Essential Coding Interview Tips: Ace Your Technical Interview
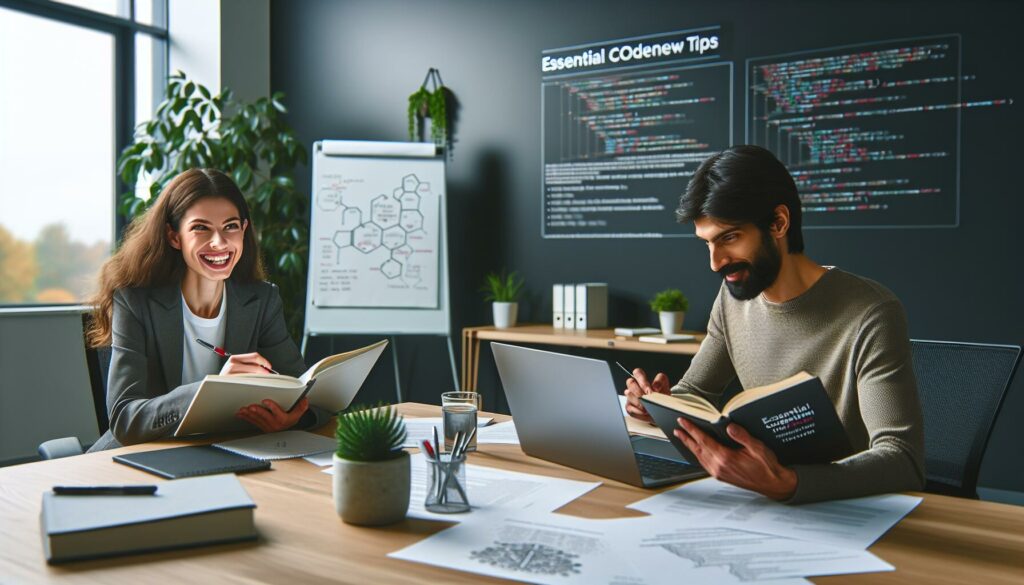
Are you preparing for a coding interview with a top tech company? Whether you’re eyeing a position at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other tech giant, mastering the art of coding interviews is crucial. In this comprehensive guide, we’ll walk you through essential coding interview tips that will help you showcase your skills, tackle challenging problems, and impress your interviewers.
Understanding the Coding Interview Process
Before diving into specific tips, it’s important to understand what to expect in a typical coding interview. Most technical interviews follow a similar structure:
- Introduction: A brief chat about your background and experience.
- Problem-solving: One or more coding challenges to assess your technical skills.
- Discussion: Explaining your approach and answering follow-up questions.
- Q&A: An opportunity for you to ask questions about the role and company.
With this structure in mind, let’s explore some key tips to help you excel in each phase of the interview.
1. Master the Fundamentals
Before you start practicing complex algorithms, ensure you have a solid grasp of fundamental data structures and algorithms. These include:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Recursion
Understanding these concepts thoroughly will provide you with a strong foundation to tackle a wide range of interview questions.
2. Practice, Practice, Practice
Consistent practice is key to improving your problem-solving skills. Here are some ways to incorporate regular practice into your preparation:
- Solve coding problems daily: Aim to solve at least one problem every day.
- Use online platforms: Websites like LeetCode, HackerRank, and AlgoCademy offer a vast array of coding challenges.
- Participate in coding contests: These can help you practice solving problems under time pressure.
- Mock interviews: Conduct practice interviews with friends or use platforms that offer mock interview services.
3. Develop a Problem-Solving Approach
Having a structured approach to problem-solving can help you tackle even the most challenging questions. Follow these steps:
- Understand the problem: Carefully read the question and ask clarifying questions if needed.
- Identify the inputs and outputs: Determine what data you’re working with and what result is expected.
- Consider edge cases: Think about potential special cases or extreme scenarios.
- Brainstorm solutions: Consider multiple approaches before settling on one.
- Explain your approach: Communicate your thought process to the interviewer.
- Implement the solution: Write clean, readable code to solve the problem.
- Test your code: Walk through your solution with sample inputs, including edge cases.
- Optimize if possible: Consider ways to improve the time or space complexity of your solution.
4. Communicate Effectively
Strong communication skills are just as important as technical prowess in a coding interview. Here’s how to communicate effectively:
- Think out loud: Share your thought process as you work through the problem.
- Ask questions: Don’t hesitate to seek clarification if something is unclear.
- Explain your decisions: Justify why you chose a particular approach or data structure.
- Be receptive to hints: If the interviewer offers a suggestion, consider it carefully.
- Stay calm and composed: Even if you’re struggling, maintain a positive attitude.
5. Write Clean and Efficient Code
Your coding style matters. Here are some tips for writing clean, efficient code during your interview:
- Use meaningful variable names: Choose descriptive names that convey the purpose of each variable.
- Keep your code modular: Break down complex problems into smaller, manageable functions.
- Comment your code: Add brief comments to explain complex logic or non-obvious decisions.
- Consider time and space complexity: Be prepared to discuss the efficiency of your solution.
- Follow coding conventions: Adhere to the standard style guidelines of the language you’re using.
Here’s an example of clean, well-commented code for a simple problem:
def is_palindrome(s: str) -> bool:
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(char.lower() for char in s if char.isalnum())
# Compare the string with its reverse
return cleaned_s == cleaned_s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
6. Know Your Resume Inside Out
Be prepared to discuss any project or technology mentioned in your resume in detail. Interviewers often use your resume as a starting point for technical discussions. For each project:
- Be ready to explain the problem it solved
- Discuss the technologies and algorithms used
- Highlight your specific contributions
- Be prepared to talk about challenges faced and how you overcame them
7. Understand Time and Space Complexity
A solid understanding of time and space complexity is crucial for coding interviews. Be prepared to:
- Analyze the time and space complexity of your solutions
- Discuss trade-offs between different approaches
- Optimize your code for better efficiency when possible
Here’s a quick reference for common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
8. Learn to Handle Difficult Questions
You may encounter questions that you’re not immediately sure how to solve. Here’s how to handle them:
- Stay calm: Don’t panic if you don’t immediately know the answer.
- Break it down: Try to split the problem into smaller, more manageable parts.
- Start with a brute force solution: Even if it’s not optimal, it shows you can approach the problem.
- Think aloud: Share your thoughts as you work through the problem.
- Be open to hints: If the interviewer offers a hint, use it to guide your thinking.
9. Prepare for System Design Questions
For more senior positions, you may encounter system design questions. These assess your ability to design large-scale systems. To prepare:
- Study common system design patterns and architectures
- Understand concepts like load balancing, caching, and database sharding
- Practice explaining complex systems in simple terms
- Be ready to make and justify design decisions
10. Familiarize Yourself with Behavioral Questions
While technical skills are crucial, many companies also assess your soft skills through behavioral questions. Prepare stories that demonstrate:
- Leadership and teamwork
- Problem-solving in non-technical contexts
- Handling conflicts or difficult situations
- Your passion for technology and learning
Use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
11. Stay Updated with Latest Technologies
The tech world evolves rapidly. Stay current with:
- New programming languages and frameworks
- Emerging technologies like AI, blockchain, or cloud computing
- Industry trends and news
This knowledge can be valuable in interviews and shows your passion for the field.
12. Brush Up on Computer Science Fundamentals
Don’t neglect the basics. Review key computer science concepts such as:
- Object-Oriented Programming principles
- Database fundamentals (SQL vs NoSQL, ACID properties)
- Basic networking concepts (TCP/IP, HTTP)
- Operating system basics
13. Use Pseudocode When Needed
If you’re struggling to implement a solution in code, start with pseudocode. This can help you:
- Organize your thoughts
- Communicate your approach to the interviewer
- Identify potential issues before diving into actual coding
Here’s an example of pseudocode for a binary search algorithm:
function binarySearch(array, target):
left = 0
right = length of array - 1
while left <= right:
mid = (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Target not found
14. Practice Coding Without an IDE
In many interviews, you won’t have access to an IDE with auto-completion and syntax highlighting. Practice coding on a simple text editor or even on paper to:
- Improve your ability to write code without relying on tools
- Enhance your understanding of syntax and language specifics
- Get comfortable with the interview environment
15. Prepare Relevant Questions for the Interviewer
At the end of the interview, you’ll usually have the chance to ask questions. Prepare thoughtful questions that demonstrate your interest in the role and the company. Some examples:
- “What are the biggest challenges facing the team right now?”
- “Can you describe the typical development cycle for a project?”
- “How does the company support professional growth and learning?”
- “What do you enjoy most about working here?”
16. Develop a Pre-Interview Routine
Establish a routine to help you feel prepared and confident on the day of your interview:
- Get a good night’s sleep
- Eat a healthy meal
- Review your notes, but don’t cram
- Prepare your space (for remote interviews) or your materials (for in-person interviews)
- Do some light coding exercises to warm up your mind
17. Learn from Each Interview Experience
Whether the interview goes well or not, treat each one as a learning experience:
- Reflect on what went well and what could be improved
- Make notes on questions you found challenging
- Seek feedback if possible
- Use these insights to refine your preparation for future interviews
Conclusion
Mastering coding interviews is a journey that requires dedication, practice, and continuous learning. By following these tips and consistently working on your skills, you’ll be well-prepared to tackle even the most challenging technical interviews. Remember, the goal is not just to solve problems, but to demonstrate your problem-solving approach, communication skills, and passion for technology.
As you prepare, take advantage of resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to advanced interview preparation. With the right preparation and mindset, you’ll be well on your way to landing your dream job in tech.
Good luck with your coding interviews!