String Algorithms: Searching and Manipulation
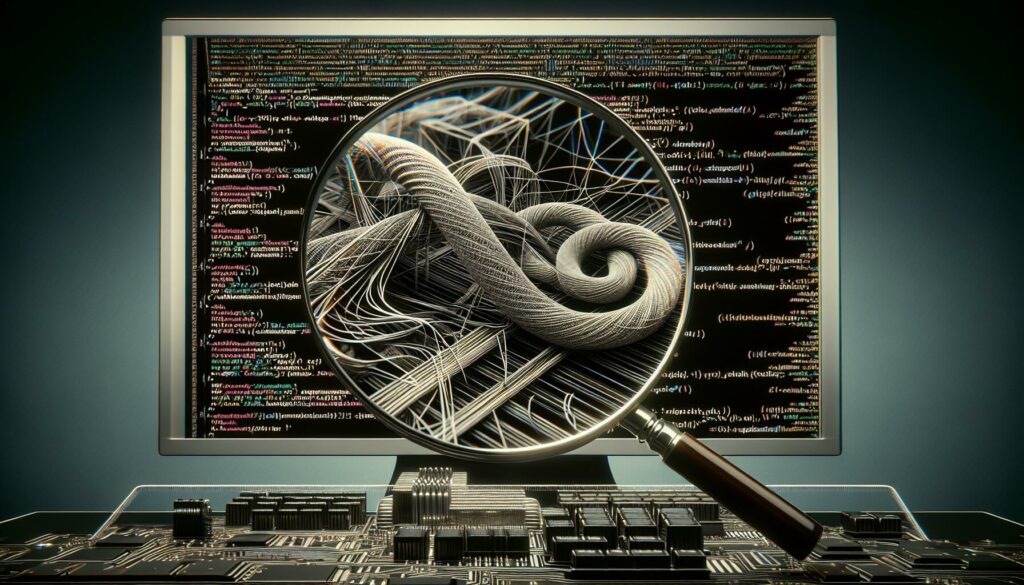
In the world of programming and computer science, strings are ubiquitous. From processing user input to analyzing large bodies of text, string manipulation is a fundamental skill that every programmer must master. In this comprehensive guide, we’ll dive deep into string algorithms, focusing on searching and manipulation techniques that are crucial for coding interviews and real-world applications.
1. Introduction to String Algorithms
String algorithms are a set of methods and techniques used to process and analyze sequences of characters. These algorithms are essential in various domains, including:
- Text processing and analysis
- Bioinformatics (DNA sequence analysis)
- Natural Language Processing (NLP)
- Cryptography
- Data compression
Understanding and implementing efficient string algorithms can significantly impact the performance of your applications, especially when dealing with large datasets.
2. Basic String Operations
Before diving into more complex algorithms, let’s review some basic string operations that form the foundation of string manipulation:
2.1. String Concatenation
Concatenation is the process of combining two or more strings. In most programming languages, this is done using the ‘+’ operator or a dedicated method.
// Python
string1 = "Hello"
string2 = "World"
result = string1 + " " + string2 // "Hello World"
// JavaScript
let string1 = "Hello";
let string2 = "World";
let result = string1.concat(" ", string2); // "Hello World"
2.2. String Length
Determining the length of a string is a common operation, often used in loops and conditional statements.
// Python
text = "AlgoCademy"
length = len(text) // 10
// JavaScript
let text = "AlgoCademy";
let length = text.length; // 10
2.3. Substring Extraction
Extracting a portion of a string is frequently used in string manipulation tasks.
// Python
text = "AlgoCademy"
substring = text[0:4] // "Algo"
// JavaScript
let text = "AlgoCademy";
let substring = text.substring(0, 4); // "Algo"
3. String Searching Algorithms
String searching is the process of finding occurrences of a pattern within a larger text. Let’s explore some popular string searching algorithms:
3.1. Naive String Matching
The naive approach involves checking every possible position in the text where the pattern could match.
def naive_search(text, pattern):
n = len(text)
m = len(pattern)
for i in range(n - m + 1):
j = 0
while j < m and text[i + j] == pattern[j]:
j += 1
if j == m:
print(f"Pattern found at index {i}")
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
naive_search(text, pattern)
Time Complexity: O(n*m), where n is the length of the text and m is the length of the pattern.
3.2. Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm improves upon the naive approach by utilizing information about the pattern to avoid unnecessary comparisons.
def kmp_search(text, pattern):
n = len(text)
m = len(pattern)
lps = [0] * m
compute_lps(pattern, lps)
i = j = 0
while i < n:
if pattern[j] == text[i]:
i += 1
j += 1
if j == m:
print(f"Pattern found at index {i - j}")
j = lps[j - 1]
elif i < n and pattern[j] != text[i]:
if j != 0:
j = lps[j - 1]
else:
i += 1
def compute_lps(pattern, lps):
length = 0
i = 1
while i < len(pattern):
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
else:
if length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
kmp_search(text, pattern)
Time Complexity: O(n + m)
3.3. Boyer-Moore Algorithm
The Boyer-Moore algorithm is known for its efficiency, especially for large alphabets. It scans the characters of the pattern from right to left and can skip portions of the text.
def boyer_moore_search(text, pattern):
n = len(text)
m = len(pattern)
if m == 0:
return
bad_char = bad_char_heuristic(pattern, m)
i = 0
while i <= n - m:
j = m - 1
while j >= 0 and pattern[j] == text[i + j]:
j -= 1
if j < 0:
print(f"Pattern found at index {i}")
i += (m - bad_char[ord(text[i + m])] if i + m < n else 1)
else:
i += max(1, j - bad_char[ord(text[i + j])])
def bad_char_heuristic(pattern, size):
bad_char = [-1] * 256
for i in range(size):
bad_char[ord(pattern[i])] = i
return bad_char
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
boyer_moore_search(text, pattern)
Time Complexity: O(n + m) in the best and average case, O(n*m) in the worst case.
4. String Manipulation Algorithms
String manipulation involves modifying, transforming, or analyzing strings. Let’s explore some common string manipulation algorithms:
4.1. Reverse a String
Reversing a string is a common interview question and a fundamental operation in many string manipulation tasks.
def reverse_string(s):
return s[::-1]
# Alternatively, using a two-pointer approach:
def reverse_string_two_pointer(s):
s = list(s)
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
text = "AlgoCademy"
print(reverse_string(text)) # "ymedaCoglA"
print(reverse_string_two_pointer(text)) # "ymedaCoglA"
4.2. Check Palindrome
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
def is_palindrome(s):
return s == s[::-1]
# Alternatively, using a two-pointer approach:
def is_palindrome_two_pointer(s):
left, right = 0, len(s) - 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
print(is_palindrome("racecar")) # True
print(is_palindrome_two_pointer("hello")) # False
4.3. Anagram Check
An anagram is a word or phrase formed by rearranging the letters of a different word or phrase.
from collections import Counter
def is_anagram(s1, s2):
return Counter(s1) == Counter(s2)
# Alternatively, using sorting:
def is_anagram_sort(s1, s2):
return sorted(s1) == sorted(s2)
print(is_anagram("listen", "silent")) # True
print(is_anagram_sort("hello", "world")) # False
4.4. String Compression
String compression is used to reduce the size of a string by replacing repeated characters with a single character followed by the count.
def compress_string(s):
if not s:
return s
compressed = []
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
compressed.append(s[i-1] + str(count))
count = 1
compressed.append(s[-1] + str(count))
compressed_str = ''.join(compressed)
return compressed_str if len(compressed_str) < len(s) else s
print(compress_string("aabcccccaaa")) # "a2b1c5a3"
print(compress_string("abcde")) # "abcde"
5. Advanced String Algorithms
Now that we’ve covered the basics, let’s explore some more advanced string algorithms that are often used in complex text processing tasks and coding interviews.
5.1. Longest Common Substring
The longest common substring problem is to find the longest string that is a substring of two or more strings.
def longest_common_substring(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
max_length = 0
end_index = 0
for i in range(1, m + 1):
for j in range(1, n + 1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
if dp[i][j] > max_length:
max_length = dp[i][j]
end_index = i
return s1[end_index - max_length:end_index]
print(longest_common_substring("ABABC", "BABCA")) # "BABC"
5.2. Longest Palindromic Substring
Finding the longest palindromic substring is a classic problem in string manipulation.
def longest_palindromic_substring(s):
if not s:
return ""
start = 0
max_length = 1
for i in range(len(s)):
# Check for odd-length palindromes
left, right = i, i
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
# Check for even-length palindromes
left, right = i, i + 1
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
return s[start:start + max_length]
print(longest_palindromic_substring("babad")) # "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # "bb"
5.3. Edit Distance (Levenshtein Distance)
The edit distance between two strings is the minimum number of operations (insertions, deletions, or substitutions) required to transform one string into another.
def edit_distance(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(m + 1):
dp[i][0] = i
for j in range(n + 1):
dp[0][j] = j
for i in range(1, m + 1):
for j in range(1, n + 1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = 1 + min(dp[i-1][j], # Deletion
dp[i][j-1], # Insertion
dp[i-1][j-1]) # Substitution
return dp[m][n]
print(edit_distance("kitten", "sitting")) # 3
print(edit_distance("sunday", "saturday")) # 3
5.4. Rabin-Karp Algorithm
The Rabin-Karp algorithm is a string-searching algorithm that uses hashing to find patterns in strings.
def rabin_karp(text, pattern):
n, m = len(text), len(pattern)
d = 256 # Number of characters in the input alphabet
q = 101 # A prime number
h = pow(d, m - 1) % q
p = t = 0
for i in range(m):
p = (d * p + ord(pattern[i])) % q
t = (d * t + ord(text[i])) % q
for i in range(n - m + 1):
if p == t:
if text[i:i+m] == pattern:
print(f"Pattern found at index {i}")
if i < n - m:
t = (d * (t - ord(text[i]) * h) + ord(text[i + m])) % q
if t < 0:
t += q
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
rabin_karp(text, pattern)
6. Practical Applications of String Algorithms
String algorithms have numerous practical applications in various fields. Let’s explore some real-world scenarios where these algorithms are crucial:
6.1. Spell Checkers
Spell checkers use string algorithms to identify misspelled words and suggest corrections. The edit distance algorithm is particularly useful in this context to find words that are similar to the misspelled word.
6.2. DNA Sequence Analysis
In bioinformatics, string algorithms are used to analyze DNA sequences. For example, the longest common substring algorithm can be used to find shared genetic markers between different species.
6.3. Plagiarism Detection
String matching algorithms are essential in plagiarism detection systems to identify similarities between documents.
6.4. Search Engines
Search engines use advanced string algorithms to efficiently index and search through vast amounts of text data.
6.5. Data Compression
String compression algorithms are used in various data compression techniques to reduce the size of text data for storage or transmission.
7. Optimizing String Algorithms
When working with string algorithms, especially in coding interviews or large-scale applications, it’s crucial to consider optimization techniques:
7.1. Use Built-in Functions
Many programming languages provide optimized built-in functions for common string operations. Use these when possible, as they are often more efficient than custom implementations.
7.2. Avoid Unnecessary String Concatenations
String concatenation can be expensive, especially in loops. Use join() method or StringBuilder (in Java) for efficient string building.
7.3. Consider Space Complexity
While optimizing for time, don’t forget about space complexity. Some algorithms may trade space for time, which might not be suitable for all scenarios.
7.4. Use Appropriate Data Structures
Choosing the right data structure can significantly impact the performance of string algorithms. For example, using a hash table for quick lookups in anagram checks.
8. Conclusion
String algorithms are a fundamental part of computer science and programming. Mastering these algorithms will not only help you in coding interviews but also in solving real-world problems efficiently. As you practice and implement these algorithms, you’ll develop a deeper understanding of string manipulation and processing techniques.
Remember, the key to mastering string algorithms is consistent practice and application. Try to implement these algorithms from scratch, analyze their time and space complexities, and experiment with different optimization techniques. As you progress, you’ll find yourself better equipped to handle complex string-related problems in your coding journey.
Keep exploring, keep coding, and don’t hesitate to dive deeper into more advanced string algorithms and their applications in various domains of computer science and software development.