Optimized Coding Interview Preparation: A Comprehensive Guide
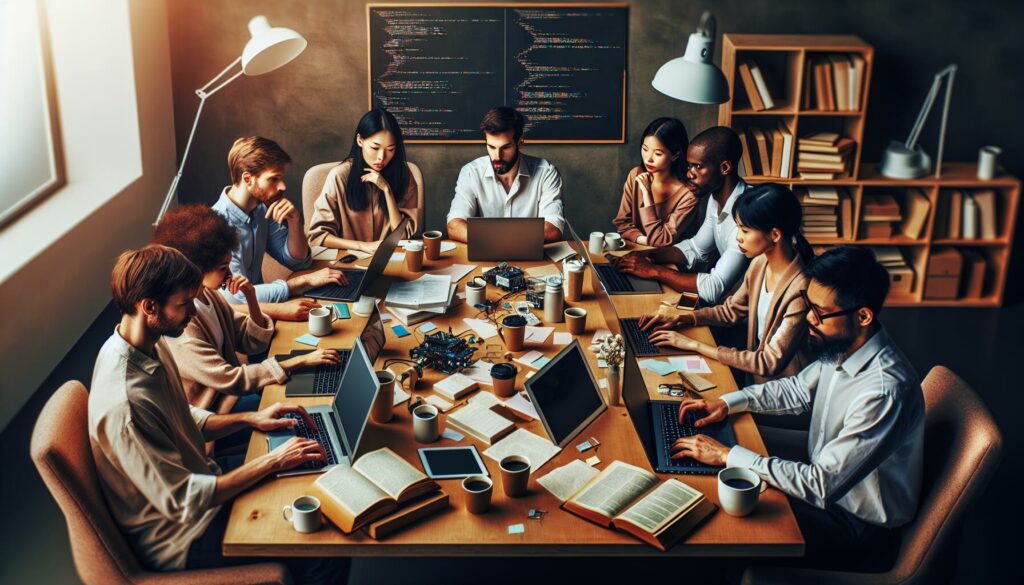
In today’s competitive tech landscape, mastering coding interviews is crucial for landing your dream job at top companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) and beyond. This comprehensive guide will walk you through the essential steps and strategies for optimized coding interview preparation, helping you stand out from the crowd and ace your technical interviews.
Table of Contents
- Understanding Coding Interviews
- Core Concepts to Master
- Developing Problem-Solving Skills
- Effective Practice Strategies
- Essential Data Structures
- Key Algorithms to Know
- Understanding Time and Space Complexity
- Leveraging Coding Platforms
- Conducting Mock Interviews
- Developing Soft Skills
- Preparing for Interview Day
- Continuous Learning and Improvement
1. Understanding Coding Interviews
Before diving into preparation strategies, it’s essential to understand the structure and purpose of coding interviews. These interviews are designed to assess your problem-solving skills, coding ability, and thought process rather than just testing your memorization of algorithms.
Typical coding interviews consist of:
- Introduction and brief chat about your background
- One or more coding problems to solve
- Discussion of your approach and potential optimizations
- Questions about your solution and its efficiency
- Time for you to ask questions about the company or role
Understanding this structure helps you prepare more effectively and feel more confident during the actual interview.
2. Core Concepts to Master
To excel in coding interviews, you need a strong foundation in core computer science concepts. Focus on mastering the following areas:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Recursion, Dynamic Programming, Greedy Algorithms
- Object-Oriented Programming (OOP) principles
- Time and Space Complexity analysis
- Basic design patterns
- Database concepts and SQL basics
- Operating Systems fundamentals
- Networking basics
While you may not encounter all of these topics in every interview, having a solid understanding of these concepts will give you the confidence to tackle a wide range of problems.
3. Developing Problem-Solving Skills
Problem-solving is at the heart of coding interviews. To improve your problem-solving skills, follow these steps:
- Understand the problem: Read the question carefully and ask clarifying questions if needed.
- Break it down: Divide the problem into smaller, manageable sub-problems.
- Plan your approach: Outline your solution before writing any code.
- Implement your solution: Write clean, organized code to solve the problem.
- Test and debug: Run through test cases and fix any issues.
- Optimize: Consider ways to improve the efficiency of your solution.
Practice this approach consistently to internalize the problem-solving process and apply it effortlessly during interviews.
4. Effective Practice Strategies
Consistent practice is key to improving your coding interview skills. Here are some effective strategies to optimize your practice sessions:
- Solve a diverse range of problems: Don’t stick to one type of problem. Expose yourself to various difficulties and topics.
- Time yourself: Practice solving problems within a time limit to simulate interview conditions.
- Write code on paper or a whiteboard: Many interviews still use these methods, so get comfortable coding without an IDE.
- Explain your thought process out loud: This helps you practice articulating your approach, which is crucial during interviews.
- Review and learn from others’ solutions: After solving a problem, study other efficient solutions to broaden your perspective.
- Consistent daily practice: Aim for at least one problem a day, even if it’s a simple one.
Remember, quality practice is more important than quantity. Focus on understanding and learning from each problem rather than rushing through as many as possible.
5. Essential Data Structures
A solid understanding of data structures is crucial for coding interviews. Here’s a brief overview of the most important ones:
Arrays
Arrays are the most basic and widely used data structure. They offer constant-time access to elements but can be inefficient for insertions and deletions, especially at the beginning of the array.
Linked Lists
Linked lists consist of nodes where each node contains a data field and a reference to the next node. They’re efficient for insertions and deletions but less so for random access.
Stacks and Queues
Stacks follow the Last-In-First-Out (LIFO) principle, while queues follow the First-In-First-Out (FIFO) principle. Both are useful for managing data with specific access patterns.
Trees
Trees are hierarchical structures with a root node and child nodes. Binary trees, binary search trees, and balanced trees like AVL and Red-Black trees are commonly used in interviews.
Graphs
Graphs represent relationships between objects and are crucial for solving network, social connection, and pathfinding problems.
Hash Tables
Hash tables provide fast insertion, deletion, and lookup operations, making them excellent for problems requiring quick data retrieval.
For each data structure, make sure you understand:
- Basic operations and their time complexities
- Common use cases
- Advantages and disadvantages
- Implementation details in your preferred programming language
6. Key Algorithms to Know
While it’s impossible to memorize every algorithm, understanding these key algorithms will give you a strong foundation:
Sorting Algorithms
- Quick Sort
- Merge Sort
- Heap Sort
- Bubble Sort (for understanding, not practical use)
Searching Algorithms
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Graph Algorithms
- Dijkstra’s Algorithm
- Floyd-Warshall Algorithm
- Kruskal’s Algorithm
- Prim’s Algorithm
Dynamic Programming
Understanding the concept of dynamic programming and being able to identify problems where it can be applied is crucial. Practice classic dynamic programming problems like:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack Problem
String Manipulation Algorithms
- KMP Algorithm
- Rabin-Karp Algorithm
For each algorithm, focus on:
- The problem it solves
- How it works (step-by-step)
- Time and space complexity
- Implementation in your preferred language
- Common variations and applications
7. Understanding Time and Space Complexity
Analyzing the efficiency of your solutions is a critical skill in coding interviews. You should be comfortable with:
Big O Notation
Big O notation is used to describe the upper bound of the time or space complexity of an algorithm. Common complexities include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
- O(2^n): Exponential time
Analyzing Your Solutions
For each solution you develop, practice:
- Identifying the time complexity
- Calculating the space complexity
- Explaining why the complexity is what it is
- Discussing potential trade-offs between time and space
Optimization Techniques
Learn common optimization techniques such as:
- Using appropriate data structures
- Avoiding unnecessary computations
- Applying caching or memoization
- Utilizing space to improve time complexity
Being able to analyze and optimize your solutions will set you apart in coding interviews and demonstrate your ability to write efficient, scalable code.
8. Leveraging Coding Platforms
Online coding platforms are invaluable resources for interview preparation. They offer a wide range of problems, editorial solutions, and a community of learners. Some popular platforms include:
- LeetCode: Offers a vast collection of coding problems, many of which are commonly asked in tech interviews.
- HackerRank: Provides a mix of algorithmic challenges and skill-based assessments.
- CodeSignal: Offers coding challenges and also hosts coding competitions.
- AlgoExpert: Provides curated lists of coding problems with video explanations.
- Project Euler: Focuses on mathematical and computer programming problems.
When using these platforms:
- Start with easier problems and gradually increase difficulty
- Focus on understanding the problem-solving approach rather than just getting the right answer
- Participate in coding contests to practice solving problems under time pressure
- Engage with the community by reading discussions and sharing your own solutions
Remember, while these platforms are excellent for practice, they shouldn’t be your only method of preparation. Complement them with other resources and real-world coding projects.
9. Conducting Mock Interviews
Mock interviews are crucial for simulating real interview conditions and getting comfortable with the process. Here’s how to make the most of mock interviews:
Finding Mock Interview Partners
- Reach out to friends or colleagues in the tech industry
- Join coding communities or study groups
- Use platforms like Pramp or InterviewBit that offer peer-to-peer mock interviews
Structuring Mock Interviews
- Choose a realistic problem that could be asked in an actual interview
- Set a time limit (usually 45-60 minutes)
- Have the interviewee solve the problem while thinking aloud
- The interviewer should provide hints if the interviewee gets stuck
- Discuss the solution and provide feedback at the end
Alternating Roles
Take turns being the interviewer and interviewee. Being an interviewer helps you understand the perspective of the person assessing you and can provide insights into what makes a good candidate.
Seeking Feedback
After each mock interview, ask for detailed feedback on:
- Problem-solving approach
- Code quality and efficiency
- Communication skills
- Areas for improvement
Regular mock interviews will help you become more comfortable with the interview process, improve your ability to solve problems under pressure, and refine your communication skills.
10. Developing Soft Skills
While technical skills are crucial, soft skills can often make the difference in coding interviews. Focus on developing these key soft skills:
Communication
- Practice explaining your thought process clearly and concisely
- Learn to ask clarifying questions about the problem
- Improve your ability to discuss trade-offs in your solutions
Collaboration
- Show willingness to consider alternative approaches
- Demonstrate how you would work as part of a team
- Be open to feedback and suggestions
Problem-solving Mindset
- Stay calm under pressure
- Demonstrate persistence when faced with challenging problems
- Show enthusiasm for tackling complex issues
Adaptability
- Be ready to pivot your approach if your initial solution isn’t optimal
- Show willingness to learn new concepts quickly
Develop these skills by practicing them during mock interviews, participating in coding challenges, and working on collaborative projects.
11. Preparing for Interview Day
As your interview day approaches, follow these tips to ensure you’re fully prepared:
Technical Preparation
- Review your notes on key algorithms and data structures
- Solve a few easy problems to warm up your coding skills
- Prepare a cheat sheet of common problem-solving patterns
Logistics
- Confirm the interview time and format (in-person or virtual)
- Test your equipment if it’s a virtual interview
- Prepare your coding environment (IDE, compiler, etc.)
- Have a backup plan in case of technical issues
Mental Preparation
- Get a good night’s sleep
- Eat a healthy meal before the interview
- Practice relaxation techniques to manage stress
- Review your achievements to boost confidence
During the Interview
- Listen carefully to the problem statement
- Ask clarifying questions if needed
- Think aloud as you solve the problem
- Stay calm if you get stuck – it’s okay to ask for hints
- Be prepared to optimize your initial solution
Remember, the interviewer is not just assessing your coding skills but also evaluating how you approach problems and how you’d fit into their team.
12. Continuous Learning and Improvement
The tech industry is constantly evolving, and so should your skills. Here are some strategies for continuous learning and improvement:
Stay Updated with Industry Trends
- Follow tech blogs and news sites
- Attend tech conferences or webinars
- Participate in online tech communities
Expand Your Skill Set
- Learn new programming languages
- Explore different areas of computer science (e.g., machine learning, cloud computing)
- Work on personal projects to apply your skills
Contribute to Open Source
- Contribute to open-source projects on GitHub
- Collaborate with developers from around the world
- Gain experience working on large-scale projects
Teach Others
- Start a tech blog or YouTube channel
- Mentor junior developers
- Participate in coding forums to help others
Reflect and Improve
- Regularly assess your strengths and weaknesses
- Set specific learning goals and track your progress
- Seek feedback from peers and mentors
By committing to continuous learning, you’ll not only prepare yourself for coding interviews but also for a successful long-term career in tech.
Conclusion
Preparing for coding interviews is a challenging but rewarding process. By following this comprehensive guide, you’ll be well-equipped to tackle even the most demanding technical interviews. Remember that success in coding interviews comes from a combination of strong technical skills, effective problem-solving abilities, and excellent communication.
Key takeaways:
- Master core computer science concepts and data structures
- Develop strong problem-solving skills through consistent practice
- Utilize online coding platforms and participate in mock interviews
- Focus on both technical skills and soft skills
- Prepare thoroughly for interview day
- Commit to continuous learning and improvement
With dedication and the right approach, you can significantly improve your chances of success in coding interviews and land your dream job in the tech industry. Good luck with your interview preparation!