Computational Geometry: Algorithms for Spatial Data
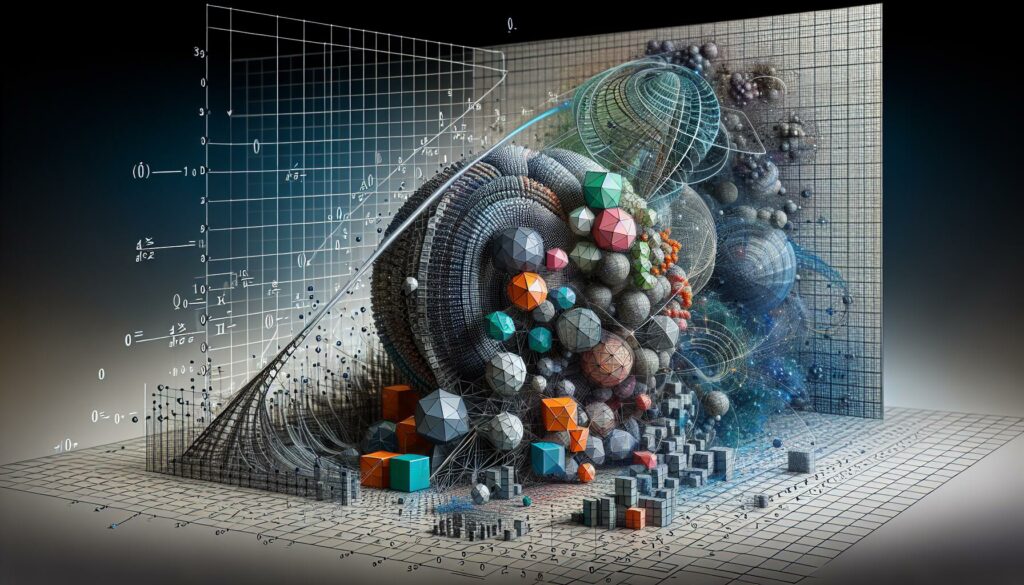
Computational geometry is a fascinating branch of computer science that focuses on developing algorithms and data structures for solving geometric problems. This field has numerous applications in areas such as computer graphics, geographic information systems (GIS), robotics, and computer-aided design (CAD). In this comprehensive guide, we’ll explore the fundamental concepts of computational geometry and delve into some of the most important algorithms for handling spatial data.
1. Introduction to Computational Geometry
Computational geometry deals with the study of algorithms for solving geometric problems. These problems often involve manipulating and analyzing spatial data, such as points, lines, polygons, and higher-dimensional objects. The field combines elements of pure mathematics, particularly geometry and topology, with algorithmic techniques from computer science.
Some of the key areas of focus in computational geometry include:
- Convex hull computation
- Polygon triangulation
- Nearest neighbor search
- Point location
- Intersection detection
- Voronoi diagrams
- Delaunay triangulations
Understanding these concepts and the algorithms associated with them is crucial for developers working on projects that involve spatial data processing or geometric computations.
2. Fundamental Data Structures
Before diving into specific algorithms, it’s important to understand the basic data structures used in computational geometry. These structures form the foundation for more complex algorithms and operations.
2.1 Point
A point is the most basic geometric object, typically represented by its coordinates in a Cartesian plane. In 2D space, a point is defined by its x and y coordinates.
class Point {
double x, y;
Point(double x, double y) {
this.x = x;
this.y = y;
}
}
2.2 Line Segment
A line segment is defined by two endpoints. It can be represented using two Point objects.
class LineSegment {
Point start, end;
LineSegment(Point start, Point end) {
this.start = start;
this.end = end;
}
}
2.3 Polygon
A polygon is a closed shape consisting of a series of connected line segments. It can be represented as a list of points, where each point is connected to the next one in the list, and the last point is connected to the first.
class Polygon {
List<Point> vertices;
Polygon(List<Point> vertices) {
this.vertices = vertices;
}
}
3. Convex Hull Algorithm
The convex hull of a set of points is the smallest convex polygon that encloses all the points. It’s a fundamental concept in computational geometry with applications in pattern recognition, image processing, and optimization problems.
3.1 Graham Scan Algorithm
One of the most popular algorithms for computing the convex hull is the Graham Scan algorithm. This algorithm has a time complexity of O(n log n), where n is the number of input points.
Here’s a high-level overview of the Graham Scan algorithm:
- Find the point with the lowest y-coordinate (and leftmost if there’s a tie).
- Sort the remaining points by polar angle with respect to the lowest point.
- Iterate through the sorted points, maintaining a stack of points that form the convex hull.
- For each point, pop points from the stack that make a non-left turn until a left turn is found.
- Push the current point onto the stack.
Here’s a Java implementation of the Graham Scan algorithm:
import java.util.*;
public class ConvexHull {
public static List<Point> grahamScan(List<Point> points) {
if (points.size() < 3) return points;
// Find the point with the lowest y-coordinate (and leftmost if tie)
Point lowest = points.get(0);
for (Point p : points) {
if (p.y < lowest.y || (p.y == lowest.y && p.x < lowest.x)) {
lowest = p;
}
}
// Sort points by polar angle with respect to the lowest point
final Point finalLowest = lowest;
points.sort((p1, p2) -> {
double angle1 = Math.atan2(p1.y - finalLowest.y, p1.x - finalLowest.x);
double angle2 = Math.atan2(p2.y - finalLowest.y, p2.x - finalLowest.x);
return Double.compare(angle1, angle2);
});
// Graham scan algorithm
Stack<Point> stack = new Stack<>();
stack.push(lowest);
for (Point p : points) {
while (stack.size() > 1 && !isLeftTurn(stack.get(stack.size() - 2), stack.peek(), p)) {
stack.pop();
}
stack.push(p);
}
return new ArrayList<>(stack);
}
private static boolean isLeftTurn(Point a, Point b, Point c) {
return ((b.x - a.x) * (c.y - a.y) - (b.y - a.y) * (c.x - a.x)) > 0;
}
}
4. Polygon Triangulation
Polygon triangulation is the process of dividing a polygon into a set of triangles. This is a crucial operation in computer graphics and computational geometry, with applications in terrain modeling, mesh generation, and computer-aided design.
4.1 Ear Clipping Algorithm
One of the simplest algorithms for triangulating a simple polygon is the ear clipping algorithm. This algorithm has a time complexity of O(n^2), where n is the number of vertices in the polygon.
The basic idea of the ear clipping algorithm is:
- Find an “ear” of the polygon (a triangle formed by three consecutive vertices that lies entirely inside the polygon).
- Remove this ear from the polygon, creating a triangle.
- Repeat steps 1 and 2 until the polygon is reduced to a single triangle.
Here’s a Java implementation of the ear clipping algorithm:
import java.util.*;
public class PolygonTriangulation {
public static List<Triangle> triangulate(List<Point> polygon) {
List<Triangle> triangles = new ArrayList<>();
List<Point> vertices = new ArrayList<>(polygon);
while (vertices.size() > 3) {
for (int i = 0; i < vertices.size(); i++) {
Point p1 = vertices.get(i);
Point p2 = vertices.get((i + 1) % vertices.size());
Point p3 = vertices.get((i + 2) % vertices.size());
if (isEar(p1, p2, p3, vertices)) {
triangles.add(new Triangle(p1, p2, p3));
vertices.remove((i + 1) % vertices.size());
break;
}
}
}
triangles.add(new Triangle(vertices.get(0), vertices.get(1), vertices.get(2)));
return triangles;
}
private static boolean isEar(Point p1, Point p2, Point p3, List<Point> polygon) {
if (!isConvex(p1, p2, p3)) return false;
for (Point p : polygon) {
if (p != p1 && p != p2 && p != p3 && isPointInTriangle(p, p1, p2, p3)) {
return false;
}
}
return true;
}
private static boolean isConvex(Point a, Point b, Point c) {
return (b.x - a.x) * (c.y - a.y) - (b.y - a.y) * (c.x - a.x) >= 0;
}
private static boolean isPointInTriangle(Point p, Point a, Point b, Point c) {
double area = 0.5 * (-b.y * c.x + a.y * (-b.x + c.x) + a.x * (b.y - c.y) + b.x * c.y);
double s = 1 / (2 * area) * (a.y * c.x - a.x * c.y + (c.y - a.y) * p.x + (a.x - c.x) * p.y);
double t = 1 / (2 * area) * (a.x * b.y - a.y * b.x + (a.y - b.y) * p.x + (b.x - a.x) * p.y);
return s > 0 && t > 0 && 1 - s - t > 0;
}
}
class Triangle {
Point p1, p2, p3;
Triangle(Point p1, Point p2, Point p3) {
this.p1 = p1;
this.p2 = p2;
this.p3 = p3;
}
}
5. Nearest Neighbor Search
Nearest neighbor search is a fundamental problem in computational geometry that involves finding the closest point in a set to a given query point. This problem has applications in various fields, including machine learning, data compression, and pattern recognition.
5.1 K-d Tree
One efficient data structure for nearest neighbor search is the k-d tree (k-dimensional tree). A k-d tree is a binary tree that recursively partitions the space along different dimensions. This structure allows for efficient searching in multidimensional space.
Here’s a basic implementation of a 2-d tree in Java:
class KdTree {
private class Node {
Point point;
Node left, right;
int depth;
Node(Point point, int depth) {
this.point = point;
this.depth = depth;
}
}
private Node root;
public void insert(Point point) {
root = insert(root, point, 0);
}
private Node insert(Node node, Point point, int depth) {
if (node == null) return new Node(point, depth);
int cd = depth % 2;
if (cd == 0) {
if (point.x < node.point.x) {
node.left = insert(node.left, point, depth + 1);
} else {
node.right = insert(node.right, point, depth + 1);
}
} else {
if (point.y < node.point.y) {
node.left = insert(node.left, point, depth + 1);
} else {
node.right = insert(node.right, point, depth + 1);
}
}
return node;
}
public Point nearestNeighbor(Point query) {
return nearestNeighbor(root, query, root.point);
}
private Point nearestNeighbor(Node node, Point query, Point best) {
if (node == null) return best;
if (distance(query, node.point) < distance(query, best)) {
best = node.point;
}
int cd = node.depth % 2;
Node goodSide, badSide;
if ((cd == 0 && query.x < node.point.x) || (cd == 1 && query.y < node.point.y)) {
goodSide = node.left;
badSide = node.right;
} else {
goodSide = node.right;
badSide = node.left;
}
best = nearestNeighbor(goodSide, query, best);
double distanceToBoundary = cd == 0 ? Math.abs(query.x - node.point.x) : Math.abs(query.y - node.point.y);
if (distanceToBoundary < distance(query, best)) {
best = nearestNeighbor(badSide, query, best);
}
return best;
}
private double distance(Point p1, Point p2) {
return Math.sqrt(Math.pow(p1.x - p2.x, 2) + Math.pow(p1.y - p2.y, 2));
}
}
6. Line Segment Intersection
Detecting intersections between line segments is a fundamental operation in computational geometry. It has applications in computer graphics, geographic information systems, and collision detection in video games.
6.1 Sweep Line Algorithm
The sweep line algorithm is an efficient method for finding all intersections among a set of line segments. The basic idea is to sweep an imaginary vertical line from left to right across the plane, maintaining a data structure of active line segments as we go.
Here’s a simplified implementation of the sweep line algorithm for detecting intersections between line segments:
import java.util.*;
public class LineIntersection {
public static List<Point> findIntersections(List<LineSegment> segments) {
List<Point> intersections = new ArrayList<>();
PriorityQueue<Event> events = new PriorityQueue<>();
// Add all segment endpoints to the event queue
for (LineSegment segment : segments) {
events.offer(new Event(segment.start, EventType.START, segment));
events.offer(new Event(segment.end, EventType.END, segment));
}
TreeSet<LineSegment> activeSegments = new TreeSet<>((a, b) -> Double.compare(a.start.y, b.start.y));
while (!events.isEmpty()) {
Event event = events.poll();
if (event.type == EventType.START) {
for (LineSegment segment : activeSegments) {
Point intersection = findIntersection(segment, event.segment);
if (intersection != null) {
intersections.add(intersection);
}
}
activeSegments.add(event.segment);
} else {
activeSegments.remove(event.segment);
}
}
return intersections;
}
private static Point findIntersection(LineSegment a, LineSegment b) {
double x1 = a.start.x, y1 = a.start.y;
double x2 = a.end.x, y2 = a.end.y;
double x3 = b.start.x, y3 = b.start.y;
double x4 = b.end.x, y4 = b.end.y;
double denom = (y4 - y3) * (x2 - x1) - (x4 - x3) * (y2 - y1);
if (denom == 0) return null; // parallel lines
double ua = ((x4 - x3) * (y1 - y3) - (y4 - y3) * (x1 - x3)) / denom;
double ub = ((x2 - x1) * (y1 - y3) - (y2 - y1) * (x1 - x3)) / denom;
if (ua < 0 || ua > 1 || ub < 0 || ub > 1) return null; // intersection outside segment
double x = x1 + ua * (x2 - x1);
double y = y1 + ua * (y2 - y1);
return new Point(x, y);
}
private enum EventType { START, END }
private static class Event implements Comparable<Event> {
Point point;
EventType type;
LineSegment segment;
Event(Point point, EventType type, LineSegment segment) {
this.point = point;
this.type = type;
this.segment = segment;
}
@Override
public int compareTo(Event other) {
return Double.compare(this.point.x, other.point.x);
}
}
}
7. Voronoi Diagrams and Delaunay Triangulations
Voronoi diagrams and Delaunay triangulations are dual structures that have numerous applications in computational geometry, including nearest neighbor search, mesh generation, and spatial analysis.
7.1 Fortune’s Algorithm for Voronoi Diagrams
Fortune’s algorithm is an efficient method for constructing Voronoi diagrams in O(n log n) time. The algorithm uses a sweep line approach, similar to the line segment intersection algorithm we discussed earlier.
Here’s a high-level overview of Fortune’s algorithm:
- Initialize a priority queue with site events (input points) and circle events.
- Maintain a beach line data structure (usually a balanced binary tree) to represent the parabolas formed by the sweep line.
- Process events in order:
- For site events, insert a new arc into the beach line.
- For circle events, remove the corresponding arc and add a Voronoi vertex.
- Update the diagram structure as events are processed.
Implementing Fortune’s algorithm is quite complex and beyond the scope of this article. However, understanding its principles is valuable for anyone working with spatial data and geometric algorithms.
7.2 Delaunay Triangulation
The Delaunay triangulation is the dual graph of the Voronoi diagram. It has the property that no point in the input set falls inside the circumcircle of any triangle in the triangulation.
One way to compute a Delaunay triangulation is to first construct the Voronoi diagram using Fortune’s algorithm, and then compute its dual. Alternatively, there are direct algorithms for Delaunay triangulation, such as the incremental algorithm and the divide-and-conquer algorithm.
8. Conclusion
Computational geometry is a rich and complex field with numerous algorithms and data structures for handling spatial data. In this article, we’ve explored some of the fundamental concepts and algorithms, including convex hulls, polygon triangulation, nearest neighbor search, line segment intersection, and Voronoi diagrams.
These algorithms form the foundation for many applications in computer graphics, geographic information systems, robotics, and more. As you continue to develop your skills in algorithmic thinking and problem-solving, understanding these geometric algorithms will prove invaluable in tackling complex spatial problems.
Remember that while we’ve provided implementations for some of these algorithms, production-ready code often requires additional optimizations and error handling. Libraries like CGAL (Computational Geometry Algorithms Library) provide robust implementations of many geometric algorithms and are worth exploring for serious computational geometry work.
As you practice and apply these concepts, you’ll develop a deeper understanding of how to efficiently manipulate and analyze spatial data, opening up new possibilities in your programming projects and career.