The Power of Divide and Conquer Algorithms: Mastering Efficient Problem-Solving
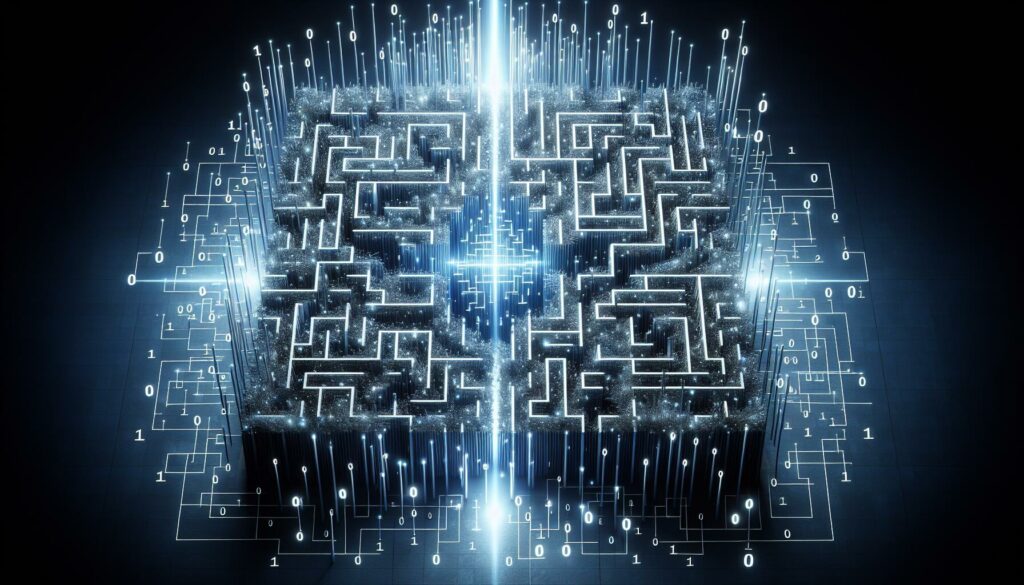
In the world of computer science and programming, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and solve complex problems with elegance and speed. One of the most powerful techniques in our algorithmic arsenal is the divide and conquer approach. This strategy has revolutionized the way we tackle large-scale problems, breaking them down into manageable pieces and paving the way for some of the most efficient algorithms in use today.
In this comprehensive guide, we’ll dive deep into the world of divide and conquer algorithms, exploring their principles, applications, and why they’re so crucial for aspiring programmers and seasoned developers alike. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your problem-solving skills, understanding divide and conquer is essential.
What is Divide and Conquer?
At its core, divide and conquer is a problem-solving paradigm that breaks down a complex problem into smaller, more manageable subproblems. The approach follows three main steps:
- Divide: Break the original problem into smaller subproblems.
- Conquer: Solve these subproblems recursively.
- Combine: Merge the solutions of the subproblems to create a solution to the original problem.
This strategy is particularly effective for problems that exhibit optimal substructure, meaning the optimal solution to the larger problem can be constructed from optimal solutions to its subproblems.
The Advantages of Divide and Conquer
Divide and conquer algorithms offer several significant advantages:
- Efficiency: Many divide and conquer algorithms have logarithmic time complexities, making them highly efficient for large datasets.
- Parallelization: The independent nature of subproblems often allows for parallel processing, further improving performance.
- Simplicity: Breaking complex problems into smaller, more manageable parts can lead to cleaner, more understandable code.
- Recursion: The recursive nature of these algorithms often results in elegant and concise implementations.
Classic Examples of Divide and Conquer Algorithms
Let’s explore some of the most well-known and widely used divide and conquer algorithms:
1. Merge Sort
Merge Sort is a classic example of the divide and conquer approach. It sorts an array by repeatedly dividing it into two halves, sorting them, and then merging the sorted halves.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Merge Sort has a time complexity of O(n log n), making it efficient for large datasets. It’s stable, meaning it preserves the relative order of equal elements, and its performance is consistent regardless of the initial order of the input.
2. Quick Sort
Quick Sort is another efficient sorting algorithm that uses the divide and conquer strategy. It works by selecting a ‘pivot’ element and partitioning the array around it.
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
arr = [3, 6, 8, 10, 1, 2, 1]
sorted_arr = quick_sort(arr)
print(sorted_arr) # Output: [1, 1, 2, 3, 6, 8, 10]
Quick Sort has an average-case time complexity of O(n log n), but can degrade to O(n^2) in the worst case. However, with good pivot selection strategies, it often outperforms other sorting algorithms in practice.
3. Binary Search
Binary Search is a highly efficient search algorithm that works on sorted arrays. It repeatedly divides the search interval in half, significantly reducing the search space.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(arr, target)
print(f"Target found at index: {result}") # Output: Target found at index: 3
Binary Search has a time complexity of O(log n), making it extremely efficient for large sorted datasets. It’s widely used in various applications, including database searches and computer graphics.
Advanced Divide and Conquer Algorithms
While the previous examples are foundational, there are more advanced divide and conquer algorithms that tackle complex problems efficiently:
1. Strassen’s Matrix Multiplication
Strassen’s algorithm is a clever divide and conquer approach to matrix multiplication. It reduces the number of recursive calls from 8 (in the naive recursive algorithm) to 7, resulting in a time complexity of approximately O(n^2.8) instead of O(n^3) for large matrices.
import numpy as np
def strassen(A, B):
n = A.shape[0]
if n <= 32: # Base case: use standard multiplication for small matrices
return A @ B
# Divide matrices into quadrants
mid = n // 2
A11 = A[:mid, :mid]
A12 = A[:mid, mid:]
A21 = A[mid:, :mid]
A22 = A[mid:, mid:]
B11 = B[:mid, :mid]
B12 = B[:mid, mid:]
B21 = B[mid:, :mid]
B22 = B[mid:, mid:]
# Compute 7 products recursively
P1 = strassen(A11 + A22, B11 + B22)
P2 = strassen(A21 + A22, B11)
P3 = strassen(A11, B12 - B22)
P4 = strassen(A22, B21 - B11)
P5 = strassen(A11 + A12, B22)
P6 = strassen(A21 - A11, B11 + B12)
P7 = strassen(A12 - A22, B21 + B22)
# Compute quadrants of the result
C11 = P1 + P4 - P5 + P7
C12 = P3 + P5
C21 = P2 + P4
C22 = P1 - P2 + P3 + P6
# Combine quadrants into the result matrix
C = np.vstack((np.hstack((C11, C12)), np.hstack((C21, C22))))
return C
# Example usage
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
result = strassen(A, B)
print(result)
While Strassen’s algorithm is theoretically faster for very large matrices, it’s worth noting that the constant factors and the complexity of implementation often make it less practical for smaller matrices compared to optimized versions of the standard algorithm.
2. Karatsuba Algorithm for Fast Multiplication
The Karatsuba algorithm is a fast multiplication algorithm that uses divide and conquer to multiply two large numbers more efficiently than the grade-school method.
def karatsuba(x, y):
if x < 10 or y < 10:
return x * y
n = max(len(str(x)), len(str(y)))
m = n // 2
high1, low1 = divmod(x, 10**m)
high2, low2 = divmod(y, 10**m)
z0 = karatsuba(low1, low2)
z1 = karatsuba((low1 + high1), (low2 + high2))
z2 = karatsuba(high1, high2)
return (z2 * 10**(2*m)) + ((z1 - z2 - z0) * 10**m) + z0
# Example usage
result = karatsuba(1234, 5678)
print(result) # Output: 7006652
The Karatsuba algorithm reduces the number of single-digit multiplications from n^2 to approximately n^1.585, making it more efficient for multiplying very large numbers.
Applying Divide and Conquer to Real-World Problems
The divide and conquer paradigm extends far beyond these classic algorithms. Let’s explore how this approach can be applied to solve more complex, real-world problems:
1. Closest Pair of Points
Finding the closest pair of points in a set of points in a 2D plane is a common problem in computational geometry. A naive approach would compare all pairs of points, resulting in O(n^2) time complexity. However, using a divide and conquer approach, we can solve this problem in O(n log n) time.
import math
def distance(p1, p2):
return math.sqrt((p1[0] - p2[0])**2 + (p1[1] - p2[1])**2)
def brute_force(points):
min_dist = float('inf')
for i in range(len(points)):
for j in range(i+1, len(points)):
dist = distance(points[i], points[j])
if dist < min_dist:
min_dist = dist
return min_dist
def strip_closest(strip, d):
min_dist = d
strip.sort(key=lambda point: point[1])
for i in range(len(strip)):
j = i + 1
while j < len(strip) and (strip[j][1] - strip[i][1]) < min_dist:
dist = distance(strip[i], strip[j])
if dist < min_dist:
min_dist = dist
j += 1
return min_dist
def closest_pair(points):
n = len(points)
if n <= 3:
return brute_force(points)
mid = n // 2
mid_point = points[mid]
left = points[:mid]
right = points[mid:]
d1 = closest_pair(left)
d2 = closest_pair(right)
d = min(d1, d2)
strip = [p for p in points if abs(p[0] - mid_point[0]) < d]
return min(d, strip_closest(strip, d))
# Example usage
points = [(2, 3), (12, 30), (40, 50), (5, 1), (12, 10), (3, 4)]
points.sort() # Sort points based on x-coordinate
min_distance = closest_pair(points)
print(f"The smallest distance is {min_distance}")
This algorithm demonstrates how divide and conquer can be applied to geometric problems, significantly improving efficiency over naive approaches.
2. The Skyline Problem
The Skyline Problem is a classic algorithmic challenge that involves determining the skyline of a city given a set of buildings. This problem can be efficiently solved using a divide and conquer approach.
def merge_skylines(left, right):
result = []
h1, h2 = 0, 0
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i][0] < right[j][0]:
x = left[i][0]
h1 = left[i][1]
max_h = max(h1, h2)
result.append([x, max_h])
i += 1
elif right[j][0] < left[i][0]:
x = right[j][0]
h2 = right[j][1]
max_h = max(h1, h2)
result.append([x, max_h])
j += 1
else:
x = left[i][0]
h1 = left[i][1]
h2 = right[j][1]
max_h = max(h1, h2)
result.append([x, max_h])
i += 1
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
def get_skyline(buildings):
if not buildings:
return []
if len(buildings) == 1:
left, height, right = buildings[0]
return [[left, height], [right, 0]]
mid = len(buildings) // 2
left_skyline = get_skyline(buildings[:mid])
right_skyline = get_skyline(buildings[mid:])
return merge_skylines(left_skyline, right_skyline)
# Example usage
buildings = [[2,9,10], [3,7,15], [5,12,12], [15,20,10], [19,24,8]]
skyline = get_skyline(buildings)
print("The skyline is:", skyline)
This solution demonstrates how divide and conquer can be applied to complex geometric problems, breaking down the skyline calculation into smaller subproblems and efficiently merging the results.
Optimizing Divide and Conquer Algorithms
While divide and conquer algorithms are inherently efficient, there are several strategies to further optimize their performance:
1. Tail Recursion Optimization
Many divide and conquer algorithms use recursion, which can lead to stack overflow for very large inputs. Tail recursion optimization can help mitigate this issue:
def tail_recursive_binary_search(arr, target, low=0, high=None):
if high is None:
high = len(arr) - 1
if low > high:
return -1
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] > target:
return tail_recursive_binary_search(arr, target, low, mid-1)
else:
return tail_recursive_binary_search(arr, target, mid+1, high)
# Example usage
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = tail_recursive_binary_search(arr, target)
print(f"Target found at index: {result}")
2. Iterative Implementations
In some cases, converting a recursive divide and conquer algorithm to an iterative version can improve performance by reducing function call overhead:
def iterative_merge_sort(arr):
width = 1
n = len(arr)
temp = [0] * n
while width < n:
for i in range(0, n, 2*width):
left = i
mid = min(i + width, n)
right = min(i + 2*width, n)
merge(arr, left, mid, right, temp)
arr, temp = temp, arr
width *= 2
return arr
def merge(arr, left, mid, right, temp):
i, j, k = left, mid, left
while i < mid and j < right:
if arr[i] <= arr[j]:
temp[k] = arr[i]
i += 1
else:
temp[k] = arr[j]
j += 1
k += 1
while i < mid:
temp[k] = arr[i]
i += 1
k += 1
while j < right:
temp[k] = arr[j]
j += 1
k += 1
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = iterative_merge_sort(arr)
print("Sorted array:", sorted_arr)
3. Hybrid Algorithms
Combining divide and conquer with other algorithmic paradigms can lead to highly optimized solutions. For example, Introsort, used in many standard library implementations of sorting algorithms, combines Quicksort with Heapsort:
import math
def introsort(arr):
max_depth = 2 * math.floor(math.log2(len(arr)))
introsort_helper(arr, 0, len(arr) - 1, max_depth)
return arr
def introsort_helper(arr, start, end, max_depth):
if end - start <= 16:
insertion_sort(arr, start, end)
elif max_depth == 0:
heapsort(arr, start, end)
else:
p = partition(arr, start, end)
introsort_helper(arr, start, p - 1, max_depth - 1)
introsort_helper(arr, p + 1, end, max_depth - 1)
def insertion_sort(arr, start, end):
for i in range(start + 1, end + 1):
key = arr[i]
j = i - 1
while j >= start and arr[j] > key:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
def heapsort(arr, start, end):
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
n = end - start + 1
for i in range(n // 2 - 1, -1, -1):
heapify(arr[start:end+1], n, i)
for i in range(n - 1, 0, -1):
arr[start], arr[start + i] = arr[start + i], arr[start]
heapify(arr[start:start+i], i, 0)
def partition(arr, low, high):
pivot = arr[high]
i = low - 1
for j in range(low, high):
if arr[j] <= pivot:
i += 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return i + 1
# Example usage
arr = [12, 11, 13, 5, 6, 7]
sorted_arr = introsort(arr)
print("Sorted array:", sorted_arr)
Conclusion: The Ongoing Relevance of Divide and Conquer
As we’ve explored throughout this article, divide and conquer algorithms remain a cornerstone of efficient problem-solving in computer science. From classic sorting and searching algorithms to advanced applications in computational geometry and beyond, this paradigm offers a powerful approach to tackling complex problems.
For aspiring programmers and those preparing for technical interviews, especially at top tech companies, a deep understanding of divide and conquer is invaluable. It not only helps in solving specific algorithmic challenges but also cultivates a problem-solving mindset that’s crucial in software development.
As you continue your journey in programming and algorithm design, remember that divide and conquer is more than just a set of algorithms—it’s a way of thinking about problems. By breaking down complex issues into manageable parts, you’ll be better equipped to tackle the challenges of modern software development, whether you’re optimizing performance-critical code or designing scalable systems.
Keep practicing, experimenting with different divide and conquer applications, and don’t hesitate to apply this powerful paradigm to new and unique problems. The ability to effectively divide and conquer will serve you well throughout your programming career, helping you stand out in technical interviews and excel in real-world software development challenges.