Algorithmic Strategies for Competitive Programming: Mastering the Art of Problem-Solving
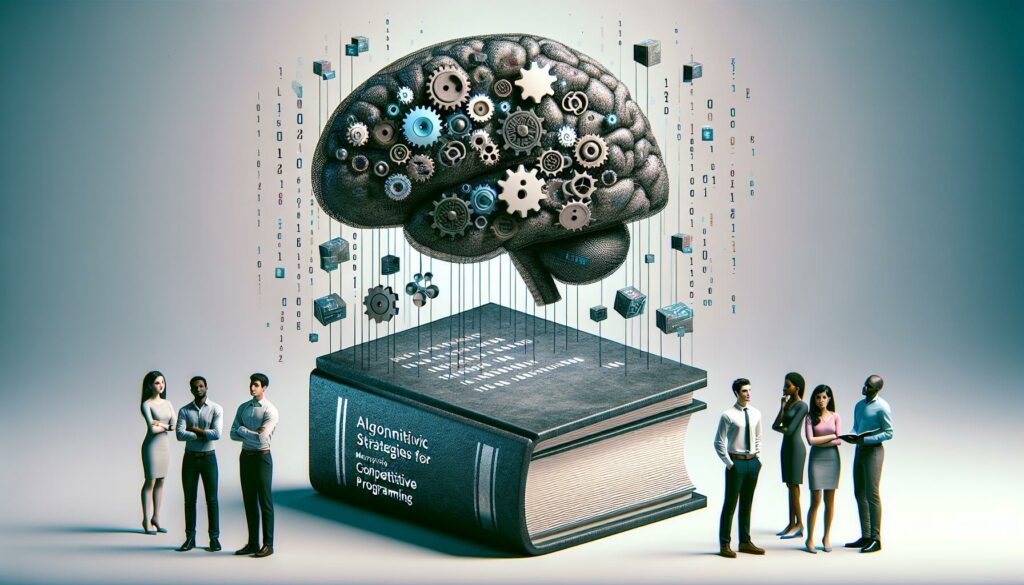
In the world of competitive programming and technical interviews, having a robust set of algorithmic strategies is crucial. These strategies not only help you solve complex problems efficiently but also demonstrate your problem-solving skills to potential employers. This comprehensive guide will delve into various algorithmic strategies that are essential for success in competitive programming and technical interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
1. Understanding the Importance of Algorithmic Strategies
Before we dive into specific strategies, it’s essential to understand why they are so important:
- Efficiency: Well-designed algorithms can solve problems faster and with fewer resources.
- Problem-solving skills: Mastering these strategies enhances your ability to approach and solve complex problems.
- Interview preparation: Many technical interviews, especially at FAANG companies, focus on algorithmic problem-solving.
- Code optimization: These strategies often lead to more optimized and maintainable code.
2. Divide and Conquer
The divide and conquer strategy involves breaking a problem into smaller, more manageable subproblems, solving them independently, and then combining the solutions to solve the original problem.
Key Concepts:
- Divide the problem into smaller subproblems
- Solve the subproblems recursively
- Combine the solutions of subproblems to solve the original problem
Example: Merge Sort
Merge Sort is a classic example of the divide and conquer strategy. Here’s a simple implementation in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
3. Dynamic Programming
Dynamic Programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems.
Key Concepts:
- Optimal substructure: The optimal solution to the problem contains optimal solutions to subproblems
- Overlapping subproblems: The same subproblems are solved multiple times
- Memoization: Storing the results of expensive function calls to avoid redundant computations
Example: Fibonacci Sequence
Here’s an implementation of the Fibonacci sequence using dynamic programming:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
n = 10
result = fibonacci(n)
print(f"The {n}th Fibonacci number is: {result}") # Output: The 10th Fibonacci number is: 55
4. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. While they don’t always yield the best solution, they are often simple and efficient.
Key Concepts:
- Make the best possible decision at each step
- Never reconsider previous choices
- Hope that a series of locally optimal choices leads to a globally optimal solution
Example: Coin Change Problem
Here’s a greedy approach to the coin change problem:
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
# Example usage
coins = [25, 10, 5, 1]
amount = 67
result = coin_change(coins, amount)
print(f"Minimum number of coins needed: {result}") # Output: Minimum number of coins needed: 6
5. Graph Algorithms
Graph algorithms are essential for solving problems involving networks, relationships, and interconnected systems. Some key graph algorithms include:
5.1 Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. Here’s a simple implementation:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal:")
dfs(graph, 'A') # Output: DFS traversal: A B D E F C
5.2 Breadth-First Search (BFS)
BFS explores all the neighbor nodes at the present depth before moving to nodes at the next depth level. Here’s an implementation:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal:")
bfs(graph, 'A') # Output: BFS traversal: A B C D E F
5.3 Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path between nodes in a graph. Here’s a basic implementation:
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
start_node = 'A'
shortest_distances = dijkstra(graph, start_node)
print(f"Shortest distances from {start_node}:")
for node, distance in shortest_distances.items():
print(f"{node}: {distance}")
6. Binary Search
Binary search is an efficient algorithm for searching a sorted array by repeatedly dividing the search interval in half.
Key Concepts:
- The array must be sorted
- Divide the search space in half at each step
- Time complexity: O(log n)
Example: Binary Search Implementation
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(arr, target)
print(f"Target {target} found at index: {result}") # Output: Target 7 found at index: 3
7. Two Pointers Technique
The two pointers technique is an algorithmic pattern that uses two pointers to solve problems efficiently, often reducing time complexity from O(n^2) to O(n).
Key Concepts:
- Use two pointers to traverse an array or string
- Pointers can move in the same direction or opposite directions
- Useful for problems involving searching, reversing, or finding pairs
Example: Two Sum Problem
def two_sum(numbers, target):
left, right = 0, len(numbers) - 1
while left < right:
current_sum = numbers[left] + numbers[right]
if current_sum == target:
return [left + 1, right + 1] # Adding 1 for 1-indexed array
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
numbers = [2, 7, 11, 15]
target = 9
result = two_sum(numbers, target)
print(f"Indices of the two numbers: {result}") # Output: Indices of the two numbers: [1, 2]
8. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or string. It’s particularly useful for solving problems related to subarrays or substrings.
Key Concepts:
- Maintain a window of elements
- Slide the window over the data structure
- Update the window and compute results as you slide
Example: Maximum Sum Subarray of Size K
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
result = max_sum_subarray(arr, k)
print(f"Maximum sum of subarray of size {k}: {result}") # Output: Maximum sum of subarray of size 4: 39
9. Backtracking
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It builds candidates to the solution and abandons those that cannot possibly be developed into a valid solution.
Key Concepts:
- Explore all potential solutions by trying to build a solution incrementally
- Abandon a solution (“backtrack”) as soon as it determines that the solution cannot be completed
- Often implemented using recursion
Example: N-Queens Problem
def solve_n_queens(n):
def is_safe(board, row, col):
# Check column
for i in range(row):
if board[i][col] == 'Q':
return False
# Check upper left diagonal
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 'Q':
return False
# Check upper right diagonal
for i, j in zip(range(row, -1, -1), range(col, n)):
if board[i][j] == 'Q':
return False
return True
def backtrack(board, row):
if row == n:
solutions.append([".".join(row) for row in board])
return
for col in range(n):
if is_safe(board, row, col):
board[row][col] = 'Q'
backtrack(board, row + 1)
board[row][col] = '.'
board = [['.'] * n for _ in range(n)]
solutions = []
backtrack(board, 0)
return solutions
# Example usage
n = 4
solutions = solve_n_queens(n)
print(f"Number of solutions for {n}-Queens: {len(solutions)}")
for solution in solutions:
print("\nSolution:")
for row in solution:
print(row)
10. Bit Manipulation
Bit manipulation involves the use of bitwise operators to perform operations at the bit level. This can lead to highly efficient algorithms for certain problems.
Key Concepts:
- Bitwise AND (&), OR (|), XOR (^), NOT (~)
- Left shift (<<) and right shift (>>) operators
- Useful for optimizing space and time complexity
Example: Counting Set Bits
def count_set_bits(n):
count = 0
while n:
count += n & 1
n >>= 1
return count
# Example usage
num = 13 # Binary: 1101
result = count_set_bits(num)
print(f"Number of set bits in {num}: {result}") # Output: Number of set bits in 13: 3
Conclusion
Mastering these algorithmic strategies is crucial for success in competitive programming and technical interviews, especially those conducted by major tech companies like FAANG. Each strategy has its own strengths and is suited for different types of problems. The key to becoming proficient is practice and understanding when to apply each strategy.
Remember that while knowing these strategies is important, the ability to analyze problems, recognize patterns, and apply the appropriate strategy is equally crucial. As you practice, focus on understanding the underlying principles and how they can be applied to various problem scenarios.
Continuous learning and practice are essential in the ever-evolving field of computer science and programming. Platforms like AlgoCademy provide valuable resources and interactive coding tutorials to help you hone your skills and prepare for technical interviews. By mastering these algorithmic strategies and continuously challenging yourself with new problems, you’ll be well-equipped to tackle complex coding challenges and excel in your programming career.