
Output: 3
Explanation:
horse -> rorse (replace 'h' with 'r')
rorse -> rose (remove 'r')
rose -> ros (remove 'e')
Solution Approach
We can solve this problem using a 2D DP table:
- Create a 2D DP table where dp[i][j] represents the minimum number of operations to convert the first i characters of word1 to the first j characters of word2.
- Initialize the first row and column of the DP table.
- For each cell in the DP table:
- If the characters match, copy the value from the diagonal (top-left).
- If they don’t match, take the minimum of insert, delete, and replace operations and add 1.
Implementation
class Solution {
public:
int minDistance(string word1, string word2) {
int m = word1.length();
int n = word2.length();
vector<vector<int>> dp(m + 1, vector<int>(n + 1, 0));
// Initialize first row and column
for (int i = 0; i <= m; i++) dp[i][0] = i;
for (int j = 0; j <= n; j++) dp[0][j] = j;
// Fill the DP table
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
if (word1[i-1] == word2[j-1]) {
dp[i][j] = dp[i-1][j-1];
} else {
dp[i][j] = min({dp[i-1][j], dp[i][j-1], dp[i-1][j-1]}) + 1;
}
}
}
return dp[m][n];
}
};
Time Complexity: O(mn), where m and n are the lengths of word1 and word2 respectively.
Space Complexity: O(mn) for the DP table.
Problem 3: Knapsack Problem
The Knapsack Problem is a fundamental problem in combinatorial optimization. It has many practical applications and is often used as a subproblem in more complex algorithms.
Problem Statement
Given weights and values of n items, put these items in a knapsack of capacity W to get the maximum total value in the knapsack. In other words, given two integer arrays val[0..n-1] and wt[0..n-1] which represent values and weights associated with n items respectively. Also given an integer W which represents knapsack capacity, find out the maximum value subset of val[] such that sum of the weights of this subset is smaller than or equal to W.
Example
Input:
values[] = {60, 100, 120}
weights[] = {10, 20, 30}
W = 50
Output: 220
Explanation: We can take the 2nd and 3rd items with weights 20 and 30, and value 100 + 120 = 220
Solution Approach
We can solve this problem using a 2D DP table:
- Create a 2D DP table where dp[i][w] represents the maximum value that can be achieved with first i items and weight limit w.
- Initialize the first row and column of the DP table with 0.
- For each item and each possible weight:
- If the item’s weight is more than the current weight limit, we can’t include it, so we take the value from the previous row.
- Otherwise, we take the maximum of including the item (its value plus the value we can get with the remaining weight) and not including the item (value from the previous row).
Implementation
class Solution {
public:
int knapsack(vector<int>& values, vector<int>& weights, int W) {
int n = values.size();
vector<vector<int>> dp(n + 1, vector<int>(W + 1, 0));
for (int i = 1; i <= n; i++) {
for (int w = 1; w <= W; w++) {
if (weights[i-1] <= w) {
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w]);
} else {
dp[i][w] = dp[i-1][w];
}
}
}
return dp[n][W];
}
};
Time Complexity: O(nW), where n is the number of items and W is the knapsack capacity.
Space Complexity: O(nW) for the DP table.
Problem 4: Matrix Chain Multiplication
Matrix Chain Multiplication is an optimization problem that seeks to find the most efficient way to multiply a chain of matrices. This problem demonstrates the power of DP in solving complex optimization problems.
Problem Statement
Given a sequence of matrices, find the most efficient way to multiply these matrices together. The problem is not actually to perform the multiplications, but merely to decide in which order to perform the multiplications.
Example
Input: p[] = {40, 20, 30, 10, 30}
Output: 26000
Explanation: There are 4 matrices of dimensions 40x20, 20x30, 30x10 and 10x30.
Let the input 4 matrices be A, B, C and D. The minimum number of
multiplications are obtained by putting parenthesis in following way
(A(BC))D --> 20*30*10 + 40*20*10 + 40*10*30 = 26000
Solution Approach
We can solve this problem using a 2D DP table:
- Create a 2D DP table where dp[i][j] represents the minimum number of scalar multiplications needed to compute the matrix product from the ith matrix to the jth matrix.
- The base case is when i == j, which means a single matrix, so the cost is 0.
- Fill the table diagonally (as we need results of smaller chains to compute larger chains).
- For each cell, try all possible ways to split the chain and choose the one with minimum cost.
- The top-right cell of the DP table will contain the minimum cost for the entire chain.
Implementation
class Solution {
public:
int matrixChainMultiplication(vector<int>& p) {
int n = p.size() - 1;
vector<vector<int>> dp(n, vector<int>(n, 0));
for (int len = 2; len <= n; len++) {
for (int i = 0; i < n - len + 1; i++) {
int j = i + len - 1;
dp[i][j] = INT_MAX;
for (int k = i; k < j; k++) {
int cost = dp[i][k] + dp[k+1][j] + p[i]*p[k+1]*p[j+1];
dp[i][j] = min(dp[i][j], cost);
}
}
}
return dp[0][n-1];
}
};
Time Complexity: O(n^3), where n is the number of matrices.
Space Complexity: O(n^2) for the DP table.
Problem 5: Palindrome Partitioning
Palindrome Partitioning is an interesting problem that combines string manipulation with dynamic programming. It’s a great example of how DP can be applied to seemingly complex problems.
Problem Statement
Given a string s, partition s such that every substring of the partition is a palindrome. Return the minimum cuts needed for a palindrome partitioning of s.
Example
Input: s = "aab"
Output: 1
Explanation: The palindrome partitioning ["aa","b"] could be produced using 1 cut.
Solution Approach
We can solve this problem using two DP tables:
- First, create a 2D DP table to store whether substrings are palindromes.
- Then, create a 1D DP table where dp[i] represents the minimum number of cuts needed for the first i characters.
- For each ending position j:
- Check all starting positions i from 0 to j.
- If the substring from i to j is a palindrome, update dp[j] to be the minimum of its current value and dp[i-1] + 1.
Implementation
class Solution {
public:
int minCut(string s) {
int n = s.length();
vector<vector<bool>> isPalindrome(n, vector<bool>(n, false));
vector<int> dp(n);
for (int j = 0; j < n; j++) {
int minCut = j;
for (int i = 0; i <= j; i++) {
if (s[i] == s[j] && (j - i <= 2 || isPalindrome[i+1][j-1])) {
isPalindrome[i][j] = true;
minCut = i == 0 ? 0 : min(minCut, dp[i-1] + 1);
}
}
dp[j] = minCut;
}
return dp[n-1];
}
};
Time Complexity: O(n^2), where n is the length of the string.
Space Complexity: O(n^2) for the palindrome DP table and O(n) for the cuts DP array.
Tips for Solving Advanced DP Problems
- Identify the subproblems: The key to solving DP problems is recognizing the overlapping subproblems. Try to break down the problem into smaller, similar subproblems.
- Define the state: Clearly define what each element in your DP table represents. This is crucial for correctly formulating the recurrence relation.
- Formulate the recurrence relation: This is the heart of the DP solution. It describes how the solution to a larger problem can be built from solutions to smaller problems.
- Determine the base cases: Identify the simplest instances of the problem and their solutions. These will serve as the starting point for your DP table.
- Decide the order of computation: Ensure that when you’re calculating a value, all the values it depends on have already been calculated.
- Optimize space: Often, you can reduce the space complexity by using only the necessary part of the DP table or by using rolling arrays.
- Practice, practice, practice: The more problems you solve, the better you’ll become at recognizing DP patterns and formulating solutions.
Conclusion
Advanced Dynamic Programming problems can be challenging, but they are also incredibly rewarding to solve. They require a deep understanding of DP principles and often involve creative thinking to formulate the correct state and recurrence relation. By mastering these advanced DP techniques, you’ll be well-equipped to tackle a wide range of complex algorithmic problems, giving you a significant advantage in technical interviews and competitive programming.
Remember, the key to mastering DP is practice. Start with simpler problems and gradually work your way up to more complex ones. Don’t be discouraged if you don’t immediately see the solution – DP problems often require time and patience to fully understand and solve efficiently.
As you continue your journey in algorithmic problem-solving, keep exploring new problems and techniques. Dynamic Programming is just one tool in your algorithmic toolkit, albeit a powerful one. Combine it with other techniques like greedy algorithms, divide and conquer, and graph algorithms to become a well-rounded problem solver.
Happy coding, and may your DP solutions always be optimal!