Sorting Algorithms Explained: From Bubble Sort to Quick Sort
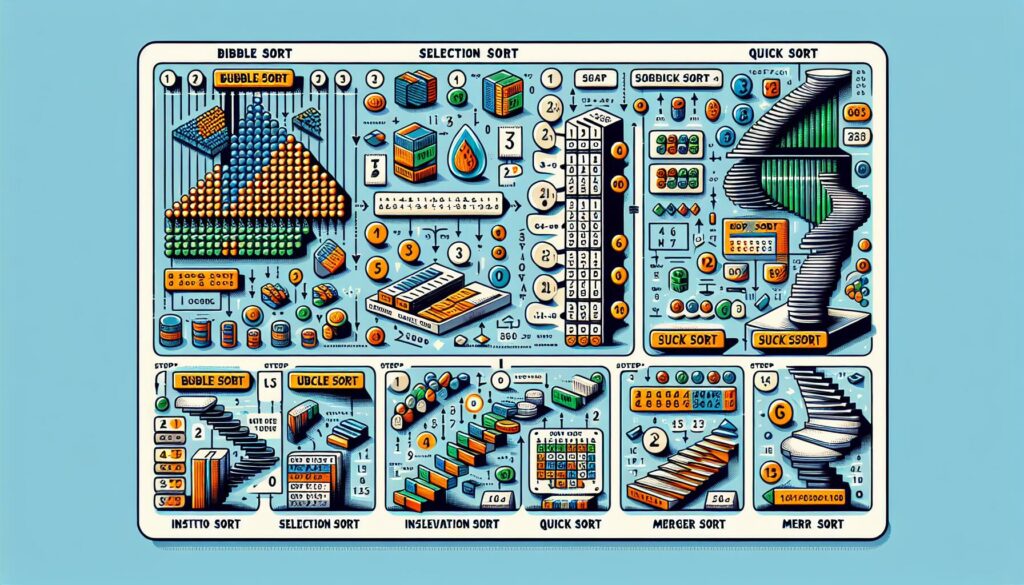
Sorting algorithms are fundamental concepts in computer science and play a crucial role in organizing data efficiently. Whether you’re a beginner programmer or preparing for technical interviews at top tech companies, understanding these algorithms is essential. In this comprehensive guide, we’ll explore various sorting algorithms, from the simple but inefficient Bubble Sort to the more advanced and widely-used Quick Sort.
Why Are Sorting Algorithms Important?
Before diving into the specifics of each algorithm, let’s consider why sorting algorithms are so important:
- Data Organization: Sorting algorithms help organize data in a specific order, making it easier to search, retrieve, and analyze information.
- Efficiency: Different sorting algorithms have varying levels of efficiency, which can significantly impact the performance of larger applications.
- Problem-Solving Skills: Understanding sorting algorithms enhances your problem-solving abilities and algorithmic thinking.
- Interview Preparation: Sorting algorithms are a common topic in technical interviews, especially for positions at major tech companies.
1. Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
How Bubble Sort Works
- Start with the first element of the array.
- Compare it with the next element.
- If the first element is greater than the second, swap them.
- Move to the next pair of adjacent elements and repeat steps 2-3.
- Continue this process until you reach the end of the array.
- Repeat steps 1-5 for each pass through the array until no more swaps are needed.
Bubble Sort Implementation in Python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(unsorted_array)
print(sorted_array) # Output: [11, 12, 22, 25, 34, 64, 90]
Time Complexity
- Best Case: O(n) when the array is already sorted
- Average Case: O(n²)
- Worst Case: O(n²)
Bubble Sort is not efficient for large datasets but can be useful for small lists or as an educational tool to understand the basics of sorting algorithms.
2. Selection Sort
Selection Sort is an in-place comparison sorting algorithm. It divides the input list into two parts: a sorted portion at the left end and an unsorted portion at the right end. Initially, the sorted portion is empty, and the unsorted portion is the entire list.
How Selection Sort Works
- Find the smallest element in the unsorted portion of the array.
- Swap it with the first element of the unsorted portion.
- Move the boundary between the sorted and unsorted portions one element to the right.
- Repeat steps 1-3 until the entire array is sorted.
Selection Sort Implementation in Python
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i + 1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
unsorted_array = [64, 25, 12, 22, 11]
sorted_array = selection_sort(unsorted_array)
print(sorted_array) # Output: [11, 12, 22, 25, 64]
Time Complexity
- Best Case: O(n²)
- Average Case: O(n²)
- Worst Case: O(n²)
Selection Sort performs the same number of comparisons regardless of the initial order of the elements, making it inefficient for large lists. However, it has the advantage of minimizing the number of swaps.
3. Insertion Sort
Insertion Sort is a simple sorting algorithm that builds the final sorted array one item at a time. It is much less efficient on large lists than more advanced algorithms such as Quick Sort, Heap Sort, or Merge Sort. However, it performs well for small datasets or partially sorted arrays.
How Insertion Sort Works
- Start with the second element of the array (assume the first element is already sorted).
- Compare the current element with the previous elements in the sorted portion.
- If the current element is smaller, shift the larger elements to the right.
- Insert the current element in its correct position in the sorted portion.
- Move to the next unsorted element and repeat steps 2-4 until the entire array is sorted.
Insertion Sort Implementation in Python
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
unsorted_array = [12, 11, 13, 5, 6]
sorted_array = insertion_sort(unsorted_array)
print(sorted_array) # Output: [5, 6, 11, 12, 13]
Time Complexity
- Best Case: O(n) when the array is already sorted
- Average Case: O(n²)
- Worst Case: O(n²)
Insertion Sort is efficient for small datasets and performs well on partially sorted arrays. It’s also an online algorithm, meaning it can sort a list as it receives it.
4. Merge Sort
Merge Sort is an efficient, stable, and comparison-based sorting algorithm. It uses a divide-and-conquer strategy to sort the elements. Merge Sort is often preferred for sorting linked lists and is used in external sorting.
How Merge Sort Works
- Divide the unsorted list into n sublists, each containing one element (a list of one element is considered sorted).
- Repeatedly merge sublists to produce new sorted sublists until there is only one sublist remaining. This will be the sorted list.
Merge Sort Implementation in Python
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] <= R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
# Example usage
unsorted_array = [38, 27, 43, 3, 9, 82, 10]
sorted_array = merge_sort(unsorted_array)
print(sorted_array) # Output: [3, 9, 10, 27, 38, 43, 82]
Time Complexity
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n log n)
Merge Sort has a consistent time complexity of O(n log n), making it efficient for large datasets. However, it requires additional space proportional to the size of the input array.
5. Quick Sort
Quick Sort is a highly efficient sorting algorithm and is based on the divide-and-conquer approach. It’s widely used and is often the go-to sorting algorithm for many applications due to its performance.
How Quick Sort Works
- Choose a ‘pivot’ element from the array.
- Partition the other elements into two sub-arrays, according to whether they are less than or greater than the pivot.
- Recursively apply the above steps to the sub-arrays.
Quick Sort Implementation in Python
def quick_sort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
unsorted_array = [3, 6, 8, 10, 1, 2, 1]
sorted_array = quick_sort(unsorted_array)
print(sorted_array) # Output: [1, 1, 2, 3, 6, 8, 10]
Time Complexity
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n²) – occurs when the pivot is always the smallest or largest element
Quick Sort is generally considered the fastest sorting algorithm in practice. Its performance can be improved by using techniques like choosing a random pivot or using the median-of-three method for pivot selection.
Comparison of Sorting Algorithms
Algorithm | Time Complexity (Best) | Time Complexity (Average) | Time Complexity (Worst) | Space Complexity | Stability |
---|---|---|---|---|---|
Bubble Sort | O(n) | O(n²) | O(n²) | O(1) | Stable |
Selection Sort | O(n²) | O(n²) | O(n²) | O(1) | Not Stable |
Insertion Sort | O(n) | O(n²) | O(n²) | O(1) | Stable |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) | Stable |
Quick Sort | O(n log n) | O(n log n) | O(n²) | O(log n) | Not Stable |
Choosing the Right Sorting Algorithm
Selecting the appropriate sorting algorithm depends on various factors:
- Size of the dataset: For small datasets, simpler algorithms like Insertion Sort may be sufficient. For larger datasets, more efficient algorithms like Merge Sort or Quick Sort are preferred.
- Partially sorted data: If the data is already partially sorted, algorithms like Insertion Sort can perform well.
- Stability requirement: If maintaining the relative order of equal elements is important, choose a stable sorting algorithm like Merge Sort.
- Memory constraints: In-place sorting algorithms like Quick Sort are preferable when memory is limited.
- Time complexity: For consistently good performance across different scenarios, algorithms with O(n log n) complexity like Merge Sort or Quick Sort are often chosen.
Advanced Sorting Algorithms
While we’ve covered the most common sorting algorithms, there are several advanced algorithms worth mentioning:
- Heap Sort: Uses a binary heap data structure to sort elements. It has a time complexity of O(n log n) for all cases.
- Counting Sort: Efficient for sorting integers with a small range. It has a linear time complexity of O(n + k), where k is the range of input.
- Radix Sort: Sorts integers by processing each digit. It has a time complexity of O(d(n + k)), where d is the number of digits and k is the range of digits.
- Tim Sort: A hybrid sorting algorithm derived from Merge Sort and Insertion Sort, used as the default sorting algorithm in Python’s sorted() function and Java’s Arrays.sort() for objects.
Practical Applications of Sorting Algorithms
Understanding sorting algorithms is crucial for several reasons:
- Database Management: Efficient sorting is essential for database operations, especially when dealing with large datasets.
- Search Algorithms: Many search algorithms require sorted data to function efficiently, such as binary search.
- Data Analysis: Sorting is often a preprocessing step in data analysis and visualization tasks.
- Computer Graphics: Sorting algorithms are used in computer graphics for tasks like rendering objects in the correct order.
- Operating Systems: Process scheduling in operating systems often involves sorting tasks based on priority.
Tips for Mastering Sorting Algorithms
- Understand the basics: Start with simpler algorithms like Bubble Sort and Insertion Sort to grasp the fundamental concepts.
- Visualize the process: Use online visualization tools or create your own diagrams to understand how each algorithm works step-by-step.
- Implement from scratch: Try coding each algorithm without referring to existing implementations to deepen your understanding.
- Analyze time and space complexity: Practice calculating the time and space complexity for different input scenarios.
- Compare algorithms: Implement multiple sorting algorithms and compare their performance on various datasets.
- Practice with variations: Try sorting in descending order or sorting objects based on multiple criteria.
- Solve related problems: Work on problems that involve sorting as a subtask to see how these algorithms are applied in broader contexts.
Conclusion
Sorting algorithms are a fundamental part of computer science and are essential for efficient data manipulation and analysis. From the simple Bubble Sort to the more advanced Quick Sort, each algorithm has its strengths and use cases. Understanding these algorithms not only prepares you for technical interviews but also enhances your problem-solving skills and algorithmic thinking.
As you continue your journey in programming and computer science, remember that mastering sorting algorithms is just one step. The principles behind these algorithms – divide and conquer, in-place operations, and efficiency trade-offs – apply to many other areas of software development and problem-solving.
Keep practicing, experimenting with different datasets, and challenging yourself to optimize your implementations. With time and experience, you’ll develop an intuition for choosing the right algorithm for each situation, a skill that’s invaluable in any programming career.