Mastering Algorithmic Problem-Solving: A Comprehensive Guide
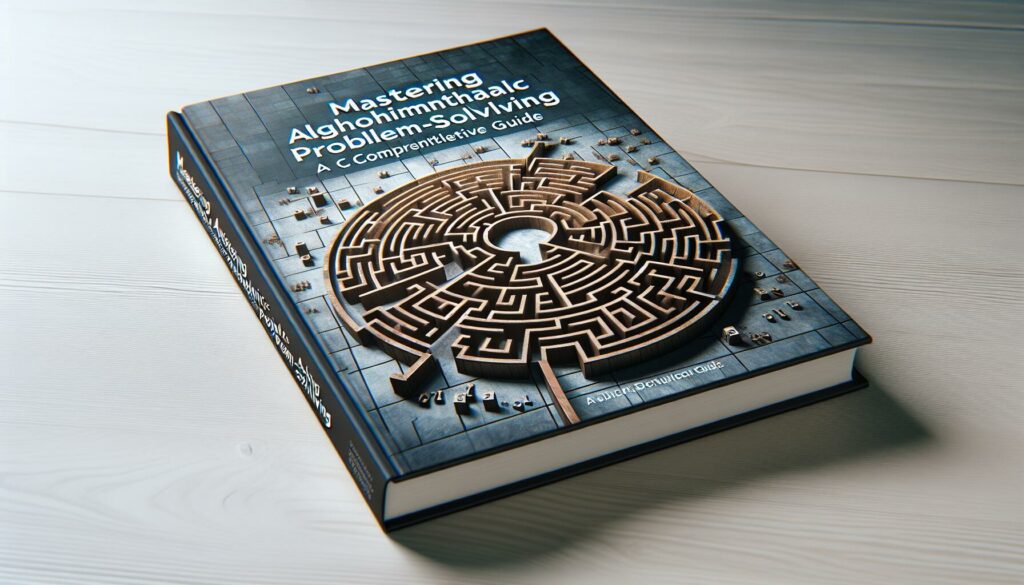
In the ever-evolving world of technology, algorithmic problem-solving stands as a cornerstone skill for programmers, software engineers, and computer scientists. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, honing your algorithmic thinking is crucial. This comprehensive guide will dive deep into the art and science of algorithmic problem-solving, providing you with the knowledge and strategies to excel in this critical area.
Understanding Algorithmic Problem-Solving
Before we delve into the specifics, let’s establish a clear understanding of what algorithmic problem-solving entails. At its core, algorithmic problem-solving is the process of identifying a problem, breaking it down into manageable steps, and devising a systematic approach to solve it efficiently using a set of well-defined instructions or algorithms.
This approach is fundamental in computer science and is essential for several reasons:
- It forms the basis of efficient software development
- It’s crucial for optimizing code performance
- It’s a key skill assessed in technical interviews, especially at major tech companies
- It enhances logical thinking and analytical skills
The Problem-Solving Process
Effective algorithmic problem-solving typically follows a structured process. Let’s break it down into steps:
1. Understand the Problem
The first step is to thoroughly understand the problem at hand. This involves:
- Carefully reading the problem statement
- Identifying the inputs and expected outputs
- Recognizing any constraints or special conditions
- Asking clarifying questions if anything is unclear
2. Plan the Approach
Once you understand the problem, it’s time to plan your approach. This step includes:
- Breaking down the problem into smaller, manageable sub-problems
- Identifying relevant data structures and algorithms
- Sketching out a high-level solution
- Considering multiple approaches and their trade-offs
3. Implement the Solution
With a plan in place, you can start implementing your solution:
- Write clean, readable code
- Use meaningful variable and function names
- Implement the core logic step by step
- Add comments to explain complex parts of your code
4. Test and Debug
After implementation, it’s crucial to test your solution:
- Start with simple test cases
- Progress to more complex and edge cases
- Use debugging tools to identify and fix any issues
- Verify that your solution meets all requirements
5. Optimize and Refine
Finally, look for ways to optimize your solution:
- Analyze the time and space complexity
- Identify areas for improvement in efficiency
- Refactor code for better readability and maintainability
- Consider alternative approaches that might be more optimal
Essential Algorithmic Concepts
To excel in algorithmic problem-solving, you need to be well-versed in several key concepts. Let’s explore some of the most important ones:
1. Time and Space Complexity
Understanding the efficiency of your algorithms is crucial. Time complexity refers to how the runtime of an algorithm grows with the input size, while space complexity deals with the amount of memory used. The Big O notation is commonly used to express these complexities.
For example, consider a simple linear search algorithm:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
This algorithm has a time complexity of O(n), where n is the length of the array, because in the worst case, it might need to check every element.
2. Data Structures
Choosing the right data structure can significantly impact the efficiency of your algorithm. Some essential data structures include:
- Arrays and Lists
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees, AVL Trees)
- Graphs
- Heaps
Each data structure has its strengths and is suited for different types of problems. For instance, a hash table provides O(1) average-case time complexity for insertions and lookups, making it ideal for problems requiring fast data retrieval.
3. Sorting and Searching Algorithms
Efficient sorting and searching are fundamental to many algorithmic solutions. Key algorithms in this category include:
- Bubble Sort, Selection Sort, Insertion Sort
- Merge Sort, Quick Sort
- Binary Search
- Depth-First Search (DFS) and Breadth-First Search (BFS) for graphs
Here’s a simple implementation of binary search in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
4. Dynamic Programming
Dynamic programming is a powerful technique for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems. The key idea is to store the results of subproblems to avoid redundant computations.
A classic example is the Fibonacci sequence. Here’s a dynamic programming approach:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
5. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While they don’t always yield the best solution, they’re often simple and efficient for certain types of problems.
An example of a greedy algorithm is the coin change problem, where you need to make change with the fewest number of coins:
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
Problem-Solving Strategies
Now that we’ve covered some essential concepts, let’s discuss strategies that can help you approach algorithmic problems more effectively:
1. Break It Down
When faced with a complex problem, break it down into smaller, more manageable sub-problems. This approach, often called “divide and conquer,” can make seemingly insurmountable challenges much more approachable.
2. Look for Patterns
Many algorithmic problems have underlying patterns. Try to identify these patterns and see if you can leverage them in your solution. For instance, many string manipulation problems can be solved efficiently using sliding window techniques.
3. Use Visualization
Drawing diagrams or visualizing the problem can often lead to insights that aren’t immediately apparent from the problem description. This is particularly useful for graph and tree problems.
4. Start with Brute Force
If you’re stuck, start with a brute force solution. While it may not be efficient, it can help you understand the problem better and potentially lead to optimizations.
5. Think About Edge Cases
Consider edge cases early in your problem-solving process. These might include empty inputs, extremely large inputs, or other special scenarios that could break a naive implementation.
6. Learn from Similar Problems
Many algorithmic problems are variations on classic themes. By studying and understanding common problem types, you’ll be better equipped to tackle new challenges.
Common Problem Types and Approaches
Let’s explore some common types of algorithmic problems and approaches to solving them:
1. Two Pointer Technique
This technique is often used for array or string problems. It involves maintaining two pointers that traverse the data structure, often in opposite directions or at different speeds.
Example: Finding a pair of numbers in a sorted array that sum to a target value.
def find_pair_with_sum(arr, target):
left, right = 0, len(arr) - 1
while left < right:
current_sum = arr[left] + arr[right]
if current_sum == target:
return [arr[left], arr[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return None
2. Sliding Window
This technique is useful for problems involving subarrays or substrings. It maintains a “window” that slides over the data, expanding or contracting as needed.
Example: Finding the longest substring with at most k distinct characters.
from collections import defaultdict
def longest_substring_with_k_distinct(s, k):
char_count = defaultdict(int)
max_length = start = 0
for end in range(len(s)):
char_count[s[end]] += 1
while len(char_count) > k:
char_count[s[start]] -= 1
if char_count[s[start]] == 0:
del char_count[s[start]]
start += 1
max_length = max(max_length, end - start + 1)
return max_length
3. Binary Search Variations
Binary search can be applied to more than just searching in sorted arrays. It’s often useful for problems where you need to find a specific value that satisfies certain conditions.
Example: Finding the square root of a number (rounded down to the nearest integer).
def square_root(x):
if x < 2:
return x
left, right = 2, x // 2
while left <= right:
mid = left + (right - left) // 2
square = mid * mid
if square == x:
return mid
elif square < x:
left = mid + 1
else:
right = mid - 1
return right
4. Graph Traversal
Many problems can be modeled as graphs, and understanding graph traversal algorithms is crucial. The two primary traversal methods are Depth-First Search (DFS) and Breadth-First Search (BFS).
Example: Implementing DFS to detect a cycle in a directed graph.
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.graph = defaultdict(list)
self.V = vertices
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic_util(self, v, visited, rec_stack):
visited[v] = True
rec_stack[v] = True
for neighbor in self.graph[v]:
if not visited[neighbor]:
if self.is_cyclic_util(neighbor, visited, rec_stack):
return True
elif rec_stack[neighbor]:
return True
rec_stack[v] = False
return False
def is_cyclic(self):
visited = [False] * self.V
rec_stack = [False] * self.V
for node in range(self.V):
if not visited[node]:
if self.is_cyclic_util(node, visited, rec_stack):
return True
return False
5. Dynamic Programming
Dynamic programming is particularly useful for optimization problems. The key is to identify overlapping subproblems and optimal substructure in the problem.
Example: Solving the classic “Longest Common Subsequence” problem.
def longest_common_subsequence(text1, text2):
m, n = len(text1), len(text2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if text1[i-1] == text2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
Preparing for Technical Interviews
Algorithmic problem-solving is a crucial component of technical interviews, especially at major tech companies. Here are some tips to help you prepare:
1. Practice Regularly
Consistent practice is key. Aim to solve at least one or two problems daily. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice with.
2. Focus on Understanding
Don’t just memorize solutions. Focus on understanding the underlying principles and patterns. This will help you apply your knowledge to new, unfamiliar problems.
3. Time Yourself
In interviews, you’ll often be working under time constraints. Practice solving problems within a set time limit to improve your speed and efficiency.
4. Explain Your Thought Process
Interviewers are often more interested in your problem-solving approach than the final solution. Practice explaining your thought process out loud as you solve problems.
5. Review and Reflect
After solving a problem, take time to review your solution. Look for ways to optimize it or solve it using different approaches. Reflect on what you learned from each problem.
6. Study Core Computer Science Concepts
While problem-solving is crucial, don’t neglect fundamental computer science concepts. Review topics like data structures, algorithms, operating systems, and databases.
7. Mock Interviews
Participate in mock interviews to simulate the real interview experience. This can help you get comfortable with explaining your solutions and handling pressure.
Conclusion
Mastering algorithmic problem-solving is a journey that requires dedication, practice, and continuous learning. By understanding fundamental concepts, applying effective problem-solving strategies, and regularly practicing with diverse problems, you can significantly improve your skills.
Remember, the goal is not just to solve problems, but to develop a systematic approach to tackling new challenges. As you progress, you’ll find that many seemingly complex problems can be broken down into familiar patterns and solved using the techniques you’ve learned.
Whether you’re preparing for technical interviews, looking to improve your coding skills, or simply enjoy the challenge of solving algorithmic puzzles, the skills you develop through this process will serve you well throughout your career in technology.
Keep practicing, stay curious, and don’t be afraid to tackle difficult problems. With time and effort, you’ll find yourself approaching even the most complex algorithmic challenges with confidence and skill.