Implementing Heaps and Priority Queues: A Comprehensive Guide
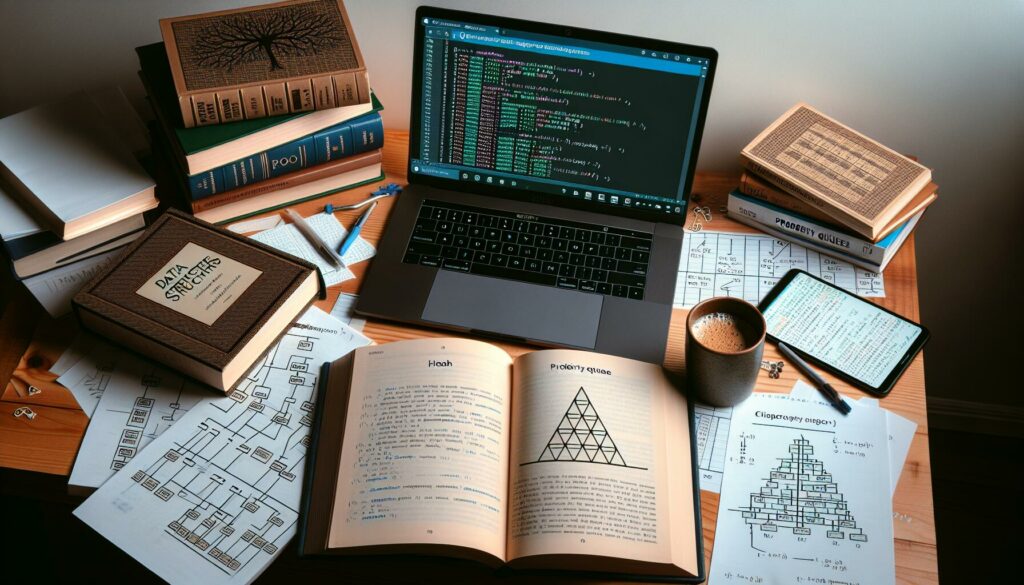
In the world of computer science and algorithms, efficient data structures play a crucial role in solving complex problems. Among these structures, heaps and priority queues stand out for their ability to maintain a collection of elements with quick access to the highest (or lowest) priority item. This article will dive deep into the implementation of heaps and priority queues, exploring their properties, operations, and real-world applications.
Table of Contents
- Understanding Heaps
- Types of Heaps
- Heap Operations
- Implementing a Binary Heap
- Priority Queues
- Implementing a Priority Queue
- Applications of Heaps and Priority Queues
- Optimization Techniques
- Common Interview Questions
- Conclusion
1. Understanding Heaps
A heap is a specialized tree-based data structure that satisfies the heap property. In a max-heap, for any given node I, the value of I is greater than or equal to the values of its children. Conversely, in a min-heap, the value of I is less than or equal to the values of its children.
Heaps are commonly implemented as binary trees, where each node has at most two children. The beauty of heaps lies in their ability to provide O(1) access to the maximum (for max-heap) or minimum (for min-heap) element, and O(log n) time for insertion and deletion operations.
2. Types of Heaps
There are several types of heaps, each with its own properties and use cases:
- Binary Heap: The most common implementation, where each node has at most two children.
- Fibonacci Heap: A more complex structure that provides better amortized performance for some operations.
- Binomial Heap: A heap similar to a binary heap but also allows for efficient merging of heaps.
- Leftist Heap: A variant of binary heap that supports efficient merging operations.
- Skew Heap: A self-adjusting form of leftist heap with simpler implementation.
For this article, we’ll focus primarily on binary heaps, as they are the most commonly used and form the basis for understanding more complex heap structures.
3. Heap Operations
The fundamental operations that define a heap’s functionality are:
- Insert: Add a new element to the heap while maintaining the heap property.
- Delete: Remove the root element (maximum in max-heap, minimum in min-heap) and reorganize the heap.
- Peek: View the root element without removing it.
- Heapify: Convert an array into a heap structure.
Let’s explore each of these operations in detail:
3.1 Insert Operation
Insertion in a heap follows these steps:
- Add the new element to the end of the heap.
- Compare the added element with its parent.
- If the heap property is violated, swap the element with its parent.
- Repeat steps 2-3 until the heap property is satisfied.
This process is often called “bubble-up” or “sift-up”.
3.2 Delete Operation
Deletion (usually of the root element) involves:
- Replace the root with the last element in the heap.
- Remove the last element.
- Compare the new root with its children.
- If the heap property is violated, swap the element with its largest (for max-heap) or smallest (for min-heap) child.
- Repeat steps 3-4 until the heap property is satisfied.
This process is known as “bubble-down” or “sift-down”.
3.3 Peek Operation
Peeking is straightforward – it simply returns the value of the root node without modifying the heap structure.
3.4 Heapify Operation
Heapify converts an array into a heap. It can be done in two ways:
- Bottom-up approach: Start from the last non-leaf node and sift down each element.
- Top-down approach: Insert elements one by one into an initially empty heap.
The bottom-up approach is generally more efficient, with a time complexity of O(n) compared to O(n log n) for the top-down approach.
4. Implementing a Binary Heap
Now that we understand the concepts, let’s implement a binary max-heap in Python:
class MaxHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def insert(self, key):
self.heap.append(key)
self._sift_up(len(self.heap) - 1)
def _sift_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] > self.heap[parent]:
self.swap(i, parent)
self._sift_up(parent)
def extract_max(self):
if len(self.heap) == 0:
return None
if len(self.heap) == 1:
return self.heap.pop()
max_val = self.heap[0]
self.heap[0] = self.heap.pop()
self._sift_down(0)
return max_val
def _sift_down(self, i):
max_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] > self.heap[max_index]:
max_index = left
if right < len(self.heap) and self.heap[right] > self.heap[max_index]:
max_index = right
if i != max_index:
self.swap(i, max_index)
self._sift_down(max_index)
def peek(self):
if self.heap:
return self.heap[0]
return None
This implementation provides the basic operations of a max-heap: insertion, extraction of the maximum element, and peeking at the maximum element.
5. Priority Queues
A priority queue is an abstract data type that operates similarly to a regular queue, with the addition that each element has a “priority” associated with it. In a priority queue, an element with high priority is served before an element with low priority.
While priority queues can be implemented using various data structures, heaps are often the most efficient choice. The highest (or lowest) priority element is always at the root of the heap, which allows for constant-time retrieval.
6. Implementing a Priority Queue
Let’s implement a priority queue using our MaxHeap class:
class PriorityQueue:
def __init__(self):
self.heap = MaxHeap()
def enqueue(self, item, priority):
self.heap.insert((priority, item))
def dequeue(self):
if self.is_empty():
return None
return self.heap.extract_max()[1]
def peek(self):
if self.is_empty():
return None
return self.heap.peek()[1]
def is_empty(self):
return len(self.heap.heap) == 0
In this implementation, we use tuples to store both the priority and the item. The MaxHeap will automatically order these tuples based on the priority (the first element of the tuple).
7. Applications of Heaps and Priority Queues
Heaps and priority queues find applications in various algorithms and real-world scenarios:
- Dijkstra’s algorithm: Uses a priority queue to efficiently find the shortest path in a graph.
- Huffman coding: Employs a priority queue in building optimal prefix codes for data compression.
- Task scheduling: In operating systems, priority queues can manage task execution based on priority levels.
- Event-driven simulation: Priority queues can manage events based on their scheduled time.
- Heap sort: An efficient sorting algorithm that uses a heap to sort elements.
- K-way merge: Efficiently merges k sorted arrays using a heap.
- Media streaming: Can prioritize packets based on their importance in video streaming applications.
8. Optimization Techniques
While the basic implementation of heaps and priority queues is efficient for most use cases, there are several optimization techniques that can enhance performance in specific scenarios:
8.1 Decrease-Key Operation
Some applications require updating the priority of an element in the queue. This operation, known as decrease-key (or increase-key for max-heaps), can be optimized in advanced heap implementations like Fibonacci heaps.
8.2 Lazy Deletion
Instead of removing elements immediately, mark them as deleted. This can be more efficient when combined with periodic cleanup operations.
8.3 Cache-Friendly Implementations
Store the heap in a contiguous array to improve cache performance. This is one reason why binary heaps are often preferred in practice despite theoretically slower asymptotic performance for some operations compared to more complex heap structures.
8.4 Bulk Operations
For scenarios where multiple elements are inserted or removed at once, implementing bulk operations can be more efficient than performing individual operations.
9. Common Interview Questions
Heaps and priority queues are popular topics in technical interviews. Here are some common questions you might encounter:
- Implement a min-heap from scratch.
- Given an array of integers, find the kth largest element. (Hint: Use a min-heap of size k)
- Merge k sorted linked lists. (Hint: Use a min-heap to efficiently select the next element)
- Implement a median finder. (Hint: Use two heaps – a max-heap for the lower half and a min-heap for the upper half)
- Design a system to continuously find the median of a data stream.
Let’s solve one of these problems to illustrate how heaps can be used in algorithmic problem-solving:
Finding the kth Largest Element
import heapq
def find_kth_largest(nums, k):
# Create a min-heap
heap = []
for num in nums:
# If the heap size is less than k, just add the element
if len(heap) < k:
heapq.heappush(heap, num)
# If the current number is larger than the smallest in the heap,
# remove the smallest and add the current number
elif num > heap[0]:
heapq.heapreplace(heap, num)
# The root of the heap will be the kth largest element
return heap[0]
# Example usage
nums = [3, 2, 1, 5, 6, 4]
k = 2
print(f"The {k}th largest element is: {find_kth_largest(nums, k)}")
This solution maintains a min-heap of size k. After processing all numbers, the root of the heap will be the kth largest element. The time complexity of this solution is O(n log k), which is more efficient than sorting the entire array when k is significantly smaller than n.
10. Conclusion
Heaps and priority queues are powerful data structures that play a crucial role in many algorithms and real-world applications. Their ability to efficiently maintain a collection of elements with quick access to the highest (or lowest) priority item makes them indispensable in scenarios ranging from graph algorithms to system design.
As you continue your journey in computer science and prepare for technical interviews, mastering heaps and priority queues will significantly enhance your problem-solving toolkit. Practice implementing these structures from scratch, solve problems that utilize them, and explore their applications in various domains.
Remember, the key to truly understanding these concepts is through consistent practice and application. Try to identify scenarios in your own projects where heaps or priority queues could optimize performance, and don’t hesitate to dive deeper into more advanced heap structures as you progress in your studies.
Happy coding, and may your algorithms always be efficient!