An Overview of Cryptographic Algorithms
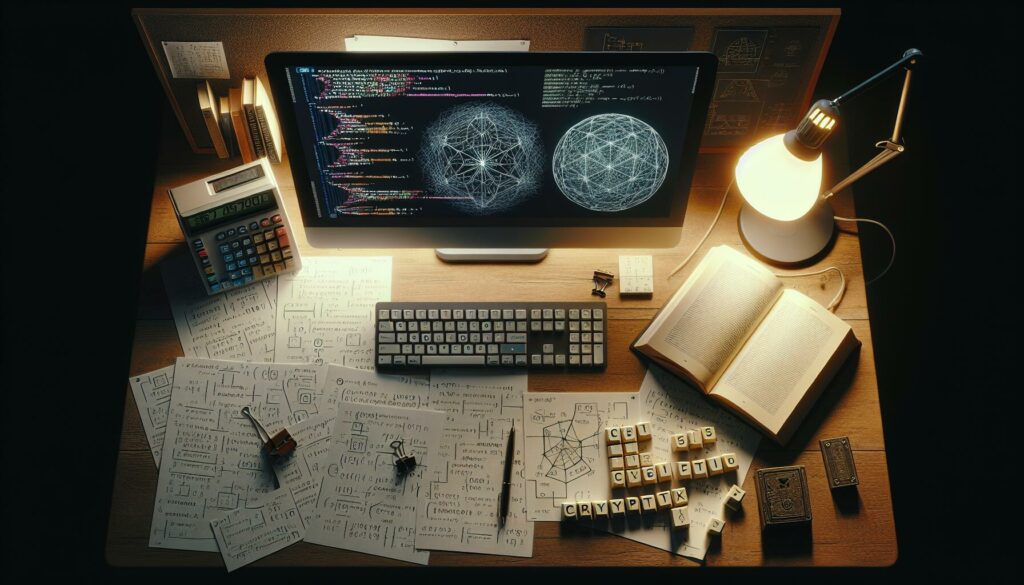
In the ever-evolving landscape of digital security, cryptographic algorithms play a pivotal role in safeguarding sensitive information. As we delve into the world of coding and programming, understanding these algorithms becomes crucial for developing secure applications and systems. This comprehensive guide will explore various cryptographic algorithms, their applications, and their significance in modern computing.
1. Introduction to Cryptography
Cryptography is the practice and study of techniques for secure communication in the presence of adversaries. It encompasses a wide range of concepts and algorithms designed to protect information from unauthorized access or tampering. Before we dive into specific algorithms, let’s review some fundamental concepts:
- Encryption: The process of converting plaintext into ciphertext to protect its confidentiality.
- Decryption: The reverse process of encryption, converting ciphertext back into plaintext.
- Key: A piece of information used in the encryption and decryption processes.
- Symmetric encryption: Uses the same key for both encryption and decryption.
- Asymmetric encryption: Uses different keys for encryption (public key) and decryption (private key).
2. Symmetric Encryption Algorithms
Symmetric encryption algorithms use the same key for both encryption and decryption. These algorithms are generally faster and more efficient than asymmetric algorithms, making them suitable for encrypting large amounts of data.
2.1 Data Encryption Standard (DES)
DES is one of the oldest symmetric encryption algorithms, developed in the 1970s. Although it is now considered insecure due to its small key size (56 bits), it laid the foundation for modern block ciphers.
2.2 Advanced Encryption Standard (AES)
AES is the current standard for symmetric encryption, replacing DES. It supports key sizes of 128, 192, and 256 bits, making it significantly more secure than its predecessor. AES operates on blocks of 128 bits and uses a series of substitution and permutation operations to achieve encryption.
Here’s a simple example of how to use AES encryption in Python using the PyCryptodome library:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_aes(plaintext, key):
cipher = AES.new(key, AES.MODE_ECB)
return cipher.encrypt(plaintext)
def decrypt_aes(ciphertext, key):
cipher = AES.new(key, AES.MODE_ECB)
return cipher.decrypt(ciphertext)
# Generate a random 256-bit key
key = get_random_bytes(32)
# Encrypt and decrypt a message
message = b'Hello, AES!'
encrypted = encrypt_aes(message, key)
decrypted = decrypt_aes(encrypted, key)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
2.3 Blowfish
Blowfish is another symmetric block cipher designed as an alternative to DES. It uses a variable-length key, from 32 to 448 bits, making it flexible for different security requirements. Blowfish is known for its speed and efficiency, especially on 32-bit processors.
2.4 Twofish
Twofish is a symmetric block cipher that was one of the finalists in the AES selection process. It supports key sizes up to 256 bits and is designed to be efficient on a wide range of platforms. Twofish is notable for its flexibility and customizable security/performance trade-offs.
3. Asymmetric Encryption Algorithms
Asymmetric encryption algorithms use a pair of keys: a public key for encryption and a private key for decryption. These algorithms are generally slower than symmetric algorithms but offer additional security features and enable secure key exchange over insecure channels.
3.1 RSA (Rivest-Shamir-Adleman)
RSA is one of the first and most widely used asymmetric encryption algorithms. It is based on the mathematical property of factoring large prime numbers. RSA can be used for both encryption and digital signatures.
Here’s a simple example of RSA encryption and decryption using Python’s pycryptodome library:
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
import binascii
def generate_rsa_keys():
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
return private_key, public_key
def encrypt_rsa(message, public_key):
key = RSA.import_key(public_key)
cipher = PKCS1_OAEP.new(key)
encrypted = cipher.encrypt(message)
return binascii.hexlify(encrypted)
def decrypt_rsa(encrypted, private_key):
key = RSA.import_key(private_key)
cipher = PKCS1_OAEP.new(key)
decrypted = cipher.decrypt(binascii.unhexlify(encrypted))
return decrypted
# Generate RSA key pair
private_key, public_key = generate_rsa_keys()
# Encrypt and decrypt a message
message = b'Hello, RSA!'
encrypted = encrypt_rsa(message, public_key)
decrypted = decrypt_rsa(encrypted, private_key)
print(f"Original: {message}")
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
3.2 Elliptic Curve Cryptography (ECC)
ECC is based on the algebraic structure of elliptic curves over finite fields. It offers comparable security to RSA with smaller key sizes, making it more efficient in terms of computational resources and bandwidth. ECC is widely used in mobile and embedded systems.
3.3 Diffie-Hellman Key Exchange
While not an encryption algorithm per se, the Diffie-Hellman key exchange protocol is a fundamental asymmetric algorithm used to securely exchange cryptographic keys over a public channel. It forms the basis for many secure communication protocols, including HTTPS.
4. Hash Functions
Hash functions are one-way cryptographic algorithms that transform input data into a fixed-size output, called a hash or digest. They are used for data integrity verification, password storage, and digital signatures.
4.1 MD5 (Message Digest algorithm 5)
MD5 is a widely used hash function that produces a 128-bit hash value. However, it is no longer considered cryptographically secure due to collision vulnerabilities.
4.2 SHA (Secure Hash Algorithm) Family
The SHA family includes several hash functions developed by the U.S. National Security Agency (NSA). The most commonly used variants are:
- SHA-1: Produces a 160-bit hash, but is no longer considered secure for cryptographic purposes.
- SHA-2: Includes SHA-256 and SHA-512, which produce 256-bit and 512-bit hashes respectively. These are widely used and considered secure.
- SHA-3: The latest member of the SHA family, designed to be more resistant to certain types of attacks.
Here’s an example of using SHA-256 in Python:
import hashlib
def sha256_hash(data):
return hashlib.sha256(data.encode()).hexdigest()
# Example usage
message = "Hello, SHA-256!"
hashed = sha256_hash(message)
print(f"Original: {message}")
print(f"SHA-256 Hash: {hashed}")
5. Digital Signatures
Digital signatures are cryptographic techniques used to verify the authenticity and integrity of digital messages or documents. They typically involve asymmetric encryption algorithms and hash functions.
5.1 DSA (Digital Signature Algorithm)
DSA is a Federal Information Processing Standard for digital signatures. It is based on the discrete logarithm problem and is widely used in government and commercial applications.
5.2 ECDSA (Elliptic Curve Digital Signature Algorithm)
ECDSA is a variant of DSA that uses elliptic curve cryptography. It offers smaller key sizes and faster computations compared to DSA, making it suitable for resource-constrained environments.
6. Practical Applications of Cryptographic Algorithms
Understanding cryptographic algorithms is crucial for developers working on secure systems. Here are some common applications:
6.1 Secure Communication Protocols
Protocols like HTTPS, SSL/TLS, and SSH use a combination of symmetric and asymmetric encryption algorithms to establish secure communication channels.
6.2 Password Hashing
Secure systems never store passwords in plaintext. Instead, they use cryptographic hash functions to store password hashes. Here’s an example using bcrypt, a popular password hashing algorithm:
import bcrypt
def hash_password(password):
# Generate a salt and hash the password
salt = bcrypt.gensalt()
hashed = bcrypt.hashpw(password.encode(), salt)
return hashed
def check_password(password, hashed):
# Check if the password matches the hash
return bcrypt.checkpw(password.encode(), hashed)
# Example usage
password = "mySecurePassword123"
hashed_password = hash_password(password)
print(f"Hashed password: {hashed_password}")
# Verify the password
is_correct = check_password(password, hashed_password)
print(f"Password is correct: {is_correct}")
6.3 File Encryption
Cryptographic algorithms are used to encrypt sensitive files and documents, ensuring that only authorized users can access the contents.
6.4 Blockchain and Cryptocurrencies
Cryptocurrencies like Bitcoin rely heavily on cryptographic algorithms for securing transactions and maintaining the integrity of the blockchain.
7. Choosing the Right Algorithm
Selecting the appropriate cryptographic algorithm depends on various factors:
- Security requirements: Consider the sensitivity of the data and the potential threats.
- Performance needs: Symmetric algorithms are generally faster than asymmetric ones.
- Key management: Asymmetric algorithms simplify key distribution but require more computational resources.
- Compliance requirements: Some industries have specific regulations regarding acceptable cryptographic algorithms.
- Platform constraints: Consider the available computational resources, especially for mobile or embedded systems.
8. Future of Cryptography
As computing power increases and new threats emerge, the field of cryptography continues to evolve. Some areas of ongoing research and development include:
8.1 Post-Quantum Cryptography
With the advent of quantum computers, many current cryptographic algorithms may become vulnerable. Post-quantum cryptography aims to develop algorithms that are resistant to attacks by quantum computers.
8.2 Homomorphic Encryption
Homomorphic encryption allows computations to be performed on encrypted data without decrypting it first. This has significant implications for cloud computing and data privacy.
8.3 Lightweight Cryptography
As the Internet of Things (IoT) grows, there’s an increasing need for cryptographic algorithms that can run efficiently on resource-constrained devices.
9. Best Practices for Implementing Cryptography
When working with cryptographic algorithms, keep these best practices in mind:
- Don’t implement cryptographic algorithms yourself: Use well-vetted libraries and implementations.
- Keep cryptographic keys secure: Use proper key management techniques and never hardcode keys in your source code.
- Use strong, random initialization vectors (IVs) and salts: This helps prevent various types of attacks.
- Stay updated: Keep your cryptographic libraries up to date to protect against newly discovered vulnerabilities.
- Use appropriate key sizes: Larger keys generally provide more security but may impact performance.
- Implement proper error handling: Don’t reveal sensitive information through error messages.
- Consider using authenticated encryption: This provides both confidentiality and integrity protection.
Conclusion
Cryptographic algorithms are the backbone of digital security, playing a crucial role in protecting sensitive information and ensuring secure communication. As a developer, understanding these algorithms and their applications is essential for building secure systems and applications.
From symmetric encryption algorithms like AES to asymmetric algorithms like RSA and ECC, each has its strengths and use cases. Hash functions and digital signatures further expand the toolkit available for securing digital assets and verifying authenticity.
As technology advances, new challenges and opportunities in cryptography continue to emerge. Staying informed about the latest developments and best practices in cryptography is crucial for any developer working in the field of information security.
By mastering these concepts and implementing them correctly, you’ll be well-equipped to tackle the security challenges of modern software development and contribute to creating a safer digital world.