Understanding Memory Management in Algorithms
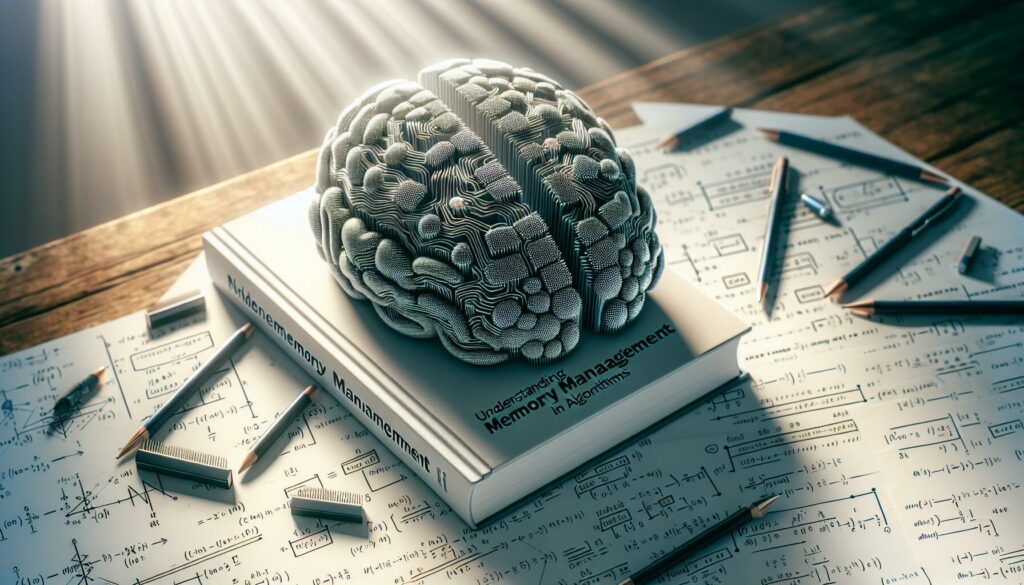
Memory management is a crucial aspect of algorithm design and implementation. Efficient memory usage can significantly impact the performance and scalability of your algorithms. In this comprehensive guide, we’ll dive deep into the world of memory management in algorithms, exploring various techniques, best practices, and common pitfalls to avoid.
Table of Contents
- Introduction to Memory Management
- Importance of Efficient Memory Management
- Types of Memory
- Memory Allocation Techniques
- Memory Deallocation and Garbage Collection
- Memory-Efficient Data Structures
- Memory-Optimized Algorithms
- Detecting and Preventing Memory Leaks
- Best Practices for Memory Management
- Tools for Memory Profiling and Analysis
- Case Studies: Memory Optimization in Real-World Scenarios
- Future Trends in Memory Management
- Conclusion
1. Introduction to Memory Management
Memory management is the process of controlling and coordinating computer memory, assigning portions called blocks to various running programs to optimize overall system performance. In the context of algorithms, it involves efficiently allocating, using, and freeing memory resources to ensure optimal execution of your code.
Understanding memory management is essential for several reasons:
- Improved performance: Efficient memory usage leads to faster algorithm execution.
- Scalability: Proper memory management allows algorithms to handle larger datasets and more complex operations.
- Resource optimization: It helps in making the best use of available system resources.
- Bug prevention: Many common programming errors, such as memory leaks and segmentation faults, can be avoided with good memory management practices.
2. Importance of Efficient Memory Management
Efficient memory management is crucial for several reasons:
2.1 Performance Optimization
Proper memory management can significantly improve the performance of your algorithms. By minimizing unnecessary memory allocations and deallocations, you can reduce the overhead associated with memory operations, leading to faster execution times.
2.2 Resource Utilization
Efficient memory management ensures that your algorithm makes the best use of available system resources. This is particularly important when working with limited memory environments or when dealing with large datasets that push the limits of available memory.
2.3 Scalability
As your algorithms grow in complexity and the size of input data increases, efficient memory management becomes even more critical. Well-managed memory allows your algorithms to scale gracefully, handling larger inputs without excessive memory consumption.
2.4 Reliability
Proper memory management helps prevent common issues such as memory leaks, buffer overflows, and dangling pointers. These problems can lead to crashes, unpredictable behavior, and security vulnerabilities in your software.
3. Types of Memory
Before diving into memory management techniques, it’s essential to understand the different types of memory available to your algorithms:
3.1 Stack Memory
Stack memory is used for storing local variables and function call information. It’s automatically managed by the system and follows a Last-In-First-Out (LIFO) structure. Stack memory is typically faster to allocate and deallocate but has limited size.
3.2 Heap Memory
Heap memory is used for dynamic memory allocation. It allows for more flexible memory management but requires manual allocation and deallocation in languages without garbage collection. Heap memory is larger than stack memory but slower to access.
3.3 Static/Global Memory
This memory is allocated at compile-time and exists throughout the program’s lifetime. It’s used for storing global variables and static local variables.
3.4 Text/Code Memory
This read-only memory segment stores the compiled program code.
4. Memory Allocation Techniques
Understanding various memory allocation techniques is crucial for efficient algorithm implementation. Let’s explore some common methods:
4.1 Static Allocation
Static allocation involves reserving memory at compile-time. This is typically used for fixed-size arrays and global variables. While it’s simple and efficient, it lacks flexibility for dynamic data structures.
Example in C:
int staticArray[100]; // Static allocation of an array with 100 integers
4.2 Dynamic Allocation
Dynamic allocation allows for memory to be allocated at runtime. This provides more flexibility but requires manual management in languages without garbage collection.
Example in C:
int* dynamicArray = (int*)malloc(100 * sizeof(int)); // Dynamic allocation of 100 integers
// Don't forget to free the memory when done
free(dynamicArray);
4.3 Automatic Allocation
Automatic allocation occurs for local variables within functions. The memory is automatically allocated when the function is called and deallocated when it returns.
Example in C++:
void function() {
int localVar = 10; // Automatically allocated on the stack
// No need to manually free localVar
}
4.4 Memory Pools
Memory pools involve pre-allocating a large chunk of memory and managing it for smaller allocations. This can improve performance by reducing the number of system calls for memory allocation.
Example concept in C++:
class MemoryPool {
char* memory;
size_t size;
size_t used;
public:
MemoryPool(size_t size) : size(size), used(0) {
memory = new char[size];
}
void* allocate(size_t bytes) {
if (used + bytes > size) return nullptr; // Out of memory
void* result = &memory[used];
used += bytes;
return result;
}
// ... (deallocation and other methods)
};
5. Memory Deallocation and Garbage Collection
Proper memory deallocation is crucial to prevent memory leaks and ensure efficient resource utilization. There are two main approaches to memory deallocation:
5.1 Manual Deallocation
In languages like C and C++, memory must be manually deallocated when it’s no longer needed. This gives the programmer full control but also increases the risk of memory leaks if not done properly.
Example in C:
int* array = (int*)malloc(100 * sizeof(int));
// Use the array...
free(array); // Manual deallocation
5.2 Garbage Collection
Many modern languages like Java, Python, and JavaScript use garbage collection to automatically identify and free unused memory. While this reduces the burden on the programmer, it can introduce performance overhead and less predictable memory usage patterns.
Example in Java:
List<Integer> list = new ArrayList<>();
// Use the list...
// No need to manually free; the garbage collector will handle it
5.3 Reference Counting
Some languages use reference counting as a form of automatic memory management. Each object keeps track of how many references point to it, and when the count reaches zero, the object is deallocated.
Example concept in Python:
class RefCounted:
def __init__(self):
self._ref_count = 0
def inc_ref(self):
self._ref_count += 1
def dec_ref(self):
self._ref_count -= 1
if self._ref_count == 0:
self._cleanup()
def _cleanup(self):
# Perform cleanup operations
pass
6. Memory-Efficient Data Structures
Choosing the right data structure can significantly impact memory usage in your algorithms. Let’s explore some memory-efficient data structures:
6.1 Arrays
Arrays are contiguous blocks of memory and are generally memory-efficient for storing and accessing elements. However, they have a fixed size, which can lead to wasted space or expensive resizing operations.
6.2 Linked Lists
Linked lists can be more memory-efficient than arrays when the size of the data is unknown or frequently changing. However, they have higher memory overhead per element due to storing pointers.
6.3 Hash Tables
Hash tables provide fast access times but can be memory-intensive due to potential unused buckets. Open addressing techniques can help reduce memory usage compared to chaining.
6.4 Tries
Tries are efficient for storing and searching strings, especially when there are many common prefixes. They can be more memory-efficient than storing complete strings in some scenarios.
6.5 Bit Arrays
Bit arrays can be extremely memory-efficient for storing boolean values or sets of small integers. Each bit represents a boolean value, allowing you to store 8 times more elements compared to using bytes.
Example of a simple bit array in C++:
class BitArray {
std::vector<unsigned char> data;
size_t size;
public:
BitArray(size_t size) : size(size) {
data.resize((size + 7) / 8, 0);
}
void set(size_t index, bool value) {
if (index >= size) return;
size_t byte_index = index / 8;
size_t bit_index = index % 8;
if (value) {
data[byte_index] |= (1 << bit_index);
} else {
data[byte_index] &= ~(1 << bit_index);
}
}
bool get(size_t index) {
if (index >= size) return false;
size_t byte_index = index / 8;
size_t bit_index = index % 8;
return (data[byte_index] & (1 << bit_index)) != 0;
}
};
7. Memory-Optimized Algorithms
Many classic algorithms can be optimized for better memory usage. Let’s explore some examples:
7.1 In-Place Sorting Algorithms
In-place sorting algorithms, such as QuickSort and HeapSort, sort the array without requiring significant extra memory. This makes them more memory-efficient compared to algorithms like MergeSort, which typically require additional space proportional to the input size.
Example of in-place QuickSort in Python:
def quicksort(arr, low, high):
if low < high:
pi = partition(arr, low, high)
quicksort(arr, low, pi - 1)
quicksort(arr, pi + 1, high)
def partition(arr, low, high):
pivot = arr[high]
i = low - 1
for j in range(low, high):
if arr[j] <= pivot:
i += 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return i + 1
# Usage
arr = [10, 7, 8, 9, 1, 5]
quicksort(arr, 0, len(arr) - 1)
print(arr) # Output: [1, 5, 7, 8, 9, 10]
7.2 Streaming Algorithms
Streaming algorithms process data in a single pass, using only a small amount of memory regardless of the input size. These are particularly useful for processing large datasets that don’t fit entirely in memory.
Example: Reservoir sampling for selecting a random sample from a stream
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if len(reservoir) < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Usage
stream = range(1000000) # Simulating a large stream
sample = reservoir_sampling(stream, 10)
print(sample) # Output: A random sample of 10 items from the stream
7.3 Divide and Conquer Algorithms
Divide and conquer algorithms can be memory-efficient when implemented carefully. By processing subproblems recursively and combining results, they can avoid storing large intermediate results.
7.4 Dynamic Programming with Space Optimization
Many dynamic programming solutions can be optimized to use less memory by only storing the necessary previous states instead of the entire DP table.
Example: Space-optimized Fibonacci calculation
def fibonacci(n):
if n <= 1:
return n
prev, curr = 0, 1
for _ in range(2, n + 1):
prev, curr = curr, prev + curr
return curr
print(fibonacci(10)) # Output: 55
8. Detecting and Preventing Memory Leaks
Memory leaks occur when allocated memory is not properly freed, leading to gradual memory consumption over time. Detecting and preventing memory leaks is crucial for maintaining the long-term stability and performance of your algorithms.
8.1 Common Causes of Memory Leaks
- Forgetting to free dynamically allocated memory
- Losing the last reference to an allocated object
- Circular references in garbage-collected languages
- Improperly implemented custom memory management
8.2 Techniques for Detecting Memory Leaks
- Use memory profiling tools (e.g., Valgrind for C/C++, memory_profiler for Python)
- Implement logging and tracking of memory allocations and deallocations
- Regularly monitor memory usage during long-running processes
- Use smart pointers in C++ to automate memory management
8.3 Preventing Memory Leaks
- Always pair allocations with deallocations
- Use RAII (Resource Acquisition Is Initialization) in C++
- Implement proper error handling to ensure resources are freed in case of exceptions
- Be cautious with circular references in garbage-collected languages
- Use weak references when appropriate to break reference cycles
Example of RAII in C++:
class ResourceManager {
int* resource;
public:
ResourceManager() : resource(new int[100]) {}
~ResourceManager() { delete[] resource; }
// Disable copy to prevent double deletion
ResourceManager(const ResourceManager&) = delete;
ResourceManager& operator=(const ResourceManager&) = delete;
// Move semantics if needed
ResourceManager(ResourceManager&& other) noexcept : resource(other.resource) {
other.resource = nullptr;
}
ResourceManager& operator=(ResourceManager&& other) noexcept {
if (this != &other) {
delete[] resource;
resource = other.resource;
other.resource = nullptr;
}
return *this;
}
};
9. Best Practices for Memory Management
Adhering to best practices can significantly improve the memory efficiency and reliability of your algorithms. Here are some key guidelines:
9.1 Use Appropriate Data Structures
Choose data structures that best fit your algorithm’s needs and memory constraints. Consider factors like access patterns, insertion/deletion frequency, and expected data size.
9.2 Minimize Copying
Avoid unnecessary copying of data, especially for large objects. Use references or pointers when possible, and consider move semantics in C++.
9.3 Reuse Memory
When possible, reuse existing memory allocations instead of repeatedly allocating and deallocating memory. This can be achieved through object pooling or by resizing existing containers.
9.4 Be Mindful of Memory Alignment
Proper memory alignment can improve performance and reduce memory fragmentation. Most modern compilers handle this automatically, but it’s important to be aware of alignment issues when working with low-level memory management.
9.5 Use Smart Pointers
In C++, use smart pointers like std::unique_ptr and std::shared_ptr to automate memory management and reduce the risk of memory leaks.
9.6 Profile and Optimize
Regularly profile your code to identify memory bottlenecks and optimize accordingly. Don’t prematurely optimize; focus on areas that have the most significant impact on performance and memory usage.
9.7 Consider Memory-Mapped Files
For large datasets, memory-mapped files can provide efficient access without loading the entire file into memory.
9.8 Use Lazy Initialization
Initialize resources only when they are needed to avoid unnecessary memory allocation.
9.9 Be Cautious with Recursion
Recursive algorithms can lead to stack overflow for deep recursions. Consider iterative alternatives or tail recursion optimization where possible.
10. Tools for Memory Profiling and Analysis
Various tools can help you analyze and optimize memory usage in your algorithms:
10.1 Valgrind
Valgrind is a powerful tool suite for debugging and profiling. Its Memcheck tool is particularly useful for detecting memory leaks and other memory-related errors in C and C++ programs.
10.2 Address Sanitizer (ASan)
ASan is a fast memory error detector for C/C++. It’s part of the LLVM project and can be used with GCC and Clang compilers.
10.3 Memory Profiler for Python
The memory_profiler module allows line-by-line analysis of memory consumption in Python programs.
10.4 Java VisualVM
VisualVM is a visual tool integrating command-line JDK tools and lightweight profiling capabilities for the Java platform.
10.5 Instruments (for iOS/macOS)
Instruments is a powerful tool for analyzing the performance of iOS and macOS applications, including memory usage and leaks.
10.6 Windows Performance Analyzer
This tool provides detailed performance analysis capabilities for Windows applications, including memory usage tracking.
11. Case Studies: Memory Optimization in Real-World Scenarios
Let’s examine some real-world scenarios where memory optimization played a crucial role in improving algorithm performance:
11.1 Case Study 1: Optimizing a Web Crawler
A web crawler was experiencing memory issues when processing large websites. The team implemented the following optimizations:
- Used a streaming HTML parser to avoid loading entire pages into memory
- Implemented a URL frontier with disk-based storage for managing the crawl queue
- Employed memory-mapped files for storing intermediate results
These changes reduced memory usage by 60% and allowed the crawler to handle websites 5 times larger than before.
11.2 Case Study 2: Improving a Machine Learning Pipeline
A data science team was working on a machine learning pipeline that processed large datasets. They made the following improvements:
- Implemented online learning algorithms to process data in batches
- Used sparse matrix representations for high-dimensional, sparse data
- Employed feature hashing to reduce memory usage for categorical variables
These optimizations allowed them to train models on datasets 10 times larger without increasing memory requirements.
11.3 Case Study 3: Optimizing a Mobile Game Engine
A game development team was struggling with memory constraints on mobile devices. They implemented these optimizations:
- Developed a custom memory allocator optimized for small, frequent allocations
- Implemented object pooling for frequently created and destroyed game objects
- Used texture atlases to reduce memory fragmentation and improve rendering performance
These changes resulted in a 40% reduction in memory usage and eliminated most out-of-memory crashes on low-end devices.
12. Future Trends in Memory Management
As technology evolves, new trends in memory management are emerging:
12.1 Non-Volatile Memory (NVM)
The advent of non-volatile memory technologies like Intel’s Optane is blurring the line between storage and memory. This may lead to new programming models and memory management techniques that can leverage the persistence and large capacity of NVM.
12.2 Heterogeneous Memory Architectures
Future systems may incorporate different types of memory with varying performance characteristics. Algorithms and memory managers will need to be adapted to efficiently utilize these heterogeneous memory systems.
12.3 Quantum Computing
As quantum computing advances, new paradigms for managing quantum memory and dealing with quantum decoherence will need to be developed.
12.4 AI-Assisted Memory Management
Machine learning techniques may be employed to predict memory usage patterns and optimize memory allocation and deallocation strategies dynamically.
12.5 Advanced Garbage Collection Techniques
Research into more efficient and less intrusive garbage collection algorithms continues, with a focus on reducing pause times and improving performance for large-scale applications.
13. Conclusion
Efficient memory management is a critical skill for any programmer working on algorithms and data structures. By understanding the principles of memory allocation, choosing appropriate data structures, and following best practices, you can significantly improve the performance and reliability of your algorithms.
Remember that memory optimization is often a balancing act between efficiency and code complexity. Always profile your code and focus your optimization efforts where they will have the most significant impact. As you gain experience, you’ll develop an intuition for writing memory-efficient code from the start.
As we look to the future, new hardware technologies and programming paradigms will continue to shape the landscape of memory management. Staying informed about these developments and adapting your skills accordingly will be crucial for maintaining expertise in this fundamental aspect of computer science.
By mastering memory management, you’ll not only write better algorithms but also gain a deeper understanding of how computers work at a fundamental level. This knowledge will serve you well throughout your programming career, whether you’re developing high-performance systems, working on resource-constrained devices, or tackling complex algorithmic challenges in competitive programming.