The Significance of Randomized Algorithms: Enhancing Efficiency and Problem-Solving in Computer Science
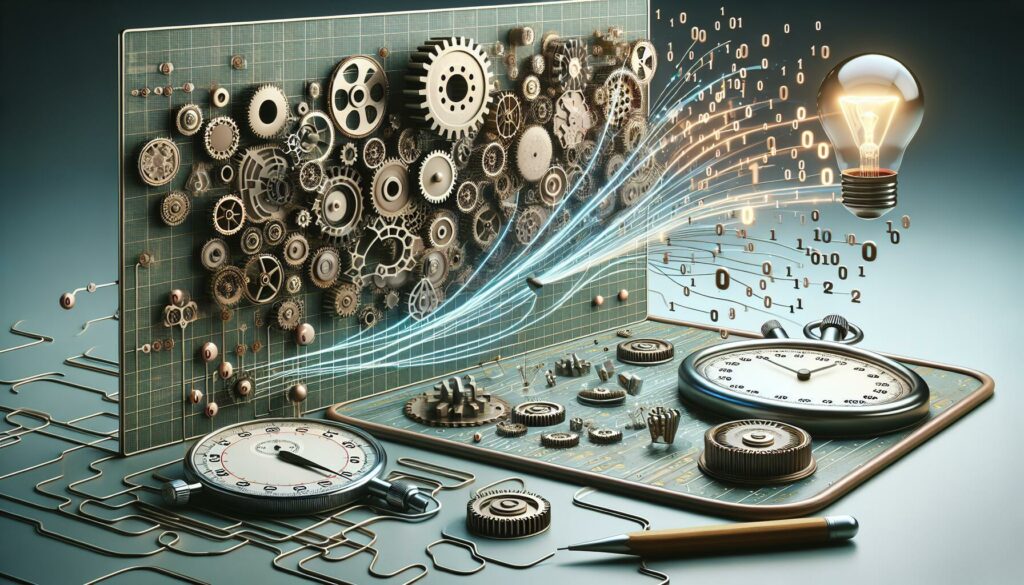
In the ever-evolving landscape of computer science and programming, randomized algorithms have emerged as a powerful tool for solving complex problems efficiently. These algorithms incorporate an element of randomness into their decision-making process, often leading to faster and more elegant solutions than their deterministic counterparts. As we delve into the world of randomized algorithms, we’ll explore their importance, applications, and how they can enhance your problem-solving skills as a programmer.
What Are Randomized Algorithms?
Randomized algorithms are computational methods that make random choices during their execution. Unlike deterministic algorithms, which always produce the same output for a given input, randomized algorithms may produce different outputs or take different paths to reach a solution. This inherent randomness allows these algorithms to overcome certain limitations and achieve better average-case performance in many scenarios.
There are two main types of randomized algorithms:
- Las Vegas algorithms: These algorithms always produce the correct result, but their running time may vary randomly.
- Monte Carlo algorithms: These algorithms have a fixed running time but may occasionally produce an incorrect result (with a bounded probability of error).
The Advantages of Randomized Algorithms
Randomized algorithms offer several advantages over their deterministic counterparts:
- Simplicity: Many randomized algorithms are simpler to design and implement than their deterministic equivalents.
- Efficiency: In many cases, randomized algorithms can achieve better average-case time complexity than the best-known deterministic algorithms for the same problem.
- Overcoming lower bounds: Randomization can help algorithms surpass theoretical lower bounds that apply to deterministic algorithms.
- Breaking symmetry: In distributed computing and game theory, randomization can help break symmetry and resolve conflicts.
- Handling worst-case scenarios: Randomized algorithms can often avoid consistently poor performance on specific input patterns that might challenge deterministic algorithms.
Key Concepts in Randomized Algorithms
To fully appreciate the power of randomized algorithms, it’s essential to understand some key concepts:
1. Probability and Expectation
Randomized algorithms rely heavily on probability theory. Understanding concepts like probability distributions, expected values, and variance is crucial for analyzing the performance of these algorithms.
2. Randomized Data Structures
Many randomized algorithms use specialized data structures that incorporate randomness. Examples include skip lists, treaps, and bloom filters. These structures often provide probabilistic guarantees on their performance.
3. Probabilistic Analysis
Analyzing randomized algorithms requires a different approach compared to deterministic algorithms. Techniques like probability bounds, Markov’s inequality, and Chernoff bounds are commonly used to prove the correctness and efficiency of randomized algorithms.
4. Derandomization
In some cases, it’s possible to convert a randomized algorithm into a deterministic one without significantly sacrificing performance. This process, known as derandomization, often involves techniques like the method of conditional probabilities.
Popular Randomized Algorithms
Let’s explore some well-known randomized algorithms to better understand their applications and benefits:
1. Quicksort with Random Pivot Selection
Quicksort is a widely used sorting algorithm known for its efficiency. By randomly selecting the pivot element, we can avoid worst-case scenarios and achieve an expected time complexity of O(n log n) for all inputs.
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = random.choice(arr)
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
2. Randomized Primality Testing (Miller-Rabin)
The Miller-Rabin primality test is a probabilistic algorithm used to determine whether a given number is prime. It’s much faster than deterministic primality tests for large numbers and is widely used in cryptographic applications.
def miller_rabin(n, k=5):
if n < 2:
return False
for p in [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]:
if n % p == 0:
return n == p
s, d = 0, n - 1
while d % 2 == 0:
s += 1
d //= 2
for _ in range(k):
a = random.randrange(2, n - 1)
x = pow(a, d, n)
if x == 1 or x == n - 1:
continue
for _ in range(s - 1):
x = pow(x, 2, n)
if x == n - 1:
break
else:
return False
return True
3. Randomized Minimum Cut Algorithm (Karger’s Algorithm)
Karger’s algorithm is a randomized algorithm for finding the minimum cut of a graph. It repeatedly contracts random edges until only two vertices remain, providing a simple and efficient approach to this complex problem.
def karger_min_cut(graph):
while len(graph) > 2:
u = random.choice(list(graph.keys()))
v = random.choice(graph[u])
merge_vertices(graph, u, v)
return len(graph[list(graph.keys())[0]])
def merge_vertices(graph, u, v):
graph[u].extend(graph[v])
for w in graph[v]:
graph[w].remove(v)
graph[w].append(u)
graph[u] = [x for x in graph[u] if x != u]
del graph[v]
4. Randomized Selection (QuickSelect)
The QuickSelect algorithm is a randomized variant of the selection algorithm used to find the k-th smallest element in an unsorted array. It has an expected time complexity of O(n), making it more efficient than sorting the entire array for this specific task.
def quick_select(arr, k):
if len(arr) == 1:
return arr[0]
pivot = random.choice(arr)
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
if k <= len(left):
return quick_select(left, k)
elif k <= len(left) + len(middle):
return pivot
else:
return quick_select(right, k - len(left) - len(middle))
Applications of Randomized Algorithms
Randomized algorithms find applications in various domains of computer science and beyond:
1. Cryptography
Randomized algorithms play a crucial role in cryptography, from generating random keys to implementing secure protocols. The unpredictability introduced by randomness is essential for maintaining security in cryptographic systems.
2. Machine Learning and Artificial Intelligence
Many machine learning algorithms incorporate randomness to improve their performance and generalization capabilities. Examples include:
- Random forests in ensemble learning
- Stochastic gradient descent in optimization
- Monte Carlo methods for approximate inference
3. Numerical Algorithms
Randomized algorithms are used in various numerical computations, including:
- Monte Carlo integration for high-dimensional integrals
- Randomized linear algebra for large matrix operations
- Simulated annealing for optimization problems
4. Graph Algorithms
Many graph problems benefit from randomized approaches, such as:
- Minimum spanning tree algorithms (e.g., Karger’s algorithm)
- Graph coloring
- Max-cut approximation
5. Data Streaming and Big Data
Randomized algorithms are particularly useful in processing large data streams and big data applications, where deterministic algorithms might be too slow or memory-intensive. Examples include:
- Bloom filters for membership testing
- Count-Min sketch for frequency estimation
- Reservoir sampling for maintaining a representative sample of a data stream
Implementing Randomized Algorithms: Best Practices
When working with randomized algorithms, consider the following best practices:
1. Choose the Right Random Number Generator
The quality of your random number generator can significantly impact the performance of your randomized algorithm. Use cryptographically secure random number generators for security-critical applications.
2. Seed Management
Properly manage random seeds to ensure reproducibility when necessary. This is especially important for debugging and testing purposes.
import random
def reproducible_random_function(seed=None):
if seed is not None:
random.seed(seed)
# Your randomized algorithm here
return result
3. Error Probability Analysis
For Monte Carlo algorithms, carefully analyze and control the probability of error. Often, running the algorithm multiple times can reduce the error probability exponentially.
4. Performance Profiling
Randomized algorithms may exhibit varying performance across different runs. Conduct thorough performance profiling with multiple runs to get a comprehensive understanding of the algorithm’s behavior.
5. Balancing Randomness and Determinism
In some cases, a hybrid approach combining randomized and deterministic elements can provide the best of both worlds. Consider such hybrid strategies when appropriate.
Challenges and Limitations of Randomized Algorithms
While randomized algorithms offer numerous advantages, they also come with certain challenges and limitations:
1. Reproducibility
The non-deterministic nature of randomized algorithms can make debugging and testing more challenging. Implementing proper seed management is crucial for reproducibility.
2. Worst-Case Guarantees
While randomized algorithms often have good average-case performance, they may lack strong worst-case guarantees. This can be a concern in certain critical applications.
3. Probabilistic Correctness
Monte Carlo algorithms may occasionally produce incorrect results. In applications where absolute correctness is required, additional verification steps or alternative approaches may be necessary.
4. Complexity Analysis
Analyzing the time and space complexity of randomized algorithms can be more involved than for deterministic algorithms, requiring probabilistic techniques and careful consideration of different scenarios.
Future Trends in Randomized Algorithms
As the field of computer science continues to evolve, we can expect to see new developments and applications of randomized algorithms:
1. Quantum Computing
The intersection of randomized algorithms and quantum computing is an exciting area of research. Quantum randomness and quantum algorithms may lead to new classes of randomized algorithms with unprecedented capabilities.
2. Privacy-Preserving Computations
Randomized algorithms are likely to play an increasingly important role in privacy-preserving computations, such as differential privacy and secure multi-party computation.
3. AI and Machine Learning
As AI and machine learning systems become more complex, randomized algorithms may find new applications in improving their efficiency, robustness, and generalization capabilities.
4. Distributed Systems and Blockchain
Randomized algorithms are expected to continue playing a crucial role in distributed systems, including blockchain technologies, for consensus mechanisms and network protocols.
Conclusion: Embracing Randomness in Algorithm Design
Randomized algorithms represent a powerful paradigm in computer science, offering elegant solutions to complex problems and often surpassing the limitations of deterministic approaches. As a programmer or computer scientist, understanding and leveraging randomized algorithms can significantly enhance your problem-solving toolkit.
By incorporating randomness into algorithm design, we can achieve better average-case performance, simplify complex procedures, and tackle problems that might be intractable with purely deterministic methods. From sorting and searching to cryptography and machine learning, the applications of randomized algorithms span a wide range of domains in computer science and beyond.
As you continue your journey in coding education and skill development, we encourage you to explore randomized algorithms further. Experiment with implementing these algorithms, analyze their performance, and consider how they might be applied to solve real-world problems. By mastering randomized algorithms, you’ll be better equipped to tackle challenging coding problems, optimize your solutions, and prepare for technical interviews at top tech companies.
Remember, the power of randomness in algorithm design is not just about introducing arbitrary choices; it’s about harnessing controlled unpredictability to achieve remarkable results. Embrace this powerful tool, and watch as it opens up new possibilities in your programming endeavors.